
VT52 is an enhancement of class Serial and offers some Methods for Printing in VT52 Terminal Style: clearScreen() - to clear the whole Terminal Screen clearLine() - to clear the current Line setCursor(lin, col) - to set cursor in column col of line lin
Test_VT52.cpp
00001 /******************************************************************** 00002 * Project: Test_VT52 00003 * Program: Test_VT52.cpp 00004 * Description: Print to PC-Terminal and PC-Log 00005 * 2 Terminal Emulations (VT52) are needed at PC 00006 * Serial Connection (p13, p14) is connected 00007 * to RS232-USB Adapter 00008 * 00009 * Author: Rainer Krugmann 00010 * Date: 2011.01.23 00011 ********************************************************************/ 00012 00013 /* 00014 00015 Copyright (c) 2011 Rainer Krugmann 00016 (rainer (dot) krugmann [at] gmail [dot] com) 00017 00018 Permission is hereby granted, free of charge, to any person obtaining a copy 00019 of this software and associated documentation files (the "Software"), to deal 00020 in the Software without restriction, including without limitation the rights 00021 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00022 copies of the Software, and to permit persons to whom the Software is 00023 furnished to do so, subject to the following conditions: 00024 00025 The above copyright notice and this permission notice shall be included in 00026 all copies or substantial portions of the Software. 00027 00028 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00029 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00030 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00031 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00032 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00033 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00034 THE SOFTWARE. 00035 00036 */ 00037 00038 #include "mbed.h" 00039 #include "VT52.h" 00040 00041 VT52 pc(USBTX, USBRX); // PC-Terminal 00042 VT52 myLog(p13, p14); // PC-Terminal (Log) 00043 00044 int main() { 00045 00046 unsigned char c = 0; 00047 00048 myLog.baud(9600); 00049 myLog.clearScreen(); 00050 myLog.printf("Hello World - Log\r\n"); 00051 myLog.printf("=================\r\n\n"); 00052 00053 pc.clearScreen(); 00054 pc.printf("Hello World!\r\n"); 00055 pc.printf("============\r\n\n"); 00056 00057 pc.setCursor(12,3); 00058 pc.printf("Press any Characters ('x' to Exit): "); 00059 myLog.printf("Press any Characters ('x' to Exit)!\r\n"); 00060 00061 while (c != 'x') 00062 { 00063 c = pc.getc(); 00064 myLog.printf("You pressed: %c\r\n", c); 00065 pc.putc(c); 00066 } 00067 pc.setCursor(12, 9); 00068 pc.clearLine(); 00069 myLog.printf("\nLine cleared after 'Press'\r\n"); 00070 pc.setCursor(12, 9); 00071 00072 pc.printf("nothing!"); 00073 myLog.printf("Word 'nothing!' was printed\r\n"); 00074 00075 pc.setCursor(23, 75); 00076 pc.printf("End"); 00077 myLog.printf("\nWord 'End' was printed screen bottom\r\n"); 00078 myLog.printf("Cursor position is at screen end (line=23 and column = 79)\r\n"); 00079 00080 myLog.printf("Program ends\r\n"); 00081 while(1); 00082 } 00083 //*******************************************************************
Generated on Thu Jul 14 2022 14:56:47 by
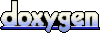