Simple interface for Mbed Cloud Client
Embed:
(wiki syntax)
Show/hide line numbers
m2msecurity.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef M2M_SECURITY_H 00017 #define M2M_SECURITY_H 00018 00019 #include "mbed-client/m2mobject.h" 00020 00021 // FORWARD DECLARATION 00022 class M2MResource; 00023 00024 /*! \file m2msecurity.h 00025 * \brief M2MSecurity. 00026 * This class represents an interface for the Security Object model of the LWM2M framework. 00027 * It handles the security object instances and all corresponding 00028 * resources. 00029 */ 00030 00031 class M2MSecurity : public M2MObject { 00032 00033 friend class M2MInterfaceFactory; 00034 friend class M2MNsdlInterface; 00035 00036 public: 00037 00038 /** 00039 * \brief An enum defining all resources associated with a 00040 * Security Object in the LWM2M framework. 00041 */ 00042 typedef enum { 00043 M2MServerUri, 00044 BootstrapServer, 00045 SecurityMode, 00046 PublicKey, 00047 ServerPublicKey, 00048 Secretkey, 00049 SMSSecurityMode, 00050 SMSBindingKey, 00051 SMSBindingSecretKey, 00052 M2MServerSMSNumber, 00053 ShortServerID, 00054 ClientHoldOffTime 00055 }SecurityResource; 00056 00057 /** 00058 * \brief An enum defining the type of the security attribute 00059 * used by the Security Object. 00060 */ 00061 typedef enum { 00062 SecurityNotSet = -1, 00063 Psk = 0, 00064 Certificate = 2, 00065 NoSecurity = 3 00066 } SecurityModeType; 00067 00068 /** 00069 * \brief An enum defining an interface operation that can be 00070 * handled by the Security Object. 00071 */ 00072 typedef enum { 00073 M2MServer = 0x0, 00074 Bootstrap = 0x1 00075 } ServerType; 00076 00077 private: 00078 00079 /** 00080 * \brief Constructor 00081 * \param server_type The type of the security object created. Either bootstrap or LWM2M server. 00082 */ 00083 M2MSecurity(ServerType server_type); 00084 00085 00086 /** 00087 * \brief Destructor 00088 */ 00089 virtual ~M2MSecurity(); 00090 00091 // Prevents the use of default constructor. 00092 M2MSecurity(); 00093 00094 // Prevents the use of assignment operator. 00095 M2MSecurity& operator=( const M2MSecurity& /*other*/ ); 00096 00097 // Prevents the use of copy constructor 00098 M2MSecurity( const M2MSecurity& /*other*/ ); 00099 00100 public: 00101 00102 /** 00103 * \brief Get the singleton instance of M2MSecurity 00104 */ 00105 static M2MSecurity* get_instance(); 00106 00107 /** 00108 * \brief Delete the singleton instance of M2MSecurity 00109 */ 00110 static void delete_instance(); 00111 00112 /** 00113 * \brief Creates a new object instance. 00114 * \param server_type Server type for new object instance. 00115 * \return M2MObjectInstance if created successfully, else NULL. 00116 */ 00117 M2MObjectInstance* create_object_instance(ServerType server_type); 00118 00119 /** 00120 * \brief Remove all security object instances. 00121 */ 00122 void remove_security_instances(); 00123 00124 /** 00125 * \brief Creates a new resource for a given resource enum. 00126 * \param rescource With this function, the following resources can be created: 00127 * ' BootstrapServer', 'SecurityMode', 'SMSSecurityMode', 00128 * 'M2MServerSMSNumber', 'ShortServerID', 'ClientHoldOffTime'. 00129 * \param value The value to be set on the resource, in integer format. 00130 * \param instance_id Instance id of the security instance where resource should be created. 00131 * \return M2MResource if created successfully, else NULL. 00132 */ 00133 M2MResource* create_resource(SecurityResource rescource, uint32_t value, uint16_t instance_id); 00134 00135 /** 00136 * \brief Deletes a resource with a given resource enum. 00137 * Mandatory resources cannot be deleted. 00138 * \param resource The resource to be deleted. 00139 * \param instance_id Instance id of the security instance where resource should be deleted. 00140 * \return True if deleted, else false. 00141 */ 00142 bool delete_resource(SecurityResource rescource, uint16_t instance_id); 00143 00144 /** 00145 * \brief Sets the value of a given resource enum. 00146 * \param resource With this function, a value can be set for the following resources: 00147 * 'M2MServerUri', 'SMSBindingKey', 'SMSBindingSecretKey'. 00148 * \param value The value to be set on the resource, in string format. 00149 * \param instance_id Instance id of the security instance where resource value should be set. 00150 * \return True if successfully set, else false. 00151 */ 00152 bool set_resource_value(SecurityResource resource, 00153 const String &value, 00154 uint16_t instance_id); 00155 00156 /** 00157 * \brief Sets the value of a given resource enum. 00158 * \param resource With this function, a value can be set for the following resourecs: 00159 * 'BootstrapServer', 'SecurityMode', 'SMSSecurityMode', 00160 * 'M2MServerSMSNumber', 'ShortServerID', 'ClientHoldOffTime'. 00161 * \param value The value to be set on the resource, in integer format. 00162 * \param instance_id Instance id of the security instance where resource value should be set. 00163 * \return True if successfully set, else false. 00164 */ 00165 bool set_resource_value(SecurityResource resource, 00166 uint32_t value, 00167 uint16_t instance_id); 00168 00169 /** 00170 * \brief Sets the value of a given resource enum. 00171 * \param resource With this function, a value can be set for the follwing resources: 00172 * 'PublicKey', 'ServerPublicKey', 'Secretkey'. 00173 * \param value The value to be set on the resource, in uint8_t format. 00174 * \param length The size of the buffer value to be set on the resource. 00175 * \param instance_id Instance id of the security instance where resource value should be set. 00176 * \return True if successfully set, else false. 00177 */ 00178 bool set_resource_value(SecurityResource resource, 00179 const uint8_t *value, 00180 const uint16_t length, 00181 uint16_t instance_id); 00182 00183 /** 00184 * \brief Returns the value of a given resource enum, in string format. 00185 * \param resource With this function, the following resources can return a value: 00186 * 'M2MServerUri','SMSBindingKey', 'SMSBindingSecretKey'. 00187 * \param instance_id Instance id of the security instance where resource value should be retrieved. 00188 * \return The value associated with the resource. If the resource is not valid an empty string is returned. 00189 */ 00190 String resource_value_string(SecurityResource resource, uint16_t instance_id) const; 00191 00192 /** 00193 * \brief Populates the data buffer and returns the size of the buffer. 00194 * \param resource With this function, the following resources can return a value: 00195 * 'PublicKey', 'ServerPublicKey', 'Secretkey'. 00196 * \param [OUT]data A copy of the data buffer that contains the value. The caller 00197 * is responsible for freeing this buffer. 00198 * \param instance_id Instance id of the security instance where resource value should be retrieve. 00199 * \return The size of the populated buffer. 00200 */ 00201 uint32_t resource_value_buffer(SecurityResource resource, 00202 uint8_t *&data, 00203 uint16_t instance_id) const; 00204 00205 /** 00206 * \brief Returns a pointer to the value and size of the buffer. 00207 * \param resource With this function, the following resources can return a value: 00208 * 'PublicKey', 'ServerPublicKey', 'Secretkey'. 00209 * \param [OUT]data A pointer to the data buffer that contains the value. 00210 * \param instance_id Instance id of the security instance where resource value should be retrieved. 00211 * \return The size of the populated buffer. 00212 */ 00213 uint32_t resource_value_buffer(SecurityResource resource, 00214 const uint8_t *&data, 00215 uint16_t instance_id) const; 00216 00217 /** 00218 * \brief Returns the value of a given resource name, in integer format. 00219 * \param resource With this function, the following resources can return a value: 00220 * 'BootstrapServer', 'SecurityMode', 'SMSSecurityMode', 00221 * 'M2MServerSMSNumber', 'ShortServerID', 'ClientHoldOffTime'. 00222 * \param instance_id Instance id of the security instance where resource should be created. 00223 * \return The value associated with the resource. If the resource is not valid 0 is returned. 00224 */ 00225 uint32_t resource_value_int(SecurityResource resource, 00226 uint16_t instance_id) const; 00227 00228 /** 00229 * \brief Returns whether a resource instance with a given resource enum exists or not 00230 * \param resource Resource enum. 00231 * \param instance_id Instance id of the security instance where resource should be checked. 00232 * \return True if at least one instance exists, else false. 00233 */ 00234 bool is_resource_present(SecurityResource resource, 00235 uint16_t instance_id) const; 00236 00237 /** 00238 * \brief Returns the total number of resources for a security object. 00239 * \param instance_id Instance id of the security instance where resources should be counted. 00240 * \return The total number of resources. 00241 */ 00242 uint16_t total_resource_count(uint16_t instance_id) const; 00243 00244 /** 00245 * \brief Returns the type of the Security Object. It can be either 00246 * Bootstrap or M2MServer. 00247 * \param instance_id Instance id of the security instance where resource should be created. 00248 * \return ServerType The type of the Security Object. 00249 */ 00250 ServerType server_type(uint16_t instance_id) const; 00251 00252 /** 00253 * \brief Returns first bootstrap or lwm2m server security object instance id. 00254 * \param server_type Which server type security instance to return. 00255 * \return Object instance id, or -1 if no such instance exists. 00256 */ 00257 int32_t get_security_instance_id(ServerType server_type) const; 00258 00259 private: 00260 00261 M2MResource* get_resource(SecurityResource resource, uint16_t instance_id = 0) const; 00262 void clear_resources(uint16_t instance_id = 0); 00263 00264 protected: 00265 static M2MSecurity* _instance; 00266 00267 friend class Test_M2MSecurity; 00268 friend class Test_M2MInterfaceImpl; 00269 friend class Test_M2MConnectionSecurityImpl; 00270 friend class Test_M2MConnectionHandlerPimpl_linux; 00271 friend class Test_M2MConnectionHandlerPimpl_mbed; 00272 friend class Test_M2MConnectionSecurityPimpl; 00273 friend class Test_M2MNsdlInterface; 00274 friend class Test_M2MConnectionHandlerPimpl_classic; 00275 }; 00276 00277 #endif // M2M_SECURITY_H 00278 00279
Generated on Tue Jul 12 2022 19:01:35 by
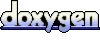