
Example program for LPC General Purpose Shield using the 5-way JoyStick
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "PCAL9555.h" 00003 00004 PCAL9555 gpio_exp(SDA, SCL); 00005 GpioBusIn joystick(gpio_exp, X0_0, X0_1, X0_2, X0_3, X0_4); 00006 Serial pc(USBTX, USBRX); 00007 00008 enum key_num { 00009 Key_Up = (1 << X0_4), 00010 Key_Down = (1 << X0_0), 00011 Key_Right = (1 << X0_3), 00012 Key_Left = (1 << X0_2), 00013 Key_Center = (1 << X0_1), 00014 }; 00015 00016 int main() 00017 { 00018 while(1) { 00019 int keys = joystick.read(); 00020 if ((keys & Key_Up) == 0) 00021 pc.printf("Up\n"); 00022 if ((keys & Key_Down) == 0) 00023 pc.printf("Down\n"); 00024 if ((keys & Key_Right) == 0) 00025 pc.printf("Right\n"); 00026 if ((keys & Key_Left) == 0) 00027 pc.printf("Left\n"); 00028 if ((keys & Key_Center) == 0) 00029 pc.printf("Center\n"); 00030 wait(0.3); 00031 } 00032 }
Generated on Tue Jul 12 2022 18:37:48 by
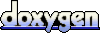