
Example program for simple-mbed-client, connecting a device to mbed Device Connector.
Dependencies: C12832 FXOS8700Q simple-mbed-client
Fork of simple-mbed-client-example by
main.cpp
00001 #include "mbed.h" 00002 #include "security.h" 00003 #include "easy-connect.h" 00004 #include "simple-mbed-client.h" 00005 #include "C12832.h" 00006 #include "FXOS8700Q.h" 00007 00008 Serial pc(USBTX, USBRX); 00009 C12832 lcd(D11, D13, D12, D7, D10); 00010 I2C i2c(PTE25, PTE24); 00011 FXOS8700QAccelerometer acc(i2c, FXOS8700CQ_SLAVE_ADDR1); 00012 FXOS8700QMagnetometer mag(i2c, FXOS8700CQ_SLAVE_ADDR1); 00013 00014 SimpleMbedClient client; 00015 00016 // Declare peripherals 00017 DigitalOut connectivityLed(LED2); // Blinks while connecting, turns solid when connected 00018 DigitalOut augmentedLed(LED1, BUILTIN_LED_OFF); // LED that can be controlled through Connector 00019 InterruptIn btn(MBED_CONF_APP_BUTTON); // Button that sends it's count to Connector 00020 00021 Ticker connectivityTicker; 00022 Ticker readAccelTicker; 00023 00024 // Callback function when the pattern resource is updated 00025 void patternUpdated(string v) 00026 { 00027 pc.printf("New pattern: %s\n", v.c_str()); 00028 } 00029 00030 void lcdTextUpdated(string v) 00031 { 00032 if (v.length() > 30) { 00033 v.erase(v.begin() + 30, v.end()); 00034 } 00035 pc.printf("New text is %s\r\n", v.c_str()); 00036 00037 lcd.cls(); 00038 lcd.locate(0, 3); 00039 lcd.printf(v.c_str()); 00040 } 00041 00042 // Define resources here. They act as normal variables, but are exposed to the internet... 00043 SimpleResourceInt btn_count = client.define_resource("button/0/clicks", 0, M2MBase::GET_ALLOWED); 00044 SimpleResourceString pattern = client.define_resource("led/0/pattern", "500:500:500:500:500:500:500", &patternUpdated); 00045 SimpleResourceString lcd_text = client.define_resource("lcd/0/text", 00046 "Hello from the cloud", M2MBase::GET_PUT_ALLOWED, true, &lcdTextUpdated); 00047 00048 SimpleResourceInt aX = client.define_resource("accel/0/x", 0, M2MBase::GET_ALLOWED); 00049 SimpleResourceInt aY = client.define_resource("accel/0/y", 0, M2MBase::GET_ALLOWED); 00050 SimpleResourceInt aZ = client.define_resource("accel/0/z", 0, M2MBase::GET_ALLOWED); 00051 00052 void readAccel() 00053 { 00054 motion_data_counts_t acc_data; 00055 00056 acc.getAxis(acc_data); 00057 aX = acc_data.x; 00058 aY = acc_data.y; 00059 aZ = acc_data.z; 00060 00061 pc.printf("ACC: X=%d Y=%d Z=%d\r\n", acc_data.x, acc_data.y, acc_data.z); 00062 } 00063 00064 void fall() 00065 { 00066 btn_count = btn_count + 1; 00067 pc.printf("Button count is now %d\r\n", static_cast<int>(btn_count)); 00068 } 00069 00070 void toggleConnectivityLed() 00071 { 00072 connectivityLed = !connectivityLed; 00073 } 00074 00075 void registered() 00076 { 00077 pc.printf("Registered\r\n"); 00078 00079 connectivityTicker.detach(); 00080 connectivityLed = BUILTIN_LED_ON; 00081 } 00082 00083 void play(void* args) 00084 { 00085 connectivityLed = BUILTIN_LED_OFF; 00086 00087 // Parse the pattern string, and toggle the LED in that pattern 00088 string s = static_cast<string>(pattern); 00089 size_t i = 0; 00090 size_t pos = s.find(':'); 00091 while (pos != string::npos) { 00092 wait_ms(atoi(s.substr(i, pos - i).c_str())); 00093 augmentedLed = !augmentedLed; 00094 00095 i = ++pos; 00096 pos = s.find(':', pos); 00097 00098 if (pos == string::npos) { 00099 wait_ms(atoi(s.substr(i, s.length()).c_str())); 00100 augmentedLed = !augmentedLed; 00101 } 00102 } 00103 00104 augmentedLed = BUILTIN_LED_OFF; 00105 connectivityLed = BUILTIN_LED_ON; 00106 } 00107 00108 int main() 00109 { 00110 pc.baud(115200); 00111 lcdTextUpdated(static_cast<string>(lcd_text).c_str()); 00112 acc.enable(); 00113 00114 // SimpleClient gives us an event queue which we can use, running on a separate thread (see https://docs.mbed.com/docs/mbed-os-handbook/en/5.1/concepts/events/) 00115 EventQueue* eventQueue = client.eventQueue(); 00116 00117 // Handle interrupts on the eventQueue - so we don't do things in interrupt context 00118 btn.fall(eventQueue->event(&fall)); 00119 00120 // Toggle the built-in LED every second (until we're connected, see `registered` function) 00121 connectivityTicker.attach(eventQueue->event(&toggleConnectivityLed), 1.0f); 00122 00123 readAccelTicker.attach(eventQueue->event(&readAccel), 1.0f); 00124 00125 // Functions can be executed through mbed Device Connector via POST calls (also defer to the event thread, so we never block) 00126 client.define_function("led/0/play", eventQueue->event(&play)); 00127 00128 // Connect to the internet (see mbed_app.json for the connectivity method) 00129 NetworkInterface* network = easy_connect(true); 00130 if (!network) { 00131 return 1; 00132 } 00133 00134 // Connect to mbed Device Connector 00135 struct MbedClientOptions opts = client.get_default_options(); // opts contains information like the DeviceType 00136 bool setup = client.setup(opts, network); 00137 if (!setup) { 00138 pc.printf("Client setup failed\n"); 00139 return 1; 00140 } 00141 client.on_registered(®istered); 00142 }
Generated on Tue Jul 12 2022 20:58:55 by
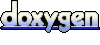