Simple interface for Mbed Cloud Client
Embed:
(wiki syntax)
Show/hide line numbers
m2mresourcebase.h
00001 /* 00002 * Copyright (c) 2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef M2M_RESOURCE_BASE_H 00017 #define M2M_RESOURCE_BASE_H 00018 00019 #include "mbed-client/m2mbase.h" 00020 #include "mbed-client/functionpointer.h" 00021 00022 00023 /*! \file m2mresourceinstance.h 00024 * \brief M2MResourceInstance. 00025 * This class is the base class for mbed Client Resources. All defined 00026 * LWM2M resource models can be created based on it. 00027 */ 00028 class M2MBlockMessage; 00029 00030 typedef FP1<void,void*> execute_callback; 00031 typedef void(*execute_callback_2) (void *arguments); 00032 00033 typedef FP0<void> notification_sent_callback; 00034 typedef void(*notification_sent_callback_2) (void); 00035 00036 #ifndef DISABLE_BLOCK_MESSAGE 00037 typedef FP1<void, M2MBlockMessage *> incoming_block_message_callback; 00038 typedef FP3<void, const String &, uint8_t *&, uint32_t &> outgoing_block_message_callback; 00039 #endif 00040 00041 class M2MResource; 00042 00043 class M2MResourceBase : public M2MBase { 00044 00045 friend class M2MObjectInstance; 00046 friend class M2MResource; 00047 friend class M2MResourceInstance; 00048 00049 public: 00050 00051 typedef enum { 00052 INIT = 0, // Initial state. 00053 SENT, // Notification created/sent but not received ACK yet. 00054 DELIVERED // Received ACK from server. 00055 } NotificationStatus; 00056 00057 typedef FP2<void, const uint16_t, const M2MResourceBase::NotificationStatus> notification_status_callback; 00058 00059 typedef void(*notification_status_callback_2) (const uint16_t msg_id, 00060 const M2MResourceBase::NotificationStatus status); 00061 00062 /** 00063 * An enum defining a resource type that can be 00064 * supported by a given resource. 00065 */ 00066 typedef enum { 00067 STRING, 00068 INTEGER, 00069 FLOAT, 00070 BOOLEAN, 00071 OPAQUE, 00072 TIME, 00073 OBJLINK 00074 }ResourceType; 00075 00076 protected: // Constructor and destructor are private 00077 // which means that these objects can be created or 00078 // deleted only through a function provided by the M2MObjectInstance. 00079 00080 M2MResourceBase( 00081 const lwm2m_parameters_s* s, 00082 M2MBase::DataType type); 00083 /** 00084 * \brief A constructor for creating a resource. 00085 * \param resource_name The name of the resource. 00086 * \param resource_type The type of the resource. 00087 * \param type The resource data type of the object. 00088 * \param object_name Object name where resource exists. 00089 * \param path Path of the object like 3/0/1 00090 * \param external_blockwise_store If true CoAP blocks are passed to application through callbacks 00091 * otherwise handled in mbed-client-c. 00092 */ 00093 M2MResourceBase( 00094 const String &resource_name, 00095 M2MBase::Mode mode, 00096 const String &resource_type, 00097 M2MBase::DataType type, 00098 char* path, 00099 bool external_blockwise_store, 00100 bool multiple_instance); 00101 00102 /** 00103 * \brief A Constructor for creating a resource. 00104 * \param resource_name The name of the resource. 00105 * \param resource_type The type of the resource. 00106 * \param type The resource data type of the object. 00107 * \param value The value pointer of the object. 00108 * \param value_length The length of the value pointer. 00109 * \param value_length The length of the value pointer. 00110 * \param object_name Object name where resource exists. 00111 * \param path Path of the object like 3/0/1 00112 * \param external_blockwise_store If true CoAP blocks are passed to application through callbacks 00113 * otherwise handled in mbed-client-c. 00114 */ 00115 M2MResourceBase( 00116 const String &resource_name, 00117 M2MBase::Mode mode, 00118 const String &resource_type, 00119 M2MBase::DataType type, 00120 const uint8_t *value, 00121 const uint8_t value_length, 00122 char* path, 00123 bool external_blockwise_store, 00124 bool multiple_instance); 00125 00126 // Prevents the use of default constructor. 00127 M2MResourceBase(); 00128 00129 // Prevents the use of assignment operator. 00130 M2MResourceBase& operator=( const M2MResourceBase& /*other*/ ); 00131 00132 // Prevents the use of copy constructor 00133 M2MResourceBase( const M2MResourceBase& /*other*/ ); 00134 00135 /** 00136 * Destructor 00137 */ 00138 virtual ~M2MResourceBase(); 00139 00140 public: 00141 00142 /** 00143 * \brief Returns the resource data type. 00144 * \return ResourceType. 00145 */ 00146 M2MResourceBase::ResourceType resource_instance_type() const; 00147 00148 /** 00149 * \brief Sets the function that should be executed when this 00150 * resource receives a POST command. 00151 * \param callback The function pointer that needs to be executed. 00152 * \return True, if callback could be set, false otherwise. 00153 */ 00154 bool set_execute_function(execute_callback callback); 00155 00156 /** 00157 * \brief Sets the function that should be executed when this 00158 * resource receives a POST command. 00159 * \param callback The function pointer that needs to be executed. 00160 * \return True, if callback could be set, false otherwise. 00161 */ 00162 bool set_execute_function(execute_callback_2 callback); 00163 00164 /** 00165 * \brief Sets a value of a given resource. 00166 * \param value A pointer to the value to be set on the resource. 00167 * \param value_length The length of the value pointer. 00168 * \return True if successfully set, else false. 00169 * \note If resource is observable, calling this API rapidly (< 1s) can fill up the CoAP resending queue 00170 * and notification sending fails. CoAP resending queue size can be modified through: 00171 * "sn-coap-resending-queue-size-msgs" and "sn-coap-resending-queue-size-bytes" parameters. 00172 * Increasing these parameters will increase the memory consumption. 00173 */ 00174 bool set_value(const uint8_t *value, const uint32_t value_length); 00175 00176 /** 00177 * \brief Sets a value of a given resource. 00178 * \param value A pointer to the value to be set on the resource, ownerhip transfered. 00179 * \param value_length The length of the value pointer. 00180 * \return True if successfully set, else false. 00181 * \note If resource is observable, calling this API rapidly (< 1s) can fill up the CoAP resending queue 00182 * and notification sending fails. CoAP resending queue size can be modified through: 00183 * "sn-coap-resending-queue-size-msgs" and "sn-coap-resending-queue-size-bytes" parameters. 00184 * Increasing these parameters will increase the memory consumption. 00185 */ 00186 bool set_value_raw(uint8_t *value, const uint32_t value_length); 00187 00188 /** 00189 * \brief Sets a value of a given resource. 00190 * \param value, A new value formatted as a string 00191 * and set on the resource. 00192 * \return True if successfully set, else false. 00193 * \note If resource is observable, calling this API rapidly (< 1s) can fill up the CoAP resending queue 00194 * and notification sending fails. CoAP resending queue size can be modified through: 00195 * "sn-coap-resending-queue-size-msgs" and "sn-coap-resending-queue-size-bytes" parameters. 00196 * Increasing these parameters will increase the memory consumption. 00197 */ 00198 bool set_value(int64_t value); 00199 00200 /** 00201 * \brief Clears the value of a given resource. 00202 */ 00203 void clear_value(); 00204 00205 /** 00206 * \brief Executes the function that is set in "set_execute_function". 00207 * \param arguments The arguments that are passed to be executed. 00208 */ 00209 void execute(void *arguments); 00210 00211 /** 00212 * \brief Provides the value of the given resource. 00213 * \param value[OUT] A pointer to the resource value. 00214 * \param value_length[OUT] The length of the value pointer. 00215 */ 00216 void get_value(uint8_t *&value, uint32_t &value_length); 00217 00218 /** 00219 * \brief Converts a value to integer and returns it. Note: Conversion 00220 * errors are not detected. 00221 */ 00222 int64_t get_value_int() const; 00223 00224 /** 00225 * Get the value as a string object. No encoding/charset conversions 00226 * are done for the value, just a raw copy. 00227 */ 00228 String get_value_string() const; 00229 00230 /** 00231 * \brief Returns the value pointer of the object. 00232 * \return The value pointer of the object. 00233 */ 00234 uint8_t* value() const; 00235 00236 /** 00237 * \brief Returns the length of the value pointer. 00238 * \return The length of the value pointer. 00239 */ 00240 uint32_t value_length() const; 00241 00242 00243 /** 00244 * \brief Handles the GET request for the registered objects. 00245 * \param nsdl An NSDL handler for the CoAP library. 00246 * \param received_coap_header The CoAP message received from the server. 00247 * \param observation_handler A handler object for sending 00248 * observation callbacks. 00249 * \return sn_coap_hdr_s The message that needs to be sent to the server. 00250 */ 00251 virtual sn_coap_hdr_s* handle_get_request(nsdl_s *nsdl, 00252 sn_coap_hdr_s *received_coap_header, 00253 M2MObservationHandler *observation_handler = NULL); 00254 /** 00255 * \brief Handles the PUT request for the registered objects. 00256 * \param nsdl An NSDL handler for the CoAP library. 00257 * \param received_coap_header The CoAP message received from the server. 00258 * \param observation_handler A handler object for sending 00259 * observation callbacks. 00260 * \param execute_value_updated True will execute the "value_updated" callback. 00261 * \return sn_coap_hdr_s The message that needs to be sent to the server. 00262 */ 00263 virtual sn_coap_hdr_s* handle_put_request(nsdl_s *nsdl, 00264 sn_coap_hdr_s *received_coap_header, 00265 M2MObservationHandler *observation_handler, 00266 bool &execute_value_updated); 00267 00268 /** 00269 * \brief Returns the instance ID of the object where the resource exists. 00270 * \return Object instance ID. 00271 */ 00272 virtual uint16_t object_instance_id() const = 0; 00273 00274 /** 00275 * \brief Returns the name of the object where the resource exists. 00276 * \return Object name. 00277 */ 00278 virtual const char* object_name() const = 0; 00279 00280 virtual M2MResource& get_parent_resource() const = 0; 00281 00282 #ifndef DISABLE_BLOCK_MESSAGE 00283 /** 00284 * @brief Sets the function that is executed when this 00285 * object receives a block-wise message. 00286 * @param callback The function pointer that is called. 00287 */ 00288 bool set_incoming_block_message_callback(incoming_block_message_callback callback); 00289 00290 /** 00291 * @brief Sets the function that is executed when this 00292 * object receives a GET request. 00293 * This is called if resource values are stored on the application side. 00294 * NOTE! Due to a limitation in the mbed-client-c library, a GET request can only contain data size up to 65KB. 00295 * @param callback The function pointer that is called. 00296 */ 00297 bool set_outgoing_block_message_callback(outgoing_block_message_callback callback); 00298 00299 /** 00300 * \brief Returns the block message object. 00301 * \return Block message. 00302 */ 00303 M2MBlockMessage* block_message() const; 00304 00305 #endif 00306 00307 /** 00308 * @brief Sets the function that is executed when this object receives 00309 * response(Empty ACK) for notification message. 00310 * @param callback The function pointer that is called. 00311 */ 00312 bool set_notification_sent_callback(notification_sent_callback callback) m2m_deprecated; 00313 00314 /** 00315 * @brief Sets the function that is executed when this object receives 00316 * response(Empty ACK) for notification message. 00317 * @param callback The function pointer that is called. 00318 */ 00319 bool set_notification_sent_callback(notification_sent_callback_2 callback) m2m_deprecated; 00320 00321 /** 00322 * @brief Sets the function that is executed when this object receives 00323 * response(Empty ACK) for notification message. 00324 * @param callback The function pointer that is called. 00325 */ 00326 bool set_notification_status_callback(notification_status_callback callback) m2m_deprecated; 00327 00328 /** 00329 * @brief Sets the function that is executed when this object receives 00330 * response(Empty ACK) for notification message. 00331 * @param callback The function pointer that is called. 00332 */ 00333 bool set_notification_status_callback(notification_status_callback_2 callback); 00334 00335 /** 00336 * \brief Executes the function that is set in "set_notification_sent_callback". 00337 */ 00338 void notification_sent(); 00339 00340 /** 00341 * \brief Executes the function that is set in "set_notification_status_callback". 00342 */ 00343 void notification_status(const uint16_t msg_id, NotificationStatus status); 00344 00345 /** 00346 * \brief Returns notification send status. 00347 * \return Notification status. 00348 */ 00349 M2MResourceBase::NotificationStatus notification_status() const; 00350 00351 /** 00352 * \brief Clears the notification send status to initial state. 00353 */ 00354 void clear_notification_status(); 00355 00356 private: 00357 00358 void report(); 00359 00360 void report_value_change(); 00361 00362 bool has_value_changed(const uint8_t* value, const uint32_t value_len); 00363 00364 M2MResourceBase::ResourceType convert_data_type(M2MBase::DataType type) const; 00365 00366 private: 00367 00368 #ifndef DISABLE_BLOCK_MESSAGE 00369 M2MBlockMessage *_block_message_data; 00370 #endif 00371 00372 NotificationStatus _notification_status : 2; 00373 00374 friend class Test_M2MResourceInstance; 00375 friend class Test_M2MResource; 00376 friend class Test_M2MObjectInstance; 00377 friend class Test_M2MObject; 00378 friend class Test_M2MDevice; 00379 friend class Test_M2MSecurity; 00380 friend class Test_M2MServer; 00381 friend class Test_M2MNsdlInterface; 00382 friend class Test_M2MFirmware; 00383 friend class Test_M2MTLVSerializer; 00384 friend class Test_M2MTLVDeserializer; 00385 }; 00386 00387 #endif // M2M_RESOURCE_BASE_H
Generated on Tue Jul 12 2022 19:01:35 by
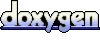