Simple interface for Mbed Cloud Client
Embed:
(wiki syntax)
Show/hide line numbers
esfs_file_name.c
00001 /* 00002 * Copyright (c) 2017 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "pal.h" 00018 #include "pal_Crypto.h" 00019 #include <string.h> 00020 #include "esfs.h" 00021 00022 00023 static char IntToBase64Char(uint8_t intVal) 00024 { 00025 const char* base64Digits = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789#_"; 00026 return base64Digits[intVal & 0x3F]; 00027 } 00028 esfs_result_e esfs_EncodeBase64(const void* buffer, uint32_t bufferSize, char* string, uint32_t stringSize) 00029 { 00030 uint32_t bitOffset = 0; 00031 00032 const uint8_t* readPtr = (const uint8_t*)buffer; 00033 const uint8_t* bufferEnd = (const uint8_t*)buffer + bufferSize; 00034 00035 char* writePtr = string; 00036 char* stringEnd = string + stringSize - 1; 00037 00038 if ((NULL == string) || (NULL == buffer) || (stringSize == 0)) 00039 return ESFS_INVALID_PARAMETER; 00040 00041 stringSize--; 00042 while(readPtr < bufferEnd && writePtr < stringEnd) 00043 { 00044 uint8_t tempVal = 0; 00045 switch (bitOffset) 00046 { 00047 case 0: 00048 *writePtr++ = IntToBase64Char(*readPtr >> 2); // take upper 6 bits 00049 break; 00050 case 6: 00051 tempVal = *readPtr++ << 4; 00052 if (readPtr < bufferEnd) 00053 tempVal |= *readPtr >> 4; 00054 *writePtr++ = IntToBase64Char(tempVal); 00055 break; 00056 case 4: 00057 tempVal = *readPtr++ << 2; 00058 if (readPtr < bufferEnd) 00059 tempVal |= *readPtr >> 6; 00060 *writePtr++ = IntToBase64Char(tempVal); 00061 break; 00062 case 2: 00063 *writePtr++ = IntToBase64Char(*readPtr++); 00064 break; 00065 default: 00066 return ESFS_INTERNAL_ERROR; // we should never reach this code. 00067 } 00068 bitOffset = (bitOffset + 6) & 0x7; 00069 } 00070 while (bitOffset > 0 && writePtr < stringEnd) 00071 { 00072 *writePtr++ = '!'; 00073 bitOffset = (bitOffset + 6) & 0x7; 00074 } 00075 *writePtr = 0; 00076 00077 if ((readPtr < bufferEnd) || (bitOffset != 0)) 00078 return (ESFS_BUFFER_TOO_SMALL); 00079 00080 return(ESFS_SUCCESS); 00081 } 00082 00083 /* size_of_file_name should should include the null at the end of the string. In our case 9*/ 00084 esfs_result_e esfs_get_name_from_blob(const uint8_t *blob, uint32_t blob_length,char *file_name, uint32_t size_of_file_name) 00085 { 00086 unsigned char output[32] = {0}; 00087 palStatus_t pal_result; 00088 esfs_result_e esfs_result; 00089 pal_result = pal_sha256 (blob, blob_length, output); 00090 if (PAL_SUCCESS != pal_result) 00091 return ESFS_INTERNAL_ERROR; 00092 esfs_result = esfs_EncodeBase64(output, (size_of_file_name - 1)*6/8, file_name, size_of_file_name); 00093 return (esfs_result); 00094 00095 00096 } 00097 00098
Generated on Tue Jul 12 2022 19:01:34 by
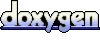