Simple interface for Mbed Cloud Client
Embed:
(wiki syntax)
Show/hide line numbers
MbedCloudClient.cpp
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // SPDX-License-Identifier: Apache-2.0 00005 // 00006 // Licensed under the Apache License, Version 2.0 (the "License"); 00007 // you may not use this file except in compliance with the License. 00008 // You may obtain a copy of the License at 00009 // 00010 // http://www.apache.org/licenses/LICENSE-2.0 00011 // 00012 // Unless required by applicable law or agreed to in writing, software 00013 // distributed under the License is distributed on an "AS IS" BASIS, 00014 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 // See the License for the specific language governing permissions and 00016 // limitations under the License. 00017 // ---------------------------------------------------------------------------- 00018 00019 #include "mbed-cloud-client/MbedCloudClientConfig.h" 00020 #include "mbed-cloud-client/MbedCloudClient.h" 00021 #include "mbed-cloud-client/SimpleM2MResource.h" 00022 00023 #include "ns_hal_init.h" 00024 #include "mbed-trace/mbed_trace.h" 00025 00026 #include <assert.h> 00027 00028 #define xstr(s) str(s) 00029 #define str(s) #s 00030 00031 #define TRACE_GROUP "mClt" 00032 00033 #ifdef MBED_CONF_MBED_CLIENT_EVENT_LOOP_SIZE 00034 #define MBED_CLIENT_EVENT_LOOP_SIZE MBED_CONF_MBED_CLIENT_EVENT_LOOP_SIZE 00035 #else 00036 #define MBED_CLIENT_EVENT_LOOP_SIZE 1024 00037 #endif 00038 00039 MbedCloudClient::MbedCloudClient() 00040 :_client(*this), 00041 _value_callback(NULL), 00042 _error_description(NULL) 00043 { 00044 ns_hal_init(NULL, MBED_CLIENT_EVENT_LOOP_SIZE, NULL, NULL); 00045 } 00046 00047 MbedCloudClient::~MbedCloudClient() 00048 { 00049 _object_list.clear(); 00050 } 00051 00052 void MbedCloudClient::add_objects(const M2MObjectList& object_list) 00053 { 00054 if(!object_list.empty()) { 00055 M2MObjectList::const_iterator it; 00056 it = object_list.begin(); 00057 for (; it!= object_list.end(); it++) { 00058 _object_list.push_back(*it); 00059 } 00060 } 00061 } 00062 00063 void MbedCloudClient::set_update_callback(MbedCloudClientCallback *callback) 00064 { 00065 _value_callback = callback; 00066 } 00067 00068 bool MbedCloudClient::setup(void* iface) 00069 { 00070 tr_debug("MbedCloudClient setup()"); 00071 // Add objects to list 00072 map<string, M2MObject*>::iterator it; 00073 for (it = _objects.begin(); it != _objects.end(); it++) 00074 { 00075 _object_list.push_back(it->second); 00076 } 00077 _client.connector_client().m2m_interface()->set_platform_network_handler(iface); 00078 00079 _client.initialize_and_register(_object_list); 00080 return true; 00081 } 00082 00083 void MbedCloudClient::on_registered(void(*fn)(void)) 00084 { 00085 FP0<void> fp(fn); 00086 _on_registered = fp; 00087 } 00088 00089 00090 void MbedCloudClient::on_error(void(*fn)(int)) 00091 { 00092 _on_error = fn; 00093 } 00094 00095 00096 void MbedCloudClient::on_unregistered(void(*fn)(void)) 00097 { 00098 FP0<void> fp(fn); 00099 _on_unregistered = fp; 00100 } 00101 00102 void MbedCloudClient::on_registration_updated(void(*fn)(void)) 00103 { 00104 FP0<void> fp(fn); 00105 _on_registration_updated = fp; 00106 } 00107 00108 void MbedCloudClient::keep_alive() 00109 { 00110 _client.connector_client().update_registration(); 00111 } 00112 00113 void MbedCloudClient::register_update() 00114 { 00115 _client.connector_client().update_registration(); 00116 } 00117 00118 void MbedCloudClient::close() 00119 { 00120 _client.connector_client().m2m_interface()->unregister_object(NULL); 00121 } 00122 00123 const ConnectorClientEndpointInfo *MbedCloudClient::endpoint_info() const 00124 { 00125 return _client.connector_client().endpoint_info(); 00126 } 00127 00128 void MbedCloudClient::set_queue_sleep_handler(callback_handler handler) 00129 { 00130 _client.connector_client().m2m_interface()->set_queue_sleep_handler(handler); 00131 } 00132 00133 void MbedCloudClient::set_random_number_callback(random_number_cb callback) 00134 { 00135 _client.connector_client().m2m_interface()->set_random_number_callback(callback); 00136 } 00137 00138 void MbedCloudClient::set_entropy_callback(entropy_cb callback) 00139 { 00140 _client.connector_client().m2m_interface()->set_entropy_callback(callback); 00141 } 00142 00143 bool MbedCloudClient::set_device_resource_value(M2MDevice::DeviceResource resource, 00144 const std::string &value) 00145 { 00146 return _client.set_device_resource_value(resource, value); 00147 } 00148 00149 #ifdef MBED_CLOUD_CLIENT_SUPPORT_UPDATE 00150 void MbedCloudClient::set_update_authorize_handler(void (*handler)(int32_t request)) 00151 { 00152 _client.set_update_authorize_handler(handler); 00153 } 00154 00155 void MbedCloudClient::set_update_progress_handler(void (*handler)(uint32_t progress, uint32_t total)) 00156 { 00157 _client.set_update_progress_handler(handler); 00158 } 00159 00160 void MbedCloudClient::update_authorize(int32_t request) 00161 { 00162 _client.update_authorize(request); 00163 } 00164 #endif 00165 00166 const char *MbedCloudClient::error_description() const 00167 { 00168 return _error_description; 00169 } 00170 00171 00172 void MbedCloudClient::register_update_callback(string route, 00173 SimpleM2MResourceBase* resource) 00174 { 00175 _update_values[route] = resource; 00176 } 00177 00178 void MbedCloudClient::complete(ServiceClientCallbackStatus status) 00179 { 00180 tr_info("MbedCloudClient::complete status (%d)", status); 00181 if (status == Service_Client_Status_Registered) { 00182 _on_registered.call(); 00183 } else if (status == Service_Client_Status_Unregistered) { 00184 _object_list.clear(); 00185 _on_unregistered.call(); 00186 } else if (status == Service_Client_Status_Register_Updated) { 00187 _on_registration_updated.call(); 00188 } 00189 } 00190 00191 void MbedCloudClient::error(int error, const char *reason) 00192 { 00193 tr_error("MbedCloudClient::error code (%d)", error); 00194 _error_description = reason; 00195 _on_error(error); 00196 } 00197 00198 void MbedCloudClient::value_updated(M2MBase *base, M2MBase::BaseType type) 00199 { 00200 if (base) { 00201 tr_info("MbedCloudClient::value_updated path %s", base->uri_path()); 00202 if (base->uri_path()) { 00203 if (_update_values.count(base->uri_path()) != 0) { 00204 tr_debug("MbedCloudClient::value_updated calling update() for %s", base->uri_path()); 00205 _update_values[base->uri_path()]->update(); 00206 } else { 00207 // way to tell application that there is a value update 00208 if (_value_callback) { 00209 _value_callback->value_updated(base, type); 00210 } 00211 } 00212 } 00213 } 00214 }
Generated on Tue Jul 12 2022 19:01:35 by
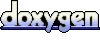