Simple interface for Mbed Cloud Client
Embed:
(wiki syntax)
Show/hide line numbers
pal_rtos_test_utils.c
00001 /******************************************************************************* 00002 * Copyright 2016, 2017 ARM Ltd. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 *******************************************************************************/ 00016 00017 #include <stdio.h> 00018 #include "pal.h" 00019 #include "pal_rtos_test_utils.h" 00020 00021 #include "pal_BSP.h" 00022 #include "unity_fixture.h" 00023 00024 #include "pal.h" 00025 00026 00027 extern threadsArgument_t g_threadsArg; 00028 extern palThreadLocalStore_t g_threadStorage; 00029 timerArgument_t timerArgs; 00030 00031 void palThreadFunc1(void const *argument) 00032 { 00033 volatile palThreadID_t threadID; 00034 palThreadLocalStore_t * threadStorage = NULL; 00035 threadsArgument_t *tmp = (threadsArgument_t*)argument; 00036 #ifdef MUTEX_UNITY_TEST 00037 palStatus_t status = PAL_SUCCESS; 00038 PAL_PRINTF("palThreadFunc1::before mutex\n"); 00039 status = pal_osMutexWait(mutex1, 100); 00040 PAL_PRINTF("palThreadFunc1::after mutex: 0x%08x\n", status); 00041 PAL_PRINTF("palThreadFunc1::after mutex (expected): 0x%08x\n", PAL_ERR_RTOS_TIMEOUT ); 00042 TEST_ASSERT_EQUAL_HEX(PAL_ERR_RTOS_TIMEOUT , status); 00043 return; // for Mutex scenario, this should end here 00044 #endif //MUTEX_UNITY_TEST 00045 00046 tmp->arg1 = 10; 00047 threadID = pal_osThreadGetId(); 00048 TEST_ASSERT_NOT_EQUAL(threadID, NULLPTR); 00049 PAL_PRINTF("palThreadFunc1::Thread ID is %"PRIuPTR " \n", threadID); 00050 00051 threadStorage = pal_osThreadGetLocalStore(); 00052 if (threadStorage == &g_threadStorage) 00053 { 00054 PAL_PRINTF("Thread storage updated as expected\n"); 00055 } 00056 TEST_ASSERT_EQUAL_HEX((uintptr_t)threadStorage, (uintptr_t)(&g_threadStorage)); 00057 00058 #ifdef MUTEX_UNITY_TEST 00059 status = pal_osMutexRelease(mutex1); 00060 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00061 #endif //MUTEX_UNITY_TEST 00062 PAL_PRINTF("palThreadFunc1::STAAAAM\n"); 00063 } 00064 00065 void palThreadFunc2(void const *argument) 00066 { 00067 volatile palThreadID_t threadID; 00068 threadsArgument_t *tmp = (threadsArgument_t*)argument; 00069 #ifdef MUTEX_UNITY_TEST 00070 palStatus_t status = PAL_SUCCESS; 00071 PAL_PRINTF("palThreadFunc2::before mutex\n"); 00072 status = pal_osMutexWait(mutex2, 300); 00073 PAL_PRINTF("palThreadFunc2::after mutex: 0x%08x\n", status); 00074 PAL_PRINTF("palThreadFunc2::after mutex (expected): 0x%08x\n", PAL_SUCCESS); 00075 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00076 #endif //MUTEX_UNITY_TEST 00077 00078 tmp->arg2 = 20; 00079 threadID = pal_osThreadGetId(); 00080 TEST_ASSERT_NOT_EQUAL(threadID, NULLPTR); 00081 PAL_PRINTF("palThreadFunc2::Thread ID is %"PRIuPTR "\n", threadID); 00082 #ifdef MUTEX_UNITY_TEST 00083 status = pal_osMutexRelease(mutex2); 00084 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00085 #endif //MUTEX_UNITY_TEST 00086 PAL_PRINTF("palThreadFunc2::STAAAAM\n"); 00087 } 00088 00089 void palThreadFunc3(void const *argument) 00090 { 00091 volatile palThreadID_t threadID; 00092 threadsArgument_t *tmp = (threadsArgument_t*)argument; 00093 00094 #ifdef SEMAPHORE_UNITY_TEST 00095 palStatus_t status = PAL_SUCCESS; 00096 uint32_t semaphoresAvailable = 10; 00097 status = pal_osSemaphoreWait(semaphore1, 200, &semaphoresAvailable); 00098 00099 if (PAL_SUCCESS == status) 00100 { 00101 PAL_PRINTF("palThreadFunc3::semaphoresAvailable: %d\n", semaphoresAvailable); 00102 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00103 } 00104 else if(PAL_ERR_RTOS_TIMEOUT == status) 00105 { 00106 PAL_PRINTF("palThreadFunc3::semaphoresAvailable: %d\n", semaphoresAvailable); 00107 PAL_PRINTF("palThreadFunc3::status: 0x%08x\n", status); 00108 PAL_PRINTF("palThreadFunc3::failed to get Semaphore as expected\n", status); 00109 TEST_ASSERT_EQUAL_HEX(PAL_ERR_RTOS_TIMEOUT , status); 00110 return; 00111 } 00112 pal_osDelay(6000); 00113 #endif //SEMAPHORE_UNITY_TEST 00114 tmp->arg3 = 30; 00115 threadID = pal_osThreadGetId(); 00116 TEST_ASSERT_NOT_EQUAL(threadID, NULLPTR); 00117 PAL_PRINTF("palThreadFunc3::Thread ID is %"PRIuPTR "\n", threadID); 00118 00119 #ifdef SEMAPHORE_UNITY_TEST 00120 status = pal_osSemaphoreRelease(semaphore1); 00121 PAL_PRINTF("palThreadFunc3::pal_osSemaphoreRelease res: 0x%08x\n", status); 00122 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00123 #endif //SEMAPHORE_UNITY_TEST 00124 PAL_PRINTF("palThreadFunc3::STAAAAM\n"); 00125 } 00126 00127 void palThreadFunc4(void const *argument) 00128 { 00129 volatile palThreadID_t threadID; 00130 threadsArgument_t *tmp = (threadsArgument_t*)argument; 00131 #ifdef MUTEX_UNITY_TEST 00132 palStatus_t status = PAL_SUCCESS; 00133 PAL_PRINTF("palThreadFunc4::before mutex\n"); 00134 status = pal_osMutexWait(mutex1, 200); 00135 PAL_PRINTF("palThreadFunc4::after mutex: 0x%08x\n", status); 00136 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00137 pal_osDelay(3500); //wait 3.5 seconds to make sure that the next thread arrive to this point 00138 #endif //MUTEX_UNITY_TEST 00139 00140 tmp->arg4 = 40; 00141 threadID = pal_osThreadGetId(); 00142 TEST_ASSERT_NOT_EQUAL(threadID, NULLPTR); 00143 PAL_PRINTF("Thread ID is %"PRIuPTR "\n", threadID); 00144 00145 #ifdef MUTEX_UNITY_TEST 00146 status = pal_osMutexRelease(mutex1); 00147 PAL_PRINTF("palThreadFunc4::after release mutex: 0x%08x\n", status); 00148 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00149 #endif //MUTEX_UNITY_TEST 00150 PAL_PRINTF("palThreadFunc4::STAAAAM\n"); 00151 } 00152 00153 void palThreadFunc5(void const *argument) 00154 { 00155 volatile palThreadID_t threadID; 00156 threadsArgument_t *tmp = (threadsArgument_t*)argument; 00157 #ifdef MUTEX_UNITY_TEST 00158 palStatus_t status = PAL_SUCCESS; 00159 PAL_PRINTF("palThreadFunc5::before mutex\n"); 00160 status = pal_osMutexWait(mutex1, 4500); 00161 PAL_PRINTF("palThreadFunc5::after mutex: 0x%08x\n", status); 00162 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00163 #endif //MUTEX_UNITY_TEST 00164 tmp->arg5 = 50; 00165 threadID = pal_osThreadGetId(); 00166 TEST_ASSERT_NOT_EQUAL(threadID, NULLPTR); 00167 PAL_PRINTF("Thread ID is %"PRIuPTR "\n", threadID); 00168 #ifdef MUTEX_UNITY_TEST 00169 status = pal_osMutexRelease(mutex1); 00170 PAL_PRINTF("palThreadFunc5::after release mutex: 0x%08x\n", status); 00171 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00172 #endif //MUTEX_UNITY_TEST 00173 PAL_PRINTF("palThreadFunc5::STAAAAM\n"); 00174 } 00175 00176 void palThreadFunc6(void const *argument) 00177 { 00178 volatile palThreadID_t threadID; 00179 threadsArgument_t *tmp = (threadsArgument_t*)argument; 00180 #ifdef SEMAPHORE_UNITY_TEST 00181 palStatus_t status = PAL_SUCCESS; 00182 uint32_t semaphoresAvailable = 10; 00183 status = pal_osSemaphoreWait(123456, 200, &semaphoresAvailable); //MUST fail, since there is no semaphore with ID=3 00184 PAL_PRINTF("palThreadFunc6::semaphoresAvailable: %d\n", semaphoresAvailable); 00185 TEST_ASSERT_EQUAL_HEX(PAL_ERR_RTOS_PARAMETER , status); 00186 return; 00187 #endif //SEMAPHORE_UNITY_TEST 00188 tmp->arg6 = 60; 00189 threadID = pal_osThreadGetId(); 00190 TEST_ASSERT_NOT_EQUAL(threadID, NULLPTR); 00191 PAL_PRINTF("Thread ID is %"PRIuPTR "\n", threadID); 00192 #ifdef SEMAPHORE_UNITY_TEST 00193 status = pal_osSemaphoreRelease(123456); 00194 PAL_PRINTF("palThreadFunc6::pal_osSemaphoreRelease res: 0x%08x\n", status); 00195 TEST_ASSERT_EQUAL_HEX(PAL_ERR_RTOS_PARAMETER , status); 00196 #endif //SEMAPHORE_UNITY_TEST 00197 PAL_PRINTF("palThreadFunc6::STAAAAM\n"); 00198 } 00199 00200 00201 void palTimerFunc1(void const *argument) 00202 { 00203 g_timerArgs.ticksInFunc1 = pal_osKernelSysTick(); 00204 PAL_PRINTF("ticks in palTimerFunc1: 0 - %" PRIu32 "\n", g_timerArgs.ticksInFunc1); 00205 PAL_PRINTF("Once Timer function was called\n"); 00206 } 00207 00208 void palTimerFunc2(void const *argument) 00209 { 00210 g_timerArgs.ticksInFunc2 = pal_osKernelSysTick(); 00211 PAL_PRINTF("ticks in palTimerFunc2: 0 - %" PRIu32 "\n", g_timerArgs.ticksInFunc2); 00212 PAL_PRINTF("Periodic Timer function was called\n"); 00213 } 00214 00215 void palTimerFunc3(void const *argument) 00216 { 00217 static int counter =0; 00218 counter++; 00219 } 00220 00221 void palTimerFunc4(void const *argument) 00222 { 00223 static int counter =0; 00224 counter++; 00225 g_timerArgs.ticksInFunc1 = counter; 00226 } 00227 00228 void palTimerFunc5(void const *argument) // function to count calls + wait alternatin short and long periods for timer drift test 00229 { 00230 static int counter = 0; 00231 counter++; 00232 g_timerArgs.ticksInFunc1 = counter; 00233 if (counter % 2 == 0) 00234 { 00235 pal_osDelay(PAL_TIMER_TEST_TIME_TO_WAIT_MS_LONG); 00236 } 00237 else 00238 { 00239 pal_osDelay(PAL_TIMER_TEST_TIME_TO_WAIT_MS_SHORT); 00240 } 00241 } 00242 00243 00244 void palThreadFuncCustom1(void const *argument) 00245 { 00246 PAL_PRINTF("palThreadFuncCustom1 was called\n"); 00247 } 00248 00249 void palThreadFuncCustom2(void const *argument) 00250 { 00251 PAL_PRINTF("palThreadFuncCustom2 was called\n"); 00252 } 00253 00254 void palThreadFuncCustom3(void const *argument) 00255 { 00256 palStatus_t status = PAL_SUCCESS; 00257 PAL_PRINTF("palThreadFuncCustom3 was called\n"); 00258 status = pal_osMutexWait(mutex1, PAL_RTOS_WAIT_FOREVER); 00259 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00260 00261 status = pal_osMutexRelease(mutex1); 00262 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00263 } 00264 00265 void palThreadFuncCustom4(void const *argument) 00266 { 00267 PAL_PRINTF("palThreadFuncCustom4 was called\n"); 00268 } 00269 00270 void palThreadFuncWaitForEverTest(void const *argument) 00271 { 00272 pal_osDelay(PAL_TIME_TO_WAIT_MS/2); 00273 pal_osSemaphoreRelease(*((palSemaphoreID_t*)(argument))); 00274 } 00275 00276 void palRunThreads() 00277 { 00278 palStatus_t status = PAL_SUCCESS; 00279 palThreadID_t threadID1 = NULLPTR; 00280 palThreadID_t threadID2 = NULLPTR; 00281 palThreadID_t threadID3 = NULLPTR; 00282 palThreadID_t threadID4 = NULLPTR; 00283 palThreadID_t threadID5 = NULLPTR; 00284 palThreadID_t threadID6 = NULLPTR; 00285 00286 status = pal_osThreadCreateWithAlloc(palThreadFunc1, &g_threadsArg, PAL_osPriorityIdle, PAL_TEST_THREAD_STACK_SIZE, &g_threadStorage, &threadID1); 00287 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00288 00289 status = pal_osThreadCreateWithAlloc(palThreadFunc2, &g_threadsArg, PAL_osPriorityLow, PAL_TEST_THREAD_STACK_SIZE, NULL, &threadID2); 00290 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00291 00292 status = pal_osThreadCreateWithAlloc(palThreadFunc3, &g_threadsArg, PAL_osPriorityNormal, PAL_TEST_THREAD_STACK_SIZE, NULL, &threadID3); 00293 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00294 00295 status = pal_osThreadCreateWithAlloc(palThreadFunc4, &g_threadsArg, PAL_osPriorityBelowNormal, PAL_TEST_THREAD_STACK_SIZE, NULL, &threadID4); 00296 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00297 00298 status = pal_osDelay(PAL_RTOS_THREAD_CLEANUP_TIMER_MILISEC * 2); // dealy to work around mbedOS timer issue (starting more than 6 timers at once will cause a hang) 00299 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00300 00301 status = pal_osThreadCreateWithAlloc(palThreadFunc5, &g_threadsArg, PAL_osPriorityAboveNormal, PAL_TEST_THREAD_STACK_SIZE, NULL, &threadID5); 00302 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00303 00304 status = pal_osThreadCreateWithAlloc(palThreadFunc6, &g_threadsArg, PAL_osPriorityHigh , PAL_TEST_THREAD_STACK_SIZE, NULL, &threadID6); 00305 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00306 00307 pal_osDelay(PAL_TIME_TO_WAIT_MS/5); 00308 00309 status = pal_osThreadTerminate(&threadID1); 00310 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00311 00312 status = pal_osThreadTerminate(&threadID2); 00313 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00314 00315 status = pal_osThreadTerminate(&threadID3); 00316 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00317 00318 status = pal_osThreadTerminate(&threadID4); 00319 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00320 00321 status = pal_osThreadTerminate(&threadID5); 00322 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00323 00324 status = pal_osThreadTerminate(&threadID6); 00325 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00326 } 00327 00328 void RecursiveLockThread(void const *param) 00329 { 00330 size_t i = 0; 00331 palStatus_t status; 00332 palRecursiveMutexParam_t *actualParams = (palRecursiveMutexParam_t*)param; 00333 size_t countbeforeStart = 0; 00334 volatile palThreadID_t threadID = 10; 00335 00336 status = pal_osSemaphoreWait(actualParams->sem, PAL_RTOS_WAIT_FOREVER, NULL); 00337 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00338 00339 for (i = 0; i < 100; ++i) 00340 { 00341 status = pal_osMutexWait(actualParams->mtx, PAL_RTOS_WAIT_FOREVER); 00342 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00343 if (i == 0) 00344 { 00345 countbeforeStart = actualParams->count; 00346 TEST_ASSERT_EQUAL_HEX(NULLPTR, actualParams->activeThread); 00347 actualParams->activeThread = pal_osThreadGetId(); 00348 } 00349 actualParams->count++; 00350 threadID = pal_osThreadGetId(); 00351 TEST_ASSERT_NOT_EQUAL(NULLPTR, threadID); 00352 TEST_ASSERT_EQUAL(actualParams->activeThread, threadID); 00353 pal_osDelay(1); 00354 } 00355 00356 threadID = 10; 00357 pal_osDelay(50); 00358 TEST_ASSERT_EQUAL(100, actualParams->count - countbeforeStart); 00359 for (i = 0; i < 100; ++i) 00360 { 00361 threadID = pal_osThreadGetId(); 00362 TEST_ASSERT_NOT_EQUAL(NULLPTR, threadID); 00363 TEST_ASSERT_EQUAL(actualParams->activeThread, threadID); 00364 actualParams->count++; 00365 if (i == 99) 00366 { 00367 TEST_ASSERT_EQUAL(200, actualParams->count - countbeforeStart); 00368 actualParams->activeThread = NULLPTR; 00369 } 00370 00371 status = pal_osMutexRelease(actualParams->mtx); 00372 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00373 pal_osDelay(1); 00374 } 00375 00376 status = pal_osSemaphoreRelease(actualParams->sem); 00377 TEST_ASSERT_EQUAL_HEX(PAL_SUCCESS, status); 00378 } 00379
Generated on Tue Jul 12 2022 19:01:36 by
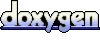