Simple interface for Mbed Cloud Client
Embed:
(wiki syntax)
Show/hide line numbers
fcc_bundle_utils.h
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // Licensed under the Apache License, Version 2.0 (the "License"); 00005 // you may not use this file except in compliance with the License. 00006 // You may obtain a copy of the License at 00007 // 00008 // http://www.apache.org/licenses/LICENSE-2.0 00009 // 00010 // Unless required by applicable law or agreed to in writing, software 00011 // distributed under the License is distributed on an "AS IS" BASIS, 00012 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 // See the License for the specific language governing permissions and 00014 // limitations under the License. 00015 // ---------------------------------------------------------------------------- 00016 00017 #ifndef __FCC_BUNDLE_UTILS_H__ 00018 #define __FCC_BUNDLE_UTILS_H__ 00019 00020 #include <stdlib.h> 00021 #include <stdbool.h> 00022 #include <inttypes.h> 00023 #include "fcc_status.h" 00024 #include "key_config_manager.h" 00025 #include "fcc_sotp.h" 00026 00027 #ifdef __cplusplus 00028 extern "C" { 00029 #endif 00030 00031 #define FCC_CBOR_MAP_LENGTH 2 00032 00033 /** 00034 * Names of key parameters 00035 */ 00036 #define FCC_BUNDLE_DATA_PARAMETER_NAME "Name" 00037 #define FCC_BUNDLE_DATA_PARAMETER_SCHEME "Type" 00038 #define FCC_BUNDLE_DATA_PARAMETER_FORMAT "Format" 00039 #define FCC_BUNDLE_DATA_PARAMETER_DATA "Data" 00040 #define FCC_BUNDLE_DATA_PARAMETER_ACL "ACL" 00041 #define FCC_BUNDLE_DATA_PARAMETER_ARRAY "DataArray" 00042 00043 /** 00044 * Types of key parameters 00045 */ 00046 typedef enum { 00047 FCC_BUNDLE_DATA_PARAM_NAME_TYPE, 00048 FCC_BUNDLE_DATA_PARAM_SCHEME_TYPE, 00049 FCC_BUNDLE_DATA_PARAM_FORMAT_TYPE, 00050 FCC_BUNDLE_DATA_PARAM_DATA_TYPE, 00051 FCC_BUNDLE_DATA_PARAM_ACL_TYPE, 00052 FCC_BUNDLE_DATA_PARAM_ARRAY_TYPE, 00053 FCC_BUNDLE_DATA_PARAM_MAX_TYPE 00054 } fcc_bundle_data_param_type_e; 00055 00056 /** 00057 * Key lookup record, correlating key's param type and name 00058 */ 00059 typedef struct fcc_bundle_data_param_lookup_record_ { 00060 fcc_bundle_data_param_type_e data_param_type; 00061 const char *data_param_name; 00062 } fcc_bundle_data_param_lookup_record_s; 00063 00064 /** 00065 * Key lookup table, correlating for each key its param type and param name 00066 */ 00067 static const fcc_bundle_data_param_lookup_record_s fcc_bundle_data_param_lookup_table[FCC_BUNDLE_DATA_PARAM_MAX_TYPE] = { 00068 { FCC_BUNDLE_DATA_PARAM_NAME_TYPE, FCC_BUNDLE_DATA_PARAMETER_NAME }, 00069 { FCC_BUNDLE_DATA_PARAM_SCHEME_TYPE, FCC_BUNDLE_DATA_PARAMETER_SCHEME }, 00070 { FCC_BUNDLE_DATA_PARAM_FORMAT_TYPE, FCC_BUNDLE_DATA_PARAMETER_FORMAT }, 00071 { FCC_BUNDLE_DATA_PARAM_DATA_TYPE, FCC_BUNDLE_DATA_PARAMETER_DATA }, 00072 { FCC_BUNDLE_DATA_PARAM_ACL_TYPE, FCC_BUNDLE_DATA_PARAMETER_ACL }, 00073 { FCC_BUNDLE_DATA_PARAM_ARRAY_TYPE, FCC_BUNDLE_DATA_PARAMETER_ARRAY } 00074 }; 00075 00076 /** 00077 * Source type of buffer 00078 */ 00079 typedef enum { 00080 FCC_EXTERNAL_BUFFER_TYPE, 00081 FCC_INTERNAL_BUFFER_TYPE, 00082 FCC_MAX_BUFFER_TYPE 00083 } fcc_bundle_buffer_type_e; 00084 00085 /** 00086 * Data formats supported by FC 00087 */ 00088 typedef enum { 00089 FCC_INVALID_DATA_FORMAT, 00090 FCC_DER_DATA_FORMAT, 00091 FCC_PEM_DATA_FORMAT, 00092 FCC_MAX_DATA_FORMAT 00093 } fcc_bundle_data_format_e; 00094 00095 /** 00096 * Names of data formats 00097 */ 00098 #define FCC_BUNDLE_DER_DATA_FORMAT_NAME "der" 00099 #define FCC_BUNDLE_PEM_DATA_FORMAT_NAME "pem" 00100 00101 /** 00102 * Group lookup record, correlating group's type and name 00103 */ 00104 typedef struct fcc_bundle_data_format_lookup_record_ { 00105 fcc_bundle_data_format_e data_format_type; 00106 const char *data_format_name; 00107 } fcc_bundle_data_format_lookup_record_s; 00108 00109 /** 00110 * Group lookup table, correlating for each group its type and name 00111 */ 00112 static const fcc_bundle_data_format_lookup_record_s fcc_bundle_data_format_lookup_table[FCC_MAX_DATA_FORMAT] = { 00113 { FCC_DER_DATA_FORMAT, FCC_BUNDLE_DER_DATA_FORMAT_NAME }, 00114 { FCC_PEM_DATA_FORMAT, FCC_BUNDLE_PEM_DATA_FORMAT_NAME }, 00115 }; 00116 00117 /** 00118 * Key types supported by FC 00119 */ 00120 typedef enum { 00121 FCC_INVALID_KEY_TYPE, 00122 FCC_ECC_PRIVATE_KEY_TYPE,//do not change this type's place.FCC_ECC_PRIVATE_KEY_TYPE should be at first place. 00123 FCC_ECC_PUBLIC_KEY_TYPE, 00124 FCC_RSA_PRIVATE_KEY_TYPE, 00125 FCC_RSA_PUBLIC_KEY_TYPE, 00126 FCC_SYM_KEY_TYPE, 00127 FCC_MAX_KEY_TYPE 00128 } fcc_bundle_key_type_e; 00129 00130 typedef struct fcc_bundle_data_param_ { 00131 uint8_t *name; 00132 size_t name_len; 00133 fcc_bundle_data_format_e format; 00134 fcc_bundle_key_type_e type; 00135 uint8_t *data; 00136 size_t data_size; 00137 uint8_t *data_der; 00138 size_t data_der_size; 00139 fcc_bundle_buffer_type_e data_type; 00140 uint8_t *acl; 00141 size_t acl_size; 00142 cn_cbor *array_cn; 00143 } fcc_bundle_data_param_s; 00144 00145 00146 /** Frees all allocated memory of data parameter struct and sets initial values. 00147 * 00148 * @param data_param[in/out] The data parameter structure 00149 */ 00150 void fcc_bundle_clean_and_free_data_param(fcc_bundle_data_param_s *data_param); 00151 00152 /** Gets data buffer from cbor struct. 00153 * 00154 * @param data_cb[in] The cbor text structure 00155 * @param out_data_buffer[out] The out buffer for string data 00156 * @param out_size[out] The actual size of output buffer 00157 * 00158 * @return 00159 * true for success, false otherwise. 00160 */ 00161 bool get_data_buffer_from_cbor(const cn_cbor *data_cb, uint8_t **out_data_buffer, size_t *out_size); 00162 00163 /** Processes keys list. 00164 * The function extracts data parameters for each key and stores its according to it type. 00165 * 00166 * @param keys_list_cb[in] The cbor structure with keys list. 00167 * 00168 * @return 00169 * fcc_status_e status. 00170 */ 00171 fcc_status_e fcc_bundle_process_keys(const cn_cbor *keys_list_cb); 00172 00173 /** Processes certificate list. 00174 * The function extracts data parameters for each certificate and stores it. 00175 * 00176 * @param certs_list_cb[in] The cbor structure with certificate list. 00177 * 00178 * @return 00179 * fcc_status_e status. 00180 */ 00181 fcc_status_e fcc_bundle_process_certificates(const cn_cbor *certs_list_cb); 00182 /** Processes certificate chain list. 00183 * The function extracts data parameters for each certificate chain and stores it. 00184 * 00185 * @param certs_list_cb[in] The cbor structure with certificate chain list. 00186 * 00187 * @return 00188 * fcc_status_e status. 00189 */ 00190 fcc_status_e fcc_bundle_process_certificate_chains(const cn_cbor *cert_chains_list_cb); 00191 00192 /** Processes configuration parameters list. 00193 * The function extracts data parameters for each config param and stores it. 00194 * 00195 * @param config_params_list_cb[in] The cbor structure with config param list. 00196 * 00197 * @return 00198 * fcc_status_e status. 00199 */ 00200 fcc_status_e fcc_bundle_process_config_params(const cn_cbor *config_params_list_cb); 00201 00202 /** Gets data parameters. 00203 * 00204 * The function goes over all existing parameters (name,type,format,data,acl and etc) and 00205 * tries to find correlating parameter in cbor structure and saves it to data parameter structure. 00206 * 00207 * @param data_param_cb[in] The cbor structure with relevant data parameters. 00208 * @param data_param[out] The data parameter structure 00209 * 00210 * @return 00211 * true for success, false otherwise. 00212 */ 00213 bool fcc_bundle_get_data_param(const cn_cbor *data_param_list_cb, fcc_bundle_data_param_s *data_param); 00214 00215 /** Gets type of key form cbor structure 00216 * 00217 * The function goes over all key types and compares it with type inside cbor structure. 00218 * 00219 * @param key_type_cb[in] The cbor structure with key type data. 00220 * @param key_type[out] The key type 00221 * 00222 * @return 00223 * true for success, false otherwise. 00224 */ 00225 bool fcc_bundle_get_key_type(const cn_cbor *key_type_cb, fcc_bundle_key_type_e *key_type); 00226 00227 /** Writes buffer to SOTP 00228 * 00229 * @param cbor_bytes[in] The pointer to a cn_cbor object of type CN_CBOR_BYTES. 00230 * @param sotp_type[in] enum representing the type of the item to be stored in SOTP. 00231 * @return 00232 * true for success, false otherwise. 00233 */ 00234 00235 fcc_status_e fcc_bundle_process_sotp_buffer(cn_cbor *cbor_bytes, sotp_type_e sotp_type); 00236 00237 /** Gets the status groups value 00238 * 00239 * - if value is '0' - set status to false 00240 * - if value is '1' - set status to true 00241 * 00242 * @param cbor_blob[in] The pointer to main CBOR blob. 00243 * @param cbor_group_name[in] CBOT group name. 00244 * @param cbor_group_name_size[in] CBOR group name size . 00245 * @param fcc_field_status[out] Status of the field. 00246 * 00247 * @return 00248 * One of FCC_STATUS_* error codes 00249 */ 00250 fcc_status_e bundle_process_status_field(const cn_cbor *cbor_blob, char *cbor_group_name, size_t cbor_group_name_size, bool *fcc_field_status); 00251 00252 /** The function sets factory disable flag to sotp. 00253 * 00254 * @return 00255 * One of FCC_STATUS_* error codes 00256 */ 00257 fcc_status_e fcc_bundle_factory_disable(void); 00258 #ifdef __cplusplus 00259 } 00260 #endif 00261 00262 #endif //__FCC_BUNDLE_UTILS_H__
Generated on Tue Jul 12 2022 19:01:34 by
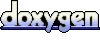