Simple interface for Mbed Cloud Client
Embed:
(wiki syntax)
Show/hide line numbers
arm_uc_test_sanity.cpp
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // SPDX-License-Identifier: Apache-2.0 00005 // 00006 // Licensed under the Apache License, Version 2.0 (the "License"); 00007 // you may not use this file except in compliance with the License. 00008 // You may obtain a copy of the License at 00009 // 00010 // http://www.apache.org/licenses/LICENSE-2.0 00011 // 00012 // Unless required by applicable law or agreed to in writing, software 00013 // distributed under the License is distributed on an "AS IS" BASIS, 00014 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 // See the License for the specific language governing permissions and 00016 // limitations under the License. 00017 // ---------------------------------------------------------------------------- 00018 00019 #include "test-utils/arm_uc_test_sanity.h" 00020 00021 #include "update-client-firmware-manager/arm_uc_firmware_manager.h" 00022 #include "update-client-paal/arm_uc_paal_update.h" 00023 00024 #include "pal.h" 00025 00026 #include "test-utils/arm_uc_test_alice.h" 00027 00028 #ifdef TARGET_LIKE_POSIX 00029 #include <unistd.h> 00030 #define __WFI() sleep(1) 00031 #endif 00032 00033 using namespace utest::v1; 00034 00035 static uint8_t temp_nc[16]; 00036 static arm_uc_buffer_t keyBuffer = { .size_max = 32, .size = 32, .ptr = (uint8_t*) key }; 00037 static arm_uc_buffer_t ivBuffer = { .size_max = 16, .size = 16, .ptr = (uint8_t*) temp_nc }; 00038 static arm_uc_buffer_t hashBuffer = { .size_max = 32, .size = 32, .ptr = (uint8_t*) hash }; 00039 00040 #define BUF_SIZE 1024 00041 static uint8_t buf[BUF_SIZE] = {0}; 00042 static arm_uc_buffer_t fragment = { 00043 .size_max = BUF_SIZE, 00044 .size = 0, 00045 .ptr = buf 00046 }; 00047 00048 static bool FLAG_INITIALIZE_DONE = false; 00049 static bool FLAG_PREPARE_DONE = false; 00050 static bool FLAG_WRITE_DONE = false; 00051 static bool FLAG_FINALIZE_DONE = false; 00052 static bool FLAG_GET_FIRMWARE_DETAILS_DONE = false; 00053 00054 static void event_handler(uint32_t event) 00055 { 00056 switch (event) 00057 { 00058 case UCFM_EVENT_INITIALIZE_DONE: 00059 printf("UCFM_EVENT_INITIALIZE_DONE\r\n"); 00060 FLAG_INITIALIZE_DONE = true; 00061 break; 00062 00063 case UCFM_EVENT_PREPARE_DONE: 00064 printf("UCFM_EVENT_PREPARE_DONE\r\n"); 00065 FLAG_PREPARE_DONE = true; 00066 break; 00067 00068 case UCFM_EVENT_WRITE_DONE: 00069 FLAG_WRITE_DONE = true; 00070 break; 00071 00072 case UCFM_EVENT_FINALIZE_DONE: 00073 printf("UCFM_EVENT_FINALIZE_DONE\r\n"); 00074 FLAG_FINALIZE_DONE = true; 00075 break; 00076 00077 case UCFM_EVENT_GET_FIRMWARE_DETAILS_DONE: 00078 printf("UCFM_EVENT_GET_FIRMWARE_DETAILS_DONE\r\n"); 00079 FLAG_GET_FIRMWARE_DETAILS_DONE = true; 00080 break; 00081 00082 default: 00083 TEST_ASSERT_MESSAGE(false, "callback failed"); 00084 break; 00085 } 00086 } 00087 00088 control_t test_update_check_input_buffer() 00089 { 00090 arm_uc_error_t result; 00091 result.code = ERR_NONE; 00092 00093 /* Setup new firmware */ 00094 memcpy(temp_nc, nc, sizeof(nc)); 00095 ARM_UCFM_Setup_t setup; 00096 setup.mode = UCFM_MODE_AES_CTR_256_SHA_256; 00097 setup.key = &keyBuffer; 00098 setup.iv = &ivBuffer; 00099 setup.hash = &hashBuffer; 00100 setup.package_id = 0; 00101 setup.package_size = sizeof(ecila); 00102 00103 FLAG_PREPARE_DONE = false; 00104 printf("Setup\r\n"); 00105 00106 /* temporary buffer */ 00107 arm_uc_buffer_t buffer = { 00108 .size_max = BUF_SIZE, 00109 .size = 0, 00110 .ptr = buf 00111 }; 00112 00113 /* firmware details struct */ 00114 arm_uc_firmware_details_t details = { 0 }; 00115 00116 memcpy(details.hash, hashBuffer.ptr, 32); 00117 details.version = 0; 00118 details.size = sizeof(ecila); 00119 00120 result = ARM_UC_FirmwareManager.Initialize(event_handler); 00121 TEST_ASSERT_TRUE_MESSAGE(result.error == ERR_NONE, "initialize"); 00122 while(!FLAG_INITIALIZE_DONE) 00123 { 00124 ARM_UC_ProcessQueue(); 00125 __WFI(); 00126 } 00127 00128 result = ARM_UC_FirmwareManager.Prepare(&setup, &details, &buffer); 00129 TEST_ASSERT_TRUE_MESSAGE(result.error == ERR_NONE, "setup"); 00130 while(!FLAG_PREPARE_DONE) 00131 { 00132 ARM_UC_ProcessQueue(); 00133 __WFI(); 00134 } 00135 00136 fragment.size = fragment.size_max; 00137 result = ARM_UC_FirmwareManager.Write(&fragment); 00138 TEST_ASSERT_EQUAL_HEX(ERR_NONE, result.error); 00139 while(!UCFM_EVENT_WRITE_DONE) 00140 { 00141 ARM_UC_ProcessQueue(); 00142 __WFI(); 00143 } 00144 00145 fragment.size = fragment.size_max + 1; 00146 result = ARM_UC_FirmwareManager.Write(&fragment); 00147 TEST_ASSERT_EQUAL_HEX(FIRM_ERR_INVALID_PARAMETER, result.code); 00148 00149 fragment.size_max = 0; 00150 result = ARM_UC_FirmwareManager.Write(&fragment); 00151 TEST_ASSERT_EQUAL_HEX(FIRM_ERR_INVALID_PARAMETER, result.code); 00152 00153 fragment.ptr = NULL; 00154 result = ARM_UC_FirmwareManager.Write(&fragment); 00155 TEST_ASSERT_EQUAL_HEX(FIRM_ERR_INVALID_PARAMETER, result.code); 00156 00157 result = ARM_UC_FirmwareManager.Write(NULL); 00158 TEST_ASSERT_EQUAL_HEX(FIRM_ERR_INVALID_PARAMETER, result.code); 00159 00160 return CaseNext; 00161 } 00162
Generated on Tue Jul 12 2022 19:01:33 by
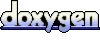