Simple interface for Mbed Cloud Client
Embed:
(wiki syntax)
Show/hide line numbers
arm_uc_paal_update_api.h
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // SPDX-License-Identifier: Apache-2.0 00005 // 00006 // Licensed under the Apache License, Version 2.0 (the "License"); 00007 // you may not use this file except in compliance with the License. 00008 // You may obtain a copy of the License at 00009 // 00010 // http://www.apache.org/licenses/LICENSE-2.0 00011 // 00012 // Unless required by applicable law or agreed to in writing, software 00013 // distributed under the License is distributed on an "AS IS" BASIS, 00014 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 // See the License for the specific language governing permissions and 00016 // limitations under the License. 00017 // ---------------------------------------------------------------------------- 00018 00019 #ifndef ARM_UC_PAAL_UPDATE_API_H 00020 #define ARM_UC_PAAL_UPDATE_API_H 00021 00022 /* Not including arm_uc_common.h to avoid the scheduler from being 00023 included in the mbed-bootloader. 00024 */ 00025 #include "update-client-common/arm_uc_error.h" 00026 #include "update-client-common/arm_uc_types.h" 00027 00028 #include <stdint.h> 00029 00030 /** 00031 * @brief Prototype for event handler. 00032 */ 00033 typedef void (*ARM_UC_PAAL_UPDATE_SignalEvent_t)(uint32_t event); 00034 00035 /** 00036 * @brief Asynchronous events. 00037 */ 00038 enum 00039 { 00040 ARM_UC_PAAL_EVENT_INITIALIZE_DONE, 00041 ARM_UC_PAAL_EVENT_PREPARE_DONE, 00042 ARM_UC_PAAL_EVENT_WRITE_DONE, 00043 ARM_UC_PAAL_EVENT_FINALIZE_DONE, 00044 ARM_UC_PAAL_EVENT_READ_DONE, 00045 ARM_UC_PAAL_EVENT_ACTIVATE_DONE, 00046 ARM_UC_PAAL_EVENT_GET_ACTIVE_FIRMWARE_DETAILS_DONE, 00047 ARM_UC_PAAL_EVENT_GET_FIRMWARE_DETAILS_DONE, 00048 ARM_UC_PAAL_EVENT_GET_INSTALLER_DETAILS_DONE, 00049 ARM_UC_PAAL_EVENT_INITIALIZE_ERROR, 00050 ARM_UC_PAAL_EVENT_PREPARE_ERROR, 00051 ARM_UC_PAAL_EVENT_WRITE_ERROR, 00052 ARM_UC_PAAL_EVENT_FINALIZE_ERROR, 00053 ARM_UC_PAAL_EVENT_READ_ERROR, 00054 ARM_UC_PAAL_EVENT_ACTIVATE_ERROR, 00055 ARM_UC_PAAL_EVENT_GET_ACTIVE_FIRMWARE_DETAILS_ERROR, 00056 ARM_UC_PAAL_EVENT_GET_FIRMWARE_DETAILS_ERROR, 00057 ARM_UC_PAAL_EVENT_GET_INSTALLER_DETAILS_ERROR, 00058 }; 00059 00060 /** 00061 * @brief Bitmap with supported header features. 00062 * @details The PAAL Update implementation indicates what features are 00063 * supported. This can be used after a call to one of the GetDetails 00064 * to see which fields have valid values and what fields should be set 00065 * in the call to Prepare. 00066 */ 00067 typedef struct _ARM_UC_PAAL_UPDATE_CAPABILITIES { 00068 uint32_t installer_arm_hash: 1; 00069 uint32_t installer_oem_hash: 1; 00070 uint32_t installer_layout: 1; 00071 uint32_t firmware_hash: 1; 00072 uint32_t firmware_hmac: 1; 00073 uint32_t firmware_campaign: 1; 00074 uint32_t firmware_version: 1; 00075 uint32_t firmware_size: 1; 00076 uint32_t reserved: 24; 00077 } ARM_UC_PAAL_UPDATE_CAPABILITIES; 00078 00079 /** 00080 * @brief Structure definition holding API function pointers. 00081 */ 00082 typedef struct _ARM_UC_PAAL_UPDATE { 00083 00084 /** 00085 * @brief Initialize the underlying storage and set the callback handler. 00086 * 00087 * @param callback Function pointer to event handler. 00088 * @return Returns ERR_NONE on accept, and signals the event handler with 00089 * either DONE or ERROR when complete. 00090 * Returns ERR_INVALID_PARAMETER on reject, and no signal is sent. 00091 */ 00092 arm_uc_error_t (*Initialize)(ARM_UC_PAAL_UPDATE_SignalEvent_t callback); 00093 00094 /** 00095 * @brief Get a bitmap indicating supported features. 00096 * @details The bitmap is used in conjunction with the firmware and 00097 * installer details struct to indicate what fields are supported 00098 * and which values are valid. 00099 * 00100 * @return Capability bitmap. 00101 */ 00102 ARM_UC_PAAL_UPDATE_CAPABILITIES (*GetCapabilities)(void); 00103 00104 /** 00105 * @brief Get maximum number of supported storage locations. 00106 * 00107 * @return Number of storage locations. 00108 */ 00109 uint32_t (*GetMaxID)(void); 00110 00111 /** 00112 * @brief Prepare the storage layer for a new firmware image. 00113 * @details The storage location is set up to receive an image with 00114 * the details passed in the details struct. 00115 * 00116 * @param location Storage location ID. 00117 * @param details Pointer to a struct with firmware details. 00118 * @param buffer Temporary buffer for formatting and storing metadata. 00119 * @return Returns ERR_NONE on accept, and signals the event handler with 00120 * either DONE or ERROR when complete. 00121 * Returns ERR_INVALID_PARAMETER on reject, and no signal is sent. 00122 */ 00123 arm_uc_error_t (*Prepare)(uint32_t location, 00124 const arm_uc_firmware_details_t* details, 00125 arm_uc_buffer_t* buffer); 00126 00127 /** 00128 * @brief Write a fragment to the indicated storage location. 00129 * @details The storage location must have been allocated using the Prepare 00130 * call. The call is expected to write the entire fragment before 00131 * signaling completion. 00132 * 00133 * @param location Storage location ID. 00134 * @param offset Offset in bytes to where the fragment should be written. 00135 * @param buffer Pointer to buffer struct with fragment. 00136 * @return Returns ERR_NONE on accept, and signals the event handler with 00137 * either DONE or ERROR when complete. 00138 * Returns ERR_INVALID_PARAMETER on reject, and no signal is sent. 00139 */ 00140 arm_uc_error_t (*Write)(uint32_t location, 00141 uint32_t offset, 00142 const arm_uc_buffer_t* buffer); 00143 00144 /** 00145 * @brief Close storage location for writing and flush pending data. 00146 * 00147 * @param location Storage location ID. 00148 * @return Returns ERR_NONE on accept, and signals the event handler with 00149 * either DONE or ERROR when complete. 00150 * Returns ERR_INVALID_PARAMETER on reject, and no signal is sent. 00151 */ 00152 arm_uc_error_t (*Finalize)(uint32_t location); 00153 00154 /** 00155 * @brief Read a fragment from the indicated storage location. 00156 * @details The function will read until the buffer is full or the end of 00157 * the storage location has been reached. The actual amount of 00158 * bytes read is set in the buffer struct. 00159 * 00160 * @param location Storage location ID. 00161 * @param offset Offset in bytes to read from. 00162 * @param buffer Pointer to buffer struct to store fragment. buffer->size 00163 * contains the intended read size. 00164 * @return Returns ERR_NONE on accept, and signals the event handler with 00165 * either DONE or ERROR when complete. 00166 * Returns ERR_INVALID_PARAMETER on reject, and no signal is sent. 00167 * buffer->size contains actual bytes read on return. 00168 */ 00169 arm_uc_error_t (*Read)(uint32_t location, 00170 uint32_t offset, 00171 arm_uc_buffer_t* buffer); 00172 00173 /** 00174 * @brief Set the firmware image in the slot to be the new active image. 00175 * @details This call is responsible for initiating the process for 00176 * applying a new/different image. Depending on the platform this 00177 * could be: 00178 * * An empty call, if the installer can deduce which slot to 00179 * choose from based on the firmware details. 00180 * * Setting a flag to indicate which slot to use next. 00181 * * Decompressing/decrypting/installing the firmware image on 00182 * top of another. 00183 * 00184 * @param location Storage location ID. 00185 * @return Returns ERR_NONE on accept, and signals the event handler with 00186 * either DONE or ERROR when complete. 00187 * Returns ERR_INVALID_PARAMETER on reject, and no signal is sent. 00188 */ 00189 arm_uc_error_t (*Activate)(uint32_t location); 00190 00191 /** 00192 * @brief Get firmware details for the actively running firmware. 00193 * @details This call populates the passed details struct with information 00194 * about the currently active firmware image. Only the fields 00195 * marked as supported in the capabilities bitmap will have valid 00196 * values. 00197 * 00198 * @param details Pointer to firmware details struct to be populated. 00199 * @return Returns ERR_NONE on accept, and signals the event handler with 00200 * either DONE or ERROR when complete. 00201 * Returns ERR_INVALID_PARAMETER on reject, and no signal is sent. 00202 */ 00203 arm_uc_error_t (*GetActiveFirmwareDetails)(arm_uc_firmware_details_t* details); 00204 00205 /** 00206 * @brief Get firmware details for the firmware image in the slot passed. 00207 * @details This call populates the passed details struct with information 00208 * about the firmware image in the slot passed. Only the fields 00209 * marked as supported in the capabilities bitmap will have valid 00210 * values. 00211 * 00212 * @param details Pointer to firmware details struct to be populated. 00213 * @return Returns ERR_NONE on accept, and signals the event handler with 00214 * either DONE or ERROR when complete. 00215 * Returns ERR_INVALID_PARAMETER on reject, and no signal is sent. 00216 */ 00217 arm_uc_error_t (*GetFirmwareDetails)(uint32_t location, 00218 arm_uc_firmware_details_t* details); 00219 00220 /** 00221 * @brief Get details for the component responsible for installation. 00222 * @details This call populates the passed details struct with information 00223 * about the local installer. Only the fields marked as supported 00224 * in the capabilities bitmap will have valid values. The 00225 * installer could be the bootloader, a recovery image, or some 00226 * other component responsible for applying the new firmware 00227 * image. 00228 * 00229 * @param details Pointer to installer details struct to be populated. 00230 * @return Returns ERR_NONE on accept, and signals the event handler with 00231 * either DONE or ERROR when complete. 00232 * Returns ERR_INVALID_PARAMETER on reject, and no signal is sent. 00233 */ 00234 arm_uc_error_t (*GetInstallerDetails)(arm_uc_installer_details_t* details); 00235 00236 } ARM_UC_PAAL_UPDATE; 00237 00238 #endif /* ARM_UC_PAAL_UPDATE_API_H */
Generated on Tue Jul 12 2022 19:01:33 by
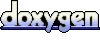