Simple interface for Mbed Cloud Client
Embed:
(wiki syntax)
Show/hide line numbers
mbed_cloud_client_resource.cpp
00001 #include "mbed.h" 00002 #include "mbed_cloud_client_resource.h" 00003 #include "simple-mbed-cloud-client.h" 00004 00005 void path_to_ids(const char* path, unsigned int *object_id, 00006 unsigned int *instance_id, unsigned int *resource_id) { 00007 int len = strlen(path); 00008 char *buffer = new char[len + 1]; 00009 buffer[len] = '\0'; 00010 strncpy(buffer, path, len); 00011 unsigned int index = 0; 00012 char * pch = strtok (buffer, "/"); 00013 00014 unsigned int *ptr; 00015 while (pch != NULL && index < 3) { 00016 switch (index) { 00017 case 0: 00018 ptr = object_id; 00019 break; 00020 00021 case 1: 00022 ptr = instance_id; 00023 break; 00024 00025 case 2: 00026 ptr = resource_id; 00027 break; 00028 } 00029 00030 *ptr = atoi(pch); 00031 pch = strtok (NULL, "/"); 00032 index++; 00033 } 00034 00035 delete[] buffer; 00036 } 00037 00038 MbedCloudClientResource::MbedCloudClientResource(SimpleMbedCloudClient *client, const char *path, const char *name) 00039 : client(client), resource(NULL), path(path), name(name), putCallback(NULL), 00040 postCallback(NULL), notificationCallback(NULL) { 00041 } 00042 00043 void MbedCloudClientResource::observable(bool observable) { 00044 this->isObservable = observable; 00045 } 00046 00047 void MbedCloudClientResource::methods(unsigned int methodMask) { 00048 this->methodMask = methodMask; 00049 } 00050 00051 void MbedCloudClientResource::attach_put_callback(Callback<void(const char*)> callback) { 00052 this->putCallback = callback; 00053 } 00054 00055 void MbedCloudClientResource::attach_post_callback(Callback<void(void*)> callback) { 00056 this->postCallback = callback; 00057 } 00058 00059 void MbedCloudClientResource::attach_notification_callback(Callback<void(const M2MBase&, const NoticationDeliveryStatus)> callback) { 00060 this->notificationCallback = callback; 00061 } 00062 00063 void MbedCloudClientResource::detach_put_callback() { 00064 this->putCallback = NULL; 00065 } 00066 00067 void MbedCloudClientResource::detach_post_callback() { 00068 this->postCallback = NULL; 00069 } 00070 00071 void MbedCloudClientResource::detach_notification_callback() { 00072 this->notificationCallback = NULL; 00073 } 00074 00075 void MbedCloudClientResource::set_value(int value) { 00076 this->value = ""; 00077 this->value.append_int(value); 00078 00079 if (this->resource) { 00080 this->resource->set_value((uint8_t*)this->value.c_str(), this->value.size()); 00081 } 00082 } 00083 00084 void MbedCloudClientResource::set_value(char *value) { 00085 this->value = value; 00086 00087 if (this->resource) { 00088 this->resource->set_value((uint8_t*)this->value.c_str(), strlen(value)); 00089 } 00090 } 00091 00092 String MbedCloudClientResource::get_value() { 00093 if (this->resource) { 00094 return this->resource->get_value_string(); 00095 } else { 00096 return this->value; 00097 } 00098 } 00099 00100 void MbedCloudClientResource::get_data(mcc_resource_def *resourceDef) { 00101 path_to_ids(this->path.c_str(), &(resourceDef->object_id), &(resourceDef->instance_id), &(resourceDef->resource_id)); 00102 resourceDef->name = this->name; 00103 resourceDef->method_mask = this->methodMask; 00104 resourceDef->observable = this->isObservable; 00105 resourceDef->value = this->get_value(); 00106 resourceDef->put_callback = &(this->putCallback); 00107 resourceDef->post_callback = &(this->postCallback); 00108 resourceDef->notification_callback = &(this->notificationCallback); 00109 } 00110 00111 void MbedCloudClientResource::set_resource(M2MResource *res) { 00112 this->resource = res; 00113 }
Generated on Tue Jul 12 2022 19:01:35 by
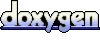