Simple interface for Mbed Cloud Client
Embed:
(wiki syntax)
Show/hide line numbers
pal_plat_update.h
Go to the documentation of this file.
00001 /******************************************************************************* 00002 * Copyright 2016, 2017 ARM Ltd. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 *******************************************************************************/ 00016 00017 00018 #ifndef PAL_PLAT_UPDATE_HEADER 00019 #define PAL_PLAT_UPDATE_HEADER 00020 00021 #ifdef __cplusplus 00022 extern "C" { 00023 #endif 00024 00025 #include "pal.h" 00026 00027 00028 /*! \file pal_plat_update.h 00029 * \brief PAL update - platform. 00030 * This file contains the firmware update APIs that need to be implemented in the platform layer. 00031 */ 00032 00033 00034 /*! Set the callback function that is called before the end of each API (except `imageGetDirectMemAccess`). 00035 * @param[in] CBfunction A pointer to the callback function. 00036 * \return PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure. 00037 */ 00038 palStatus_t pal_plat_imageInitAPI (palImageSignalEvent_t CBfunction); 00039 00040 00041 /*! Clear all the resources used by the `pal_update` APIs. 00042 * \return PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure. 00043 */ 00044 palStatus_t pal_plat_imageDeInit (void); 00045 00046 /*! Set the `imageNumber` to the number of available images. You can do this through the hard coded define inside the linker script. 00047 * @param[out] imageNumber The total number of images the system supports. 00048 * \return PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure. 00049 */ 00050 palStatus_t pal_plat_imageGetMaxNumberOfImages (uint8_t* imageNumber); 00051 00052 /*! Claim space in the relevant storage region for `imageId` with the size of the image. 00053 * @param[in] imageId The image ID. 00054 * @param[in] imageSize The size of the images. 00055 * \return PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure. 00056 */ 00057 palStatus_t pal_plat_imageReserveSpace (palImageId_t imageId, size_t imageSize); 00058 00059 00060 /*! Set up the details for the image header. The data is written when the image write is called for the first time. 00061 * @param[in] imageId The image ID. 00062 * @param[in] details The data needed to build the image header. 00063 * \return PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure. 00064 */ 00065 palStatus_t pal_plat_imageSetHeader (palImageId_t imageId,palImageHeaderDeails_t* details); 00066 00067 /*! Write the data in the chunk buffer with the size written in chunk `bufferLength` in the location of `imageId` adding the relative offset. 00068 * @param[in] imageId The image ID. 00069 * @param[in] offset The relative offset to write the data into. 00070 * @param[in] chunk A pointer to the struct containing the data and the data length to write. 00071 * \return PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure. 00072 */ 00073 palStatus_t pal_plat_imageWrite (palImageId_t imageId, size_t offset, palConstBuffer_t* chunk); 00074 00075 /*! Update the image version of `imageId` to the version written in version buffer with version `bufferLength`. 00076 * @param[in] imageId The image ID. 00077 * @param[in] version The image version and its length. 00078 * \return PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure. 00079 */ 00080 palStatus_t pal_plat_imageSetVersion (palImageId_t imageId, const palConstBuffer_t* version); 00081 00082 /*! Flush the entire image data after writing ends for `imageId`. 00083 * @param[in] imageId The image ID. 00084 * \return PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure. 00085 */ 00086 palStatus_t pal_plat_imageFlush (palImageId_t imageId); 00087 00088 /*! Verify whether the `imageId` is readable and set `imagePtr` to point to the beginning of the image in the memory and `imageSizeInBytes` to the image size. \n 00089 * @param[in] imageId The image ID. 00090 * @param[out] imagePtr A pointer to the start of the image. 00091 * @param[out] imageSizeInBytes The size of the image. 00092 * \return PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure and sets `imagePtr` to NULL. 00093 */ 00094 palStatus_t pal_plat_imageGetDirectMemAccess (palImageId_t imageId, void** imagePtr, size_t* imageSizeInBytes); 00095 00096 00097 /*! Read the max of chunk `maxBufferLength` bytes from the `imageId` with relative offset and store it in chunk buffer. \n 00098 * Set the chunk `bufferLength` value to the actual number of bytes read. 00099 * \note Please use this API in case the image is not directly accessible via the `imageGetDirectMemAccess` function. 00100 * @param[in] imageId The image ID. 00101 * @param[in] offset The offset to start reading from. 00102 * @param[out] chunk The data and actual bytes read. 00103 */ 00104 palStatus_t pal_plat_imageReadToBuffer (palImageId_t imageId, size_t offset, palBuffer_t* chunk); 00105 00106 /*! Set the `imageId` to be the active image (after device reset). 00107 * @param[in] imageId The image ID. 00108 */ 00109 palStatus_t pal_plat_imageActivate (palImageId_t imageId); 00110 00111 /*! Retrieve the hash value of the active image to the hash buffer with the max size hash `maxBufferLength` and set the hash `bufferLength` to the hash size. 00112 * @param[out] hash The hash and actual size of hash read. 00113 */ 00114 palStatus_t pal_plat_imageGetActiveHash (palBuffer_t* hash); 00115 00116 /*! Retrieve the version of the active image to the version buffer with the size set to version `bufferLength`. 00117 * @param[out] version The version and actual size of version read. 00118 */ 00119 palStatus_t pal_plat_imageGetActiveVersion (palBuffer_t* version); 00120 00121 /*! Write the `dataId` stored in `dataBuffer` to the memory accessible to the bootloader. Currently, only HASH is available. 00122 * @param[in] hashValue The data and size of the HASH. 00123 */ 00124 palStatus_t pal_plat_imageWriteHashToMemory (const palConstBuffer_t* const hashValue); 00125 00126 00127 00128 00129 #endif /* SOURCE_PAL_IMPL_MODULES_UPDATE_PAL_PALT_UPDATE_H_ */ 00130 #ifdef __cplusplus 00131 } 00132 #endif
Generated on Tue Jul 12 2022 19:01:36 by
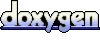