
Simple Mbed Cloud client application using features of K64 including Wi-Fi and SD Card
Fork of mbed-cloud-connect-example-ethernet by
main.cpp
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // SPDX-License-Identifier: Apache-2.0 00005 // 00006 // Licensed under the Apache License, Version 2.0 (the "License"); 00007 // you may not use this file except in compliance with the License. 00008 // You may obtain a copy of the License at 00009 // 00010 // http://www.apache.org/licenses/LICENSE-2.0 00011 // 00012 // Unless required by applicable law or agreed to in writing, software 00013 // distributed under the License is distributed on an "AS IS" BASIS, 00014 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 // See the License for the specific language governing permissions and 00016 // limitations under the License. 00017 // ---------------------------------------------------------------------------- 00018 00019 #include "mbed.h" 00020 #include "mbed-trace/mbed_trace.h" 00021 #include "mbed-trace-helper.h" 00022 #include "simple-mbed-cloud-client.h" 00023 #include "key-config-manager/kcm_status.h" 00024 #include "key-config-manager/key_config_manager.h" 00025 #include "SDBlockDevice.h" 00026 #include "FATFileSystem.h" 00027 #include "ESP8266Interface.h" 00028 00029 #define WIFI_SSID "SSID" 00030 #define WIFI_PSWD "PASSWORD" 00031 00032 /* The following app uses Mbed Cloud with SD Card storage, button, & led */ 00033 00034 // Placeholder to hardware that trigger events (timer, button, etc) 00035 //Ticker timer; 00036 /* K64 & K66 */ 00037 InterruptIn sw2(SW2); 00038 DigitalOut led2(LED2); 00039 ESP8266Interface net(D1, D0); 00040 00041 /* */ 00042 00043 // Placeholder for storage 00044 /* K64 & K66 */ 00045 SDBlockDevice sd(PTE3, PTE1, PTE2, PTE4); 00046 FATFileSystem fs("sd"); 00047 /* */ 00048 00049 // Pointers to the resources that will be created in main_application(). 00050 static MbedCloudClientResource* pattern_ptr; 00051 static MbedCloudClientResource* button_ptr; 00052 00053 // Pointer to mbedClient, used for calling close function. 00054 static SimpleMbedCloudClient *client; 00055 00056 static bool button_pressed = false; 00057 static int button_count = 0; 00058 00059 void button_press() { 00060 button_pressed = true; 00061 ++button_count; 00062 button_ptr->set_value(button_count); 00063 } 00064 00065 void pattern_updated(const char *) { 00066 printf("PUT received, new value: %s\n", pattern_ptr->get_value().c_str()); 00067 // Placeholder for PUT action 00068 } 00069 00070 void blink_callback(void *) { 00071 String pattern_str = pattern_ptr->get_value(); 00072 const char *pattern = pattern_str.c_str(); 00073 printf("POST received. LED pattern = %s\n", pattern); 00074 // Placeholder for POST action 00075 // The pattern is something like 500:200:500, so parse that. 00076 // LED blinking is done while parsing. 00077 00078 while (*pattern != '\0') { 00079 //make a short blink on the led 00080 led2 = 0; 00081 wait_ms(20); 00082 led2 = 1; 00083 // Wait for requested time. 00084 wait_ms(atoi(pattern)); 00085 // Search for next value. 00086 pattern = strchr(pattern, ':'); 00087 if(!pattern) { 00088 //we're done, give one last blink to end the pattern 00089 led2 = 0; 00090 wait_ms(20); 00091 led2 = 1; 00092 break; // while 00093 } 00094 pattern++; 00095 } 00096 } 00097 00098 void button_callback(const M2MBase& object, const NoticationDeliveryStatus status) 00099 { 00100 printf("Button notification. Callback: (%s)\n", object.uri_path()); 00101 // Placeholder for GET 00102 } 00103 00104 00105 int main(void) 00106 { 00107 // Requires DAPLink 245+ (https://github.com/ARMmbed/DAPLink/pull/364) 00108 // Older versions: workaround to prevent possible deletion of credentials: 00109 wait(2); 00110 00111 // Misc OS setup 00112 srand(time(NULL)); 00113 00114 printf("Start Simple Mbed Cloud Client\n"); 00115 00116 // Initialize SD card 00117 int status = sd.init(); 00118 if (status != BD_ERROR_OK) { 00119 printf("Failed to init SD card\r\n"); 00120 return -1; 00121 } 00122 00123 // Mount the file system (reformatting on failure) 00124 status = fs.mount(&sd); 00125 if (status) { 00126 printf("Failed to mount FAT file system, reformatting...\r\n"); 00127 status = fs.reformat(&sd); 00128 if (status) { 00129 printf("Failed to reformat FAT file system\r\n"); 00130 return -1; 00131 } else { 00132 printf("Reformat and mount complete\r\n"); 00133 } 00134 } 00135 00136 printf("Connecting to the network using Wi-Fi...\n"); 00137 00138 status = net.connect(WIFI_SSID, WIFI_PSWD, NSAPI_SECURITY_WPA_WPA2); 00139 00140 if (status) { 00141 printf("Connection to Network Failed %d!\n", status); 00142 return -1; 00143 } else { 00144 const char *ip_addr = net.get_ip_address(); 00145 printf("Connected successfully\n"); 00146 printf("IP address %s\n", ip_addr); 00147 } 00148 00149 SimpleMbedCloudClient mbedClient(&net); 00150 // Save pointer to mbedClient so that other functions can access it. 00151 client = &mbedClient; 00152 00153 status = mbedClient.init(); 00154 if (status) { 00155 return -1; 00156 } 00157 00158 printf("Client initialized\r\n"); 00159 00160 // Mbed Cloud Client resource setup 00161 MbedCloudClientResource *button = mbedClient.create_resource("3200/0/5501", "button_resource"); 00162 button->set_value("0"); 00163 button->methods(M2MMethod::GET); 00164 button->observable(true); 00165 button->attach_notification_callback(button_callback); 00166 button_ptr = button; 00167 00168 MbedCloudClientResource *pattern = mbedClient.create_resource("3201/0/5853", "pattern_resource"); 00169 pattern->set_value("500:500:500:500"); 00170 pattern->methods(M2MMethod::GET | M2MMethod::PUT); 00171 pattern->attach_put_callback(pattern_updated); 00172 pattern_ptr = pattern; 00173 00174 MbedCloudClientResource *blink = mbedClient.create_resource("3201/0/5850", "blink_resource"); 00175 blink->methods(M2MMethod::POST); 00176 blink->attach_post_callback(blink_callback); 00177 00178 mbedClient.register_and_connect(); 00179 00180 // Wait for client to finish registering 00181 while (!mbedClient.is_client_registered()) { 00182 wait_ms(100); 00183 } 00184 00185 // Placeholder for callback to update local resource when GET comes. 00186 //timer.attach(&button_press, 5.0); 00187 sw2.mode(PullUp); 00188 sw2.fall(button_press); 00189 button_count = 0; 00190 00191 // Check if client is registering or registered, if true sleep and repeat. 00192 while (mbedClient.is_register_called()) { 00193 //static int button_count = 0; 00194 00195 wait_ms(100); 00196 00197 if (button_pressed) { 00198 button_pressed = false; 00199 //printf("button clicked %d times\r\n", ++button_count); 00200 //button->set_value(button_count); 00201 printf("button clicked %d times\r\n", button_count); 00202 } 00203 00204 } 00205 00206 // Client unregistered, exit program. 00207 return 0; 00208 }
Generated on Tue Jul 26 2022 16:57:07 by
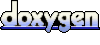