
Piezo Vibration Sensor demo
Dependencies: PCA9622_LED8x8 mbed
main.cpp
00001 /** 00002 * MFT2015 demo : shake-shake-machine 00003 * 00004 * @author Toyomasa WATARAI 00005 * @version 1.0 00006 * @date 24-July-2015 00007 * 00008 * 00009 */ 00010 00011 #include "mbed.h" 00012 #include "PCA9622_LED8x8.h" 00013 #include "font3x5.h" 00014 #include "tone_table.h" 00015 00016 PCA9622_LED8x8 matrix(D14, D15); 00017 AnalogIn ain(A0); 00018 PwmOut out(D4); 00019 Serial pc(USBTX, USBRX); 00020 00021 #define DURATION_TIME (5 * 1000) // n * milisecond 00022 00023 void draw_number(int num, int reverse=0); 00024 00025 void draw_number(int num, int reverse) 00026 { 00027 float image[8][8]; 00028 num %= 100; 00029 00030 for (int x=0; x<8; x++) 00031 for (int y=0; y<8; y++) { 00032 if (reverse) 00033 image[x][y] = 0.5f; 00034 else 00035 image[x][y] = 0.0f; 00036 } 00037 00038 for (int i=0; i<5; i++) { 00039 for (int j=0; j<3; j++) { 00040 if (((font3x5[((num/10)*3) + j]) & (1<<(4-i))) != 0) { 00041 if (reverse) 00042 image[i+1][j+1] = (float)0.0f; 00043 else 00044 image[i+1][j+1] = (float)0.5f; 00045 } else { 00046 if (reverse) 00047 image[i+1][j+1] = (float)0.5f; 00048 else 00049 image[i+1][j+1] = (float)0.0f; 00050 } 00051 } 00052 } 00053 00054 for (int i=0; i<5; i++) { 00055 for (int j=0; j<3; j++) { 00056 if (((font3x5[((num%10)*3) + j]) & (1<<(4-i))) != 0) { 00057 if (reverse) 00058 image[i+1][j+5] = (float)0.0f; 00059 else 00060 image[i+1][j+5] = (float)0.5f; 00061 } else { 00062 if (reverse) 00063 image[i+1][j+5] = (float)0.5f; 00064 else 00065 image[i+1][j+5] = (float)0.0f; 00066 } 00067 } 00068 } 00069 00070 matrix.set_data( image ); 00071 } 00072 00073 00074 void count_down(int cnt) 00075 { 00076 for(int i=cnt; i>0; i--) { 00077 draw_number(i); 00078 out.period_us(tone_table_us[1]); 00079 out.write(0.5f); 00080 wait(0.5); 00081 out.period(0); 00082 wait(0.5); 00083 } 00084 draw_number(0); 00085 out.period_us(tone_table_us[13]); 00086 out.write(0.5f); 00087 } 00088 00089 int main() 00090 { 00091 int count = 0; 00092 float f = 0; 00093 float vv; 00094 Timer timer; 00095 00096 timer.reset(); 00097 matrix.start(); 00098 00099 count_down(3); 00100 00101 timer.start(); 00102 while(1) { 00103 if ( timer.read_ms() > 1000) { 00104 out.period(0); 00105 } 00106 vv = ain.read(); 00107 00108 if ( abs(f - vv) >= 0.015f) { 00109 count++; 00110 pc.printf("*"); 00111 draw_number(count); 00112 } 00113 f = vv; 00114 wait(0.1); 00115 if ( timer.read_ms() > DURATION_TIME) { 00116 pc.printf("count = %d\n", count); 00117 draw_number(count); 00118 break; 00119 } 00120 } 00121 00122 out.period_us(tone_table_us[13]); 00123 out.write(0.5f); 00124 wait(1); 00125 out.period(0); 00126 00127 while(1) { 00128 draw_number(count, 0); 00129 wait(1); 00130 draw_number(count, 1); 00131 wait(1); 00132 00133 } 00134 }
Generated on Wed Jul 20 2022 06:23:47 by
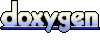