Simple interface for Mbed Cloud Client
Embed:
(wiki syntax)
Show/hide line numbers
m2mobject.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef M2M_OBJECT_H 00017 #define M2M_OBJECT_H 00018 00019 #include "mbed-client/m2mvector.h" 00020 #include "mbed-client/m2mbase.h" 00021 #include "mbed-client/m2mobjectinstance.h" 00022 00023 //FORWARD DECLARATION 00024 typedef Vector<M2MObjectInstance *> M2MObjectInstanceList; 00025 00026 /*! \file m2mobject.h 00027 * \brief M2MObject. 00028 * This class is the base class for the mbed Client Objects. All defined 00029 * LWM2M object models can be created based on it. This class also holds all object 00030 * instances associated with the given object. 00031 */ 00032 00033 class M2MObject : public M2MBase 00034 { 00035 00036 friend class M2MInterfaceFactory; 00037 00038 protected : 00039 00040 /** 00041 * \brief Constructor 00042 * \param name The name of the object. 00043 * \param path Path of the object like 3/0/1 00044 * \param external_blockwise_store If true CoAP blocks are passed to application through callbacks 00045 * otherwise handled in mbed-client-c. 00046 */ 00047 M2MObject(const String &object_name, 00048 char *path, 00049 bool external_blockwise_store = false); 00050 00051 // Prevents the use of default constructor. 00052 M2MObject(); 00053 00054 // Prevents the use of assignment operator. 00055 M2MObject& operator=( const M2MObject& /*other*/ ); 00056 00057 // Prevents the use of copy constructor. 00058 M2MObject( const M2MObject& /*other*/ ); 00059 00060 /** 00061 * \brief Constructor 00062 * \param name The name of the object. 00063 */ 00064 M2MObject(const M2MBase::lwm2m_parameters_s* static_res); 00065 00066 public: 00067 00068 /** 00069 * \brief Destructor 00070 */ 00071 virtual ~M2MObject(); 00072 00073 /** 00074 * \brief Creates a new object instance for a given mbed Client Interface object. With this, 00075 * the client can respond to server's GET methods with the provided value. 00076 * \return M2MObjectInstance. An object instance for managing other client operations. 00077 */ 00078 M2MObjectInstance* create_object_instance(uint16_t instance_id = 0); 00079 00080 /** 00081 * TODO!! 00082 */ 00083 M2MObjectInstance* create_object_instance(const lwm2m_parameters_s* s); 00084 00085 /** 00086 * \brief Removes the object instance resource with the given instance id. 00087 * \param instance_id The instance ID of the object instance to be removed, default is 0. 00088 * \return True if removed, else false. 00089 */ 00090 bool remove_object_instance(uint16_t instance_id = 0); 00091 00092 /** 00093 * \brief Returns the object instance with the the given instance ID. 00094 * \param instance_id The instance ID of the requested object instance ID, default is 0. 00095 * \return Object instance reference if found, else NULL. 00096 */ 00097 M2MObjectInstance* object_instance(uint16_t instance_id = 0) const; 00098 00099 /** 00100 * \brief Returns a list of object instances. 00101 * \return A list of object instances. 00102 */ 00103 const M2MObjectInstanceList& instances() const; 00104 00105 /** 00106 * \brief Returns the total number of object instances- 00107 * \return The total number of the object instances. 00108 */ 00109 uint16_t instance_count() const; 00110 00111 /** 00112 * \brief Returns the Observation Handler object. 00113 * \return M2MObservationHandler object. 00114 */ 00115 virtual M2MObservationHandler* observation_handler() const; 00116 00117 /** 00118 * \brief Sets the observation handler 00119 * \param handler Observation handler 00120 */ 00121 virtual void set_observation_handler(M2MObservationHandler *handler); 00122 00123 /** 00124 * \brief Adds the observation level for the object. 00125 * \param observation_level The level of observation. 00126 */ 00127 virtual void add_observation_level(M2MBase::Observation observation_level); 00128 00129 /** 00130 * \brief Removes the observation level from the object. 00131 * \param observation_level The level of observation. 00132 */ 00133 virtual void remove_observation_level(M2MBase::Observation observation_level); 00134 00135 /** 00136 * \brief Handles GET request for the registered objects. 00137 * \param nsdl The NSDL handler for the CoAP library. 00138 * \param received_coap_header The CoAP message received from the server. 00139 * \param observation_handler The handler object for sending 00140 * observation callbacks. 00141 * \return sn_coap_hdr_s The message that needs to be sent to server. 00142 */ 00143 virtual sn_coap_hdr_s* handle_get_request(nsdl_s *nsdl, 00144 sn_coap_hdr_s *received_coap_header, 00145 M2MObservationHandler *observation_handler = NULL); 00146 00147 /** 00148 * \brief Handles PUT request for the registered objects. 00149 * \param nsdl The NSDL handler for the CoAP library. 00150 * \param received_coap_header The received CoAP message from the server. 00151 * \param observation_handler The handler object for sending 00152 * observation callbacks. 00153 * \param execute_value_updated True will execute the "value_updated" callback. 00154 * \return sn_coap_hdr_s The message that needs to be sent to server. 00155 */ 00156 virtual sn_coap_hdr_s* handle_put_request(nsdl_s *nsdl, 00157 sn_coap_hdr_s *received_coap_header, 00158 M2MObservationHandler *observation_handler, 00159 bool &execute_value_updated); 00160 00161 /** 00162 * \brief Handles GET request for the registered objects. 00163 * \param nsdl The NSDL handler for the CoAP library. 00164 * \param received_coap_header The received CoAP message from the server. 00165 * \param observation_handler The handler object for sending 00166 * observation callbacks. 00167 * \param execute_value_updated True will execute the "value_updated" callback. 00168 * \return sn_coap_hdr_s The message that needs to be sent to server. 00169 */ 00170 virtual sn_coap_hdr_s* handle_post_request(nsdl_s *nsdl, 00171 sn_coap_hdr_s *received_coap_header, 00172 M2MObservationHandler *observation_handler, 00173 bool &execute_value_updated, 00174 sn_nsdl_addr_s *address = NULL); 00175 00176 void notification_update(uint16_t obj_instance_id); 00177 00178 protected : 00179 00180 00181 private: 00182 00183 M2MObjectInstanceList _instance_list; // owned 00184 00185 M2MObservationHandler *_observation_handler; // Not owned 00186 00187 friend class Test_M2MObject; 00188 friend class Test_M2MInterfaceImpl; 00189 friend class Test_M2MNsdlInterface; 00190 friend class Test_M2MTLVSerializer; 00191 friend class Test_M2MTLVDeserializer; 00192 friend class Test_M2MDevice; 00193 friend class Test_M2MFirmware; 00194 friend class Test_M2MBase; 00195 friend class Test_M2MObjectInstance; 00196 friend class Test_M2MResource; 00197 friend class Test_M2MSecurity; 00198 friend class Test_M2MServer; 00199 friend class Test_M2MResourceInstance; 00200 }; 00201 00202 #endif // M2M_OBJECT_H
Generated on Tue Jul 12 2022 19:01:35 by
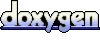