Simple interface for Mbed Cloud Client
Embed:
(wiki syntax)
Show/hide line numbers
arm_uc_test_getactivehash.cpp
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // SPDX-License-Identifier: Apache-2.0 00005 // 00006 // Licensed under the Apache License, Version 2.0 (the "License"); 00007 // you may not use this file except in compliance with the License. 00008 // You may obtain a copy of the License at 00009 // 00010 // http://www.apache.org/licenses/LICENSE-2.0 00011 // 00012 // Unless required by applicable law or agreed to in writing, software 00013 // distributed under the License is distributed on an "AS IS" BASIS, 00014 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 // See the License for the specific language governing permissions and 00016 // limitations under the License. 00017 // ---------------------------------------------------------------------------- 00018 00019 #include "test-utils/arm_uc_test_getactivehash.h" 00020 00021 #include "update-client-firmware-manager/arm_uc_firmware_manager.h" 00022 #include "update-client-paal/arm_uc_paal_update.h" 00023 #include "update-client-common/arm_uc_metadata_header_v2.h" 00024 00025 #include <mbedtls/sha256.h> 00026 00027 #if defined(TARGET_LIKE_POSIX) 00028 #include <pal_plat_update.h> 00029 #include <unistd.h> 00030 #define __WFI() usleep(100) 00031 #endif 00032 00033 using namespace utest::v1; 00034 00035 static bool FLAG_INITIALIZE_DONE = false; 00036 static bool FLAG_GET_ACTIVE_FIRMWARE_DETAILS_DONE = false; 00037 00038 static void event_handler(uint32_t event) 00039 { 00040 switch (event) 00041 { 00042 case UCFM_EVENT_INITIALIZE_DONE: 00043 printf("UCFM_EVENT_INITIALIZE_DONE\r\n"); 00044 FLAG_INITIALIZE_DONE = true; 00045 break; 00046 00047 case UCFM_EVENT_GET_ACTIVE_FIRMWARE_DETAILS_DONE: 00048 printf("UCFM_EVENT_GET_ACTIVE_FIRMWARE_DETAILS_DONE\r\n"); 00049 FLAG_GET_ACTIVE_FIRMWARE_DETAILS_DONE = true; 00050 break; 00051 00052 case UCFM_EVENT_GET_ACTIVE_FIRMWARE_DETAILS_ERROR: 00053 TEST_ASSERT_MESSAGE(false, "UCFM_EVENT_GET_ACTIVE_FIRMWARE_DETAILS_ERROR"); 00054 break; 00055 00056 default: 00057 TEST_ASSERT_MESSAGE(false, "callback failed"); 00058 break; 00059 } 00060 } 00061 00062 control_t test_get_active_hash() 00063 { 00064 arm_uc_error_t result; 00065 00066 #if defined(TARGET_K64F) 00067 int32_t rc; 00068 00069 FlashIAP flash; 00070 rc = flash.init(); 00071 TEST_ASSERT_TRUE_MESSAGE(rc == 0, "main: FlashIAP::init failed"); 00072 00073 uint32_t sector_size = flash.get_sector_size(MBED_CONF_UPDATE_CLIENT_APPLICATION_DETAILS); 00074 TEST_ASSERT_TRUE_MESSAGE(sector_size == 0x1000, "main: FlashIAP::get_sector_size failed"); 00075 00076 rc = flash.erase(MBED_CONF_UPDATE_CLIENT_APPLICATION_DETAILS, sector_size); 00077 TEST_ASSERT_TRUE_MESSAGE(rc == 0, "main: FlashIAP::erase failed"); 00078 00079 uint8_t buffer[1024] = { 0 }; 00080 arm_uc_firmware_details_t details = { 0 }; 00081 arm_uc_buffer_t output = { 00082 .size_max = 1024, 00083 .size = 0, 00084 .ptr = buffer 00085 }; 00086 00087 result = arm_uc_create_internal_header_v2(&details, &output); 00088 TEST_ASSERT_TRUE_MESSAGE(result.error == ERR_NONE, "main: arm_uc_create_internal_header_v2 failed"); 00089 00090 rc = flash.program(output.ptr, MBED_CONF_UPDATE_CLIENT_APPLICATION_DETAILS, output.size); 00091 TEST_ASSERT_TRUE_MESSAGE(rc == 0, "main: FlashIAP::program failed"); 00092 00093 #elif defined(TARGET_LIKE_POSIX) 00094 #warning Active firmware details not implemented on Linux 00095 #endif 00096 00097 result = ARM_UC_FirmwareManager.Initialize(event_handler); 00098 TEST_ASSERT_TRUE_MESSAGE(result.error == ERR_NONE, "initialize"); 00099 while(!FLAG_INITIALIZE_DONE) 00100 { 00101 ARM_UC_ProcessQueue(); 00102 __WFI(); 00103 } 00104 00105 FLAG_GET_ACTIVE_FIRMWARE_DETAILS_DONE = false; 00106 00107 printf("GetActiveHash\r\n"); 00108 arm_uc_firmware_details_t readback = { 0 }; 00109 result = ARM_UC_FirmwareManager.GetActiveFirmwareDetails(&readback); 00110 if (result.error != ERR_NONE) 00111 { 00112 printf("error: %s\r\n", ARM_UC_err2Str(result)); 00113 } 00114 TEST_ASSERT_TRUE_MESSAGE(result.error == ERR_NONE, "GetActiveHash"); 00115 while(!FLAG_GET_ACTIVE_FIRMWARE_DETAILS_DONE) 00116 { 00117 ARM_UC_ProcessQueue(); 00118 __WFI(); 00119 } 00120 00121 return CaseNext; 00122 } 00123
Generated on Tue Jul 12 2022 19:01:33 by
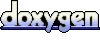