Simple interface for Mbed Cloud Client
Embed:
(wiki syntax)
Show/hide line numbers
arm_uc_socket_help.c
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // SPDX-License-Identifier: Apache-2.0 00005 // 00006 // Licensed under the Apache License, Version 2.0 (the "License"); 00007 // you may not use this file except in compliance with the License. 00008 // You may obtain a copy of the License at 00009 // 00010 // http://www.apache.org/licenses/LICENSE-2.0 00011 // 00012 // Unless required by applicable law or agreed to in writing, software 00013 // distributed under the License is distributed on an "AS IS" BASIS, 00014 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 // See the License for the specific language governing permissions and 00016 // limitations under the License. 00017 // ---------------------------------------------------------------------------- 00018 00019 #include "arm_uc_socket_help.h" 00020 00021 #include <string.h> 00022 00023 /** 00024 * @brief Helper function for picking out values from an HTTP header. 00025 * @details The buffer is searched for the tag provided tag and if found the 00026 * remainder of that line is MOVED to the front of the buffer and the 00027 * size is shrunk to only include the detected value. 00028 * 00029 * @param buffer Pointer to an arm_uc_buffer_t. 00030 * @param tag_buffer Pointer to tag (a string). 00031 * @param tag_size Length of the tag. 00032 * @return True if tag was found. False otherwise. 00033 */ 00034 bool arm_uc_socket_trim_value(arm_uc_buffer_t* buffer, 00035 const char* tag_buffer, 00036 uint32_t tag_size) 00037 { 00038 /* default return value */ 00039 bool result = false; 00040 00041 /* check for NULL pointers */ 00042 if (buffer && buffer->ptr && tag_buffer) 00043 { 00044 /* search for the tag */ 00045 uint32_t start = arm_uc_strnstrn(buffer->ptr, 00046 buffer->size, 00047 (const uint8_t*) tag_buffer, 00048 tag_size); 00049 00050 /* check if index is within bounds */ 00051 if (start < buffer->size) 00052 { 00053 /* remove tag */ 00054 start += tag_size; 00055 00056 /* remove ": " between tag and value */ 00057 start += 2; 00058 00059 /* search for the end of the line */ 00060 uint32_t length = arm_uc_strnstrn(&(buffer->ptr[start]), 00061 buffer->size - start, 00062 (const uint8_t*) "\r", 00063 1); 00064 00065 /* move value to front of buffer if all indices are within bounds */ 00066 if ((start + length) < buffer->size) 00067 { 00068 /* memmove handles overlapping regions */ 00069 memmove(buffer->ptr, &(buffer->ptr[start]), length); 00070 00071 /* resize buffer */ 00072 buffer->size = length; 00073 00074 /* set return value to success */ 00075 result = true; 00076 } 00077 } 00078 } 00079 00080 return result; 00081 }
Generated on Tue Jul 12 2022 19:01:33 by
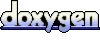