
Mbed Cloud example program for workshop in W27 2018.
Embed:
(wiki syntax)
Show/hide line numbers
fcc_bundle_common_utils.c
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // Licensed under the Apache License, Version 2.0 (the "License"); 00005 // you may not use this file except in compliance with the License. 00006 // You may obtain a copy of the License at 00007 // 00008 // http://www.apache.org/licenses/LICENSE-2.0 00009 // 00010 // Unless required by applicable law or agreed to in writing, software 00011 // distributed under the License is distributed on an "AS IS" BASIS, 00012 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 // See the License for the specific language governing permissions and 00014 // limitations under the License. 00015 // ---------------------------------------------------------------------------- 00016 00017 #include "fcc_bundle_handler.h" 00018 #include "cn-cbor.h" 00019 #include "pv_error_handling.h" 00020 #include "fcc_bundle_utils.h" 00021 #include "fcc_malloc.h" 00022 #include "general_utils.h" 00023 00024 #define FCC_MAX_SIZE_OF_STRING 512 00025 00026 /** Gets name from cbor struct. 00027 * 00028 * @param text_cb[in] The cbor text structure 00029 * @param name_out[out] The out buffer for string data 00030 * @param name_len_out[out] The actual size of output buffer 00031 * 00032 * @return 00033 * true for success, false otherwise. 00034 */ 00035 static bool get_data_name(const cn_cbor *text_cb, uint8_t **name_out, size_t *name_len_out) 00036 { 00037 SA_PV_LOG_TRACE_FUNC_ENTER_NO_ARGS(); 00038 SA_PV_ERR_RECOVERABLE_RETURN_IF((text_cb == NULL), false, "Cbor pointer is NULL"); 00039 SA_PV_ERR_RECOVERABLE_RETURN_IF((name_out == NULL), false, "Invalid pointer for name"); 00040 SA_PV_ERR_RECOVERABLE_RETURN_IF((name_len_out == NULL), false, "Invalid pointer for name_len"); 00041 00042 *name_out = (uint8_t*)fcc_malloc((size_t)(text_cb->length)); 00043 SA_PV_ERR_RECOVERABLE_RETURN_IF((*name_out == NULL), false, "Failed to allocate buffer for name"); 00044 00045 memcpy(*name_out, text_cb->v.bytes, (size_t)text_cb->length); 00046 *name_len_out = (size_t)text_cb->length; 00047 SA_PV_LOG_TRACE_FUNC_EXIT_NO_ARGS(); 00048 00049 return true; 00050 } 00051 00052 /** Gets data format from cbor struct 00053 * 00054 * The function goes over all formats and compares it with format from cbor structure. 00055 * 00056 * @param data_cb[in] The cbor text structure 00057 * @param data_format[out] The format of data 00058 * 00059 * @return 00060 * true for success, false otherwise. 00061 */ 00062 static bool get_data_format(const cn_cbor *data_cb, fcc_bundle_data_format_e *data_format) 00063 { 00064 00065 int data_format_index; 00066 bool res; 00067 00068 SA_PV_LOG_TRACE_FUNC_ENTER_NO_ARGS(); 00069 SA_PV_ERR_RECOVERABLE_RETURN_IF((data_cb == NULL), false, "data_cb is null"); 00070 SA_PV_ERR_RECOVERABLE_RETURN_IF((data_format == NULL), false, "data_format is null"); 00071 SA_PV_ERR_RECOVERABLE_RETURN_IF((*data_format != FCC_INVALID_DATA_FORMAT), false, "wrong data format value"); 00072 00073 for (data_format_index = 0; data_format_index < FCC_MAX_DATA_FORMAT -1; data_format_index++) { 00074 res = is_memory_equal(fcc_bundle_data_format_lookup_table[data_format_index].data_format_name, 00075 strlen(fcc_bundle_data_format_lookup_table[data_format_index].data_format_name), 00076 data_cb->v.bytes, 00077 data_cb->length); 00078 if (res) { 00079 *data_format = fcc_bundle_data_format_lookup_table[data_format_index].data_format_type; 00080 return true; 00081 } 00082 } 00083 SA_PV_LOG_TRACE_FUNC_EXIT_NO_ARGS(); 00084 return false; 00085 } 00086 00087 bool get_data_buffer_from_cbor(const cn_cbor *data_cb, uint8_t **out_data_buffer, size_t *out_size) 00088 { 00089 00090 cn_cbor_type cb_type; 00091 00092 SA_PV_LOG_TRACE_FUNC_ENTER_NO_ARGS(); 00093 SA_PV_ERR_RECOVERABLE_RETURN_IF((data_cb == NULL), false, "key_data_cb is null"); 00094 SA_PV_ERR_RECOVERABLE_RETURN_IF((out_size == NULL), false, "Size buffer is null "); 00095 SA_PV_ERR_RECOVERABLE_RETURN_IF((out_data_buffer == NULL), false, "Data buffer is null"); 00096 cb_type = data_cb->type; 00097 00098 switch (cb_type) { 00099 case CN_CBOR_TAG: 00100 *out_data_buffer = (uint8_t*)data_cb->first_child->v.bytes; 00101 *out_size = (size_t)data_cb->first_child->length; 00102 break; 00103 case CN_CBOR_TEXT: 00104 case CN_CBOR_BYTES: 00105 *out_data_buffer = (uint8_t*)data_cb->v.bytes; 00106 *out_size = data_cb->length; 00107 break; 00108 case CN_CBOR_UINT: 00109 *out_data_buffer = (uint8_t*)(&(data_cb->v.uint)); 00110 *out_size = data_cb->length; 00111 break; 00112 case CN_CBOR_INT: 00113 *out_data_buffer = (uint8_t*)(&(data_cb->v.sint)); 00114 *out_size = data_cb->length; 00115 break; 00116 default: 00117 SA_PV_LOG_ERR("Invalid cbor data type (%u)!", data_cb->type); 00118 return false; 00119 } 00120 SA_PV_LOG_TRACE_FUNC_EXIT("out_size=%" PRIu32 "", (uint32_t)*out_size); 00121 return true; 00122 } 00123 /** Frees all allocated memory of data parameter struct and sets initial values. 00124 * 00125 * @param data_param[in/out] The data parameter structure 00126 */ 00127 void fcc_bundle_clean_and_free_data_param(fcc_bundle_data_param_s *data_param) 00128 { 00129 SA_PV_LOG_TRACE_FUNC_ENTER_NO_ARGS(); 00130 00131 if (data_param->name != NULL) { 00132 fcc_free(data_param->name); 00133 data_param->name = NULL; 00134 } 00135 00136 data_param->array_cn = NULL; 00137 00138 //FIXME - in case we will support pem, add additional pointer data_der, that will point to allocated 00139 // memory and will always released in case not NULL nad data pointer will relate to user buffer allways. 00140 /*if (data_param->data_der != NULL) { 00141 fcc_stats_free(data_param->data_der); 00142 data_param->data_der = NULL; 00143 }*/ 00144 00145 memset(data_param, 0, sizeof(fcc_bundle_data_param_s)); 00146 SA_PV_LOG_TRACE_FUNC_EXIT_NO_ARGS(); 00147 00148 } 00149 bool fcc_bundle_get_data_param(const cn_cbor *data_param_cb, fcc_bundle_data_param_s *data_param) 00150 { 00151 bool status = false; 00152 int data_param_index = 0; 00153 cn_cbor *data_param_value_cb; 00154 fcc_bundle_data_param_type_e data_param_type; 00155 00156 SA_PV_LOG_TRACE_FUNC_ENTER_NO_ARGS(); 00157 00158 //Prepare key struct 00159 fcc_bundle_clean_and_free_data_param(data_param); 00160 00161 //Go over all key's parameters and extract it to appropriate key struct member 00162 for (data_param_index = FCC_BUNDLE_DATA_PARAM_NAME_TYPE; data_param_index < FCC_BUNDLE_DATA_PARAM_MAX_TYPE; data_param_index++) { 00163 00164 //Get value of parameter 00165 data_param_value_cb = cn_cbor_mapget_string(data_param_cb, fcc_bundle_data_param_lookup_table[data_param_index].data_param_name); 00166 00167 if (data_param_value_cb != NULL) { 00168 //Get type of parameter 00169 data_param_type = fcc_bundle_data_param_lookup_table[data_param_index].data_param_type; 00170 00171 switch (data_param_type) { 00172 00173 case FCC_BUNDLE_DATA_PARAM_NAME_TYPE: 00174 status = get_data_name(data_param_value_cb, &(data_param->name), &(data_param->name_len)); 00175 SA_PV_ERR_RECOVERABLE_GOTO_IF((status != true), status = false, error_exit, "Failed to get data parameter name"); 00176 break; 00177 00178 case FCC_BUNDLE_DATA_PARAM_SCHEME_TYPE: 00179 status = fcc_bundle_get_key_type(data_param_value_cb, (fcc_bundle_key_type_e*)&(data_param->type)); 00180 SA_PV_ERR_RECOVERABLE_GOTO_IF((status != true), status = false, error_exit, "Failed to get parameter type"); 00181 break; 00182 00183 case FCC_BUNDLE_DATA_PARAM_FORMAT_TYPE: 00184 status = get_data_format(data_param_value_cb, (fcc_bundle_data_format_e*)&(data_param->format)); 00185 SA_PV_ERR_RECOVERABLE_GOTO_IF((status != true), status = false, error_exit, "Failed to get key format"); 00186 break; 00187 00188 case FCC_BUNDLE_DATA_PARAM_DATA_TYPE: 00189 status = get_data_buffer_from_cbor(data_param_value_cb, &(data_param->data), &(data_param->data_size)); 00190 data_param->data_type = FCC_EXTERNAL_BUFFER_TYPE; 00191 SA_PV_ERR_RECOVERABLE_GOTO_IF((status != true), status = false, error_exit, "Failed to get parameter data"); 00192 break; 00193 case FCC_BUNDLE_DATA_PARAM_ARRAY_TYPE: 00194 data_param->array_cn = data_param_value_cb; 00195 break; 00196 case FCC_BUNDLE_DATA_PARAM_ACL_TYPE: 00197 status = get_data_buffer_from_cbor(data_param_value_cb, &(data_param->acl), &data_param->acl_size); 00198 SA_PV_ERR_RECOVERABLE_GOTO_IF((status != true), status = false, error_exit, "Failed to get acl data"); 00199 break; 00200 default: 00201 SA_PV_ERR_RECOVERABLE_GOTO_IF((true), status = false, error_exit, "Parameter's field name is illegal"); 00202 }//switch 00203 }//if 00204 }//for 00205 00206 //FIXME: should be uncommented if PEM format is supported. 00207 /* 00208 if (data_param->format == FCC_PEM_DATA_FORMAT) { 00209 //status = convert_certificate_from_pem_to_der((uint8_t**)&(data_param->data), &(data_param->data_size)); 00210 SA_PV_ERR_RECOVERABLE_GOTO_IF((status != true), status = false, error_exit, "Failed to convert the key from pem to der"); 00211 //key->data_type = FCC_INTERNAL_BUFFER_TYPE; 00212 } 00213 */ 00214 00215 SA_PV_LOG_TRACE_FUNC_EXIT_NO_ARGS(); 00216 return status; 00217 00218 error_exit: 00219 fcc_bundle_clean_and_free_data_param(data_param); 00220 SA_PV_LOG_TRACE_FUNC_EXIT_NO_ARGS(); 00221 return false; 00222 }
Generated on Tue Jul 12 2022 16:22:05 by
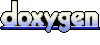