Simple interface for Mbed Cloud Client
Embed:
(wiki syntax)
Show/hide line numbers
mbed_cloud_client_resource.h
00001 #ifndef MBED_CLOUD_CLIENT_RESOURCE_H 00002 #define MBED_CLOUD_CLIENT_RESOURCE_H 00003 00004 #include "mbed.h" 00005 #include "simple-mbed-cloud-client.h" 00006 #include "mbed-client/m2mstring.h" 00007 00008 namespace M2MMethod { 00009 00010 enum M2MMethod { 00011 GET = 0x01, 00012 PUT = 0x02, 00013 POST = 0x04, 00014 DELETE = 0x08 00015 }; 00016 00017 }; 00018 00019 struct mcc_resource_def { 00020 unsigned int object_id; 00021 unsigned int instance_id; 00022 unsigned int resource_id; 00023 String name; 00024 unsigned int method_mask; 00025 String value; 00026 bool observable; 00027 Callback<void(const char*)> *put_callback; 00028 Callback<void(void*)> *post_callback; 00029 Callback<void(const M2MBase&, const NoticationDeliveryStatus)> *notification_callback; 00030 }; 00031 00032 class SimpleMbedCloudClient; 00033 00034 class MbedCloudClientResource { 00035 public: 00036 MbedCloudClientResource(SimpleMbedCloudClient *client, const char *path, const char *name); 00037 00038 void observable(bool observable); 00039 void methods(unsigned int methodMask); 00040 void attach_put_callback(Callback<void(const char*)> callback); 00041 void attach_post_callback(Callback<void(void*)> callback); 00042 void attach_notification_callback(Callback<void(const M2MBase&, const NoticationDeliveryStatus)> callback); 00043 void detach_put_callback(); 00044 void detach_post_callback(); 00045 void detach_notification_callback(); 00046 void set_value(int value); 00047 void set_value(char *value); 00048 String get_value(); 00049 00050 void get_data(mcc_resource_def *resourceDef); 00051 void set_resource(M2MResource *res); 00052 00053 private: 00054 SimpleMbedCloudClient *client; 00055 M2MResource *resource; 00056 String path; 00057 String name; 00058 String value; 00059 bool isObservable; 00060 unsigned int methodMask; 00061 00062 Callback<void(const char*)> putCallback; 00063 Callback<void(void*)> postCallback; 00064 Callback<void(const M2MBase&, const NoticationDeliveryStatus)> notificationCallback; 00065 }; 00066 00067 #endif // MBED_CLOUD_CLIENT_RESOURCE_H
Generated on Tue Jul 12 2022 19:01:35 by
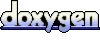