Simple interface for Mbed Cloud Client
Embed:
(wiki syntax)
Show/hide line numbers
ftcd_comm_base.h
Go to the documentation of this file.
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // Licensed under the Apache License, Version 2.0 (the "License"); 00005 // you may not use this file except in compliance with the License. 00006 // You may obtain a copy of the License at 00007 // 00008 // http://www.apache.org/licenses/LICENSE-2.0 00009 // 00010 // Unless required by applicable law or agreed to in writing, software 00011 // distributed under the License is distributed on an "AS IS" BASIS, 00012 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 // See the License for the specific language governing permissions and 00014 // limitations under the License. 00015 // ---------------------------------------------------------------------------- 00016 00017 #ifndef __FTCD_COMM_BASE_H__ 00018 #define __FTCD_COMM_BASE_H__ 00019 00020 #include <stdint.h> 00021 00022 /** 00023 * @file ftcd_comm_base.h 00024 * 00025 * Token [64bit] : The message identifier 00026 * Status [32 bit] : Status of message parameters (exists in response messages only) 00027 * Length [32bit] : The message length in bytes 00028 * Message [Length] : A message to be processed 00029 * Signature [32B] : The hash (SHA256) value of the message 00030 */ 00031 00032 00033 /** Unique message identifiers 00034 */ 00035 #define FTCD_MSG_HEADER_TOKEN_FCC { 0x6d, 0x62, 0x65, 0x64, 0x70, 0x72, 0x6f, 0x76 } 00036 #define FTCD_MSG_HEADER_TOKEN_SDA { 0x6d, 0x62, 0x65, 0x64, 0x64, 0x62, 0x61, 0x70 } 00037 #define FTCD_MSG_HEADER_TOKEN_SIZE_BYTES 8 00038 00039 00040 typedef enum { 00041 FTCD_COMM_STATUS_SUCCESS, 00042 FTCD_COMM_STATUS_ERROR, //generic error 00043 FTCD_COMM_INVALID_PARAMETER, 00044 FTCD_COMM_MEMORY_OUT, 00045 FTCD_COMM_FAILED_TO_READ_MESSAGE_SIZE, 00046 FTCD_COMM_FAILED_TO_READ_MESSAGE_BYTES, 00047 FTCD_COMM_FAILED_TO_READ_MESSAGE_SIGNATURE, 00048 FTCD_COMM_FAILED_TO_CALCULATE_MESSAGE_SIGNATURE, 00049 FTCD_COMM_INCONSISTENT_MESSAGE_SIGNATURE, 00050 FTCD_COMM_FAILED_TO_PROCESS_DATA, 00051 FTCD_COMM_FAILED_TO_PROCESS_MESSAGE, 00052 FTCD_COMM_FAILED_TO_SEND_VALID_RESPONSE, 00053 00054 FTCD_COMM_NETWORK_TIMEOUT, //socket timeout error 00055 FTCD_COMM_NETWORK_CONNECTION_ERROR, //socket error 00056 FTCD_COMM_INTERNAL_ERROR, 00057 00058 FTCD_COMM_STATUS_MAX_ERROR = 0xFFFFFFFF 00059 } ftcd_comm_status_e; 00060 00061 typedef enum { 00062 FTCD_COMM_NET_ENDIANNESS_LITTLE, 00063 FTCD_COMM_NET_ENDIANNESS_BIG, 00064 } ftcd_comm_network_endianness_e; 00065 00066 /** 00067 * \brief ::FtcdCommBase implements the logic of processing incoming requests from the remote Factory Tool Demo. 00068 */ 00069 class FtcdCommBase { 00070 00071 public: 00072 00073 FtcdCommBase(ftcd_comm_network_endianness_e network_endianness,const uint8_t *header_token = NULL, bool use_signature = false); 00074 00075 /** Not certain that we need to do anything here, but just in case we need 00076 * to do some clean-up at some point. 00077 */ 00078 virtual ~FtcdCommBase() = 0; 00079 00080 /** 00081 * Initializes Network interface and opens socket 00082 * Prints IP address 00083 */ 00084 virtual bool init(void); 00085 00086 /** 00087 * Closes the opened socket 00088 */ 00089 virtual void finish(void); 00090 00091 /** Wait and read complete message from the communication line. 00092 * The method waits in blocking mode for new message, 00093 * allocate and read the message, 00094 * and sets message_out and message_size_out 00095 * 00096 * @param message_out The message allocated and read from the communication line 00097 * @param message_size_out The message size in bytes 00098 * 00099 * @returns 00100 * FTCD_COMM_STATUS_SUCCESS on success, otherwise appropriate error from ftcd_comm_status_e 00101 */ 00102 virtual ftcd_comm_status_e wait_for_message(uint8_t **message_out, uint32_t *message_size_out); 00103 00104 /** Writes a response message to the communication line. 00105 * The method build response message with header and signature (if requested) 00106 * and writes it to the line 00107 * 00108 * @param response_message The message to send through the communication line medium 00109 * @param response_message_size The message size in bytes 00110 * 00111 * @returns 00112 * FTCD_COMM_STATUS_SUCCESS on success, otherwise appropriate error from ftcd_comm_status_e 00113 */ 00114 ftcd_comm_status_e send_response(const uint8_t *response_message, uint32_t response_message_size); 00115 00116 /** Writes a response message with status to the communication line. 00117 * The method build response message with status, header and signature (if requested) 00118 * and writes it to the line 00119 * 00120 * @param response_message The message to send through the communication line medium 00121 * @param response_message_size The message size in bytes 00122 * 00123 * @returns 00124 * FTCD_COMM_STATUS_SUCCESS on success, otherwise appropriate error from ftcd_comm_status_e 00125 */ 00126 ftcd_comm_status_e send_response(const uint8_t *response_message, uint32_t response_message_size, ftcd_comm_status_e status_code); 00127 00128 /** Writes an allocated response message to the communication line medium. 00129 * 00130 * @param response_message The message to send through the communication line medium 00131 * @param response_message_size The message size in bytes 00132 * 00133 * @returns 00134 * true upon success, false otherwise 00135 */ 00136 virtual bool send(const uint8_t *response_message, uint32_t response_message_size) = 0; 00137 00138 /** Detects the message token from the communication line medium. 00139 * 00140 * @returns 00141 * zero, if token detected and different value otherwise 00142 */ 00143 virtual ftcd_comm_status_e is_token_detected(void) = 0; 00144 00145 /** Reads the message size in bytes from the communication line medium. 00146 * This is the amount of bytes needed to allocate for the upcoming message bytes. 00147 * 00148 * @returns 00149 * The message size in bytes in case of success, zero bytes otherwise. 00150 */ 00151 virtual uint32_t read_message_size(void) = 0; 00152 00153 /** Reads the message size in bytes from the communication line medium. 00154 * This is the amount of bytes needed to allocate for the upcoming message bytes. 00155 * 00156 * @param message_out The buffer to read into and return to the caller. 00157 * @param message_size The message size in bytes. 00158 * 00159 * @returns 00160 * true upon success, false otherwise 00161 */ 00162 virtual bool read_message(uint8_t *message_out, size_t message_size) = 0; 00163 00164 /** Reads the message size in bytes from the communication line medium. 00165 * This is the amount of bytes needed to allocate for the upcoming message bytes. 00166 * 00167 * @param sig The buffer to read into and return to the caller. 00168 * @param sig_size The sig buffer size in bytes. 00169 * 00170 * @returns 00171 * The message size in bytes in case of success, zero bytes otherwise. 00172 */ 00173 virtual bool read_message_signature(uint8_t *sig, size_t sig_size) = 0; 00174 00175 protected: 00176 00177 /* Member point to the token array */ 00178 uint8_t *_header_token; 00179 00180 private: 00181 00182 /* Internal method that build response message with status, header and signature (if requested) 00183 * and writes it to the line */ 00184 ftcd_comm_status_e _send_response(const uint8_t *response_message, uint32_t response_message_size, bool send_status_code, ftcd_comm_status_e status_code); 00185 00186 /** Holds the requested network bytes order */ 00187 ftcd_comm_network_endianness_e _network_endianness; 00188 00189 /** Holds the requested message format (with token or without) */ 00190 bool _use_token; 00191 00192 /** Holds the requested message format (with signature or without) */ 00193 bool _use_signature; 00194 }; 00195 00196 #endif // __FTCD_COMM_BASE_H__
Generated on Tue Jul 12 2022 19:01:34 by
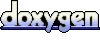