forked
Embed:
(wiki syntax)
Show/hide line numbers
core_cm33.h
Go to the documentation of this file.
00001 /**************************************************************************//** 00002 * @file core_cm33.h 00003 * @brief CMSIS Cortex-M33 Core Peripheral Access Layer Header File 00004 * @version V5.0.2 00005 * @date 13. February 2017 00006 ******************************************************************************/ 00007 /* 00008 * Copyright (c) 2009-2017 ARM Limited. All rights reserved. 00009 * 00010 * SPDX-License-Identifier: Apache-2.0 00011 * 00012 * Licensed under the Apache License, Version 2.0 (the License); you may 00013 * not use this file except in compliance with the License. 00014 * You may obtain a copy of the License at 00015 * 00016 * www.apache.org/licenses/LICENSE-2.0 00017 * 00018 * Unless required by applicable law or agreed to in writing, software 00019 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00020 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00021 * See the License for the specific language governing permissions and 00022 * limitations under the License. 00023 */ 00024 00025 #if defined ( __ICCARM__ ) 00026 #pragma system_include /* treat file as system include file for MISRA check */ 00027 #elif defined (__ARMCC_VERSION) && (__ARMCC_VERSION >= 6010050) 00028 #pragma clang system_header /* treat file as system include file */ 00029 #endif 00030 00031 #ifndef __CORE_CM33_H_GENERIC 00032 #define __CORE_CM33_H_GENERIC 00033 00034 #include <stdint.h> 00035 00036 #ifdef __cplusplus 00037 extern "C" { 00038 #endif 00039 00040 /** 00041 \page CMSIS_MISRA_Exceptions MISRA-C:2004 Compliance Exceptions 00042 CMSIS violates the following MISRA-C:2004 rules: 00043 00044 \li Required Rule 8.5, object/function definition in header file.<br> 00045 Function definitions in header files are used to allow 'inlining'. 00046 00047 \li Required Rule 18.4, declaration of union type or object of union type: '{...}'.<br> 00048 Unions are used for effective representation of core registers. 00049 00050 \li Advisory Rule 19.7, Function-like macro defined.<br> 00051 Function-like macros are used to allow more efficient code. 00052 */ 00053 00054 00055 /******************************************************************************* 00056 * CMSIS definitions 00057 ******************************************************************************/ 00058 /** 00059 \ingroup Cortex_M33 00060 @{ 00061 */ 00062 00063 /* CMSIS CM33 definitions */ 00064 #define __CM33_CMSIS_VERSION_MAIN ( 5U) /*!< [31:16] CMSIS HAL main version */ 00065 #define __CM33_CMSIS_VERSION_SUB ( 0U) /*!< [15:0] CMSIS HAL sub version */ 00066 #define __CM33_CMSIS_VERSION ((__CM33_CMSIS_VERSION_MAIN << 16U) | \ 00067 __CM33_CMSIS_VERSION_SUB ) /*!< CMSIS HAL version number */ 00068 00069 #define __CORTEX_M (33U) /*!< Cortex-M Core */ 00070 00071 /** __FPU_USED indicates whether an FPU is used or not. 00072 For this, __FPU_PRESENT has to be checked prior to making use of FPU specific registers and functions. 00073 */ 00074 #if defined ( __CC_ARM ) 00075 #if defined __TARGET_FPU_VFP 00076 #if defined (__FPU_PRESENT) && (__FPU_PRESENT == 1U) 00077 #define __FPU_USED 1U 00078 #else 00079 #error "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00080 #define __FPU_USED 0U 00081 #endif 00082 #else 00083 #define __FPU_USED 0U 00084 #endif 00085 00086 #elif defined (__ARMCC_VERSION) && (__ARMCC_VERSION >= 6010050) 00087 #if defined __ARM_PCS_VFP 00088 #if defined (__FPU_PRESENT) && (__FPU_PRESENT == 1U) 00089 #define __FPU_USED 1U 00090 #else 00091 #warning "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00092 #define __FPU_USED 0U 00093 #endif 00094 #else 00095 #define __FPU_USED 0U 00096 #endif 00097 00098 #elif defined ( __GNUC__ ) 00099 #if defined (__VFP_FP__) && !defined(__SOFTFP__) 00100 #if defined (__FPU_PRESENT) && (__FPU_PRESENT == 1U) 00101 #define __FPU_USED 1U 00102 #else 00103 #error "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00104 #define __FPU_USED 0U 00105 #endif 00106 #else 00107 #define __FPU_USED 0U 00108 #endif 00109 00110 #elif defined ( __ICCARM__ ) 00111 #if defined __ARMVFP__ 00112 #if defined (__FPU_PRESENT) && (__FPU_PRESENT == 1U) 00113 #define __FPU_USED 1U 00114 #else 00115 #error "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00116 #define __FPU_USED 0U 00117 #endif 00118 #else 00119 #define __FPU_USED 0U 00120 #endif 00121 00122 #elif defined ( __TI_ARM__ ) 00123 #if defined __TI_VFP_SUPPORT__ 00124 #if defined (__FPU_PRESENT) && (__FPU_PRESENT == 1U) 00125 #define __FPU_USED 1U 00126 #else 00127 #error "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00128 #define __FPU_USED 0U 00129 #endif 00130 #else 00131 #define __FPU_USED 0U 00132 #endif 00133 00134 #elif defined ( __TASKING__ ) 00135 #if defined __FPU_VFP__ 00136 #if defined (__FPU_PRESENT) && (__FPU_PRESENT == 1U) 00137 #define __FPU_USED 1U 00138 #else 00139 #error "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00140 #define __FPU_USED 0U 00141 #endif 00142 #else 00143 #define __FPU_USED 0U 00144 #endif 00145 00146 #elif defined ( __CSMC__ ) 00147 #if ( __CSMC__ & 0x400U) 00148 #if defined (__FPU_PRESENT) && (__FPU_PRESENT == 1U) 00149 #define __FPU_USED 1U 00150 #else 00151 #error "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00152 #define __FPU_USED 0U 00153 #endif 00154 #else 00155 #define __FPU_USED 0U 00156 #endif 00157 00158 #endif 00159 00160 #include "cmsis_compiler.h" /* CMSIS compiler specific defines */ 00161 00162 00163 #ifdef __cplusplus 00164 } 00165 #endif 00166 00167 #endif /* __CORE_CM33_H_GENERIC */ 00168 00169 #ifndef __CMSIS_GENERIC 00170 00171 #ifndef __CORE_CM33_H_DEPENDANT 00172 #define __CORE_CM33_H_DEPENDANT 00173 00174 #ifdef __cplusplus 00175 extern "C" { 00176 #endif 00177 00178 /* check device defines and use defaults */ 00179 #if defined __CHECK_DEVICE_DEFINES 00180 #ifndef __CM33_REV 00181 #define __CM33_REV 0x0000U 00182 #warning "__CM33_REV not defined in device header file; using default!" 00183 #endif 00184 00185 #ifndef __FPU_PRESENT 00186 #define __FPU_PRESENT 0U 00187 #warning "__FPU_PRESENT not defined in device header file; using default!" 00188 #endif 00189 00190 #ifndef __MPU_PRESENT 00191 #define __MPU_PRESENT 0U 00192 #warning "__MPU_PRESENT not defined in device header file; using default!" 00193 #endif 00194 00195 #ifndef __SAUREGION_PRESENT 00196 #define __SAUREGION_PRESENT 0U 00197 #warning "__SAUREGION_PRESENT not defined in device header file; using default!" 00198 #endif 00199 00200 #ifndef __DSP_PRESENT 00201 #define __DSP_PRESENT 0U 00202 #warning "__DSP_PRESENT not defined in device header file; using default!" 00203 #endif 00204 00205 #ifndef __NVIC_PRIO_BITS 00206 #define __NVIC_PRIO_BITS 3U 00207 #warning "__NVIC_PRIO_BITS not defined in device header file; using default!" 00208 #endif 00209 00210 #ifndef __Vendor_SysTickConfig 00211 #define __Vendor_SysTickConfig 0U 00212 #warning "__Vendor_SysTickConfig not defined in device header file; using default!" 00213 #endif 00214 #endif 00215 00216 /* IO definitions (access restrictions to peripheral registers) */ 00217 /** 00218 \defgroup CMSIS_glob_defs CMSIS Global Defines 00219 00220 <strong>IO Type Qualifiers</strong> are used 00221 \li to specify the access to peripheral variables. 00222 \li for automatic generation of peripheral register debug information. 00223 */ 00224 #ifdef __cplusplus 00225 #define __I volatile /*!< Defines 'read only' permissions */ 00226 #else 00227 #define __I volatile const /*!< Defines 'read only' permissions */ 00228 #endif 00229 #define __O volatile /*!< Defines 'write only' permissions */ 00230 #define __IO volatile /*!< Defines 'read / write' permissions */ 00231 00232 /* following defines should be used for structure members */ 00233 #define __IM volatile const /*! Defines 'read only' structure member permissions */ 00234 #define __OM volatile /*! Defines 'write only' structure member permissions */ 00235 #define __IOM volatile /*! Defines 'read / write' structure member permissions */ 00236 00237 /*@} end of group Cortex_M33 */ 00238 00239 00240 00241 /******************************************************************************* 00242 * Register Abstraction 00243 Core Register contain: 00244 - Core Register 00245 - Core NVIC Register 00246 - Core SCB Register 00247 - Core SysTick Register 00248 - Core Debug Register 00249 - Core MPU Register 00250 - Core SAU Register 00251 - Core FPU Register 00252 ******************************************************************************/ 00253 /** 00254 \defgroup CMSIS_core_register Defines and Type Definitions 00255 \brief Type definitions and defines for Cortex-M processor based devices. 00256 */ 00257 00258 /** 00259 \ingroup CMSIS_core_register 00260 \defgroup CMSIS_CORE Status and Control Registers 00261 \brief Core Register type definitions. 00262 @{ 00263 */ 00264 00265 /** 00266 \brief Union type to access the Application Program Status Register (APSR). 00267 */ 00268 typedef union 00269 { 00270 struct 00271 { 00272 uint32_t _reserved0:16; /*!< bit: 0..15 Reserved */ 00273 uint32_t GE:4; /*!< bit: 16..19 Greater than or Equal flags */ 00274 uint32_t _reserved1:7; /*!< bit: 20..26 Reserved */ 00275 uint32_t Q:1; /*!< bit: 27 Saturation condition flag */ 00276 uint32_t V:1; /*!< bit: 28 Overflow condition code flag */ 00277 uint32_t C:1; /*!< bit: 29 Carry condition code flag */ 00278 uint32_t Z:1; /*!< bit: 30 Zero condition code flag */ 00279 uint32_t N:1; /*!< bit: 31 Negative condition code flag */ 00280 } b; /*!< Structure used for bit access */ 00281 uint32_t w; /*!< Type used for word access */ 00282 } APSR_Type; 00283 00284 /* APSR Register Definitions */ 00285 #define APSR_N_Pos 31U /*!< APSR: N Position */ 00286 #define APSR_N_Msk (1UL << APSR_N_Pos) /*!< APSR: N Mask */ 00287 00288 #define APSR_Z_Pos 30U /*!< APSR: Z Position */ 00289 #define APSR_Z_Msk (1UL << APSR_Z_Pos) /*!< APSR: Z Mask */ 00290 00291 #define APSR_C_Pos 29U /*!< APSR: C Position */ 00292 #define APSR_C_Msk (1UL << APSR_C_Pos) /*!< APSR: C Mask */ 00293 00294 #define APSR_V_Pos 28U /*!< APSR: V Position */ 00295 #define APSR_V_Msk (1UL << APSR_V_Pos) /*!< APSR: V Mask */ 00296 00297 #define APSR_Q_Pos 27U /*!< APSR: Q Position */ 00298 #define APSR_Q_Msk (1UL << APSR_Q_Pos) /*!< APSR: Q Mask */ 00299 00300 #define APSR_GE_Pos 16U /*!< APSR: GE Position */ 00301 #define APSR_GE_Msk (0xFUL << APSR_GE_Pos) /*!< APSR: GE Mask */ 00302 00303 00304 /** 00305 \brief Union type to access the Interrupt Program Status Register (IPSR). 00306 */ 00307 typedef union 00308 { 00309 struct 00310 { 00311 uint32_t ISR:9; /*!< bit: 0.. 8 Exception number */ 00312 uint32_t _reserved0:23; /*!< bit: 9..31 Reserved */ 00313 } b; /*!< Structure used for bit access */ 00314 uint32_t w; /*!< Type used for word access */ 00315 } IPSR_Type; 00316 00317 /* IPSR Register Definitions */ 00318 #define IPSR_ISR_Pos 0U /*!< IPSR: ISR Position */ 00319 #define IPSR_ISR_Msk (0x1FFUL /*<< IPSR_ISR_Pos*/) /*!< IPSR: ISR Mask */ 00320 00321 00322 /** 00323 \brief Union type to access the Special-Purpose Program Status Registers (xPSR). 00324 */ 00325 typedef union 00326 { 00327 struct 00328 { 00329 uint32_t ISR:9; /*!< bit: 0.. 8 Exception number */ 00330 uint32_t _reserved0:7; /*!< bit: 9..15 Reserved */ 00331 uint32_t GE:4; /*!< bit: 16..19 Greater than or Equal flags */ 00332 uint32_t _reserved1:4; /*!< bit: 20..23 Reserved */ 00333 uint32_t T:1; /*!< bit: 24 Thumb bit (read 0) */ 00334 uint32_t IT:2; /*!< bit: 25..26 saved IT state (read 0) */ 00335 uint32_t Q:1; /*!< bit: 27 Saturation condition flag */ 00336 uint32_t V:1; /*!< bit: 28 Overflow condition code flag */ 00337 uint32_t C:1; /*!< bit: 29 Carry condition code flag */ 00338 uint32_t Z:1; /*!< bit: 30 Zero condition code flag */ 00339 uint32_t N:1; /*!< bit: 31 Negative condition code flag */ 00340 } b; /*!< Structure used for bit access */ 00341 uint32_t w; /*!< Type used for word access */ 00342 } xPSR_Type; 00343 00344 /* xPSR Register Definitions */ 00345 #define xPSR_N_Pos 31U /*!< xPSR: N Position */ 00346 #define xPSR_N_Msk (1UL << xPSR_N_Pos) /*!< xPSR: N Mask */ 00347 00348 #define xPSR_Z_Pos 30U /*!< xPSR: Z Position */ 00349 #define xPSR_Z_Msk (1UL << xPSR_Z_Pos) /*!< xPSR: Z Mask */ 00350 00351 #define xPSR_C_Pos 29U /*!< xPSR: C Position */ 00352 #define xPSR_C_Msk (1UL << xPSR_C_Pos) /*!< xPSR: C Mask */ 00353 00354 #define xPSR_V_Pos 28U /*!< xPSR: V Position */ 00355 #define xPSR_V_Msk (1UL << xPSR_V_Pos) /*!< xPSR: V Mask */ 00356 00357 #define xPSR_Q_Pos 27U /*!< xPSR: Q Position */ 00358 #define xPSR_Q_Msk (1UL << xPSR_Q_Pos) /*!< xPSR: Q Mask */ 00359 00360 #define xPSR_IT_Pos 25U /*!< xPSR: IT Position */ 00361 #define xPSR_IT_Msk (3UL << xPSR_IT_Pos) /*!< xPSR: IT Mask */ 00362 00363 #define xPSR_T_Pos 24U /*!< xPSR: T Position */ 00364 #define xPSR_T_Msk (1UL << xPSR_T_Pos) /*!< xPSR: T Mask */ 00365 00366 #define xPSR_GE_Pos 16U /*!< xPSR: GE Position */ 00367 #define xPSR_GE_Msk (0xFUL << xPSR_GE_Pos) /*!< xPSR: GE Mask */ 00368 00369 #define xPSR_ISR_Pos 0U /*!< xPSR: ISR Position */ 00370 #define xPSR_ISR_Msk (0x1FFUL /*<< xPSR_ISR_Pos*/) /*!< xPSR: ISR Mask */ 00371 00372 00373 /** 00374 \brief Union type to access the Control Registers (CONTROL). 00375 */ 00376 typedef union 00377 { 00378 struct 00379 { 00380 uint32_t nPRIV:1; /*!< bit: 0 Execution privilege in Thread mode */ 00381 uint32_t SPSEL:1; /*!< bit: 1 Stack-pointer select */ 00382 uint32_t FPCA:1; /*!< bit: 2 Floating-point context active */ 00383 uint32_t SFPA:1; /*!< bit: 3 Secure floating-point active */ 00384 uint32_t _reserved1:28; /*!< bit: 4..31 Reserved */ 00385 } b; /*!< Structure used for bit access */ 00386 uint32_t w; /*!< Type used for word access */ 00387 } CONTROL_Type; 00388 00389 /* CONTROL Register Definitions */ 00390 #define CONTROL_SFPA_Pos 3U /*!< CONTROL: SFPA Position */ 00391 #define CONTROL_SFPA_Msk (1UL << CONTROL_SFPA_Pos) /*!< CONTROL: SFPA Mask */ 00392 00393 #define CONTROL_FPCA_Pos 2U /*!< CONTROL: FPCA Position */ 00394 #define CONTROL_FPCA_Msk (1UL << CONTROL_FPCA_Pos) /*!< CONTROL: FPCA Mask */ 00395 00396 #define CONTROL_SPSEL_Pos 1U /*!< CONTROL: SPSEL Position */ 00397 #define CONTROL_SPSEL_Msk (1UL << CONTROL_SPSEL_Pos) /*!< CONTROL: SPSEL Mask */ 00398 00399 #define CONTROL_nPRIV_Pos 0U /*!< CONTROL: nPRIV Position */ 00400 #define CONTROL_nPRIV_Msk (1UL /*<< CONTROL_nPRIV_Pos*/) /*!< CONTROL: nPRIV Mask */ 00401 00402 /*@} end of group CMSIS_CORE */ 00403 00404 00405 /** 00406 \ingroup CMSIS_core_register 00407 \defgroup CMSIS_NVIC Nested Vectored Interrupt Controller (NVIC) 00408 \brief Type definitions for the NVIC Registers 00409 @{ 00410 */ 00411 00412 /** 00413 \brief Structure type to access the Nested Vectored Interrupt Controller (NVIC). 00414 */ 00415 typedef struct 00416 { 00417 __IOM uint32_t ISER[16U]; /*!< Offset: 0x000 (R/W) Interrupt Set Enable Register */ 00418 uint32_t RESERVED0[16U]; 00419 __IOM uint32_t ICER[16U]; /*!< Offset: 0x080 (R/W) Interrupt Clear Enable Register */ 00420 uint32_t RSERVED1[16U]; 00421 __IOM uint32_t ISPR[16U]; /*!< Offset: 0x100 (R/W) Interrupt Set Pending Register */ 00422 uint32_t RESERVED2[16U]; 00423 __IOM uint32_t ICPR[16U]; /*!< Offset: 0x180 (R/W) Interrupt Clear Pending Register */ 00424 uint32_t RESERVED3[16U]; 00425 __IOM uint32_t IABR[16U]; /*!< Offset: 0x200 (R/W) Interrupt Active bit Register */ 00426 uint32_t RESERVED4[16U]; 00427 __IOM uint32_t ITNS[16U]; /*!< Offset: 0x280 (R/W) Interrupt Non-Secure State Register */ 00428 uint32_t RESERVED5[16U]; 00429 __IOM uint8_t IPR[496U]; /*!< Offset: 0x300 (R/W) Interrupt Priority Register (8Bit wide) */ 00430 uint32_t RESERVED6[580U]; 00431 __OM uint32_t STIR; /*!< Offset: 0xE00 ( /W) Software Trigger Interrupt Register */ 00432 } NVIC_Type; 00433 00434 /* Software Triggered Interrupt Register Definitions */ 00435 #define NVIC_STIR_INTID_Pos 0U /*!< STIR: INTLINESNUM Position */ 00436 #define NVIC_STIR_INTID_Msk (0x1FFUL /*<< NVIC_STIR_INTID_Pos*/) /*!< STIR: INTLINESNUM Mask */ 00437 00438 /*@} end of group CMSIS_NVIC */ 00439 00440 00441 /** 00442 \ingroup CMSIS_core_register 00443 \defgroup CMSIS_SCB System Control Block (SCB) 00444 \brief Type definitions for the System Control Block Registers 00445 @{ 00446 */ 00447 00448 /** 00449 \brief Structure type to access the System Control Block (SCB). 00450 */ 00451 typedef struct 00452 { 00453 __IM uint32_t CPUID; /*!< Offset: 0x000 (R/ ) CPUID Base Register */ 00454 __IOM uint32_t ICSR; /*!< Offset: 0x004 (R/W) Interrupt Control and State Register */ 00455 __IOM uint32_t VTOR; /*!< Offset: 0x008 (R/W) Vector Table Offset Register */ 00456 __IOM uint32_t AIRCR; /*!< Offset: 0x00C (R/W) Application Interrupt and Reset Control Register */ 00457 __IOM uint32_t SCR; /*!< Offset: 0x010 (R/W) System Control Register */ 00458 __IOM uint32_t CCR; /*!< Offset: 0x014 (R/W) Configuration Control Register */ 00459 __IOM uint8_t SHPR[12U]; /*!< Offset: 0x018 (R/W) System Handlers Priority Registers (4-7, 8-11, 12-15) */ 00460 __IOM uint32_t SHCSR; /*!< Offset: 0x024 (R/W) System Handler Control and State Register */ 00461 __IOM uint32_t CFSR; /*!< Offset: 0x028 (R/W) Configurable Fault Status Register */ 00462 __IOM uint32_t HFSR; /*!< Offset: 0x02C (R/W) HardFault Status Register */ 00463 __IOM uint32_t DFSR; /*!< Offset: 0x030 (R/W) Debug Fault Status Register */ 00464 __IOM uint32_t MMFAR; /*!< Offset: 0x034 (R/W) MemManage Fault Address Register */ 00465 __IOM uint32_t BFAR; /*!< Offset: 0x038 (R/W) BusFault Address Register */ 00466 __IOM uint32_t AFSR; /*!< Offset: 0x03C (R/W) Auxiliary Fault Status Register */ 00467 __IM uint32_t ID_PFR[2U]; /*!< Offset: 0x040 (R/ ) Processor Feature Register */ 00468 __IM uint32_t ID_DFR; /*!< Offset: 0x048 (R/ ) Debug Feature Register */ 00469 __IM uint32_t ID_ADR; /*!< Offset: 0x04C (R/ ) Auxiliary Feature Register */ 00470 __IM uint32_t ID_MMFR[4U]; /*!< Offset: 0x050 (R/ ) Memory Model Feature Register */ 00471 __IM uint32_t ID_ISAR[6U]; /*!< Offset: 0x060 (R/ ) Instruction Set Attributes Register */ 00472 __IM uint32_t CLIDR; /*!< Offset: 0x078 (R/ ) Cache Level ID register */ 00473 __IM uint32_t CTR; /*!< Offset: 0x07C (R/ ) Cache Type register */ 00474 __IM uint32_t CCSIDR; /*!< Offset: 0x080 (R/ ) Cache Size ID Register */ 00475 __IOM uint32_t CSSELR; /*!< Offset: 0x084 (R/W) Cache Size Selection Register */ 00476 __IOM uint32_t CPACR; /*!< Offset: 0x088 (R/W) Coprocessor Access Control Register */ 00477 __IOM uint32_t NSACR; /*!< Offset: 0x08C (R/W) Non-Secure Access Control Register */ 00478 uint32_t RESERVED3[92U]; 00479 __OM uint32_t STIR; /*!< Offset: 0x200 ( /W) Software Triggered Interrupt Register */ 00480 uint32_t RESERVED4[15U]; 00481 __IM uint32_t MVFR0; /*!< Offset: 0x240 (R/ ) Media and VFP Feature Register 0 */ 00482 __IM uint32_t MVFR1; /*!< Offset: 0x244 (R/ ) Media and VFP Feature Register 1 */ 00483 __IM uint32_t MVFR2; /*!< Offset: 0x248 (R/ ) Media and VFP Feature Register 1 */ 00484 uint32_t RESERVED5[1U]; 00485 __OM uint32_t ICIALLU; /*!< Offset: 0x250 ( /W) I-Cache Invalidate All to PoU */ 00486 uint32_t RESERVED6[1U]; 00487 __OM uint32_t ICIMVAU; /*!< Offset: 0x258 ( /W) I-Cache Invalidate by MVA to PoU */ 00488 __OM uint32_t DCIMVAC; /*!< Offset: 0x25C ( /W) D-Cache Invalidate by MVA to PoC */ 00489 __OM uint32_t DCISW; /*!< Offset: 0x260 ( /W) D-Cache Invalidate by Set-way */ 00490 __OM uint32_t DCCMVAU; /*!< Offset: 0x264 ( /W) D-Cache Clean by MVA to PoU */ 00491 __OM uint32_t DCCMVAC; /*!< Offset: 0x268 ( /W) D-Cache Clean by MVA to PoC */ 00492 __OM uint32_t DCCSW; /*!< Offset: 0x26C ( /W) D-Cache Clean by Set-way */ 00493 __OM uint32_t DCCIMVAC; /*!< Offset: 0x270 ( /W) D-Cache Clean and Invalidate by MVA to PoC */ 00494 __OM uint32_t DCCISW; /*!< Offset: 0x274 ( /W) D-Cache Clean and Invalidate by Set-way */ 00495 uint32_t RESERVED7[6U]; 00496 __IOM uint32_t ITCMCR; /*!< Offset: 0x290 (R/W) Instruction Tightly-Coupled Memory Control Register */ 00497 __IOM uint32_t DTCMCR; /*!< Offset: 0x294 (R/W) Data Tightly-Coupled Memory Control Registers */ 00498 __IOM uint32_t AHBPCR; /*!< Offset: 0x298 (R/W) AHBP Control Register */ 00499 __IOM uint32_t CACR; /*!< Offset: 0x29C (R/W) L1 Cache Control Register */ 00500 __IOM uint32_t AHBSCR; /*!< Offset: 0x2A0 (R/W) AHB Slave Control Register */ 00501 uint32_t RESERVED8[1U]; 00502 __IOM uint32_t ABFSR; /*!< Offset: 0x2A8 (R/W) Auxiliary Bus Fault Status Register */ 00503 } SCB_Type; 00504 00505 /* SCB CPUID Register Definitions */ 00506 #define SCB_CPUID_IMPLEMENTER_Pos 24U /*!< SCB CPUID: IMPLEMENTER Position */ 00507 #define SCB_CPUID_IMPLEMENTER_Msk (0xFFUL << SCB_CPUID_IMPLEMENTER_Pos) /*!< SCB CPUID: IMPLEMENTER Mask */ 00508 00509 #define SCB_CPUID_VARIANT_Pos 20U /*!< SCB CPUID: VARIANT Position */ 00510 #define SCB_CPUID_VARIANT_Msk (0xFUL << SCB_CPUID_VARIANT_Pos) /*!< SCB CPUID: VARIANT Mask */ 00511 00512 #define SCB_CPUID_ARCHITECTURE_Pos 16U /*!< SCB CPUID: ARCHITECTURE Position */ 00513 #define SCB_CPUID_ARCHITECTURE_Msk (0xFUL << SCB_CPUID_ARCHITECTURE_Pos) /*!< SCB CPUID: ARCHITECTURE Mask */ 00514 00515 #define SCB_CPUID_PARTNO_Pos 4U /*!< SCB CPUID: PARTNO Position */ 00516 #define SCB_CPUID_PARTNO_Msk (0xFFFUL << SCB_CPUID_PARTNO_Pos) /*!< SCB CPUID: PARTNO Mask */ 00517 00518 #define SCB_CPUID_REVISION_Pos 0U /*!< SCB CPUID: REVISION Position */ 00519 #define SCB_CPUID_REVISION_Msk (0xFUL /*<< SCB_CPUID_REVISION_Pos*/) /*!< SCB CPUID: REVISION Mask */ 00520 00521 /* SCB Interrupt Control State Register Definitions */ 00522 #define SCB_ICSR_PENDNMISET_Pos 31U /*!< SCB ICSR: PENDNMISET Position */ 00523 #define SCB_ICSR_PENDNMISET_Msk (1UL << SCB_ICSR_PENDNMISET_Pos) /*!< SCB ICSR: PENDNMISET Mask */ 00524 00525 #define SCB_ICSR_PENDNMICLR_Pos 30U /*!< SCB ICSR: PENDNMICLR Position */ 00526 #define SCB_ICSR_PENDNMICLR_Msk (1UL << SCB_ICSR_PENDNMICLR_Pos) /*!< SCB ICSR: PENDNMICLR Mask */ 00527 00528 #define SCB_ICSR_PENDSVSET_Pos 28U /*!< SCB ICSR: PENDSVSET Position */ 00529 #define SCB_ICSR_PENDSVSET_Msk (1UL << SCB_ICSR_PENDSVSET_Pos) /*!< SCB ICSR: PENDSVSET Mask */ 00530 00531 #define SCB_ICSR_PENDSVCLR_Pos 27U /*!< SCB ICSR: PENDSVCLR Position */ 00532 #define SCB_ICSR_PENDSVCLR_Msk (1UL << SCB_ICSR_PENDSVCLR_Pos) /*!< SCB ICSR: PENDSVCLR Mask */ 00533 00534 #define SCB_ICSR_PENDSTSET_Pos 26U /*!< SCB ICSR: PENDSTSET Position */ 00535 #define SCB_ICSR_PENDSTSET_Msk (1UL << SCB_ICSR_PENDSTSET_Pos) /*!< SCB ICSR: PENDSTSET Mask */ 00536 00537 #define SCB_ICSR_PENDSTCLR_Pos 25U /*!< SCB ICSR: PENDSTCLR Position */ 00538 #define SCB_ICSR_PENDSTCLR_Msk (1UL << SCB_ICSR_PENDSTCLR_Pos) /*!< SCB ICSR: PENDSTCLR Mask */ 00539 00540 #define SCB_ICSR_STTNS_Pos 24U /*!< SCB ICSR: STTNS Position (Security Extension) */ 00541 #define SCB_ICSR_STTNS_Msk (1UL << SCB_ICSR_STTNS_Pos) /*!< SCB ICSR: STTNS Mask (Security Extension) */ 00542 00543 #define SCB_ICSR_ISRPREEMPT_Pos 23U /*!< SCB ICSR: ISRPREEMPT Position */ 00544 #define SCB_ICSR_ISRPREEMPT_Msk (1UL << SCB_ICSR_ISRPREEMPT_Pos) /*!< SCB ICSR: ISRPREEMPT Mask */ 00545 00546 #define SCB_ICSR_ISRPENDING_Pos 22U /*!< SCB ICSR: ISRPENDING Position */ 00547 #define SCB_ICSR_ISRPENDING_Msk (1UL << SCB_ICSR_ISRPENDING_Pos) /*!< SCB ICSR: ISRPENDING Mask */ 00548 00549 #define SCB_ICSR_VECTPENDING_Pos 12U /*!< SCB ICSR: VECTPENDING Position */ 00550 #define SCB_ICSR_VECTPENDING_Msk (0x1FFUL << SCB_ICSR_VECTPENDING_Pos) /*!< SCB ICSR: VECTPENDING Mask */ 00551 00552 #define SCB_ICSR_RETTOBASE_Pos 11U /*!< SCB ICSR: RETTOBASE Position */ 00553 #define SCB_ICSR_RETTOBASE_Msk (1UL << SCB_ICSR_RETTOBASE_Pos) /*!< SCB ICSR: RETTOBASE Mask */ 00554 00555 #define SCB_ICSR_VECTACTIVE_Pos 0U /*!< SCB ICSR: VECTACTIVE Position */ 00556 #define SCB_ICSR_VECTACTIVE_Msk (0x1FFUL /*<< SCB_ICSR_VECTACTIVE_Pos*/) /*!< SCB ICSR: VECTACTIVE Mask */ 00557 00558 /* SCB Vector Table Offset Register Definitions */ 00559 #define SCB_VTOR_TBLOFF_Pos 7U /*!< SCB VTOR: TBLOFF Position */ 00560 #define SCB_VTOR_TBLOFF_Msk (0x1FFFFFFUL << SCB_VTOR_TBLOFF_Pos) /*!< SCB VTOR: TBLOFF Mask */ 00561 00562 /* SCB Application Interrupt and Reset Control Register Definitions */ 00563 #define SCB_AIRCR_VECTKEY_Pos 16U /*!< SCB AIRCR: VECTKEY Position */ 00564 #define SCB_AIRCR_VECTKEY_Msk (0xFFFFUL << SCB_AIRCR_VECTKEY_Pos) /*!< SCB AIRCR: VECTKEY Mask */ 00565 00566 #define SCB_AIRCR_VECTKEYSTAT_Pos 16U /*!< SCB AIRCR: VECTKEYSTAT Position */ 00567 #define SCB_AIRCR_VECTKEYSTAT_Msk (0xFFFFUL << SCB_AIRCR_VECTKEYSTAT_Pos) /*!< SCB AIRCR: VECTKEYSTAT Mask */ 00568 00569 #define SCB_AIRCR_ENDIANESS_Pos 15U /*!< SCB AIRCR: ENDIANESS Position */ 00570 #define SCB_AIRCR_ENDIANESS_Msk (1UL << SCB_AIRCR_ENDIANESS_Pos) /*!< SCB AIRCR: ENDIANESS Mask */ 00571 00572 #define SCB_AIRCR_PRIS_Pos 14U /*!< SCB AIRCR: PRIS Position */ 00573 #define SCB_AIRCR_PRIS_Msk (1UL << SCB_AIRCR_PRIS_Pos) /*!< SCB AIRCR: PRIS Mask */ 00574 00575 #define SCB_AIRCR_BFHFNMINS_Pos 13U /*!< SCB AIRCR: BFHFNMINS Position */ 00576 #define SCB_AIRCR_BFHFNMINS_Msk (1UL << SCB_AIRCR_BFHFNMINS_Pos) /*!< SCB AIRCR: BFHFNMINS Mask */ 00577 00578 #define SCB_AIRCR_PRIGROUP_Pos 8U /*!< SCB AIRCR: PRIGROUP Position */ 00579 #define SCB_AIRCR_PRIGROUP_Msk (7UL << SCB_AIRCR_PRIGROUP_Pos) /*!< SCB AIRCR: PRIGROUP Mask */ 00580 00581 #define SCB_AIRCR_SYSRESETREQS_Pos 3U /*!< SCB AIRCR: SYSRESETREQS Position */ 00582 #define SCB_AIRCR_SYSRESETREQS_Msk (1UL << SCB_AIRCR_SYSRESETREQS_Pos) /*!< SCB AIRCR: SYSRESETREQS Mask */ 00583 00584 #define SCB_AIRCR_SYSRESETREQ_Pos 2U /*!< SCB AIRCR: SYSRESETREQ Position */ 00585 #define SCB_AIRCR_SYSRESETREQ_Msk (1UL << SCB_AIRCR_SYSRESETREQ_Pos) /*!< SCB AIRCR: SYSRESETREQ Mask */ 00586 00587 #define SCB_AIRCR_VECTCLRACTIVE_Pos 1U /*!< SCB AIRCR: VECTCLRACTIVE Position */ 00588 #define SCB_AIRCR_VECTCLRACTIVE_Msk (1UL << SCB_AIRCR_VECTCLRACTIVE_Pos) /*!< SCB AIRCR: VECTCLRACTIVE Mask */ 00589 00590 /* SCB System Control Register Definitions */ 00591 #define SCB_SCR_SEVONPEND_Pos 4U /*!< SCB SCR: SEVONPEND Position */ 00592 #define SCB_SCR_SEVONPEND_Msk (1UL << SCB_SCR_SEVONPEND_Pos) /*!< SCB SCR: SEVONPEND Mask */ 00593 00594 #define SCB_SCR_SLEEPDEEPS_Pos 3U /*!< SCB SCR: SLEEPDEEPS Position */ 00595 #define SCB_SCR_SLEEPDEEPS_Msk (1UL << SCB_SCR_SLEEPDEEPS_Pos) /*!< SCB SCR: SLEEPDEEPS Mask */ 00596 00597 #define SCB_SCR_SLEEPDEEP_Pos 2U /*!< SCB SCR: SLEEPDEEP Position */ 00598 #define SCB_SCR_SLEEPDEEP_Msk (1UL << SCB_SCR_SLEEPDEEP_Pos) /*!< SCB SCR: SLEEPDEEP Mask */ 00599 00600 #define SCB_SCR_SLEEPONEXIT_Pos 1U /*!< SCB SCR: SLEEPONEXIT Position */ 00601 #define SCB_SCR_SLEEPONEXIT_Msk (1UL << SCB_SCR_SLEEPONEXIT_Pos) /*!< SCB SCR: SLEEPONEXIT Mask */ 00602 00603 /* SCB Configuration Control Register Definitions */ 00604 #define SCB_CCR_BP_Pos 18U /*!< SCB CCR: BP Position */ 00605 #define SCB_CCR_BP_Msk (1UL << SCB_CCR_BP_Pos) /*!< SCB CCR: BP Mask */ 00606 00607 #define SCB_CCR_IC_Pos 17U /*!< SCB CCR: IC Position */ 00608 #define SCB_CCR_IC_Msk (1UL << SCB_CCR_IC_Pos) /*!< SCB CCR: IC Mask */ 00609 00610 #define SCB_CCR_DC_Pos 16U /*!< SCB CCR: DC Position */ 00611 #define SCB_CCR_DC_Msk (1UL << SCB_CCR_DC_Pos) /*!< SCB CCR: DC Mask */ 00612 00613 #define SCB_CCR_STKOFHFNMIGN_Pos 10U /*!< SCB CCR: STKOFHFNMIGN Position */ 00614 #define SCB_CCR_STKOFHFNMIGN_Msk (1UL << SCB_CCR_STKOFHFNMIGN_Pos) /*!< SCB CCR: STKOFHFNMIGN Mask */ 00615 00616 #define SCB_CCR_BFHFNMIGN_Pos 8U /*!< SCB CCR: BFHFNMIGN Position */ 00617 #define SCB_CCR_BFHFNMIGN_Msk (1UL << SCB_CCR_BFHFNMIGN_Pos) /*!< SCB CCR: BFHFNMIGN Mask */ 00618 00619 #define SCB_CCR_DIV_0_TRP_Pos 4U /*!< SCB CCR: DIV_0_TRP Position */ 00620 #define SCB_CCR_DIV_0_TRP_Msk (1UL << SCB_CCR_DIV_0_TRP_Pos) /*!< SCB CCR: DIV_0_TRP Mask */ 00621 00622 #define SCB_CCR_UNALIGN_TRP_Pos 3U /*!< SCB CCR: UNALIGN_TRP Position */ 00623 #define SCB_CCR_UNALIGN_TRP_Msk (1UL << SCB_CCR_UNALIGN_TRP_Pos) /*!< SCB CCR: UNALIGN_TRP Mask */ 00624 00625 #define SCB_CCR_USERSETMPEND_Pos 1U /*!< SCB CCR: USERSETMPEND Position */ 00626 #define SCB_CCR_USERSETMPEND_Msk (1UL << SCB_CCR_USERSETMPEND_Pos) /*!< SCB CCR: USERSETMPEND Mask */ 00627 00628 /* SCB System Handler Control and State Register Definitions */ 00629 #define SCB_SHCSR_HARDFAULTPENDED_Pos 21U /*!< SCB SHCSR: HARDFAULTPENDED Position */ 00630 #define SCB_SHCSR_HARDFAULTPENDED_Msk (1UL << SCB_SHCSR_HARDFAULTPENDED_Pos) /*!< SCB SHCSR: HARDFAULTPENDED Mask */ 00631 00632 #define SCB_SHCSR_SECUREFAULTPENDED_Pos 20U /*!< SCB SHCSR: SECUREFAULTPENDED Position */ 00633 #define SCB_SHCSR_SECUREFAULTPENDED_Msk (1UL << SCB_SHCSR_SECUREFAULTPENDED_Pos) /*!< SCB SHCSR: SECUREFAULTPENDED Mask */ 00634 00635 #define SCB_SHCSR_SECUREFAULTENA_Pos 19U /*!< SCB SHCSR: SECUREFAULTENA Position */ 00636 #define SCB_SHCSR_SECUREFAULTENA_Msk (1UL << SCB_SHCSR_SECUREFAULTENA_Pos) /*!< SCB SHCSR: SECUREFAULTENA Mask */ 00637 00638 #define SCB_SHCSR_USGFAULTENA_Pos 18U /*!< SCB SHCSR: USGFAULTENA Position */ 00639 #define SCB_SHCSR_USGFAULTENA_Msk (1UL << SCB_SHCSR_USGFAULTENA_Pos) /*!< SCB SHCSR: USGFAULTENA Mask */ 00640 00641 #define SCB_SHCSR_BUSFAULTENA_Pos 17U /*!< SCB SHCSR: BUSFAULTENA Position */ 00642 #define SCB_SHCSR_BUSFAULTENA_Msk (1UL << SCB_SHCSR_BUSFAULTENA_Pos) /*!< SCB SHCSR: BUSFAULTENA Mask */ 00643 00644 #define SCB_SHCSR_MEMFAULTENA_Pos 16U /*!< SCB SHCSR: MEMFAULTENA Position */ 00645 #define SCB_SHCSR_MEMFAULTENA_Msk (1UL << SCB_SHCSR_MEMFAULTENA_Pos) /*!< SCB SHCSR: MEMFAULTENA Mask */ 00646 00647 #define SCB_SHCSR_SVCALLPENDED_Pos 15U /*!< SCB SHCSR: SVCALLPENDED Position */ 00648 #define SCB_SHCSR_SVCALLPENDED_Msk (1UL << SCB_SHCSR_SVCALLPENDED_Pos) /*!< SCB SHCSR: SVCALLPENDED Mask */ 00649 00650 #define SCB_SHCSR_BUSFAULTPENDED_Pos 14U /*!< SCB SHCSR: BUSFAULTPENDED Position */ 00651 #define SCB_SHCSR_BUSFAULTPENDED_Msk (1UL << SCB_SHCSR_BUSFAULTPENDED_Pos) /*!< SCB SHCSR: BUSFAULTPENDED Mask */ 00652 00653 #define SCB_SHCSR_MEMFAULTPENDED_Pos 13U /*!< SCB SHCSR: MEMFAULTPENDED Position */ 00654 #define SCB_SHCSR_MEMFAULTPENDED_Msk (1UL << SCB_SHCSR_MEMFAULTPENDED_Pos) /*!< SCB SHCSR: MEMFAULTPENDED Mask */ 00655 00656 #define SCB_SHCSR_USGFAULTPENDED_Pos 12U /*!< SCB SHCSR: USGFAULTPENDED Position */ 00657 #define SCB_SHCSR_USGFAULTPENDED_Msk (1UL << SCB_SHCSR_USGFAULTPENDED_Pos) /*!< SCB SHCSR: USGFAULTPENDED Mask */ 00658 00659 #define SCB_SHCSR_SYSTICKACT_Pos 11U /*!< SCB SHCSR: SYSTICKACT Position */ 00660 #define SCB_SHCSR_SYSTICKACT_Msk (1UL << SCB_SHCSR_SYSTICKACT_Pos) /*!< SCB SHCSR: SYSTICKACT Mask */ 00661 00662 #define SCB_SHCSR_PENDSVACT_Pos 10U /*!< SCB SHCSR: PENDSVACT Position */ 00663 #define SCB_SHCSR_PENDSVACT_Msk (1UL << SCB_SHCSR_PENDSVACT_Pos) /*!< SCB SHCSR: PENDSVACT Mask */ 00664 00665 #define SCB_SHCSR_MONITORACT_Pos 8U /*!< SCB SHCSR: MONITORACT Position */ 00666 #define SCB_SHCSR_MONITORACT_Msk (1UL << SCB_SHCSR_MONITORACT_Pos) /*!< SCB SHCSR: MONITORACT Mask */ 00667 00668 #define SCB_SHCSR_SVCALLACT_Pos 7U /*!< SCB SHCSR: SVCALLACT Position */ 00669 #define SCB_SHCSR_SVCALLACT_Msk (1UL << SCB_SHCSR_SVCALLACT_Pos) /*!< SCB SHCSR: SVCALLACT Mask */ 00670 00671 #define SCB_SHCSR_NMIACT_Pos 5U /*!< SCB SHCSR: NMIACT Position */ 00672 #define SCB_SHCSR_NMIACT_Msk (1UL << SCB_SHCSR_NMIACT_Pos) /*!< SCB SHCSR: NMIACT Mask */ 00673 00674 #define SCB_SHCSR_SECUREFAULTACT_Pos 4U /*!< SCB SHCSR: SECUREFAULTACT Position */ 00675 #define SCB_SHCSR_SECUREFAULTACT_Msk (1UL << SCB_SHCSR_SECUREFAULTACT_Pos) /*!< SCB SHCSR: SECUREFAULTACT Mask */ 00676 00677 #define SCB_SHCSR_USGFAULTACT_Pos 3U /*!< SCB SHCSR: USGFAULTACT Position */ 00678 #define SCB_SHCSR_USGFAULTACT_Msk (1UL << SCB_SHCSR_USGFAULTACT_Pos) /*!< SCB SHCSR: USGFAULTACT Mask */ 00679 00680 #define SCB_SHCSR_HARDFAULTACT_Pos 2U /*!< SCB SHCSR: HARDFAULTACT Position */ 00681 #define SCB_SHCSR_HARDFAULTACT_Msk (1UL << SCB_SHCSR_HARDFAULTACT_Pos) /*!< SCB SHCSR: HARDFAULTACT Mask */ 00682 00683 #define SCB_SHCSR_BUSFAULTACT_Pos 1U /*!< SCB SHCSR: BUSFAULTACT Position */ 00684 #define SCB_SHCSR_BUSFAULTACT_Msk (1UL << SCB_SHCSR_BUSFAULTACT_Pos) /*!< SCB SHCSR: BUSFAULTACT Mask */ 00685 00686 #define SCB_SHCSR_MEMFAULTACT_Pos 0U /*!< SCB SHCSR: MEMFAULTACT Position */ 00687 #define SCB_SHCSR_MEMFAULTACT_Msk (1UL /*<< SCB_SHCSR_MEMFAULTACT_Pos*/) /*!< SCB SHCSR: MEMFAULTACT Mask */ 00688 00689 /* SCB Configurable Fault Status Register Definitions */ 00690 #define SCB_CFSR_USGFAULTSR_Pos 16U /*!< SCB CFSR: Usage Fault Status Register Position */ 00691 #define SCB_CFSR_USGFAULTSR_Msk (0xFFFFUL << SCB_CFSR_USGFAULTSR_Pos) /*!< SCB CFSR: Usage Fault Status Register Mask */ 00692 00693 #define SCB_CFSR_BUSFAULTSR_Pos 8U /*!< SCB CFSR: Bus Fault Status Register Position */ 00694 #define SCB_CFSR_BUSFAULTSR_Msk (0xFFUL << SCB_CFSR_BUSFAULTSR_Pos) /*!< SCB CFSR: Bus Fault Status Register Mask */ 00695 00696 #define SCB_CFSR_MEMFAULTSR_Pos 0U /*!< SCB CFSR: Memory Manage Fault Status Register Position */ 00697 #define SCB_CFSR_MEMFAULTSR_Msk (0xFFUL /*<< SCB_CFSR_MEMFAULTSR_Pos*/) /*!< SCB CFSR: Memory Manage Fault Status Register Mask */ 00698 00699 /* MemManage Fault Status Register (part of SCB Configurable Fault Status Register) */ 00700 #define SCB_CFSR_MMARVALID_Pos (SCB_SHCSR_MEMFAULTACT_Pos + 7U) /*!< SCB CFSR (MMFSR): MMARVALID Position */ 00701 #define SCB_CFSR_MMARVALID_Msk (1UL << SCB_CFSR_MMARVALID_Pos) /*!< SCB CFSR (MMFSR): MMARVALID Mask */ 00702 00703 #define SCB_CFSR_MLSPERR_Pos (SCB_SHCSR_MEMFAULTACT_Pos + 5U) /*!< SCB CFSR (MMFSR): MLSPERR Position */ 00704 #define SCB_CFSR_MLSPERR_Msk (1UL << SCB_CFSR_MLSPERR_Pos) /*!< SCB CFSR (MMFSR): MLSPERR Mask */ 00705 00706 #define SCB_CFSR_MSTKERR_Pos (SCB_SHCSR_MEMFAULTACT_Pos + 4U) /*!< SCB CFSR (MMFSR): MSTKERR Position */ 00707 #define SCB_CFSR_MSTKERR_Msk (1UL << SCB_CFSR_MSTKERR_Pos) /*!< SCB CFSR (MMFSR): MSTKERR Mask */ 00708 00709 #define SCB_CFSR_MUNSTKERR_Pos (SCB_SHCSR_MEMFAULTACT_Pos + 3U) /*!< SCB CFSR (MMFSR): MUNSTKERR Position */ 00710 #define SCB_CFSR_MUNSTKERR_Msk (1UL << SCB_CFSR_MUNSTKERR_Pos) /*!< SCB CFSR (MMFSR): MUNSTKERR Mask */ 00711 00712 #define SCB_CFSR_DACCVIOL_Pos (SCB_SHCSR_MEMFAULTACT_Pos + 1U) /*!< SCB CFSR (MMFSR): DACCVIOL Position */ 00713 #define SCB_CFSR_DACCVIOL_Msk (1UL << SCB_CFSR_DACCVIOL_Pos) /*!< SCB CFSR (MMFSR): DACCVIOL Mask */ 00714 00715 #define SCB_CFSR_IACCVIOL_Pos (SCB_SHCSR_MEMFAULTACT_Pos + 0U) /*!< SCB CFSR (MMFSR): IACCVIOL Position */ 00716 #define SCB_CFSR_IACCVIOL_Msk (1UL /*<< SCB_CFSR_IACCVIOL_Pos*/) /*!< SCB CFSR (MMFSR): IACCVIOL Mask */ 00717 00718 /* BusFault Status Register (part of SCB Configurable Fault Status Register) */ 00719 #define SCB_CFSR_BFARVALID_Pos (SCB_CFSR_BUSFAULTSR_Pos + 7U) /*!< SCB CFSR (BFSR): BFARVALID Position */ 00720 #define SCB_CFSR_BFARVALID_Msk (1UL << SCB_CFSR_BFARVALID_Pos) /*!< SCB CFSR (BFSR): BFARVALID Mask */ 00721 00722 #define SCB_CFSR_LSPERR_Pos (SCB_CFSR_BUSFAULTSR_Pos + 5U) /*!< SCB CFSR (BFSR): LSPERR Position */ 00723 #define SCB_CFSR_LSPERR_Msk (1UL << SCB_CFSR_LSPERR_Pos) /*!< SCB CFSR (BFSR): LSPERR Mask */ 00724 00725 #define SCB_CFSR_STKERR_Pos (SCB_CFSR_BUSFAULTSR_Pos + 4U) /*!< SCB CFSR (BFSR): STKERR Position */ 00726 #define SCB_CFSR_STKERR_Msk (1UL << SCB_CFSR_STKERR_Pos) /*!< SCB CFSR (BFSR): STKERR Mask */ 00727 00728 #define SCB_CFSR_UNSTKERR_Pos (SCB_CFSR_BUSFAULTSR_Pos + 3U) /*!< SCB CFSR (BFSR): UNSTKERR Position */ 00729 #define SCB_CFSR_UNSTKERR_Msk (1UL << SCB_CFSR_UNSTKERR_Pos) /*!< SCB CFSR (BFSR): UNSTKERR Mask */ 00730 00731 #define SCB_CFSR_IMPRECISERR_Pos (SCB_CFSR_BUSFAULTSR_Pos + 2U) /*!< SCB CFSR (BFSR): IMPRECISERR Position */ 00732 #define SCB_CFSR_IMPRECISERR_Msk (1UL << SCB_CFSR_IMPRECISERR_Pos) /*!< SCB CFSR (BFSR): IMPRECISERR Mask */ 00733 00734 #define SCB_CFSR_PRECISERR_Pos (SCB_CFSR_BUSFAULTSR_Pos + 1U) /*!< SCB CFSR (BFSR): PRECISERR Position */ 00735 #define SCB_CFSR_PRECISERR_Msk (1UL << SCB_CFSR_PRECISERR_Pos) /*!< SCB CFSR (BFSR): PRECISERR Mask */ 00736 00737 #define SCB_CFSR_IBUSERR_Pos (SCB_CFSR_BUSFAULTSR_Pos + 0U) /*!< SCB CFSR (BFSR): IBUSERR Position */ 00738 #define SCB_CFSR_IBUSERR_Msk (1UL << SCB_CFSR_IBUSERR_Pos) /*!< SCB CFSR (BFSR): IBUSERR Mask */ 00739 00740 /* UsageFault Status Register (part of SCB Configurable Fault Status Register) */ 00741 #define SCB_CFSR_DIVBYZERO_Pos (SCB_CFSR_USGFAULTSR_Pos + 9U) /*!< SCB CFSR (UFSR): DIVBYZERO Position */ 00742 #define SCB_CFSR_DIVBYZERO_Msk (1UL << SCB_CFSR_DIVBYZERO_Pos) /*!< SCB CFSR (UFSR): DIVBYZERO Mask */ 00743 00744 #define SCB_CFSR_UNALIGNED_Pos (SCB_CFSR_USGFAULTSR_Pos + 8U) /*!< SCB CFSR (UFSR): UNALIGNED Position */ 00745 #define SCB_CFSR_UNALIGNED_Msk (1UL << SCB_CFSR_UNALIGNED_Pos) /*!< SCB CFSR (UFSR): UNALIGNED Mask */ 00746 00747 #define SCB_CFSR_STKOF_Pos (SCB_CFSR_USGFAULTSR_Pos + 4U) /*!< SCB CFSR (UFSR): STKOF Position */ 00748 #define SCB_CFSR_STKOF_Msk (1UL << SCB_CFSR_STKOF_Pos) /*!< SCB CFSR (UFSR): STKOF Mask */ 00749 00750 #define SCB_CFSR_NOCP_Pos (SCB_CFSR_USGFAULTSR_Pos + 3U) /*!< SCB CFSR (UFSR): NOCP Position */ 00751 #define SCB_CFSR_NOCP_Msk (1UL << SCB_CFSR_NOCP_Pos) /*!< SCB CFSR (UFSR): NOCP Mask */ 00752 00753 #define SCB_CFSR_INVPC_Pos (SCB_CFSR_USGFAULTSR_Pos + 2U) /*!< SCB CFSR (UFSR): INVPC Position */ 00754 #define SCB_CFSR_INVPC_Msk (1UL << SCB_CFSR_INVPC_Pos) /*!< SCB CFSR (UFSR): INVPC Mask */ 00755 00756 #define SCB_CFSR_INVSTATE_Pos (SCB_CFSR_USGFAULTSR_Pos + 1U) /*!< SCB CFSR (UFSR): INVSTATE Position */ 00757 #define SCB_CFSR_INVSTATE_Msk (1UL << SCB_CFSR_INVSTATE_Pos) /*!< SCB CFSR (UFSR): INVSTATE Mask */ 00758 00759 #define SCB_CFSR_UNDEFINSTR_Pos (SCB_CFSR_USGFAULTSR_Pos + 0U) /*!< SCB CFSR (UFSR): UNDEFINSTR Position */ 00760 #define SCB_CFSR_UNDEFINSTR_Msk (1UL << SCB_CFSR_UNDEFINSTR_Pos) /*!< SCB CFSR (UFSR): UNDEFINSTR Mask */ 00761 00762 /* SCB Hard Fault Status Register Definitions */ 00763 #define SCB_HFSR_DEBUGEVT_Pos 31U /*!< SCB HFSR: DEBUGEVT Position */ 00764 #define SCB_HFSR_DEBUGEVT_Msk (1UL << SCB_HFSR_DEBUGEVT_Pos) /*!< SCB HFSR: DEBUGEVT Mask */ 00765 00766 #define SCB_HFSR_FORCED_Pos 30U /*!< SCB HFSR: FORCED Position */ 00767 #define SCB_HFSR_FORCED_Msk (1UL << SCB_HFSR_FORCED_Pos) /*!< SCB HFSR: FORCED Mask */ 00768 00769 #define SCB_HFSR_VECTTBL_Pos 1U /*!< SCB HFSR: VECTTBL Position */ 00770 #define SCB_HFSR_VECTTBL_Msk (1UL << SCB_HFSR_VECTTBL_Pos) /*!< SCB HFSR: VECTTBL Mask */ 00771 00772 /* SCB Debug Fault Status Register Definitions */ 00773 #define SCB_DFSR_EXTERNAL_Pos 4U /*!< SCB DFSR: EXTERNAL Position */ 00774 #define SCB_DFSR_EXTERNAL_Msk (1UL << SCB_DFSR_EXTERNAL_Pos) /*!< SCB DFSR: EXTERNAL Mask */ 00775 00776 #define SCB_DFSR_VCATCH_Pos 3U /*!< SCB DFSR: VCATCH Position */ 00777 #define SCB_DFSR_VCATCH_Msk (1UL << SCB_DFSR_VCATCH_Pos) /*!< SCB DFSR: VCATCH Mask */ 00778 00779 #define SCB_DFSR_DWTTRAP_Pos 2U /*!< SCB DFSR: DWTTRAP Position */ 00780 #define SCB_DFSR_DWTTRAP_Msk (1UL << SCB_DFSR_DWTTRAP_Pos) /*!< SCB DFSR: DWTTRAP Mask */ 00781 00782 #define SCB_DFSR_BKPT_Pos 1U /*!< SCB DFSR: BKPT Position */ 00783 #define SCB_DFSR_BKPT_Msk (1UL << SCB_DFSR_BKPT_Pos) /*!< SCB DFSR: BKPT Mask */ 00784 00785 #define SCB_DFSR_HALTED_Pos 0U /*!< SCB DFSR: HALTED Position */ 00786 #define SCB_DFSR_HALTED_Msk (1UL /*<< SCB_DFSR_HALTED_Pos*/) /*!< SCB DFSR: HALTED Mask */ 00787 00788 /* SCB Non-Secure Access Control Register Definitions */ 00789 #define SCB_NSACR_CP11_Pos 11U /*!< SCB NSACR: CP11 Position */ 00790 #define SCB_NSACR_CP11_Msk (1UL << SCB_NSACR_CP11_Pos) /*!< SCB NSACR: CP11 Mask */ 00791 00792 #define SCB_NSACR_CP10_Pos 10U /*!< SCB NSACR: CP10 Position */ 00793 #define SCB_NSACR_CP10_Msk (1UL << SCB_NSACR_CP10_Pos) /*!< SCB NSACR: CP10 Mask */ 00794 00795 #define SCB_NSACR_CPn_Pos 0U /*!< SCB NSACR: CPn Position */ 00796 #define SCB_NSACR_CPn_Msk (1UL /*<< SCB_NSACR_CPn_Pos*/) /*!< SCB NSACR: CPn Mask */ 00797 00798 /* SCB Cache Level ID Register Definitions */ 00799 #define SCB_CLIDR_LOUU_Pos 27U /*!< SCB CLIDR: LoUU Position */ 00800 #define SCB_CLIDR_LOUU_Msk (7UL << SCB_CLIDR_LOUU_Pos) /*!< SCB CLIDR: LoUU Mask */ 00801 00802 #define SCB_CLIDR_LOC_Pos 24U /*!< SCB CLIDR: LoC Position */ 00803 #define SCB_CLIDR_LOC_Msk (7UL << SCB_CLIDR_LOC_Pos) /*!< SCB CLIDR: LoC Mask */ 00804 00805 /* SCB Cache Type Register Definitions */ 00806 #define SCB_CTR_FORMAT_Pos 29U /*!< SCB CTR: Format Position */ 00807 #define SCB_CTR_FORMAT_Msk (7UL << SCB_CTR_FORMAT_Pos) /*!< SCB CTR: Format Mask */ 00808 00809 #define SCB_CTR_CWG_Pos 24U /*!< SCB CTR: CWG Position */ 00810 #define SCB_CTR_CWG_Msk (0xFUL << SCB_CTR_CWG_Pos) /*!< SCB CTR: CWG Mask */ 00811 00812 #define SCB_CTR_ERG_Pos 20U /*!< SCB CTR: ERG Position */ 00813 #define SCB_CTR_ERG_Msk (0xFUL << SCB_CTR_ERG_Pos) /*!< SCB CTR: ERG Mask */ 00814 00815 #define SCB_CTR_DMINLINE_Pos 16U /*!< SCB CTR: DminLine Position */ 00816 #define SCB_CTR_DMINLINE_Msk (0xFUL << SCB_CTR_DMINLINE_Pos) /*!< SCB CTR: DminLine Mask */ 00817 00818 #define SCB_CTR_IMINLINE_Pos 0U /*!< SCB CTR: ImInLine Position */ 00819 #define SCB_CTR_IMINLINE_Msk (0xFUL /*<< SCB_CTR_IMINLINE_Pos*/) /*!< SCB CTR: ImInLine Mask */ 00820 00821 /* SCB Cache Size ID Register Definitions */ 00822 #define SCB_CCSIDR_WT_Pos 31U /*!< SCB CCSIDR: WT Position */ 00823 #define SCB_CCSIDR_WT_Msk (1UL << SCB_CCSIDR_WT_Pos) /*!< SCB CCSIDR: WT Mask */ 00824 00825 #define SCB_CCSIDR_WB_Pos 30U /*!< SCB CCSIDR: WB Position */ 00826 #define SCB_CCSIDR_WB_Msk (1UL << SCB_CCSIDR_WB_Pos) /*!< SCB CCSIDR: WB Mask */ 00827 00828 #define SCB_CCSIDR_RA_Pos 29U /*!< SCB CCSIDR: RA Position */ 00829 #define SCB_CCSIDR_RA_Msk (1UL << SCB_CCSIDR_RA_Pos) /*!< SCB CCSIDR: RA Mask */ 00830 00831 #define SCB_CCSIDR_WA_Pos 28U /*!< SCB CCSIDR: WA Position */ 00832 #define SCB_CCSIDR_WA_Msk (1UL << SCB_CCSIDR_WA_Pos) /*!< SCB CCSIDR: WA Mask */ 00833 00834 #define SCB_CCSIDR_NUMSETS_Pos 13U /*!< SCB CCSIDR: NumSets Position */ 00835 #define SCB_CCSIDR_NUMSETS_Msk (0x7FFFUL << SCB_CCSIDR_NUMSETS_Pos) /*!< SCB CCSIDR: NumSets Mask */ 00836 00837 #define SCB_CCSIDR_ASSOCIATIVITY_Pos 3U /*!< SCB CCSIDR: Associativity Position */ 00838 #define SCB_CCSIDR_ASSOCIATIVITY_Msk (0x3FFUL << SCB_CCSIDR_ASSOCIATIVITY_Pos) /*!< SCB CCSIDR: Associativity Mask */ 00839 00840 #define SCB_CCSIDR_LINESIZE_Pos 0U /*!< SCB CCSIDR: LineSize Position */ 00841 #define SCB_CCSIDR_LINESIZE_Msk (7UL /*<< SCB_CCSIDR_LINESIZE_Pos*/) /*!< SCB CCSIDR: LineSize Mask */ 00842 00843 /* SCB Cache Size Selection Register Definitions */ 00844 #define SCB_CSSELR_LEVEL_Pos 1U /*!< SCB CSSELR: Level Position */ 00845 #define SCB_CSSELR_LEVEL_Msk (7UL << SCB_CSSELR_LEVEL_Pos) /*!< SCB CSSELR: Level Mask */ 00846 00847 #define SCB_CSSELR_IND_Pos 0U /*!< SCB CSSELR: InD Position */ 00848 #define SCB_CSSELR_IND_Msk (1UL /*<< SCB_CSSELR_IND_Pos*/) /*!< SCB CSSELR: InD Mask */ 00849 00850 /* SCB Software Triggered Interrupt Register Definitions */ 00851 #define SCB_STIR_INTID_Pos 0U /*!< SCB STIR: INTID Position */ 00852 #define SCB_STIR_INTID_Msk (0x1FFUL /*<< SCB_STIR_INTID_Pos*/) /*!< SCB STIR: INTID Mask */ 00853 00854 /* SCB D-Cache Invalidate by Set-way Register Definitions */ 00855 #define SCB_DCISW_WAY_Pos 30U /*!< SCB DCISW: Way Position */ 00856 #define SCB_DCISW_WAY_Msk (3UL << SCB_DCISW_WAY_Pos) /*!< SCB DCISW: Way Mask */ 00857 00858 #define SCB_DCISW_SET_Pos 5U /*!< SCB DCISW: Set Position */ 00859 #define SCB_DCISW_SET_Msk (0x1FFUL << SCB_DCISW_SET_Pos) /*!< SCB DCISW: Set Mask */ 00860 00861 /* SCB D-Cache Clean by Set-way Register Definitions */ 00862 #define SCB_DCCSW_WAY_Pos 30U /*!< SCB DCCSW: Way Position */ 00863 #define SCB_DCCSW_WAY_Msk (3UL << SCB_DCCSW_WAY_Pos) /*!< SCB DCCSW: Way Mask */ 00864 00865 #define SCB_DCCSW_SET_Pos 5U /*!< SCB DCCSW: Set Position */ 00866 #define SCB_DCCSW_SET_Msk (0x1FFUL << SCB_DCCSW_SET_Pos) /*!< SCB DCCSW: Set Mask */ 00867 00868 /* SCB D-Cache Clean and Invalidate by Set-way Register Definitions */ 00869 #define SCB_DCCISW_WAY_Pos 30U /*!< SCB DCCISW: Way Position */ 00870 #define SCB_DCCISW_WAY_Msk (3UL << SCB_DCCISW_WAY_Pos) /*!< SCB DCCISW: Way Mask */ 00871 00872 #define SCB_DCCISW_SET_Pos 5U /*!< SCB DCCISW: Set Position */ 00873 #define SCB_DCCISW_SET_Msk (0x1FFUL << SCB_DCCISW_SET_Pos) /*!< SCB DCCISW: Set Mask */ 00874 00875 /* Instruction Tightly-Coupled Memory Control Register Definitions */ 00876 #define SCB_ITCMCR_SZ_Pos 3U /*!< SCB ITCMCR: SZ Position */ 00877 #define SCB_ITCMCR_SZ_Msk (0xFUL << SCB_ITCMCR_SZ_Pos) /*!< SCB ITCMCR: SZ Mask */ 00878 00879 #define SCB_ITCMCR_RETEN_Pos 2U /*!< SCB ITCMCR: RETEN Position */ 00880 #define SCB_ITCMCR_RETEN_Msk (1UL << SCB_ITCMCR_RETEN_Pos) /*!< SCB ITCMCR: RETEN Mask */ 00881 00882 #define SCB_ITCMCR_RMW_Pos 1U /*!< SCB ITCMCR: RMW Position */ 00883 #define SCB_ITCMCR_RMW_Msk (1UL << SCB_ITCMCR_RMW_Pos) /*!< SCB ITCMCR: RMW Mask */ 00884 00885 #define SCB_ITCMCR_EN_Pos 0U /*!< SCB ITCMCR: EN Position */ 00886 #define SCB_ITCMCR_EN_Msk (1UL /*<< SCB_ITCMCR_EN_Pos*/) /*!< SCB ITCMCR: EN Mask */ 00887 00888 /* Data Tightly-Coupled Memory Control Register Definitions */ 00889 #define SCB_DTCMCR_SZ_Pos 3U /*!< SCB DTCMCR: SZ Position */ 00890 #define SCB_DTCMCR_SZ_Msk (0xFUL << SCB_DTCMCR_SZ_Pos) /*!< SCB DTCMCR: SZ Mask */ 00891 00892 #define SCB_DTCMCR_RETEN_Pos 2U /*!< SCB DTCMCR: RETEN Position */ 00893 #define SCB_DTCMCR_RETEN_Msk (1UL << SCB_DTCMCR_RETEN_Pos) /*!< SCB DTCMCR: RETEN Mask */ 00894 00895 #define SCB_DTCMCR_RMW_Pos 1U /*!< SCB DTCMCR: RMW Position */ 00896 #define SCB_DTCMCR_RMW_Msk (1UL << SCB_DTCMCR_RMW_Pos) /*!< SCB DTCMCR: RMW Mask */ 00897 00898 #define SCB_DTCMCR_EN_Pos 0U /*!< SCB DTCMCR: EN Position */ 00899 #define SCB_DTCMCR_EN_Msk (1UL /*<< SCB_DTCMCR_EN_Pos*/) /*!< SCB DTCMCR: EN Mask */ 00900 00901 /* AHBP Control Register Definitions */ 00902 #define SCB_AHBPCR_SZ_Pos 1U /*!< SCB AHBPCR: SZ Position */ 00903 #define SCB_AHBPCR_SZ_Msk (7UL << SCB_AHBPCR_SZ_Pos) /*!< SCB AHBPCR: SZ Mask */ 00904 00905 #define SCB_AHBPCR_EN_Pos 0U /*!< SCB AHBPCR: EN Position */ 00906 #define SCB_AHBPCR_EN_Msk (1UL /*<< SCB_AHBPCR_EN_Pos*/) /*!< SCB AHBPCR: EN Mask */ 00907 00908 /* L1 Cache Control Register Definitions */ 00909 #define SCB_CACR_FORCEWT_Pos 2U /*!< SCB CACR: FORCEWT Position */ 00910 #define SCB_CACR_FORCEWT_Msk (1UL << SCB_CACR_FORCEWT_Pos) /*!< SCB CACR: FORCEWT Mask */ 00911 00912 #define SCB_CACR_ECCEN_Pos 1U /*!< SCB CACR: ECCEN Position */ 00913 #define SCB_CACR_ECCEN_Msk (1UL << SCB_CACR_ECCEN_Pos) /*!< SCB CACR: ECCEN Mask */ 00914 00915 #define SCB_CACR_SIWT_Pos 0U /*!< SCB CACR: SIWT Position */ 00916 #define SCB_CACR_SIWT_Msk (1UL /*<< SCB_CACR_SIWT_Pos*/) /*!< SCB CACR: SIWT Mask */ 00917 00918 /* AHBS Control Register Definitions */ 00919 #define SCB_AHBSCR_INITCOUNT_Pos 11U /*!< SCB AHBSCR: INITCOUNT Position */ 00920 #define SCB_AHBSCR_INITCOUNT_Msk (0x1FUL << SCB_AHBPCR_INITCOUNT_Pos) /*!< SCB AHBSCR: INITCOUNT Mask */ 00921 00922 #define SCB_AHBSCR_TPRI_Pos 2U /*!< SCB AHBSCR: TPRI Position */ 00923 #define SCB_AHBSCR_TPRI_Msk (0x1FFUL << SCB_AHBPCR_TPRI_Pos) /*!< SCB AHBSCR: TPRI Mask */ 00924 00925 #define SCB_AHBSCR_CTL_Pos 0U /*!< SCB AHBSCR: CTL Position*/ 00926 #define SCB_AHBSCR_CTL_Msk (3UL /*<< SCB_AHBPCR_CTL_Pos*/) /*!< SCB AHBSCR: CTL Mask */ 00927 00928 /* Auxiliary Bus Fault Status Register Definitions */ 00929 #define SCB_ABFSR_AXIMTYPE_Pos 8U /*!< SCB ABFSR: AXIMTYPE Position*/ 00930 #define SCB_ABFSR_AXIMTYPE_Msk (3UL << SCB_ABFSR_AXIMTYPE_Pos) /*!< SCB ABFSR: AXIMTYPE Mask */ 00931 00932 #define SCB_ABFSR_EPPB_Pos 4U /*!< SCB ABFSR: EPPB Position*/ 00933 #define SCB_ABFSR_EPPB_Msk (1UL << SCB_ABFSR_EPPB_Pos) /*!< SCB ABFSR: EPPB Mask */ 00934 00935 #define SCB_ABFSR_AXIM_Pos 3U /*!< SCB ABFSR: AXIM Position*/ 00936 #define SCB_ABFSR_AXIM_Msk (1UL << SCB_ABFSR_AXIM_Pos) /*!< SCB ABFSR: AXIM Mask */ 00937 00938 #define SCB_ABFSR_AHBP_Pos 2U /*!< SCB ABFSR: AHBP Position*/ 00939 #define SCB_ABFSR_AHBP_Msk (1UL << SCB_ABFSR_AHBP_Pos) /*!< SCB ABFSR: AHBP Mask */ 00940 00941 #define SCB_ABFSR_DTCM_Pos 1U /*!< SCB ABFSR: DTCM Position*/ 00942 #define SCB_ABFSR_DTCM_Msk (1UL << SCB_ABFSR_DTCM_Pos) /*!< SCB ABFSR: DTCM Mask */ 00943 00944 #define SCB_ABFSR_ITCM_Pos 0U /*!< SCB ABFSR: ITCM Position*/ 00945 #define SCB_ABFSR_ITCM_Msk (1UL /*<< SCB_ABFSR_ITCM_Pos*/) /*!< SCB ABFSR: ITCM Mask */ 00946 00947 /*@} end of group CMSIS_SCB */ 00948 00949 00950 /** 00951 \ingroup CMSIS_core_register 00952 \defgroup CMSIS_SCnSCB System Controls not in SCB (SCnSCB) 00953 \brief Type definitions for the System Control and ID Register not in the SCB 00954 @{ 00955 */ 00956 00957 /** 00958 \brief Structure type to access the System Control and ID Register not in the SCB. 00959 */ 00960 typedef struct 00961 { 00962 uint32_t RESERVED0[1U]; 00963 __IM uint32_t ICTR; /*!< Offset: 0x004 (R/ ) Interrupt Controller Type Register */ 00964 __IOM uint32_t ACTLR; /*!< Offset: 0x008 (R/W) Auxiliary Control Register */ 00965 __IOM uint32_t CPPWR; /*!< Offset: 0x00C (R/W) Coprocessor Power Control Register */ 00966 } SCnSCB_Type; 00967 00968 /* Interrupt Controller Type Register Definitions */ 00969 #define SCnSCB_ICTR_INTLINESNUM_Pos 0U /*!< ICTR: INTLINESNUM Position */ 00970 #define SCnSCB_ICTR_INTLINESNUM_Msk (0xFUL /*<< SCnSCB_ICTR_INTLINESNUM_Pos*/) /*!< ICTR: INTLINESNUM Mask */ 00971 00972 /*@} end of group CMSIS_SCnotSCB */ 00973 00974 00975 /** 00976 \ingroup CMSIS_core_register 00977 \defgroup CMSIS_SysTick System Tick Timer (SysTick) 00978 \brief Type definitions for the System Timer Registers. 00979 @{ 00980 */ 00981 00982 /** 00983 \brief Structure type to access the System Timer (SysTick). 00984 */ 00985 typedef struct 00986 { 00987 __IOM uint32_t CTRL; /*!< Offset: 0x000 (R/W) SysTick Control and Status Register */ 00988 __IOM uint32_t LOAD; /*!< Offset: 0x004 (R/W) SysTick Reload Value Register */ 00989 __IOM uint32_t VAL; /*!< Offset: 0x008 (R/W) SysTick Current Value Register */ 00990 __IM uint32_t CALIB; /*!< Offset: 0x00C (R/ ) SysTick Calibration Register */ 00991 } SysTick_Type; 00992 00993 /* SysTick Control / Status Register Definitions */ 00994 #define SysTick_CTRL_COUNTFLAG_Pos 16U /*!< SysTick CTRL: COUNTFLAG Position */ 00995 #define SysTick_CTRL_COUNTFLAG_Msk (1UL << SysTick_CTRL_COUNTFLAG_Pos) /*!< SysTick CTRL: COUNTFLAG Mask */ 00996 00997 #define SysTick_CTRL_CLKSOURCE_Pos 2U /*!< SysTick CTRL: CLKSOURCE Position */ 00998 #define SysTick_CTRL_CLKSOURCE_Msk (1UL << SysTick_CTRL_CLKSOURCE_Pos) /*!< SysTick CTRL: CLKSOURCE Mask */ 00999 01000 #define SysTick_CTRL_TICKINT_Pos 1U /*!< SysTick CTRL: TICKINT Position */ 01001 #define SysTick_CTRL_TICKINT_Msk (1UL << SysTick_CTRL_TICKINT_Pos) /*!< SysTick CTRL: TICKINT Mask */ 01002 01003 #define SysTick_CTRL_ENABLE_Pos 0U /*!< SysTick CTRL: ENABLE Position */ 01004 #define SysTick_CTRL_ENABLE_Msk (1UL /*<< SysTick_CTRL_ENABLE_Pos*/) /*!< SysTick CTRL: ENABLE Mask */ 01005 01006 /* SysTick Reload Register Definitions */ 01007 #define SysTick_LOAD_RELOAD_Pos 0U /*!< SysTick LOAD: RELOAD Position */ 01008 #define SysTick_LOAD_RELOAD_Msk (0xFFFFFFUL /*<< SysTick_LOAD_RELOAD_Pos*/) /*!< SysTick LOAD: RELOAD Mask */ 01009 01010 /* SysTick Current Register Definitions */ 01011 #define SysTick_VAL_CURRENT_Pos 0U /*!< SysTick VAL: CURRENT Position */ 01012 #define SysTick_VAL_CURRENT_Msk (0xFFFFFFUL /*<< SysTick_VAL_CURRENT_Pos*/) /*!< SysTick VAL: CURRENT Mask */ 01013 01014 /* SysTick Calibration Register Definitions */ 01015 #define SysTick_CALIB_NOREF_Pos 31U /*!< SysTick CALIB: NOREF Position */ 01016 #define SysTick_CALIB_NOREF_Msk (1UL << SysTick_CALIB_NOREF_Pos) /*!< SysTick CALIB: NOREF Mask */ 01017 01018 #define SysTick_CALIB_SKEW_Pos 30U /*!< SysTick CALIB: SKEW Position */ 01019 #define SysTick_CALIB_SKEW_Msk (1UL << SysTick_CALIB_SKEW_Pos) /*!< SysTick CALIB: SKEW Mask */ 01020 01021 #define SysTick_CALIB_TENMS_Pos 0U /*!< SysTick CALIB: TENMS Position */ 01022 #define SysTick_CALIB_TENMS_Msk (0xFFFFFFUL /*<< SysTick_CALIB_TENMS_Pos*/) /*!< SysTick CALIB: TENMS Mask */ 01023 01024 /*@} end of group CMSIS_SysTick */ 01025 01026 01027 /** 01028 \ingroup CMSIS_core_register 01029 \defgroup CMSIS_ITM Instrumentation Trace Macrocell (ITM) 01030 \brief Type definitions for the Instrumentation Trace Macrocell (ITM) 01031 @{ 01032 */ 01033 01034 /** 01035 \brief Structure type to access the Instrumentation Trace Macrocell Register (ITM). 01036 */ 01037 typedef struct 01038 { 01039 __OM union 01040 { 01041 __OM uint8_t u8; /*!< Offset: 0x000 ( /W) ITM Stimulus Port 8-bit */ 01042 __OM uint16_t u16; /*!< Offset: 0x000 ( /W) ITM Stimulus Port 16-bit */ 01043 __OM uint32_t u32; /*!< Offset: 0x000 ( /W) ITM Stimulus Port 32-bit */ 01044 } PORT [32U]; /*!< Offset: 0x000 ( /W) ITM Stimulus Port Registers */ 01045 uint32_t RESERVED0[864U]; 01046 __IOM uint32_t TER; /*!< Offset: 0xE00 (R/W) ITM Trace Enable Register */ 01047 uint32_t RESERVED1[15U]; 01048 __IOM uint32_t TPR; /*!< Offset: 0xE40 (R/W) ITM Trace Privilege Register */ 01049 uint32_t RESERVED2[15U]; 01050 __IOM uint32_t TCR; /*!< Offset: 0xE80 (R/W) ITM Trace Control Register */ 01051 uint32_t RESERVED3[29U]; 01052 __OM uint32_t IWR; /*!< Offset: 0xEF8 ( /W) ITM Integration Write Register */ 01053 __IM uint32_t IRR; /*!< Offset: 0xEFC (R/ ) ITM Integration Read Register */ 01054 __IOM uint32_t IMCR; /*!< Offset: 0xF00 (R/W) ITM Integration Mode Control Register */ 01055 uint32_t RESERVED4[43U]; 01056 __OM uint32_t LAR; /*!< Offset: 0xFB0 ( /W) ITM Lock Access Register */ 01057 __IM uint32_t LSR; /*!< Offset: 0xFB4 (R/ ) ITM Lock Status Register */ 01058 uint32_t RESERVED5[1U]; 01059 __IM uint32_t DEVARCH; /*!< Offset: 0xFBC (R/ ) ITM Device Architecture Register */ 01060 uint32_t RESERVED6[4U]; 01061 __IM uint32_t PID4; /*!< Offset: 0xFD0 (R/ ) ITM Peripheral Identification Register #4 */ 01062 __IM uint32_t PID5; /*!< Offset: 0xFD4 (R/ ) ITM Peripheral Identification Register #5 */ 01063 __IM uint32_t PID6; /*!< Offset: 0xFD8 (R/ ) ITM Peripheral Identification Register #6 */ 01064 __IM uint32_t PID7; /*!< Offset: 0xFDC (R/ ) ITM Peripheral Identification Register #7 */ 01065 __IM uint32_t PID0; /*!< Offset: 0xFE0 (R/ ) ITM Peripheral Identification Register #0 */ 01066 __IM uint32_t PID1; /*!< Offset: 0xFE4 (R/ ) ITM Peripheral Identification Register #1 */ 01067 __IM uint32_t PID2; /*!< Offset: 0xFE8 (R/ ) ITM Peripheral Identification Register #2 */ 01068 __IM uint32_t PID3; /*!< Offset: 0xFEC (R/ ) ITM Peripheral Identification Register #3 */ 01069 __IM uint32_t CID0; /*!< Offset: 0xFF0 (R/ ) ITM Component Identification Register #0 */ 01070 __IM uint32_t CID1; /*!< Offset: 0xFF4 (R/ ) ITM Component Identification Register #1 */ 01071 __IM uint32_t CID2; /*!< Offset: 0xFF8 (R/ ) ITM Component Identification Register #2 */ 01072 __IM uint32_t CID3; /*!< Offset: 0xFFC (R/ ) ITM Component Identification Register #3 */ 01073 } ITM_Type; 01074 01075 /* ITM Stimulus Port Register Definitions */ 01076 #define ITM_STIM_DISABLED_Pos 1U /*!< ITM STIM: DISABLED Position */ 01077 #define ITM_STIM_DISABLED_Msk (0x1UL << ITM_STIM_DISABLED_Pos) /*!< ITM STIM: DISABLED Mask */ 01078 01079 #define ITM_STIM_FIFOREADY_Pos 0U /*!< ITM STIM: FIFOREADY Position */ 01080 #define ITM_STIM_FIFOREADY_Msk (0x1UL /*<< ITM_STIM_FIFOREADY_Pos*/) /*!< ITM STIM: FIFOREADY Mask */ 01081 01082 /* ITM Trace Privilege Register Definitions */ 01083 #define ITM_TPR_PRIVMASK_Pos 0U /*!< ITM TPR: PRIVMASK Position */ 01084 #define ITM_TPR_PRIVMASK_Msk (0xFUL /*<< ITM_TPR_PRIVMASK_Pos*/) /*!< ITM TPR: PRIVMASK Mask */ 01085 01086 /* ITM Trace Control Register Definitions */ 01087 #define ITM_TCR_BUSY_Pos 23U /*!< ITM TCR: BUSY Position */ 01088 #define ITM_TCR_BUSY_Msk (1UL << ITM_TCR_BUSY_Pos) /*!< ITM TCR: BUSY Mask */ 01089 01090 #define ITM_TCR_TRACEBUSID_Pos 16U /*!< ITM TCR: ATBID Position */ 01091 #define ITM_TCR_TRACEBUSID_Msk (0x7FUL << ITM_TCR_TRACEBUSID_Pos) /*!< ITM TCR: ATBID Mask */ 01092 01093 #define ITM_TCR_GTSFREQ_Pos 10U /*!< ITM TCR: Global timestamp frequency Position */ 01094 #define ITM_TCR_GTSFREQ_Msk (3UL << ITM_TCR_GTSFREQ_Pos) /*!< ITM TCR: Global timestamp frequency Mask */ 01095 01096 #define ITM_TCR_TSPRESCALE_Pos 8U /*!< ITM TCR: TSPRESCALE Position */ 01097 #define ITM_TCR_TSPRESCALE_Msk (3UL << ITM_TCR_TSPRESCALE_Pos) /*!< ITM TCR: TSPRESCALE Mask */ 01098 01099 #define ITM_TCR_STALLENA_Pos 5U /*!< ITM TCR: STALLENA Position */ 01100 #define ITM_TCR_STALLENA_Msk (1UL << ITM_TCR_STALLENA_Pos) /*!< ITM TCR: STALLENA Mask */ 01101 01102 #define ITM_TCR_SWOENA_Pos 4U /*!< ITM TCR: SWOENA Position */ 01103 #define ITM_TCR_SWOENA_Msk (1UL << ITM_TCR_SWOENA_Pos) /*!< ITM TCR: SWOENA Mask */ 01104 01105 #define ITM_TCR_DWTENA_Pos 3U /*!< ITM TCR: DWTENA Position */ 01106 #define ITM_TCR_DWTENA_Msk (1UL << ITM_TCR_DWTENA_Pos) /*!< ITM TCR: DWTENA Mask */ 01107 01108 #define ITM_TCR_SYNCENA_Pos 2U /*!< ITM TCR: SYNCENA Position */ 01109 #define ITM_TCR_SYNCENA_Msk (1UL << ITM_TCR_SYNCENA_Pos) /*!< ITM TCR: SYNCENA Mask */ 01110 01111 #define ITM_TCR_TSENA_Pos 1U /*!< ITM TCR: TSENA Position */ 01112 #define ITM_TCR_TSENA_Msk (1UL << ITM_TCR_TSENA_Pos) /*!< ITM TCR: TSENA Mask */ 01113 01114 #define ITM_TCR_ITMENA_Pos 0U /*!< ITM TCR: ITM Enable bit Position */ 01115 #define ITM_TCR_ITMENA_Msk (1UL /*<< ITM_TCR_ITMENA_Pos*/) /*!< ITM TCR: ITM Enable bit Mask */ 01116 01117 /* ITM Integration Write Register Definitions */ 01118 #define ITM_IWR_ATVALIDM_Pos 0U /*!< ITM IWR: ATVALIDM Position */ 01119 #define ITM_IWR_ATVALIDM_Msk (1UL /*<< ITM_IWR_ATVALIDM_Pos*/) /*!< ITM IWR: ATVALIDM Mask */ 01120 01121 /* ITM Integration Read Register Definitions */ 01122 #define ITM_IRR_ATREADYM_Pos 0U /*!< ITM IRR: ATREADYM Position */ 01123 #define ITM_IRR_ATREADYM_Msk (1UL /*<< ITM_IRR_ATREADYM_Pos*/) /*!< ITM IRR: ATREADYM Mask */ 01124 01125 /* ITM Integration Mode Control Register Definitions */ 01126 #define ITM_IMCR_INTEGRATION_Pos 0U /*!< ITM IMCR: INTEGRATION Position */ 01127 #define ITM_IMCR_INTEGRATION_Msk (1UL /*<< ITM_IMCR_INTEGRATION_Pos*/) /*!< ITM IMCR: INTEGRATION Mask */ 01128 01129 /* ITM Lock Status Register Definitions */ 01130 #define ITM_LSR_ByteAcc_Pos 2U /*!< ITM LSR: ByteAcc Position */ 01131 #define ITM_LSR_ByteAcc_Msk (1UL << ITM_LSR_ByteAcc_Pos) /*!< ITM LSR: ByteAcc Mask */ 01132 01133 #define ITM_LSR_Access_Pos 1U /*!< ITM LSR: Access Position */ 01134 #define ITM_LSR_Access_Msk (1UL << ITM_LSR_Access_Pos) /*!< ITM LSR: Access Mask */ 01135 01136 #define ITM_LSR_Present_Pos 0U /*!< ITM LSR: Present Position */ 01137 #define ITM_LSR_Present_Msk (1UL /*<< ITM_LSR_Present_Pos*/) /*!< ITM LSR: Present Mask */ 01138 01139 /*@}*/ /* end of group CMSIS_ITM */ 01140 01141 01142 /** 01143 \ingroup CMSIS_core_register 01144 \defgroup CMSIS_DWT Data Watchpoint and Trace (DWT) 01145 \brief Type definitions for the Data Watchpoint and Trace (DWT) 01146 @{ 01147 */ 01148 01149 /** 01150 \brief Structure type to access the Data Watchpoint and Trace Register (DWT). 01151 */ 01152 typedef struct 01153 { 01154 __IOM uint32_t CTRL; /*!< Offset: 0x000 (R/W) Control Register */ 01155 __IOM uint32_t CYCCNT; /*!< Offset: 0x004 (R/W) Cycle Count Register */ 01156 __IOM uint32_t CPICNT; /*!< Offset: 0x008 (R/W) CPI Count Register */ 01157 __IOM uint32_t EXCCNT; /*!< Offset: 0x00C (R/W) Exception Overhead Count Register */ 01158 __IOM uint32_t SLEEPCNT; /*!< Offset: 0x010 (R/W) Sleep Count Register */ 01159 __IOM uint32_t LSUCNT; /*!< Offset: 0x014 (R/W) LSU Count Register */ 01160 __IOM uint32_t FOLDCNT; /*!< Offset: 0x018 (R/W) Folded-instruction Count Register */ 01161 __IM uint32_t PCSR; /*!< Offset: 0x01C (R/ ) Program Counter Sample Register */ 01162 __IOM uint32_t COMP0; /*!< Offset: 0x020 (R/W) Comparator Register 0 */ 01163 uint32_t RESERVED1[1U]; 01164 __IOM uint32_t FUNCTION0; /*!< Offset: 0x028 (R/W) Function Register 0 */ 01165 uint32_t RESERVED2[1U]; 01166 __IOM uint32_t COMP1; /*!< Offset: 0x030 (R/W) Comparator Register 1 */ 01167 uint32_t RESERVED3[1U]; 01168 __IOM uint32_t FUNCTION1; /*!< Offset: 0x038 (R/W) Function Register 1 */ 01169 uint32_t RESERVED4[1U]; 01170 __IOM uint32_t COMP2; /*!< Offset: 0x040 (R/W) Comparator Register 2 */ 01171 uint32_t RESERVED5[1U]; 01172 __IOM uint32_t FUNCTION2; /*!< Offset: 0x048 (R/W) Function Register 2 */ 01173 uint32_t RESERVED6[1U]; 01174 __IOM uint32_t COMP3; /*!< Offset: 0x050 (R/W) Comparator Register 3 */ 01175 uint32_t RESERVED7[1U]; 01176 __IOM uint32_t FUNCTION3; /*!< Offset: 0x058 (R/W) Function Register 3 */ 01177 uint32_t RESERVED8[1U]; 01178 __IOM uint32_t COMP4; /*!< Offset: 0x060 (R/W) Comparator Register 4 */ 01179 uint32_t RESERVED9[1U]; 01180 __IOM uint32_t FUNCTION4; /*!< Offset: 0x068 (R/W) Function Register 4 */ 01181 uint32_t RESERVED10[1U]; 01182 __IOM uint32_t COMP5; /*!< Offset: 0x070 (R/W) Comparator Register 5 */ 01183 uint32_t RESERVED11[1U]; 01184 __IOM uint32_t FUNCTION5; /*!< Offset: 0x078 (R/W) Function Register 5 */ 01185 uint32_t RESERVED12[1U]; 01186 __IOM uint32_t COMP6; /*!< Offset: 0x080 (R/W) Comparator Register 6 */ 01187 uint32_t RESERVED13[1U]; 01188 __IOM uint32_t FUNCTION6; /*!< Offset: 0x088 (R/W) Function Register 6 */ 01189 uint32_t RESERVED14[1U]; 01190 __IOM uint32_t COMP7; /*!< Offset: 0x090 (R/W) Comparator Register 7 */ 01191 uint32_t RESERVED15[1U]; 01192 __IOM uint32_t FUNCTION7; /*!< Offset: 0x098 (R/W) Function Register 7 */ 01193 uint32_t RESERVED16[1U]; 01194 __IOM uint32_t COMP8; /*!< Offset: 0x0A0 (R/W) Comparator Register 8 */ 01195 uint32_t RESERVED17[1U]; 01196 __IOM uint32_t FUNCTION8; /*!< Offset: 0x0A8 (R/W) Function Register 8 */ 01197 uint32_t RESERVED18[1U]; 01198 __IOM uint32_t COMP9; /*!< Offset: 0x0B0 (R/W) Comparator Register 9 */ 01199 uint32_t RESERVED19[1U]; 01200 __IOM uint32_t FUNCTION9; /*!< Offset: 0x0B8 (R/W) Function Register 9 */ 01201 uint32_t RESERVED20[1U]; 01202 __IOM uint32_t COMP10; /*!< Offset: 0x0C0 (R/W) Comparator Register 10 */ 01203 uint32_t RESERVED21[1U]; 01204 __IOM uint32_t FUNCTION10; /*!< Offset: 0x0C8 (R/W) Function Register 10 */ 01205 uint32_t RESERVED22[1U]; 01206 __IOM uint32_t COMP11; /*!< Offset: 0x0D0 (R/W) Comparator Register 11 */ 01207 uint32_t RESERVED23[1U]; 01208 __IOM uint32_t FUNCTION11; /*!< Offset: 0x0D8 (R/W) Function Register 11 */ 01209 uint32_t RESERVED24[1U]; 01210 __IOM uint32_t COMP12; /*!< Offset: 0x0E0 (R/W) Comparator Register 12 */ 01211 uint32_t RESERVED25[1U]; 01212 __IOM uint32_t FUNCTION12; /*!< Offset: 0x0E8 (R/W) Function Register 12 */ 01213 uint32_t RESERVED26[1U]; 01214 __IOM uint32_t COMP13; /*!< Offset: 0x0F0 (R/W) Comparator Register 13 */ 01215 uint32_t RESERVED27[1U]; 01216 __IOM uint32_t FUNCTION13; /*!< Offset: 0x0F8 (R/W) Function Register 13 */ 01217 uint32_t RESERVED28[1U]; 01218 __IOM uint32_t COMP14; /*!< Offset: 0x100 (R/W) Comparator Register 14 */ 01219 uint32_t RESERVED29[1U]; 01220 __IOM uint32_t FUNCTION14; /*!< Offset: 0x108 (R/W) Function Register 14 */ 01221 uint32_t RESERVED30[1U]; 01222 __IOM uint32_t COMP15; /*!< Offset: 0x110 (R/W) Comparator Register 15 */ 01223 uint32_t RESERVED31[1U]; 01224 __IOM uint32_t FUNCTION15; /*!< Offset: 0x118 (R/W) Function Register 15 */ 01225 uint32_t RESERVED32[934U]; 01226 __IM uint32_t LSR; /*!< Offset: 0xFB4 (R ) Lock Status Register */ 01227 uint32_t RESERVED33[1U]; 01228 __IM uint32_t DEVARCH; /*!< Offset: 0xFBC (R/ ) Device Architecture Register */ 01229 } DWT_Type; 01230 01231 /* DWT Control Register Definitions */ 01232 #define DWT_CTRL_NUMCOMP_Pos 28U /*!< DWT CTRL: NUMCOMP Position */ 01233 #define DWT_CTRL_NUMCOMP_Msk (0xFUL << DWT_CTRL_NUMCOMP_Pos) /*!< DWT CTRL: NUMCOMP Mask */ 01234 01235 #define DWT_CTRL_NOTRCPKT_Pos 27U /*!< DWT CTRL: NOTRCPKT Position */ 01236 #define DWT_CTRL_NOTRCPKT_Msk (0x1UL << DWT_CTRL_NOTRCPKT_Pos) /*!< DWT CTRL: NOTRCPKT Mask */ 01237 01238 #define DWT_CTRL_NOEXTTRIG_Pos 26U /*!< DWT CTRL: NOEXTTRIG Position */ 01239 #define DWT_CTRL_NOEXTTRIG_Msk (0x1UL << DWT_CTRL_NOEXTTRIG_Pos) /*!< DWT CTRL: NOEXTTRIG Mask */ 01240 01241 #define DWT_CTRL_NOCYCCNT_Pos 25U /*!< DWT CTRL: NOCYCCNT Position */ 01242 #define DWT_CTRL_NOCYCCNT_Msk (0x1UL << DWT_CTRL_NOCYCCNT_Pos) /*!< DWT CTRL: NOCYCCNT Mask */ 01243 01244 #define DWT_CTRL_NOPRFCNT_Pos 24U /*!< DWT CTRL: NOPRFCNT Position */ 01245 #define DWT_CTRL_NOPRFCNT_Msk (0x1UL << DWT_CTRL_NOPRFCNT_Pos) /*!< DWT CTRL: NOPRFCNT Mask */ 01246 01247 #define DWT_CTRL_CYCDISS_Pos 23U /*!< DWT CTRL: CYCDISS Position */ 01248 #define DWT_CTRL_CYCDISS_Msk (0x1UL << DWT_CTRL_CYCDISS_Pos) /*!< DWT CTRL: CYCDISS Mask */ 01249 01250 #define DWT_CTRL_CYCEVTENA_Pos 22U /*!< DWT CTRL: CYCEVTENA Position */ 01251 #define DWT_CTRL_CYCEVTENA_Msk (0x1UL << DWT_CTRL_CYCEVTENA_Pos) /*!< DWT CTRL: CYCEVTENA Mask */ 01252 01253 #define DWT_CTRL_FOLDEVTENA_Pos 21U /*!< DWT CTRL: FOLDEVTENA Position */ 01254 #define DWT_CTRL_FOLDEVTENA_Msk (0x1UL << DWT_CTRL_FOLDEVTENA_Pos) /*!< DWT CTRL: FOLDEVTENA Mask */ 01255 01256 #define DWT_CTRL_LSUEVTENA_Pos 20U /*!< DWT CTRL: LSUEVTENA Position */ 01257 #define DWT_CTRL_LSUEVTENA_Msk (0x1UL << DWT_CTRL_LSUEVTENA_Pos) /*!< DWT CTRL: LSUEVTENA Mask */ 01258 01259 #define DWT_CTRL_SLEEPEVTENA_Pos 19U /*!< DWT CTRL: SLEEPEVTENA Position */ 01260 #define DWT_CTRL_SLEEPEVTENA_Msk (0x1UL << DWT_CTRL_SLEEPEVTENA_Pos) /*!< DWT CTRL: SLEEPEVTENA Mask */ 01261 01262 #define DWT_CTRL_EXCEVTENA_Pos 18U /*!< DWT CTRL: EXCEVTENA Position */ 01263 #define DWT_CTRL_EXCEVTENA_Msk (0x1UL << DWT_CTRL_EXCEVTENA_Pos) /*!< DWT CTRL: EXCEVTENA Mask */ 01264 01265 #define DWT_CTRL_CPIEVTENA_Pos 17U /*!< DWT CTRL: CPIEVTENA Position */ 01266 #define DWT_CTRL_CPIEVTENA_Msk (0x1UL << DWT_CTRL_CPIEVTENA_Pos) /*!< DWT CTRL: CPIEVTENA Mask */ 01267 01268 #define DWT_CTRL_EXCTRCENA_Pos 16U /*!< DWT CTRL: EXCTRCENA Position */ 01269 #define DWT_CTRL_EXCTRCENA_Msk (0x1UL << DWT_CTRL_EXCTRCENA_Pos) /*!< DWT CTRL: EXCTRCENA Mask */ 01270 01271 #define DWT_CTRL_PCSAMPLENA_Pos 12U /*!< DWT CTRL: PCSAMPLENA Position */ 01272 #define DWT_CTRL_PCSAMPLENA_Msk (0x1UL << DWT_CTRL_PCSAMPLENA_Pos) /*!< DWT CTRL: PCSAMPLENA Mask */ 01273 01274 #define DWT_CTRL_SYNCTAP_Pos 10U /*!< DWT CTRL: SYNCTAP Position */ 01275 #define DWT_CTRL_SYNCTAP_Msk (0x3UL << DWT_CTRL_SYNCTAP_Pos) /*!< DWT CTRL: SYNCTAP Mask */ 01276 01277 #define DWT_CTRL_CYCTAP_Pos 9U /*!< DWT CTRL: CYCTAP Position */ 01278 #define DWT_CTRL_CYCTAP_Msk (0x1UL << DWT_CTRL_CYCTAP_Pos) /*!< DWT CTRL: CYCTAP Mask */ 01279 01280 #define DWT_CTRL_POSTINIT_Pos 5U /*!< DWT CTRL: POSTINIT Position */ 01281 #define DWT_CTRL_POSTINIT_Msk (0xFUL << DWT_CTRL_POSTINIT_Pos) /*!< DWT CTRL: POSTINIT Mask */ 01282 01283 #define DWT_CTRL_POSTPRESET_Pos 1U /*!< DWT CTRL: POSTPRESET Position */ 01284 #define DWT_CTRL_POSTPRESET_Msk (0xFUL << DWT_CTRL_POSTPRESET_Pos) /*!< DWT CTRL: POSTPRESET Mask */ 01285 01286 #define DWT_CTRL_CYCCNTENA_Pos 0U /*!< DWT CTRL: CYCCNTENA Position */ 01287 #define DWT_CTRL_CYCCNTENA_Msk (0x1UL /*<< DWT_CTRL_CYCCNTENA_Pos*/) /*!< DWT CTRL: CYCCNTENA Mask */ 01288 01289 /* DWT CPI Count Register Definitions */ 01290 #define DWT_CPICNT_CPICNT_Pos 0U /*!< DWT CPICNT: CPICNT Position */ 01291 #define DWT_CPICNT_CPICNT_Msk (0xFFUL /*<< DWT_CPICNT_CPICNT_Pos*/) /*!< DWT CPICNT: CPICNT Mask */ 01292 01293 /* DWT Exception Overhead Count Register Definitions */ 01294 #define DWT_EXCCNT_EXCCNT_Pos 0U /*!< DWT EXCCNT: EXCCNT Position */ 01295 #define DWT_EXCCNT_EXCCNT_Msk (0xFFUL /*<< DWT_EXCCNT_EXCCNT_Pos*/) /*!< DWT EXCCNT: EXCCNT Mask */ 01296 01297 /* DWT Sleep Count Register Definitions */ 01298 #define DWT_SLEEPCNT_SLEEPCNT_Pos 0U /*!< DWT SLEEPCNT: SLEEPCNT Position */ 01299 #define DWT_SLEEPCNT_SLEEPCNT_Msk (0xFFUL /*<< DWT_SLEEPCNT_SLEEPCNT_Pos*/) /*!< DWT SLEEPCNT: SLEEPCNT Mask */ 01300 01301 /* DWT LSU Count Register Definitions */ 01302 #define DWT_LSUCNT_LSUCNT_Pos 0U /*!< DWT LSUCNT: LSUCNT Position */ 01303 #define DWT_LSUCNT_LSUCNT_Msk (0xFFUL /*<< DWT_LSUCNT_LSUCNT_Pos*/) /*!< DWT LSUCNT: LSUCNT Mask */ 01304 01305 /* DWT Folded-instruction Count Register Definitions */ 01306 #define DWT_FOLDCNT_FOLDCNT_Pos 0U /*!< DWT FOLDCNT: FOLDCNT Position */ 01307 #define DWT_FOLDCNT_FOLDCNT_Msk (0xFFUL /*<< DWT_FOLDCNT_FOLDCNT_Pos*/) /*!< DWT FOLDCNT: FOLDCNT Mask */ 01308 01309 /* DWT Comparator Function Register Definitions */ 01310 #define DWT_FUNCTION_ID_Pos 27U /*!< DWT FUNCTION: ID Position */ 01311 #define DWT_FUNCTION_ID_Msk (0x1FUL << DWT_FUNCTION_ID_Pos) /*!< DWT FUNCTION: ID Mask */ 01312 01313 #define DWT_FUNCTION_MATCHED_Pos 24U /*!< DWT FUNCTION: MATCHED Position */ 01314 #define DWT_FUNCTION_MATCHED_Msk (0x1UL << DWT_FUNCTION_MATCHED_Pos) /*!< DWT FUNCTION: MATCHED Mask */ 01315 01316 #define DWT_FUNCTION_DATAVSIZE_Pos 10U /*!< DWT FUNCTION: DATAVSIZE Position */ 01317 #define DWT_FUNCTION_DATAVSIZE_Msk (0x3UL << DWT_FUNCTION_DATAVSIZE_Pos) /*!< DWT FUNCTION: DATAVSIZE Mask */ 01318 01319 #define DWT_FUNCTION_ACTION_Pos 4U /*!< DWT FUNCTION: ACTION Position */ 01320 #define DWT_FUNCTION_ACTION_Msk (0x1UL << DWT_FUNCTION_ACTION_Pos) /*!< DWT FUNCTION: ACTION Mask */ 01321 01322 #define DWT_FUNCTION_MATCH_Pos 0U /*!< DWT FUNCTION: MATCH Position */ 01323 #define DWT_FUNCTION_MATCH_Msk (0xFUL /*<< DWT_FUNCTION_MATCH_Pos*/) /*!< DWT FUNCTION: MATCH Mask */ 01324 01325 /*@}*/ /* end of group CMSIS_DWT */ 01326 01327 01328 /** 01329 \ingroup CMSIS_core_register 01330 \defgroup CMSIS_TPI Trace Port Interface (TPI) 01331 \brief Type definitions for the Trace Port Interface (TPI) 01332 @{ 01333 */ 01334 01335 /** 01336 \brief Structure type to access the Trace Port Interface Register (TPI). 01337 */ 01338 typedef struct 01339 { 01340 __IOM uint32_t SSPSR; /*!< Offset: 0x000 (R/ ) Supported Parallel Port Size Register */ 01341 __IOM uint32_t CSPSR; /*!< Offset: 0x004 (R/W) Current Parallel Port Size Register */ 01342 uint32_t RESERVED0[2U]; 01343 __IOM uint32_t ACPR; /*!< Offset: 0x010 (R/W) Asynchronous Clock Prescaler Register */ 01344 uint32_t RESERVED1[55U]; 01345 __IOM uint32_t SPPR; /*!< Offset: 0x0F0 (R/W) Selected Pin Protocol Register */ 01346 uint32_t RESERVED2[131U]; 01347 __IM uint32_t FFSR; /*!< Offset: 0x300 (R/ ) Formatter and Flush Status Register */ 01348 __IOM uint32_t FFCR; /*!< Offset: 0x304 (R/W) Formatter and Flush Control Register */ 01349 __IM uint32_t FSCR; /*!< Offset: 0x308 (R/ ) Formatter Synchronization Counter Register */ 01350 uint32_t RESERVED3[759U]; 01351 __IM uint32_t TRIGGER; /*!< Offset: 0xEE8 (R/ ) TRIGGER */ 01352 __IM uint32_t FIFO0; /*!< Offset: 0xEEC (R/ ) Integration ETM Data */ 01353 __IM uint32_t ITATBCTR2; /*!< Offset: 0xEF0 (R/ ) ITATBCTR2 */ 01354 uint32_t RESERVED4[1U]; 01355 __IM uint32_t ITATBCTR0; /*!< Offset: 0xEF8 (R/ ) ITATBCTR0 */ 01356 __IM uint32_t FIFO1; /*!< Offset: 0xEFC (R/ ) Integration ITM Data */ 01357 __IOM uint32_t ITCTRL; /*!< Offset: 0xF00 (R/W) Integration Mode Control */ 01358 uint32_t RESERVED5[39U]; 01359 __IOM uint32_t CLAIMSET; /*!< Offset: 0xFA0 (R/W) Claim tag set */ 01360 __IOM uint32_t CLAIMCLR; /*!< Offset: 0xFA4 (R/W) Claim tag clear */ 01361 uint32_t RESERVED7[8U]; 01362 __IM uint32_t DEVID; /*!< Offset: 0xFC8 (R/ ) TPIU_DEVID */ 01363 __IM uint32_t DEVTYPE; /*!< Offset: 0xFCC (R/ ) TPIU_DEVTYPE */ 01364 } TPI_Type; 01365 01366 /* TPI Asynchronous Clock Prescaler Register Definitions */ 01367 #define TPI_ACPR_PRESCALER_Pos 0U /*!< TPI ACPR: PRESCALER Position */ 01368 #define TPI_ACPR_PRESCALER_Msk (0x1FFFUL /*<< TPI_ACPR_PRESCALER_Pos*/) /*!< TPI ACPR: PRESCALER Mask */ 01369 01370 /* TPI Selected Pin Protocol Register Definitions */ 01371 #define TPI_SPPR_TXMODE_Pos 0U /*!< TPI SPPR: TXMODE Position */ 01372 #define TPI_SPPR_TXMODE_Msk (0x3UL /*<< TPI_SPPR_TXMODE_Pos*/) /*!< TPI SPPR: TXMODE Mask */ 01373 01374 /* TPI Formatter and Flush Status Register Definitions */ 01375 #define TPI_FFSR_FtNonStop_Pos 3U /*!< TPI FFSR: FtNonStop Position */ 01376 #define TPI_FFSR_FtNonStop_Msk (0x1UL << TPI_FFSR_FtNonStop_Pos) /*!< TPI FFSR: FtNonStop Mask */ 01377 01378 #define TPI_FFSR_TCPresent_Pos 2U /*!< TPI FFSR: TCPresent Position */ 01379 #define TPI_FFSR_TCPresent_Msk (0x1UL << TPI_FFSR_TCPresent_Pos) /*!< TPI FFSR: TCPresent Mask */ 01380 01381 #define TPI_FFSR_FtStopped_Pos 1U /*!< TPI FFSR: FtStopped Position */ 01382 #define TPI_FFSR_FtStopped_Msk (0x1UL << TPI_FFSR_FtStopped_Pos) /*!< TPI FFSR: FtStopped Mask */ 01383 01384 #define TPI_FFSR_FlInProg_Pos 0U /*!< TPI FFSR: FlInProg Position */ 01385 #define TPI_FFSR_FlInProg_Msk (0x1UL /*<< TPI_FFSR_FlInProg_Pos*/) /*!< TPI FFSR: FlInProg Mask */ 01386 01387 /* TPI Formatter and Flush Control Register Definitions */ 01388 #define TPI_FFCR_TrigIn_Pos 8U /*!< TPI FFCR: TrigIn Position */ 01389 #define TPI_FFCR_TrigIn_Msk (0x1UL << TPI_FFCR_TrigIn_Pos) /*!< TPI FFCR: TrigIn Mask */ 01390 01391 #define TPI_FFCR_EnFCont_Pos 1U /*!< TPI FFCR: EnFCont Position */ 01392 #define TPI_FFCR_EnFCont_Msk (0x1UL << TPI_FFCR_EnFCont_Pos) /*!< TPI FFCR: EnFCont Mask */ 01393 01394 /* TPI TRIGGER Register Definitions */ 01395 #define TPI_TRIGGER_TRIGGER_Pos 0U /*!< TPI TRIGGER: TRIGGER Position */ 01396 #define TPI_TRIGGER_TRIGGER_Msk (0x1UL /*<< TPI_TRIGGER_TRIGGER_Pos*/) /*!< TPI TRIGGER: TRIGGER Mask */ 01397 01398 /* TPI Integration ETM Data Register Definitions (FIFO0) */ 01399 #define TPI_FIFO0_ITM_ATVALID_Pos 29U /*!< TPI FIFO0: ITM_ATVALID Position */ 01400 #define TPI_FIFO0_ITM_ATVALID_Msk (0x3UL << TPI_FIFO0_ITM_ATVALID_Pos) /*!< TPI FIFO0: ITM_ATVALID Mask */ 01401 01402 #define TPI_FIFO0_ITM_bytecount_Pos 27U /*!< TPI FIFO0: ITM_bytecount Position */ 01403 #define TPI_FIFO0_ITM_bytecount_Msk (0x3UL << TPI_FIFO0_ITM_bytecount_Pos) /*!< TPI FIFO0: ITM_bytecount Mask */ 01404 01405 #define TPI_FIFO0_ETM_ATVALID_Pos 26U /*!< TPI FIFO0: ETM_ATVALID Position */ 01406 #define TPI_FIFO0_ETM_ATVALID_Msk (0x3UL << TPI_FIFO0_ETM_ATVALID_Pos) /*!< TPI FIFO0: ETM_ATVALID Mask */ 01407 01408 #define TPI_FIFO0_ETM_bytecount_Pos 24U /*!< TPI FIFO0: ETM_bytecount Position */ 01409 #define TPI_FIFO0_ETM_bytecount_Msk (0x3UL << TPI_FIFO0_ETM_bytecount_Pos) /*!< TPI FIFO0: ETM_bytecount Mask */ 01410 01411 #define TPI_FIFO0_ETM2_Pos 16U /*!< TPI FIFO0: ETM2 Position */ 01412 #define TPI_FIFO0_ETM2_Msk (0xFFUL << TPI_FIFO0_ETM2_Pos) /*!< TPI FIFO0: ETM2 Mask */ 01413 01414 #define TPI_FIFO0_ETM1_Pos 8U /*!< TPI FIFO0: ETM1 Position */ 01415 #define TPI_FIFO0_ETM1_Msk (0xFFUL << TPI_FIFO0_ETM1_Pos) /*!< TPI FIFO0: ETM1 Mask */ 01416 01417 #define TPI_FIFO0_ETM0_Pos 0U /*!< TPI FIFO0: ETM0 Position */ 01418 #define TPI_FIFO0_ETM0_Msk (0xFFUL /*<< TPI_FIFO0_ETM0_Pos*/) /*!< TPI FIFO0: ETM0 Mask */ 01419 01420 /* TPI ITATBCTR2 Register Definitions */ 01421 #define TPI_ITATBCTR2_ATREADY_Pos 0U /*!< TPI ITATBCTR2: ATREADY Position */ 01422 #define TPI_ITATBCTR2_ATREADY_Msk (0x1UL /*<< TPI_ITATBCTR2_ATREADY_Pos*/) /*!< TPI ITATBCTR2: ATREADY Mask */ 01423 01424 /* TPI Integration ITM Data Register Definitions (FIFO1) */ 01425 #define TPI_FIFO1_ITM_ATVALID_Pos 29U /*!< TPI FIFO1: ITM_ATVALID Position */ 01426 #define TPI_FIFO1_ITM_ATVALID_Msk (0x3UL << TPI_FIFO1_ITM_ATVALID_Pos) /*!< TPI FIFO1: ITM_ATVALID Mask */ 01427 01428 #define TPI_FIFO1_ITM_bytecount_Pos 27U /*!< TPI FIFO1: ITM_bytecount Position */ 01429 #define TPI_FIFO1_ITM_bytecount_Msk (0x3UL << TPI_FIFO1_ITM_bytecount_Pos) /*!< TPI FIFO1: ITM_bytecount Mask */ 01430 01431 #define TPI_FIFO1_ETM_ATVALID_Pos 26U /*!< TPI FIFO1: ETM_ATVALID Position */ 01432 #define TPI_FIFO1_ETM_ATVALID_Msk (0x3UL << TPI_FIFO1_ETM_ATVALID_Pos) /*!< TPI FIFO1: ETM_ATVALID Mask */ 01433 01434 #define TPI_FIFO1_ETM_bytecount_Pos 24U /*!< TPI FIFO1: ETM_bytecount Position */ 01435 #define TPI_FIFO1_ETM_bytecount_Msk (0x3UL << TPI_FIFO1_ETM_bytecount_Pos) /*!< TPI FIFO1: ETM_bytecount Mask */ 01436 01437 #define TPI_FIFO1_ITM2_Pos 16U /*!< TPI FIFO1: ITM2 Position */ 01438 #define TPI_FIFO1_ITM2_Msk (0xFFUL << TPI_FIFO1_ITM2_Pos) /*!< TPI FIFO1: ITM2 Mask */ 01439 01440 #define TPI_FIFO1_ITM1_Pos 8U /*!< TPI FIFO1: ITM1 Position */ 01441 #define TPI_FIFO1_ITM1_Msk (0xFFUL << TPI_FIFO1_ITM1_Pos) /*!< TPI FIFO1: ITM1 Mask */ 01442 01443 #define TPI_FIFO1_ITM0_Pos 0U /*!< TPI FIFO1: ITM0 Position */ 01444 #define TPI_FIFO1_ITM0_Msk (0xFFUL /*<< TPI_FIFO1_ITM0_Pos*/) /*!< TPI FIFO1: ITM0 Mask */ 01445 01446 /* TPI ITATBCTR0 Register Definitions */ 01447 #define TPI_ITATBCTR0_ATREADY_Pos 0U /*!< TPI ITATBCTR0: ATREADY Position */ 01448 #define TPI_ITATBCTR0_ATREADY_Msk (0x1UL /*<< TPI_ITATBCTR0_ATREADY_Pos*/) /*!< TPI ITATBCTR0: ATREADY Mask */ 01449 01450 /* TPI Integration Mode Control Register Definitions */ 01451 #define TPI_ITCTRL_Mode_Pos 0U /*!< TPI ITCTRL: Mode Position */ 01452 #define TPI_ITCTRL_Mode_Msk (0x1UL /*<< TPI_ITCTRL_Mode_Pos*/) /*!< TPI ITCTRL: Mode Mask */ 01453 01454 /* TPI DEVID Register Definitions */ 01455 #define TPI_DEVID_NRZVALID_Pos 11U /*!< TPI DEVID: NRZVALID Position */ 01456 #define TPI_DEVID_NRZVALID_Msk (0x1UL << TPI_DEVID_NRZVALID_Pos) /*!< TPI DEVID: NRZVALID Mask */ 01457 01458 #define TPI_DEVID_MANCVALID_Pos 10U /*!< TPI DEVID: MANCVALID Position */ 01459 #define TPI_DEVID_MANCVALID_Msk (0x1UL << TPI_DEVID_MANCVALID_Pos) /*!< TPI DEVID: MANCVALID Mask */ 01460 01461 #define TPI_DEVID_PTINVALID_Pos 9U /*!< TPI DEVID: PTINVALID Position */ 01462 #define TPI_DEVID_PTINVALID_Msk (0x1UL << TPI_DEVID_PTINVALID_Pos) /*!< TPI DEVID: PTINVALID Mask */ 01463 01464 #define TPI_DEVID_MinBufSz_Pos 6U /*!< TPI DEVID: MinBufSz Position */ 01465 #define TPI_DEVID_MinBufSz_Msk (0x7UL << TPI_DEVID_MinBufSz_Pos) /*!< TPI DEVID: MinBufSz Mask */ 01466 01467 #define TPI_DEVID_AsynClkIn_Pos 5U /*!< TPI DEVID: AsynClkIn Position */ 01468 #define TPI_DEVID_AsynClkIn_Msk (0x1UL << TPI_DEVID_AsynClkIn_Pos) /*!< TPI DEVID: AsynClkIn Mask */ 01469 01470 #define TPI_DEVID_NrTraceInput_Pos 0U /*!< TPI DEVID: NrTraceInput Position */ 01471 #define TPI_DEVID_NrTraceInput_Msk (0x1FUL /*<< TPI_DEVID_NrTraceInput_Pos*/) /*!< TPI DEVID: NrTraceInput Mask */ 01472 01473 /* TPI DEVTYPE Register Definitions */ 01474 #define TPI_DEVTYPE_MajorType_Pos 4U /*!< TPI DEVTYPE: MajorType Position */ 01475 #define TPI_DEVTYPE_MajorType_Msk (0xFUL << TPI_DEVTYPE_MajorType_Pos) /*!< TPI DEVTYPE: MajorType Mask */ 01476 01477 #define TPI_DEVTYPE_SubType_Pos 0U /*!< TPI DEVTYPE: SubType Position */ 01478 #define TPI_DEVTYPE_SubType_Msk (0xFUL /*<< TPI_DEVTYPE_SubType_Pos*/) /*!< TPI DEVTYPE: SubType Mask */ 01479 01480 /*@}*/ /* end of group CMSIS_TPI */ 01481 01482 01483 #if defined (__MPU_PRESENT) && (__MPU_PRESENT == 1U) 01484 /** 01485 \ingroup CMSIS_core_register 01486 \defgroup CMSIS_MPU Memory Protection Unit (MPU) 01487 \brief Type definitions for the Memory Protection Unit (MPU) 01488 @{ 01489 */ 01490 01491 /** 01492 \brief Structure type to access the Memory Protection Unit (MPU). 01493 */ 01494 typedef struct 01495 { 01496 __IM uint32_t TYPE; /*!< Offset: 0x000 (R/ ) MPU Type Register */ 01497 __IOM uint32_t CTRL; /*!< Offset: 0x004 (R/W) MPU Control Register */ 01498 __IOM uint32_t RNR; /*!< Offset: 0x008 (R/W) MPU Region Number Register */ 01499 __IOM uint32_t RBAR; /*!< Offset: 0x00C (R/W) MPU Region Base Address Register */ 01500 __IOM uint32_t RLAR; /*!< Offset: 0x010 (R/W) MPU Region Limit Address Register */ 01501 __IOM uint32_t RBAR_A1; /*!< Offset: 0x014 (R/W) MPU Region Base Address Register Alias 1 */ 01502 __IOM uint32_t RLAR_A1; /*!< Offset: 0x018 (R/W) MPU Region Limit Address Register Alias 1 */ 01503 __IOM uint32_t RBAR_A2; /*!< Offset: 0x01C (R/W) MPU Region Base Address Register Alias 2 */ 01504 __IOM uint32_t RLAR_A2; /*!< Offset: 0x020 (R/W) MPU Region Limit Address Register Alias 2 */ 01505 __IOM uint32_t RBAR_A3; /*!< Offset: 0x024 (R/W) MPU Region Base Address Register Alias 3 */ 01506 __IOM uint32_t RLAR_A3; /*!< Offset: 0x028 (R/W) MPU Region Limit Address Register Alias 3 */ 01507 uint32_t RESERVED0[1]; 01508 __IOM uint32_t MAIR0; /*!< Offset: 0x030 (R/W) MPU Memory Attribute Indirection Register 0 */ 01509 __IOM uint32_t MAIR1; /*!< Offset: 0x034 (R/W) MPU Memory Attribute Indirection Register 1 */ 01510 } MPU_Type; 01511 01512 /* MPU Type Register Definitions */ 01513 #define MPU_TYPE_IREGION_Pos 16U /*!< MPU TYPE: IREGION Position */ 01514 #define MPU_TYPE_IREGION_Msk (0xFFUL << MPU_TYPE_IREGION_Pos) /*!< MPU TYPE: IREGION Mask */ 01515 01516 #define MPU_TYPE_DREGION_Pos 8U /*!< MPU TYPE: DREGION Position */ 01517 #define MPU_TYPE_DREGION_Msk (0xFFUL << MPU_TYPE_DREGION_Pos) /*!< MPU TYPE: DREGION Mask */ 01518 01519 #define MPU_TYPE_SEPARATE_Pos 0U /*!< MPU TYPE: SEPARATE Position */ 01520 #define MPU_TYPE_SEPARATE_Msk (1UL /*<< MPU_TYPE_SEPARATE_Pos*/) /*!< MPU TYPE: SEPARATE Mask */ 01521 01522 /* MPU Control Register Definitions */ 01523 #define MPU_CTRL_PRIVDEFENA_Pos 2U /*!< MPU CTRL: PRIVDEFENA Position */ 01524 #define MPU_CTRL_PRIVDEFENA_Msk (1UL << MPU_CTRL_PRIVDEFENA_Pos) /*!< MPU CTRL: PRIVDEFENA Mask */ 01525 01526 #define MPU_CTRL_HFNMIENA_Pos 1U /*!< MPU CTRL: HFNMIENA Position */ 01527 #define MPU_CTRL_HFNMIENA_Msk (1UL << MPU_CTRL_HFNMIENA_Pos) /*!< MPU CTRL: HFNMIENA Mask */ 01528 01529 #define MPU_CTRL_ENABLE_Pos 0U /*!< MPU CTRL: ENABLE Position */ 01530 #define MPU_CTRL_ENABLE_Msk (1UL /*<< MPU_CTRL_ENABLE_Pos*/) /*!< MPU CTRL: ENABLE Mask */ 01531 01532 /* MPU Region Number Register Definitions */ 01533 #define MPU_RNR_REGION_Pos 0U /*!< MPU RNR: REGION Position */ 01534 #define MPU_RNR_REGION_Msk (0xFFUL /*<< MPU_RNR_REGION_Pos*/) /*!< MPU RNR: REGION Mask */ 01535 01536 /* MPU Region Base Address Register Definitions */ 01537 #define MPU_RBAR_ADDR_Pos 5U /*!< MPU RBAR: ADDR Position */ 01538 #define MPU_RBAR_ADDR_Msk (0x7FFFFFFUL << MPU_RBAR_ADDR_Pos) /*!< MPU RBAR: ADDR Mask */ 01539 01540 #define MPU_RBAR_SH_Pos 3U /*!< MPU RBAR: SH Position */ 01541 #define MPU_RBAR_SH_Msk (0x3UL << MPU_RBAR_SH_Pos) /*!< MPU RBAR: SH Mask */ 01542 01543 #define MPU_RBAR_AP_Pos 1U /*!< MPU RBAR: AP Position */ 01544 #define MPU_RBAR_AP_Msk (0x3UL << MPU_RBAR_AP_Pos) /*!< MPU RBAR: AP Mask */ 01545 01546 #define MPU_RBAR_XN_Pos 0U /*!< MPU RBAR: XN Position */ 01547 #define MPU_RBAR_XN_Msk (01UL /*<< MPU_RBAR_XN_Pos*/) /*!< MPU RBAR: XN Mask */ 01548 01549 /* MPU Region Limit Address Register Definitions */ 01550 #define MPU_RLAR_LIMIT_Pos 5U /*!< MPU RLAR: LIMIT Position */ 01551 #define MPU_RLAR_LIMIT_Msk (0x7FFFFFFUL << MPU_RLAR_LIMIT_Pos) /*!< MPU RLAR: LIMIT Mask */ 01552 01553 #define MPU_RLAR_AttrIndx_Pos 1U /*!< MPU RLAR: AttrIndx Position */ 01554 #define MPU_RLAR_AttrIndx_Msk (0x7UL << MPU_RLAR_AttrIndx_Pos) /*!< MPU RLAR: AttrIndx Mask */ 01555 01556 #define MPU_RLAR_EN_Pos 0U /*!< MPU RLAR: Region enable bit Position */ 01557 #define MPU_RLAR_EN_Msk (1UL /*<< MPU_RLAR_EN_Pos*/) /*!< MPU RLAR: Region enable bit Disable Mask */ 01558 01559 /* MPU Memory Attribute Indirection Register 0 Definitions */ 01560 #define MPU_MAIR0_Attr3_Pos 24U /*!< MPU MAIR0: Attr3 Position */ 01561 #define MPU_MAIR0_Attr3_Msk (0xFFUL << MPU_MAIR0_Attr3_Pos) /*!< MPU MAIR0: Attr3 Mask */ 01562 01563 #define MPU_MAIR0_Attr2_Pos 16U /*!< MPU MAIR0: Attr2 Position */ 01564 #define MPU_MAIR0_Attr2_Msk (0xFFUL << MPU_MAIR0_Attr2_Pos) /*!< MPU MAIR0: Attr2 Mask */ 01565 01566 #define MPU_MAIR0_Attr1_Pos 8U /*!< MPU MAIR0: Attr1 Position */ 01567 #define MPU_MAIR0_Attr1_Msk (0xFFUL << MPU_MAIR0_Attr1_Pos) /*!< MPU MAIR0: Attr1 Mask */ 01568 01569 #define MPU_MAIR0_Attr0_Pos 0U /*!< MPU MAIR0: Attr0 Position */ 01570 #define MPU_MAIR0_Attr0_Msk (0xFFUL /*<< MPU_MAIR0_Attr0_Pos*/) /*!< MPU MAIR0: Attr0 Mask */ 01571 01572 /* MPU Memory Attribute Indirection Register 1 Definitions */ 01573 #define MPU_MAIR1_Attr7_Pos 24U /*!< MPU MAIR1: Attr7 Position */ 01574 #define MPU_MAIR1_Attr7_Msk (0xFFUL << MPU_MAIR1_Attr7_Pos) /*!< MPU MAIR1: Attr7 Mask */ 01575 01576 #define MPU_MAIR1_Attr6_Pos 16U /*!< MPU MAIR1: Attr6 Position */ 01577 #define MPU_MAIR1_Attr6_Msk (0xFFUL << MPU_MAIR1_Attr6_Pos) /*!< MPU MAIR1: Attr6 Mask */ 01578 01579 #define MPU_MAIR1_Attr5_Pos 8U /*!< MPU MAIR1: Attr5 Position */ 01580 #define MPU_MAIR1_Attr5_Msk (0xFFUL << MPU_MAIR1_Attr5_Pos) /*!< MPU MAIR1: Attr5 Mask */ 01581 01582 #define MPU_MAIR1_Attr4_Pos 0U /*!< MPU MAIR1: Attr4 Position */ 01583 #define MPU_MAIR1_Attr4_Msk (0xFFUL /*<< MPU_MAIR1_Attr4_Pos*/) /*!< MPU MAIR1: Attr4 Mask */ 01584 01585 /*@} end of group CMSIS_MPU */ 01586 #endif 01587 01588 01589 #if defined (__ARM_FEATURE_CMSE) && (__ARM_FEATURE_CMSE == 3U) 01590 /** 01591 \ingroup CMSIS_core_register 01592 \defgroup CMSIS_SAU Security Attribution Unit (SAU) 01593 \brief Type definitions for the Security Attribution Unit (SAU) 01594 @{ 01595 */ 01596 01597 /** 01598 \brief Structure type to access the Security Attribution Unit (SAU). 01599 */ 01600 typedef struct 01601 { 01602 __IOM uint32_t CTRL; /*!< Offset: 0x000 (R/W) SAU Control Register */ 01603 __IM uint32_t TYPE; /*!< Offset: 0x004 (R/ ) SAU Type Register */ 01604 #if defined (__SAUREGION_PRESENT) && (__SAUREGION_PRESENT == 1U) 01605 __IOM uint32_t RNR; /*!< Offset: 0x008 (R/W) SAU Region Number Register */ 01606 __IOM uint32_t RBAR; /*!< Offset: 0x00C (R/W) SAU Region Base Address Register */ 01607 __IOM uint32_t RLAR; /*!< Offset: 0x010 (R/W) SAU Region Limit Address Register */ 01608 #else 01609 uint32_t RESERVED0[3]; 01610 #endif 01611 __IOM uint32_t SFSR; /*!< Offset: 0x014 (R/W) Secure Fault Status Register */ 01612 __IOM uint32_t SFAR; /*!< Offset: 0x018 (R/W) Secure Fault Address Register */ 01613 } SAU_Type; 01614 01615 /* SAU Control Register Definitions */ 01616 #define SAU_CTRL_ALLNS_Pos 1U /*!< SAU CTRL: ALLNS Position */ 01617 #define SAU_CTRL_ALLNS_Msk (1UL << SAU_CTRL_ALLNS_Pos) /*!< SAU CTRL: ALLNS Mask */ 01618 01619 #define SAU_CTRL_ENABLE_Pos 0U /*!< SAU CTRL: ENABLE Position */ 01620 #define SAU_CTRL_ENABLE_Msk (1UL /*<< SAU_CTRL_ENABLE_Pos*/) /*!< SAU CTRL: ENABLE Mask */ 01621 01622 /* SAU Type Register Definitions */ 01623 #define SAU_TYPE_SREGION_Pos 0U /*!< SAU TYPE: SREGION Position */ 01624 #define SAU_TYPE_SREGION_Msk (0xFFUL /*<< SAU_TYPE_SREGION_Pos*/) /*!< SAU TYPE: SREGION Mask */ 01625 01626 #if defined (__SAUREGION_PRESENT) && (__SAUREGION_PRESENT == 1U) 01627 /* SAU Region Number Register Definitions */ 01628 #define SAU_RNR_REGION_Pos 0U /*!< SAU RNR: REGION Position */ 01629 #define SAU_RNR_REGION_Msk (0xFFUL /*<< SAU_RNR_REGION_Pos*/) /*!< SAU RNR: REGION Mask */ 01630 01631 /* SAU Region Base Address Register Definitions */ 01632 #define SAU_RBAR_BADDR_Pos 5U /*!< SAU RBAR: BADDR Position */ 01633 #define SAU_RBAR_BADDR_Msk (0x7FFFFFFUL << SAU_RBAR_BADDR_Pos) /*!< SAU RBAR: BADDR Mask */ 01634 01635 /* SAU Region Limit Address Register Definitions */ 01636 #define SAU_RLAR_LADDR_Pos 5U /*!< SAU RLAR: LADDR Position */ 01637 #define SAU_RLAR_LADDR_Msk (0x7FFFFFFUL << SAU_RLAR_LADDR_Pos) /*!< SAU RLAR: LADDR Mask */ 01638 01639 #define SAU_RLAR_NSC_Pos 1U /*!< SAU RLAR: NSC Position */ 01640 #define SAU_RLAR_NSC_Msk (1UL << SAU_RLAR_NSC_Pos) /*!< SAU RLAR: NSC Mask */ 01641 01642 #define SAU_RLAR_ENABLE_Pos 0U /*!< SAU RLAR: ENABLE Position */ 01643 #define SAU_RLAR_ENABLE_Msk (1UL /*<< SAU_RLAR_ENABLE_Pos*/) /*!< SAU RLAR: ENABLE Mask */ 01644 01645 #endif /* defined (__SAUREGION_PRESENT) && (__SAUREGION_PRESENT == 1U) */ 01646 01647 /* Secure Fault Status Register Definitions */ 01648 #define SAU_SFSR_LSERR_Pos 7U /*!< SAU SFSR: LSERR Position */ 01649 #define SAU_SFSR_LSERR_Msk (1UL << SAU_SFSR_LSERR_Pos) /*!< SAU SFSR: LSERR Mask */ 01650 01651 #define SAU_SFSR_SFARVALID_Pos 6U /*!< SAU SFSR: SFARVALID Position */ 01652 #define SAU_SFSR_SFARVALID_Msk (1UL << SAU_SFSR_SFARVALID_Pos) /*!< SAU SFSR: SFARVALID Mask */ 01653 01654 #define SAU_SFSR_LSPERR_Pos 5U /*!< SAU SFSR: LSPERR Position */ 01655 #define SAU_SFSR_LSPERR_Msk (1UL << SAU_SFSR_LSPERR_Pos) /*!< SAU SFSR: LSPERR Mask */ 01656 01657 #define SAU_SFSR_INVTRAN_Pos 4U /*!< SAU SFSR: INVTRAN Position */ 01658 #define SAU_SFSR_INVTRAN_Msk (1UL << SAU_SFSR_INVTRAN_Pos) /*!< SAU SFSR: INVTRAN Mask */ 01659 01660 #define SAU_SFSR_AUVIOL_Pos 3U /*!< SAU SFSR: AUVIOL Position */ 01661 #define SAU_SFSR_AUVIOL_Msk (1UL << SAU_SFSR_AUVIOL_Pos) /*!< SAU SFSR: AUVIOL Mask */ 01662 01663 #define SAU_SFSR_INVER_Pos 2U /*!< SAU SFSR: INVER Position */ 01664 #define SAU_SFSR_INVER_Msk (1UL << SAU_SFSR_INVER_Pos) /*!< SAU SFSR: INVER Mask */ 01665 01666 #define SAU_SFSR_INVIS_Pos 1U /*!< SAU SFSR: INVIS Position */ 01667 #define SAU_SFSR_INVIS_Msk (1UL << SAU_SFSR_INVIS_Pos) /*!< SAU SFSR: INVIS Mask */ 01668 01669 #define SAU_SFSR_INVEP_Pos 0U /*!< SAU SFSR: INVEP Position */ 01670 #define SAU_SFSR_INVEP_Msk (1UL /*<< SAU_SFSR_INVEP_Pos*/) /*!< SAU SFSR: INVEP Mask */ 01671 01672 /*@} end of group CMSIS_SAU */ 01673 #endif /* defined (__ARM_FEATURE_CMSE) && (__ARM_FEATURE_CMSE == 3U) */ 01674 01675 01676 /** 01677 \ingroup CMSIS_core_register 01678 \defgroup CMSIS_FPU Floating Point Unit (FPU) 01679 \brief Type definitions for the Floating Point Unit (FPU) 01680 @{ 01681 */ 01682 01683 /** 01684 \brief Structure type to access the Floating Point Unit (FPU). 01685 */ 01686 typedef struct 01687 { 01688 uint32_t RESERVED0[1U]; 01689 __IOM uint32_t FPCCR; /*!< Offset: 0x004 (R/W) Floating-Point Context Control Register */ 01690 __IOM uint32_t FPCAR; /*!< Offset: 0x008 (R/W) Floating-Point Context Address Register */ 01691 __IOM uint32_t FPDSCR; /*!< Offset: 0x00C (R/W) Floating-Point Default Status Control Register */ 01692 __IM uint32_t MVFR0; /*!< Offset: 0x010 (R/ ) Media and FP Feature Register 0 */ 01693 __IM uint32_t MVFR1; /*!< Offset: 0x014 (R/ ) Media and FP Feature Register 1 */ 01694 } FPU_Type; 01695 01696 /* Floating-Point Context Control Register Definitions */ 01697 #define FPU_FPCCR_ASPEN_Pos 31U /*!< FPCCR: ASPEN bit Position */ 01698 #define FPU_FPCCR_ASPEN_Msk (1UL << FPU_FPCCR_ASPEN_Pos) /*!< FPCCR: ASPEN bit Mask */ 01699 01700 #define FPU_FPCCR_LSPEN_Pos 30U /*!< FPCCR: LSPEN Position */ 01701 #define FPU_FPCCR_LSPEN_Msk (1UL << FPU_FPCCR_LSPEN_Pos) /*!< FPCCR: LSPEN bit Mask */ 01702 01703 #define FPU_FPCCR_LSPENS_Pos 29U /*!< FPCCR: LSPENS Position */ 01704 #define FPU_FPCCR_LSPENS_Msk (1UL << FPU_FPCCR_LSPENS_Pos) /*!< FPCCR: LSPENS bit Mask */ 01705 01706 #define FPU_FPCCR_CLRONRET_Pos 28U /*!< FPCCR: CLRONRET Position */ 01707 #define FPU_FPCCR_CLRONRET_Msk (1UL << FPU_FPCCR_CLRONRET_Pos) /*!< FPCCR: CLRONRET bit Mask */ 01708 01709 #define FPU_FPCCR_CLRONRETS_Pos 27U /*!< FPCCR: CLRONRETS Position */ 01710 #define FPU_FPCCR_CLRONRETS_Msk (1UL << FPU_FPCCR_CLRONRETS_Pos) /*!< FPCCR: CLRONRETS bit Mask */ 01711 01712 #define FPU_FPCCR_TS_Pos 26U /*!< FPCCR: TS Position */ 01713 #define FPU_FPCCR_TS_Msk (1UL << FPU_FPCCR_TS_Pos) /*!< FPCCR: TS bit Mask */ 01714 01715 #define FPU_FPCCR_UFRDY_Pos 10U /*!< FPCCR: UFRDY Position */ 01716 #define FPU_FPCCR_UFRDY_Msk (1UL << FPU_FPCCR_UFRDY_Pos) /*!< FPCCR: UFRDY bit Mask */ 01717 01718 #define FPU_FPCCR_SPLIMVIOL_Pos 9U /*!< FPCCR: SPLIMVIOL Position */ 01719 #define FPU_FPCCR_SPLIMVIOL_Msk (1UL << FPU_FPCCR_SPLIMVIOL_Pos) /*!< FPCCR: SPLIMVIOL bit Mask */ 01720 01721 #define FPU_FPCCR_MONRDY_Pos 8U /*!< FPCCR: MONRDY Position */ 01722 #define FPU_FPCCR_MONRDY_Msk (1UL << FPU_FPCCR_MONRDY_Pos) /*!< FPCCR: MONRDY bit Mask */ 01723 01724 #define FPU_FPCCR_SFRDY_Pos 7U /*!< FPCCR: SFRDY Position */ 01725 #define FPU_FPCCR_SFRDY_Msk (1UL << FPU_FPCCR_SFRDY_Pos) /*!< FPCCR: SFRDY bit Mask */ 01726 01727 #define FPU_FPCCR_BFRDY_Pos 6U /*!< FPCCR: BFRDY Position */ 01728 #define FPU_FPCCR_BFRDY_Msk (1UL << FPU_FPCCR_BFRDY_Pos) /*!< FPCCR: BFRDY bit Mask */ 01729 01730 #define FPU_FPCCR_MMRDY_Pos 5U /*!< FPCCR: MMRDY Position */ 01731 #define FPU_FPCCR_MMRDY_Msk (1UL << FPU_FPCCR_MMRDY_Pos) /*!< FPCCR: MMRDY bit Mask */ 01732 01733 #define FPU_FPCCR_HFRDY_Pos 4U /*!< FPCCR: HFRDY Position */ 01734 #define FPU_FPCCR_HFRDY_Msk (1UL << FPU_FPCCR_HFRDY_Pos) /*!< FPCCR: HFRDY bit Mask */ 01735 01736 #define FPU_FPCCR_THREAD_Pos 3U /*!< FPCCR: processor mode bit Position */ 01737 #define FPU_FPCCR_THREAD_Msk (1UL << FPU_FPCCR_THREAD_Pos) /*!< FPCCR: processor mode active bit Mask */ 01738 01739 #define FPU_FPCCR_S_Pos 2U /*!< FPCCR: Security status of the FP context bit Position */ 01740 #define FPU_FPCCR_S_Msk (1UL << FPU_FPCCR_S_Pos) /*!< FPCCR: Security status of the FP context bit Mask */ 01741 01742 #define FPU_FPCCR_USER_Pos 1U /*!< FPCCR: privilege level bit Position */ 01743 #define FPU_FPCCR_USER_Msk (1UL << FPU_FPCCR_USER_Pos) /*!< FPCCR: privilege level bit Mask */ 01744 01745 #define FPU_FPCCR_LSPACT_Pos 0U /*!< FPCCR: Lazy state preservation active bit Position */ 01746 #define FPU_FPCCR_LSPACT_Msk (1UL /*<< FPU_FPCCR_LSPACT_Pos*/) /*!< FPCCR: Lazy state preservation active bit Mask */ 01747 01748 /* Floating-Point Context Address Register Definitions */ 01749 #define FPU_FPCAR_ADDRESS_Pos 3U /*!< FPCAR: ADDRESS bit Position */ 01750 #define FPU_FPCAR_ADDRESS_Msk (0x1FFFFFFFUL << FPU_FPCAR_ADDRESS_Pos) /*!< FPCAR: ADDRESS bit Mask */ 01751 01752 /* Floating-Point Default Status Control Register Definitions */ 01753 #define FPU_FPDSCR_AHP_Pos 26U /*!< FPDSCR: AHP bit Position */ 01754 #define FPU_FPDSCR_AHP_Msk (1UL << FPU_FPDSCR_AHP_Pos) /*!< FPDSCR: AHP bit Mask */ 01755 01756 #define FPU_FPDSCR_DN_Pos 25U /*!< FPDSCR: DN bit Position */ 01757 #define FPU_FPDSCR_DN_Msk (1UL << FPU_FPDSCR_DN_Pos) /*!< FPDSCR: DN bit Mask */ 01758 01759 #define FPU_FPDSCR_FZ_Pos 24U /*!< FPDSCR: FZ bit Position */ 01760 #define FPU_FPDSCR_FZ_Msk (1UL << FPU_FPDSCR_FZ_Pos) /*!< FPDSCR: FZ bit Mask */ 01761 01762 #define FPU_FPDSCR_RMode_Pos 22U /*!< FPDSCR: RMode bit Position */ 01763 #define FPU_FPDSCR_RMode_Msk (3UL << FPU_FPDSCR_RMode_Pos) /*!< FPDSCR: RMode bit Mask */ 01764 01765 /* Media and FP Feature Register 0 Definitions */ 01766 #define FPU_MVFR0_FP_rounding_modes_Pos 28U /*!< MVFR0: FP rounding modes bits Position */ 01767 #define FPU_MVFR0_FP_rounding_modes_Msk (0xFUL << FPU_MVFR0_FP_rounding_modes_Pos) /*!< MVFR0: FP rounding modes bits Mask */ 01768 01769 #define FPU_MVFR0_Short_vectors_Pos 24U /*!< MVFR0: Short vectors bits Position */ 01770 #define FPU_MVFR0_Short_vectors_Msk (0xFUL << FPU_MVFR0_Short_vectors_Pos) /*!< MVFR0: Short vectors bits Mask */ 01771 01772 #define FPU_MVFR0_Square_root_Pos 20U /*!< MVFR0: Square root bits Position */ 01773 #define FPU_MVFR0_Square_root_Msk (0xFUL << FPU_MVFR0_Square_root_Pos) /*!< MVFR0: Square root bits Mask */ 01774 01775 #define FPU_MVFR0_Divide_Pos 16U /*!< MVFR0: Divide bits Position */ 01776 #define FPU_MVFR0_Divide_Msk (0xFUL << FPU_MVFR0_Divide_Pos) /*!< MVFR0: Divide bits Mask */ 01777 01778 #define FPU_MVFR0_FP_excep_trapping_Pos 12U /*!< MVFR0: FP exception trapping bits Position */ 01779 #define FPU_MVFR0_FP_excep_trapping_Msk (0xFUL << FPU_MVFR0_FP_excep_trapping_Pos) /*!< MVFR0: FP exception trapping bits Mask */ 01780 01781 #define FPU_MVFR0_Double_precision_Pos 8U /*!< MVFR0: Double-precision bits Position */ 01782 #define FPU_MVFR0_Double_precision_Msk (0xFUL << FPU_MVFR0_Double_precision_Pos) /*!< MVFR0: Double-precision bits Mask */ 01783 01784 #define FPU_MVFR0_Single_precision_Pos 4U /*!< MVFR0: Single-precision bits Position */ 01785 #define FPU_MVFR0_Single_precision_Msk (0xFUL << FPU_MVFR0_Single_precision_Pos) /*!< MVFR0: Single-precision bits Mask */ 01786 01787 #define FPU_MVFR0_A_SIMD_registers_Pos 0U /*!< MVFR0: A_SIMD registers bits Position */ 01788 #define FPU_MVFR0_A_SIMD_registers_Msk (0xFUL /*<< FPU_MVFR0_A_SIMD_registers_Pos*/) /*!< MVFR0: A_SIMD registers bits Mask */ 01789 01790 /* Media and FP Feature Register 1 Definitions */ 01791 #define FPU_MVFR1_FP_fused_MAC_Pos 28U /*!< MVFR1: FP fused MAC bits Position */ 01792 #define FPU_MVFR1_FP_fused_MAC_Msk (0xFUL << FPU_MVFR1_FP_fused_MAC_Pos) /*!< MVFR1: FP fused MAC bits Mask */ 01793 01794 #define FPU_MVFR1_FP_HPFP_Pos 24U /*!< MVFR1: FP HPFP bits Position */ 01795 #define FPU_MVFR1_FP_HPFP_Msk (0xFUL << FPU_MVFR1_FP_HPFP_Pos) /*!< MVFR1: FP HPFP bits Mask */ 01796 01797 #define FPU_MVFR1_D_NaN_mode_Pos 4U /*!< MVFR1: D_NaN mode bits Position */ 01798 #define FPU_MVFR1_D_NaN_mode_Msk (0xFUL << FPU_MVFR1_D_NaN_mode_Pos) /*!< MVFR1: D_NaN mode bits Mask */ 01799 01800 #define FPU_MVFR1_FtZ_mode_Pos 0U /*!< MVFR1: FtZ mode bits Position */ 01801 #define FPU_MVFR1_FtZ_mode_Msk (0xFUL /*<< FPU_MVFR1_FtZ_mode_Pos*/) /*!< MVFR1: FtZ mode bits Mask */ 01802 01803 /*@} end of group CMSIS_FPU */ 01804 01805 01806 /** 01807 \ingroup CMSIS_core_register 01808 \defgroup CMSIS_CoreDebug Core Debug Registers (CoreDebug) 01809 \brief Type definitions for the Core Debug Registers 01810 @{ 01811 */ 01812 01813 /** 01814 \brief Structure type to access the Core Debug Register (CoreDebug). 01815 */ 01816 typedef struct 01817 { 01818 __IOM uint32_t DHCSR; /*!< Offset: 0x000 (R/W) Debug Halting Control and Status Register */ 01819 __OM uint32_t DCRSR; /*!< Offset: 0x004 ( /W) Debug Core Register Selector Register */ 01820 __IOM uint32_t DCRDR; /*!< Offset: 0x008 (R/W) Debug Core Register Data Register */ 01821 __IOM uint32_t DEMCR; /*!< Offset: 0x00C (R/W) Debug Exception and Monitor Control Register */ 01822 uint32_t RESERVED4[1U]; 01823 __IOM uint32_t DAUTHCTRL; /*!< Offset: 0x014 (R/W) Debug Authentication Control Register */ 01824 __IOM uint32_t DSCSR; /*!< Offset: 0x018 (R/W) Debug Security Control and Status Register */ 01825 } CoreDebug_Type; 01826 01827 /* Debug Halting Control and Status Register Definitions */ 01828 #define CoreDebug_DHCSR_DBGKEY_Pos 16U /*!< CoreDebug DHCSR: DBGKEY Position */ 01829 #define CoreDebug_DHCSR_DBGKEY_Msk (0xFFFFUL << CoreDebug_DHCSR_DBGKEY_Pos) /*!< CoreDebug DHCSR: DBGKEY Mask */ 01830 01831 #define CoreDebug_DHCSR_S_RESTART_ST_Pos 26U /*!< CoreDebug DHCSR: S_RESTART_ST Position */ 01832 #define CoreDebug_DHCSR_S_RESTART_ST_Msk (1UL << CoreDebug_DHCSR_S_RESTART_ST_Pos) /*!< CoreDebug DHCSR: S_RESTART_ST Mask */ 01833 01834 #define CoreDebug_DHCSR_S_RESET_ST_Pos 25U /*!< CoreDebug DHCSR: S_RESET_ST Position */ 01835 #define CoreDebug_DHCSR_S_RESET_ST_Msk (1UL << CoreDebug_DHCSR_S_RESET_ST_Pos) /*!< CoreDebug DHCSR: S_RESET_ST Mask */ 01836 01837 #define CoreDebug_DHCSR_S_RETIRE_ST_Pos 24U /*!< CoreDebug DHCSR: S_RETIRE_ST Position */ 01838 #define CoreDebug_DHCSR_S_RETIRE_ST_Msk (1UL << CoreDebug_DHCSR_S_RETIRE_ST_Pos) /*!< CoreDebug DHCSR: S_RETIRE_ST Mask */ 01839 01840 #define CoreDebug_DHCSR_S_LOCKUP_Pos 19U /*!< CoreDebug DHCSR: S_LOCKUP Position */ 01841 #define CoreDebug_DHCSR_S_LOCKUP_Msk (1UL << CoreDebug_DHCSR_S_LOCKUP_Pos) /*!< CoreDebug DHCSR: S_LOCKUP Mask */ 01842 01843 #define CoreDebug_DHCSR_S_SLEEP_Pos 18U /*!< CoreDebug DHCSR: S_SLEEP Position */ 01844 #define CoreDebug_DHCSR_S_SLEEP_Msk (1UL << CoreDebug_DHCSR_S_SLEEP_Pos) /*!< CoreDebug DHCSR: S_SLEEP Mask */ 01845 01846 #define CoreDebug_DHCSR_S_HALT_Pos 17U /*!< CoreDebug DHCSR: S_HALT Position */ 01847 #define CoreDebug_DHCSR_S_HALT_Msk (1UL << CoreDebug_DHCSR_S_HALT_Pos) /*!< CoreDebug DHCSR: S_HALT Mask */ 01848 01849 #define CoreDebug_DHCSR_S_REGRDY_Pos 16U /*!< CoreDebug DHCSR: S_REGRDY Position */ 01850 #define CoreDebug_DHCSR_S_REGRDY_Msk (1UL << CoreDebug_DHCSR_S_REGRDY_Pos) /*!< CoreDebug DHCSR: S_REGRDY Mask */ 01851 01852 #define CoreDebug_DHCSR_C_SNAPSTALL_Pos 5U /*!< CoreDebug DHCSR: C_SNAPSTALL Position */ 01853 #define CoreDebug_DHCSR_C_SNAPSTALL_Msk (1UL << CoreDebug_DHCSR_C_SNAPSTALL_Pos) /*!< CoreDebug DHCSR: C_SNAPSTALL Mask */ 01854 01855 #define CoreDebug_DHCSR_C_MASKINTS_Pos 3U /*!< CoreDebug DHCSR: C_MASKINTS Position */ 01856 #define CoreDebug_DHCSR_C_MASKINTS_Msk (1UL << CoreDebug_DHCSR_C_MASKINTS_Pos) /*!< CoreDebug DHCSR: C_MASKINTS Mask */ 01857 01858 #define CoreDebug_DHCSR_C_STEP_Pos 2U /*!< CoreDebug DHCSR: C_STEP Position */ 01859 #define CoreDebug_DHCSR_C_STEP_Msk (1UL << CoreDebug_DHCSR_C_STEP_Pos) /*!< CoreDebug DHCSR: C_STEP Mask */ 01860 01861 #define CoreDebug_DHCSR_C_HALT_Pos 1U /*!< CoreDebug DHCSR: C_HALT Position */ 01862 #define CoreDebug_DHCSR_C_HALT_Msk (1UL << CoreDebug_DHCSR_C_HALT_Pos) /*!< CoreDebug DHCSR: C_HALT Mask */ 01863 01864 #define CoreDebug_DHCSR_C_DEBUGEN_Pos 0U /*!< CoreDebug DHCSR: C_DEBUGEN Position */ 01865 #define CoreDebug_DHCSR_C_DEBUGEN_Msk (1UL /*<< CoreDebug_DHCSR_C_DEBUGEN_Pos*/) /*!< CoreDebug DHCSR: C_DEBUGEN Mask */ 01866 01867 /* Debug Core Register Selector Register Definitions */ 01868 #define CoreDebug_DCRSR_REGWnR_Pos 16U /*!< CoreDebug DCRSR: REGWnR Position */ 01869 #define CoreDebug_DCRSR_REGWnR_Msk (1UL << CoreDebug_DCRSR_REGWnR_Pos) /*!< CoreDebug DCRSR: REGWnR Mask */ 01870 01871 #define CoreDebug_DCRSR_REGSEL_Pos 0U /*!< CoreDebug DCRSR: REGSEL Position */ 01872 #define CoreDebug_DCRSR_REGSEL_Msk (0x1FUL /*<< CoreDebug_DCRSR_REGSEL_Pos*/) /*!< CoreDebug DCRSR: REGSEL Mask */ 01873 01874 /* Debug Exception and Monitor Control Register Definitions */ 01875 #define CoreDebug_DEMCR_TRCENA_Pos 24U /*!< CoreDebug DEMCR: TRCENA Position */ 01876 #define CoreDebug_DEMCR_TRCENA_Msk (1UL << CoreDebug_DEMCR_TRCENA_Pos) /*!< CoreDebug DEMCR: TRCENA Mask */ 01877 01878 #define CoreDebug_DEMCR_MON_REQ_Pos 19U /*!< CoreDebug DEMCR: MON_REQ Position */ 01879 #define CoreDebug_DEMCR_MON_REQ_Msk (1UL << CoreDebug_DEMCR_MON_REQ_Pos) /*!< CoreDebug DEMCR: MON_REQ Mask */ 01880 01881 #define CoreDebug_DEMCR_MON_STEP_Pos 18U /*!< CoreDebug DEMCR: MON_STEP Position */ 01882 #define CoreDebug_DEMCR_MON_STEP_Msk (1UL << CoreDebug_DEMCR_MON_STEP_Pos) /*!< CoreDebug DEMCR: MON_STEP Mask */ 01883 01884 #define CoreDebug_DEMCR_MON_PEND_Pos 17U /*!< CoreDebug DEMCR: MON_PEND Position */ 01885 #define CoreDebug_DEMCR_MON_PEND_Msk (1UL << CoreDebug_DEMCR_MON_PEND_Pos) /*!< CoreDebug DEMCR: MON_PEND Mask */ 01886 01887 #define CoreDebug_DEMCR_MON_EN_Pos 16U /*!< CoreDebug DEMCR: MON_EN Position */ 01888 #define CoreDebug_DEMCR_MON_EN_Msk (1UL << CoreDebug_DEMCR_MON_EN_Pos) /*!< CoreDebug DEMCR: MON_EN Mask */ 01889 01890 #define CoreDebug_DEMCR_VC_HARDERR_Pos 10U /*!< CoreDebug DEMCR: VC_HARDERR Position */ 01891 #define CoreDebug_DEMCR_VC_HARDERR_Msk (1UL << CoreDebug_DEMCR_VC_HARDERR_Pos) /*!< CoreDebug DEMCR: VC_HARDERR Mask */ 01892 01893 #define CoreDebug_DEMCR_VC_INTERR_Pos 9U /*!< CoreDebug DEMCR: VC_INTERR Position */ 01894 #define CoreDebug_DEMCR_VC_INTERR_Msk (1UL << CoreDebug_DEMCR_VC_INTERR_Pos) /*!< CoreDebug DEMCR: VC_INTERR Mask */ 01895 01896 #define CoreDebug_DEMCR_VC_BUSERR_Pos 8U /*!< CoreDebug DEMCR: VC_BUSERR Position */ 01897 #define CoreDebug_DEMCR_VC_BUSERR_Msk (1UL << CoreDebug_DEMCR_VC_BUSERR_Pos) /*!< CoreDebug DEMCR: VC_BUSERR Mask */ 01898 01899 #define CoreDebug_DEMCR_VC_STATERR_Pos 7U /*!< CoreDebug DEMCR: VC_STATERR Position */ 01900 #define CoreDebug_DEMCR_VC_STATERR_Msk (1UL << CoreDebug_DEMCR_VC_STATERR_Pos) /*!< CoreDebug DEMCR: VC_STATERR Mask */ 01901 01902 #define CoreDebug_DEMCR_VC_CHKERR_Pos 6U /*!< CoreDebug DEMCR: VC_CHKERR Position */ 01903 #define CoreDebug_DEMCR_VC_CHKERR_Msk (1UL << CoreDebug_DEMCR_VC_CHKERR_Pos) /*!< CoreDebug DEMCR: VC_CHKERR Mask */ 01904 01905 #define CoreDebug_DEMCR_VC_NOCPERR_Pos 5U /*!< CoreDebug DEMCR: VC_NOCPERR Position */ 01906 #define CoreDebug_DEMCR_VC_NOCPERR_Msk (1UL << CoreDebug_DEMCR_VC_NOCPERR_Pos) /*!< CoreDebug DEMCR: VC_NOCPERR Mask */ 01907 01908 #define CoreDebug_DEMCR_VC_MMERR_Pos 4U /*!< CoreDebug DEMCR: VC_MMERR Position */ 01909 #define CoreDebug_DEMCR_VC_MMERR_Msk (1UL << CoreDebug_DEMCR_VC_MMERR_Pos) /*!< CoreDebug DEMCR: VC_MMERR Mask */ 01910 01911 #define CoreDebug_DEMCR_VC_CORERESET_Pos 0U /*!< CoreDebug DEMCR: VC_CORERESET Position */ 01912 #define CoreDebug_DEMCR_VC_CORERESET_Msk (1UL /*<< CoreDebug_DEMCR_VC_CORERESET_Pos*/) /*!< CoreDebug DEMCR: VC_CORERESET Mask */ 01913 01914 /* Debug Authentication Control Register Definitions */ 01915 #define CoreDebug_DAUTHCTRL_INTSPNIDEN_Pos 3U /*!< CoreDebug DAUTHCTRL: INTSPNIDEN, Position */ 01916 #define CoreDebug_DAUTHCTRL_INTSPNIDEN_Msk (1UL << CoreDebug_DAUTHCTRL_INTSPNIDEN_Pos) /*!< CoreDebug DAUTHCTRL: INTSPNIDEN, Mask */ 01917 01918 #define CoreDebug_DAUTHCTRL_SPNIDENSEL_Pos 2U /*!< CoreDebug DAUTHCTRL: SPNIDENSEL Position */ 01919 #define CoreDebug_DAUTHCTRL_SPNIDENSEL_Msk (1UL << CoreDebug_DAUTHCTRL_SPNIDENSEL_Pos) /*!< CoreDebug DAUTHCTRL: SPNIDENSEL Mask */ 01920 01921 #define CoreDebug_DAUTHCTRL_INTSPIDEN_Pos 1U /*!< CoreDebug DAUTHCTRL: INTSPIDEN Position */ 01922 #define CoreDebug_DAUTHCTRL_INTSPIDEN_Msk (1UL << CoreDebug_DAUTHCTRL_INTSPIDEN_Pos) /*!< CoreDebug DAUTHCTRL: INTSPIDEN Mask */ 01923 01924 #define CoreDebug_DAUTHCTRL_SPIDENSEL_Pos 0U /*!< CoreDebug DAUTHCTRL: SPIDENSEL Position */ 01925 #define CoreDebug_DAUTHCTRL_SPIDENSEL_Msk (1UL /*<< CoreDebug_DAUTHCTRL_SPIDENSEL_Pos*/) /*!< CoreDebug DAUTHCTRL: SPIDENSEL Mask */ 01926 01927 /* Debug Security Control and Status Register Definitions */ 01928 #define CoreDebug_DSCSR_CDS_Pos 16U /*!< CoreDebug DSCSR: CDS Position */ 01929 #define CoreDebug_DSCSR_CDS_Msk (1UL << CoreDebug_DSCSR_CDS_Pos) /*!< CoreDebug DSCSR: CDS Mask */ 01930 01931 #define CoreDebug_DSCSR_SBRSEL_Pos 1U /*!< CoreDebug DSCSR: SBRSEL Position */ 01932 #define CoreDebug_DSCSR_SBRSEL_Msk (1UL << CoreDebug_DSCSR_SBRSEL_Pos) /*!< CoreDebug DSCSR: SBRSEL Mask */ 01933 01934 #define CoreDebug_DSCSR_SBRSELEN_Pos 0U /*!< CoreDebug DSCSR: SBRSELEN Position */ 01935 #define CoreDebug_DSCSR_SBRSELEN_Msk (1UL /*<< CoreDebug_DSCSR_SBRSELEN_Pos*/) /*!< CoreDebug DSCSR: SBRSELEN Mask */ 01936 01937 /*@} end of group CMSIS_CoreDebug */ 01938 01939 01940 /** 01941 \ingroup CMSIS_core_register 01942 \defgroup CMSIS_core_bitfield Core register bit field macros 01943 \brief Macros for use with bit field definitions (xxx_Pos, xxx_Msk). 01944 @{ 01945 */ 01946 01947 /** 01948 \brief Mask and shift a bit field value for use in a register bit range. 01949 \param[in] field Name of the register bit field. 01950 \param[in] value Value of the bit field. This parameter is interpreted as an uint32_t type. 01951 \return Masked and shifted value. 01952 */ 01953 #define _VAL2FLD(field, value) (((uint32_t)(value) << field ## _Pos) & field ## _Msk) 01954 01955 /** 01956 \brief Mask and shift a register value to extract a bit filed value. 01957 \param[in] field Name of the register bit field. 01958 \param[in] value Value of register. This parameter is interpreted as an uint32_t type. 01959 \return Masked and shifted bit field value. 01960 */ 01961 #define _FLD2VAL(field, value) (((uint32_t)(value) & field ## _Msk) >> field ## _Pos) 01962 01963 /*@} end of group CMSIS_core_bitfield */ 01964 01965 01966 /** 01967 \ingroup CMSIS_core_register 01968 \defgroup CMSIS_core_base Core Definitions 01969 \brief Definitions for base addresses, unions, and structures. 01970 @{ 01971 */ 01972 01973 /* Memory mapping of Core Hardware */ 01974 #define SCS_BASE (0xE000E000UL) /*!< System Control Space Base Address */ 01975 #define ITM_BASE (0xE0000000UL) /*!< ITM Base Address */ 01976 #define DWT_BASE (0xE0001000UL) /*!< DWT Base Address */ 01977 #define TPI_BASE (0xE0040000UL) /*!< TPI Base Address */ 01978 #define CoreDebug_BASE (0xE000EDF0UL) /*!< Core Debug Base Address */ 01979 #define SysTick_BASE (SCS_BASE + 0x0010UL) /*!< SysTick Base Address */ 01980 #define NVIC_BASE (SCS_BASE + 0x0100UL) /*!< NVIC Base Address */ 01981 #define SCB_BASE (SCS_BASE + 0x0D00UL) /*!< System Control Block Base Address */ 01982 01983 #define SCnSCB ((SCnSCB_Type *) SCS_BASE ) /*!< System control Register not in SCB */ 01984 #define SCB ((SCB_Type *) SCB_BASE ) /*!< SCB configuration struct */ 01985 #define SysTick ((SysTick_Type *) SysTick_BASE ) /*!< SysTick configuration struct */ 01986 #define NVIC ((NVIC_Type *) NVIC_BASE ) /*!< NVIC configuration struct */ 01987 #define ITM ((ITM_Type *) ITM_BASE ) /*!< ITM configuration struct */ 01988 #define DWT ((DWT_Type *) DWT_BASE ) /*!< DWT configuration struct */ 01989 #define TPI ((TPI_Type *) TPI_BASE ) /*!< TPI configuration struct */ 01990 #define CoreDebug ((CoreDebug_Type *) CoreDebug_BASE ) /*!< Core Debug configuration struct */ 01991 01992 #if defined (__MPU_PRESENT) && (__MPU_PRESENT == 1U) 01993 #define MPU_BASE (SCS_BASE + 0x0D90UL) /*!< Memory Protection Unit */ 01994 #define MPU ((MPU_Type *) MPU_BASE ) /*!< Memory Protection Unit */ 01995 #endif 01996 01997 #if defined (__ARM_FEATURE_CMSE) && (__ARM_FEATURE_CMSE == 3U) 01998 #define SAU_BASE (SCS_BASE + 0x0DD0UL) /*!< Security Attribution Unit */ 01999 #define SAU ((SAU_Type *) SAU_BASE ) /*!< Security Attribution Unit */ 02000 #endif 02001 02002 #define FPU_BASE (SCS_BASE + 0x0F30UL) /*!< Floating Point Unit */ 02003 #define FPU ((FPU_Type *) FPU_BASE ) /*!< Floating Point Unit */ 02004 02005 #if defined (__ARM_FEATURE_CMSE) && (__ARM_FEATURE_CMSE == 3U) 02006 #define SCS_BASE_NS (0xE002E000UL) /*!< System Control Space Base Address (non-secure address space) */ 02007 #define CoreDebug_BASE_NS (0xE002EDF0UL) /*!< Core Debug Base Address (non-secure address space) */ 02008 #define SysTick_BASE_NS (SCS_BASE_NS + 0x0010UL) /*!< SysTick Base Address (non-secure address space) */ 02009 #define NVIC_BASE_NS (SCS_BASE_NS + 0x0100UL) /*!< NVIC Base Address (non-secure address space) */ 02010 #define SCB_BASE_NS (SCS_BASE_NS + 0x0D00UL) /*!< System Control Block Base Address (non-secure address space) */ 02011 02012 #define SCnSCB_NS ((SCnSCB_Type *) SCS_BASE_NS ) /*!< System control Register not in SCB(non-secure address space) */ 02013 #define SCB_NS ((SCB_Type *) SCB_BASE_NS ) /*!< SCB configuration struct (non-secure address space) */ 02014 #define SysTick_NS ((SysTick_Type *) SysTick_BASE_NS ) /*!< SysTick configuration struct (non-secure address space) */ 02015 #define NVIC_NS ((NVIC_Type *) NVIC_BASE_NS ) /*!< NVIC configuration struct (non-secure address space) */ 02016 #define CoreDebug_NS ((CoreDebug_Type *) CoreDebug_BASE_NS) /*!< Core Debug configuration struct (non-secure address space) */ 02017 02018 #if defined (__MPU_PRESENT) && (__MPU_PRESENT == 1U) 02019 #define MPU_BASE_NS (SCS_BASE_NS + 0x0D90UL) /*!< Memory Protection Unit (non-secure address space) */ 02020 #define MPU_NS ((MPU_Type *) MPU_BASE_NS ) /*!< Memory Protection Unit (non-secure address space) */ 02021 #endif 02022 02023 #define FPU_BASE_NS (SCS_BASE_NS + 0x0F30UL) /*!< Floating Point Unit (non-secure address space) */ 02024 #define FPU_NS ((FPU_Type *) FPU_BASE_NS ) /*!< Floating Point Unit (non-secure address space) */ 02025 02026 #endif /* defined (__ARM_FEATURE_CMSE) && (__ARM_FEATURE_CMSE == 3U) */ 02027 /*@} */ 02028 02029 02030 02031 /******************************************************************************* 02032 * Hardware Abstraction Layer 02033 Core Function Interface contains: 02034 - Core NVIC Functions 02035 - Core SysTick Functions 02036 - Core Debug Functions 02037 - Core Register Access Functions 02038 ******************************************************************************/ 02039 /** 02040 \defgroup CMSIS_Core_FunctionInterface Functions and Instructions Reference 02041 */ 02042 02043 02044 02045 /* ########################## NVIC functions #################################### */ 02046 /** 02047 \ingroup CMSIS_Core_FunctionInterface 02048 \defgroup CMSIS_Core_NVICFunctions NVIC Functions 02049 \brief Functions that manage interrupts and exceptions via the NVIC. 02050 @{ 02051 */ 02052 02053 #ifdef CMSIS_NVIC_VIRTUAL 02054 #ifndef CMSIS_NVIC_VIRTUAL_HEADER_FILE 02055 #define CMSIS_NVIC_VIRTUAL_HEADER_FILE "cmsis_nvic_virtual.h" 02056 #endif 02057 #include CMSIS_NVIC_VIRTUAL_HEADER_FILE 02058 #else 02059 #define NVIC_SetPriorityGrouping __NVIC_SetPriorityGrouping 02060 #define NVIC_GetPriorityGrouping __NVIC_GetPriorityGrouping 02061 #define NVIC_EnableIRQ __NVIC_EnableIRQ 02062 #define NVIC_GetEnableIRQ __NVIC_GetEnableIRQ 02063 #define NVIC_DisableIRQ __NVIC_DisableIRQ 02064 #define NVIC_GetPendingIRQ __NVIC_GetPendingIRQ 02065 #define NVIC_SetPendingIRQ __NVIC_SetPendingIRQ 02066 #define NVIC_ClearPendingIRQ __NVIC_ClearPendingIRQ 02067 #define NVIC_GetActive __NVIC_GetActive 02068 #define NVIC_SetPriority __NVIC_SetPriority 02069 #define NVIC_GetPriority __NVIC_GetPriority 02070 #define NVIC_SystemReset __NVIC_SystemReset 02071 #endif /* CMSIS_NVIC_VIRTUAL */ 02072 02073 #ifdef CMSIS_VECTAB_VIRTUAL 02074 #ifndef CMSIS_VECTAB_VIRTUAL_HEADER_FILE 02075 #define CMSIS_VECTAB_VIRTUAL_HEADER_FILE "cmsis_vectab_virtual.h" 02076 #endif 02077 #include CMSIS_VECTAB_VIRTUAL_HEADER_FILE 02078 #else 02079 #define NVIC_SetVector __NVIC_SetVector 02080 #define NVIC_GetVector __NVIC_GetVector 02081 #endif /* (CMSIS_VECTAB_VIRTUAL) */ 02082 02083 #define NVIC_USER_IRQ_OFFSET 16 02084 02085 02086 02087 /** 02088 \brief Set Priority Grouping 02089 \details Sets the priority grouping field using the required unlock sequence. 02090 The parameter PriorityGroup is assigned to the field SCB->AIRCR [10:8] PRIGROUP field. 02091 Only values from 0..7 are used. 02092 In case of a conflict between priority grouping and available 02093 priority bits (__NVIC_PRIO_BITS), the smallest possible priority group is set. 02094 \param [in] PriorityGroup Priority grouping field. 02095 */ 02096 __STATIC_INLINE void __NVIC_SetPriorityGrouping(uint32_t PriorityGroup) 02097 { 02098 uint32_t reg_value; 02099 uint32_t PriorityGroupTmp = (PriorityGroup & (uint32_t)0x07UL); /* only values 0..7 are used */ 02100 02101 reg_value = SCB->AIRCR; /* read old register configuration */ 02102 reg_value &= ~((uint32_t)(SCB_AIRCR_VECTKEY_Msk | SCB_AIRCR_PRIGROUP_Msk)); /* clear bits to change */ 02103 reg_value = (reg_value | 02104 ((uint32_t)0x5FAUL << SCB_AIRCR_VECTKEY_Pos) | 02105 (PriorityGroupTmp << 8U) ); /* Insert write key and priorty group */ 02106 SCB->AIRCR = reg_value; 02107 } 02108 02109 02110 /** 02111 \brief Get Priority Grouping 02112 \details Reads the priority grouping field from the NVIC Interrupt Controller. 02113 \return Priority grouping field (SCB->AIRCR [10:8] PRIGROUP field). 02114 */ 02115 __STATIC_INLINE uint32_t __NVIC_GetPriorityGrouping(void) 02116 { 02117 return ((uint32_t)((SCB->AIRCR & SCB_AIRCR_PRIGROUP_Msk) >> SCB_AIRCR_PRIGROUP_Pos)); 02118 } 02119 02120 02121 /** 02122 \brief Enable Interrupt 02123 \details Enables a device specific interrupt in the NVIC interrupt controller. 02124 \param [in] IRQn Device specific interrupt number. 02125 \note IRQn must not be negative. 02126 */ 02127 __STATIC_INLINE void __NVIC_EnableIRQ(IRQn_Type IRQn) 02128 { 02129 if ((int32_t)(IRQn) >= 0) 02130 { 02131 NVIC->ISER[(((uint32_t)(int32_t)IRQn) >> 5UL)] = (uint32_t)(1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL)); 02132 } 02133 } 02134 02135 02136 /** 02137 \brief Get Interrupt Enable status 02138 \details Returns a device specific interrupt enable status from the NVIC interrupt controller. 02139 \param [in] IRQn Device specific interrupt number. 02140 \return 0 Interrupt is not enabled. 02141 \return 1 Interrupt is enabled. 02142 \note IRQn must not be negative. 02143 */ 02144 __STATIC_INLINE uint32_t __NVIC_GetEnableIRQ(IRQn_Type IRQn) 02145 { 02146 if ((int32_t)(IRQn) >= 0) 02147 { 02148 return((uint32_t)(((NVIC->ISER[(((uint32_t)(int32_t)IRQn) >> 5UL)] & (1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL))) != 0UL) ? 1UL : 0UL)); 02149 } 02150 else 02151 { 02152 return(0U); 02153 } 02154 } 02155 02156 02157 /** 02158 \brief Disable Interrupt 02159 \details Disables a device specific interrupt in the NVIC interrupt controller. 02160 \param [in] IRQn Device specific interrupt number. 02161 \note IRQn must not be negative. 02162 */ 02163 __STATIC_INLINE void __NVIC_DisableIRQ(IRQn_Type IRQn) 02164 { 02165 if ((int32_t)(IRQn) >= 0) 02166 { 02167 NVIC->ICER[(((uint32_t)(int32_t)IRQn) >> 5UL)] = (uint32_t)(1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL)); 02168 __DSB(); 02169 __ISB(); 02170 } 02171 } 02172 02173 02174 /** 02175 \brief Get Pending Interrupt 02176 \details Reads the NVIC pending register and returns the pending bit for the specified device specific interrupt. 02177 \param [in] IRQn Device specific interrupt number. 02178 \return 0 Interrupt status is not pending. 02179 \return 1 Interrupt status is pending. 02180 \note IRQn must not be negative. 02181 */ 02182 __STATIC_INLINE uint32_t __NVIC_GetPendingIRQ(IRQn_Type IRQn) 02183 { 02184 if ((int32_t)(IRQn) >= 0) 02185 { 02186 return((uint32_t)(((NVIC->ISPR[(((uint32_t)(int32_t)IRQn) >> 5UL)] & (1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL))) != 0UL) ? 1UL : 0UL)); 02187 } 02188 else 02189 { 02190 return(0U); 02191 } 02192 } 02193 02194 02195 /** 02196 \brief Set Pending Interrupt 02197 \details Sets the pending bit of a device specific interrupt in the NVIC pending register. 02198 \param [in] IRQn Device specific interrupt number. 02199 \note IRQn must not be negative. 02200 */ 02201 __STATIC_INLINE void __NVIC_SetPendingIRQ(IRQn_Type IRQn) 02202 { 02203 if ((int32_t)(IRQn) >= 0) 02204 { 02205 NVIC->ISPR[(((uint32_t)(int32_t)IRQn) >> 5UL)] = (uint32_t)(1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL)); 02206 } 02207 } 02208 02209 02210 /** 02211 \brief Clear Pending Interrupt 02212 \details Clears the pending bit of a device specific interrupt in the NVIC pending register. 02213 \param [in] IRQn Device specific interrupt number. 02214 \note IRQn must not be negative. 02215 */ 02216 __STATIC_INLINE void __NVIC_ClearPendingIRQ(IRQn_Type IRQn) 02217 { 02218 if ((int32_t)(IRQn) >= 0) 02219 { 02220 NVIC->ICPR[(((uint32_t)(int32_t)IRQn) >> 5UL)] = (uint32_t)(1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL)); 02221 } 02222 } 02223 02224 02225 /** 02226 \brief Get Active Interrupt 02227 \details Reads the active register in the NVIC and returns the active bit for the device specific interrupt. 02228 \param [in] IRQn Device specific interrupt number. 02229 \return 0 Interrupt status is not active. 02230 \return 1 Interrupt status is active. 02231 \note IRQn must not be negative. 02232 */ 02233 __STATIC_INLINE uint32_t __NVIC_GetActive(IRQn_Type IRQn) 02234 { 02235 if ((int32_t)(IRQn) >= 0) 02236 { 02237 return((uint32_t)(((NVIC->IABR[(((uint32_t)(int32_t)IRQn) >> 5UL)] & (1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL))) != 0UL) ? 1UL : 0UL)); 02238 } 02239 else 02240 { 02241 return(0U); 02242 } 02243 } 02244 02245 02246 #if defined (__ARM_FEATURE_CMSE) && (__ARM_FEATURE_CMSE == 3U) 02247 /** 02248 \brief Get Interrupt Target State 02249 \details Reads the interrupt target field in the NVIC and returns the interrupt target bit for the device specific interrupt. 02250 \param [in] IRQn Device specific interrupt number. 02251 \return 0 if interrupt is assigned to Secure 02252 \return 1 if interrupt is assigned to Non Secure 02253 \note IRQn must not be negative. 02254 */ 02255 __STATIC_INLINE uint32_t NVIC_GetTargetState(IRQn_Type IRQn) 02256 { 02257 if ((int32_t)(IRQn) >= 0) 02258 { 02259 return((uint32_t)(((NVIC->ITNS[(((uint32_t)(int32_t)IRQn) >> 5UL)] & (1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL))) != 0UL) ? 1UL : 0UL)); 02260 } 02261 else 02262 { 02263 return(0U); 02264 } 02265 } 02266 02267 02268 /** 02269 \brief Set Interrupt Target State 02270 \details Sets the interrupt target field in the NVIC and returns the interrupt target bit for the device specific interrupt. 02271 \param [in] IRQn Device specific interrupt number. 02272 \return 0 if interrupt is assigned to Secure 02273 1 if interrupt is assigned to Non Secure 02274 \note IRQn must not be negative. 02275 */ 02276 __STATIC_INLINE uint32_t NVIC_SetTargetState(IRQn_Type IRQn) 02277 { 02278 if ((int32_t)(IRQn) >= 0) 02279 { 02280 NVIC->ITNS[(((uint32_t)(int32_t)IRQn) >> 5UL)] |= ((uint32_t)(1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL))); 02281 return((uint32_t)(((NVIC->ITNS[(((uint32_t)(int32_t)IRQn) >> 5UL)] & (1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL))) != 0UL) ? 1UL : 0UL)); 02282 } 02283 else 02284 { 02285 return(0U); 02286 } 02287 } 02288 02289 02290 /** 02291 \brief Clear Interrupt Target State 02292 \details Clears the interrupt target field in the NVIC and returns the interrupt target bit for the device specific interrupt. 02293 \param [in] IRQn Device specific interrupt number. 02294 \return 0 if interrupt is assigned to Secure 02295 1 if interrupt is assigned to Non Secure 02296 \note IRQn must not be negative. 02297 */ 02298 __STATIC_INLINE uint32_t NVIC_ClearTargetState(IRQn_Type IRQn) 02299 { 02300 if ((int32_t)(IRQn) >= 0) 02301 { 02302 NVIC->ITNS[(((uint32_t)(int32_t)IRQn) >> 5UL)] &= ~((uint32_t)(1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL))); 02303 return((uint32_t)(((NVIC->ITNS[(((uint32_t)(int32_t)IRQn) >> 5UL)] & (1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL))) != 0UL) ? 1UL : 0UL)); 02304 } 02305 else 02306 { 02307 return(0U); 02308 } 02309 } 02310 #endif /* defined (__ARM_FEATURE_CMSE) && (__ARM_FEATURE_CMSE == 3U) */ 02311 02312 02313 /** 02314 \brief Set Interrupt Priority 02315 \details Sets the priority of a device specific interrupt or a processor exception. 02316 The interrupt number can be positive to specify a device specific interrupt, 02317 or negative to specify a processor exception. 02318 \param [in] IRQn Interrupt number. 02319 \param [in] priority Priority to set. 02320 \note The priority cannot be set for every processor exception. 02321 */ 02322 __STATIC_INLINE void __NVIC_SetPriority(IRQn_Type IRQn, uint32_t priority) 02323 { 02324 if ((int32_t)(IRQn) >= 0) 02325 { 02326 NVIC->IPR[((uint32_t)(int32_t)IRQn)] = (uint8_t)((priority << (8U - __NVIC_PRIO_BITS)) & (uint32_t)0xFFUL); 02327 } 02328 else 02329 { 02330 SCB->SHPR[(((uint32_t)(int32_t)IRQn) & 0xFUL)-4UL] = (uint8_t)((priority << (8U - __NVIC_PRIO_BITS)) & (uint32_t)0xFFUL); 02331 } 02332 } 02333 02334 02335 /** 02336 \brief Get Interrupt Priority 02337 \details Reads the priority of a device specific interrupt or a processor exception. 02338 The interrupt number can be positive to specify a device specific interrupt, 02339 or negative to specify a processor exception. 02340 \param [in] IRQn Interrupt number. 02341 \return Interrupt Priority. 02342 Value is aligned automatically to the implemented priority bits of the microcontroller. 02343 */ 02344 __STATIC_INLINE uint32_t __NVIC_GetPriority(IRQn_Type IRQn) 02345 { 02346 02347 if ((int32_t)(IRQn) >= 0) 02348 { 02349 return(((uint32_t)NVIC->IPR[((uint32_t)(int32_t)IRQn)] >> (8U - __NVIC_PRIO_BITS))); 02350 } 02351 else 02352 { 02353 return(((uint32_t)SCB->SHPR[(((uint32_t)(int32_t)IRQn) & 0xFUL)-4UL] >> (8U - __NVIC_PRIO_BITS))); 02354 } 02355 } 02356 02357 02358 /** 02359 \brief Encode Priority 02360 \details Encodes the priority for an interrupt with the given priority group, 02361 preemptive priority value, and subpriority value. 02362 In case of a conflict between priority grouping and available 02363 priority bits (__NVIC_PRIO_BITS), the smallest possible priority group is set. 02364 \param [in] PriorityGroup Used priority group. 02365 \param [in] PreemptPriority Preemptive priority value (starting from 0). 02366 \param [in] SubPriority Subpriority value (starting from 0). 02367 \return Encoded priority. Value can be used in the function \ref NVIC_SetPriority(). 02368 */ 02369 __STATIC_INLINE uint32_t NVIC_EncodePriority (uint32_t PriorityGroup, uint32_t PreemptPriority, uint32_t SubPriority) 02370 { 02371 uint32_t PriorityGroupTmp = (PriorityGroup & (uint32_t)0x07UL); /* only values 0..7 are used */ 02372 uint32_t PreemptPriorityBits; 02373 uint32_t SubPriorityBits; 02374 02375 PreemptPriorityBits = ((7UL - PriorityGroupTmp) > (uint32_t)(__NVIC_PRIO_BITS)) ? (uint32_t)(__NVIC_PRIO_BITS) : (uint32_t)(7UL - PriorityGroupTmp); 02376 SubPriorityBits = ((PriorityGroupTmp + (uint32_t)(__NVIC_PRIO_BITS)) < (uint32_t)7UL) ? (uint32_t)0UL : (uint32_t)((PriorityGroupTmp - 7UL) + (uint32_t)(__NVIC_PRIO_BITS)); 02377 02378 return ( 02379 ((PreemptPriority & (uint32_t)((1UL << (PreemptPriorityBits)) - 1UL)) << SubPriorityBits) | 02380 ((SubPriority & (uint32_t)((1UL << (SubPriorityBits )) - 1UL))) 02381 ); 02382 } 02383 02384 02385 /** 02386 \brief Decode Priority 02387 \details Decodes an interrupt priority value with a given priority group to 02388 preemptive priority value and subpriority value. 02389 In case of a conflict between priority grouping and available 02390 priority bits (__NVIC_PRIO_BITS) the smallest possible priority group is set. 02391 \param [in] Priority Priority value, which can be retrieved with the function \ref NVIC_GetPriority(). 02392 \param [in] PriorityGroup Used priority group. 02393 \param [out] pPreemptPriority Preemptive priority value (starting from 0). 02394 \param [out] pSubPriority Subpriority value (starting from 0). 02395 */ 02396 __STATIC_INLINE void NVIC_DecodePriority (uint32_t Priority, uint32_t PriorityGroup, uint32_t* const pPreemptPriority, uint32_t* const pSubPriority) 02397 { 02398 uint32_t PriorityGroupTmp = (PriorityGroup & (uint32_t)0x07UL); /* only values 0..7 are used */ 02399 uint32_t PreemptPriorityBits; 02400 uint32_t SubPriorityBits; 02401 02402 PreemptPriorityBits = ((7UL - PriorityGroupTmp) > (uint32_t)(__NVIC_PRIO_BITS)) ? (uint32_t)(__NVIC_PRIO_BITS) : (uint32_t)(7UL - PriorityGroupTmp); 02403 SubPriorityBits = ((PriorityGroupTmp + (uint32_t)(__NVIC_PRIO_BITS)) < (uint32_t)7UL) ? (uint32_t)0UL : (uint32_t)((PriorityGroupTmp - 7UL) + (uint32_t)(__NVIC_PRIO_BITS)); 02404 02405 *pPreemptPriority = (Priority >> SubPriorityBits) & (uint32_t)((1UL << (PreemptPriorityBits)) - 1UL); 02406 *pSubPriority = (Priority ) & (uint32_t)((1UL << (SubPriorityBits )) - 1UL); 02407 } 02408 02409 02410 /** 02411 \brief Set Interrupt Vector 02412 \details Sets an interrupt vector in SRAM based interrupt vector table. 02413 The interrupt number can be positive to specify a device specific interrupt, 02414 or negative to specify a processor exception. 02415 VTOR must been relocated to SRAM before. 02416 \param [in] IRQn Interrupt number 02417 \param [in] vector Address of interrupt handler function 02418 */ 02419 __STATIC_INLINE void __NVIC_SetVector(IRQn_Type IRQn, uint32_t vector) 02420 { 02421 uint32_t *vectors = (uint32_t *)SCB->VTOR; 02422 vectors[(int32_t)IRQn + NVIC_USER_IRQ_OFFSET] = vector; 02423 } 02424 02425 02426 /** 02427 \brief Get Interrupt Vector 02428 \details Reads an interrupt vector from interrupt vector table. 02429 The interrupt number can be positive to specify a device specific interrupt, 02430 or negative to specify a processor exception. 02431 \param [in] IRQn Interrupt number. 02432 \return Address of interrupt handler function 02433 */ 02434 __STATIC_INLINE uint32_t __NVIC_GetVector(IRQn_Type IRQn) 02435 { 02436 uint32_t *vectors = (uint32_t *)SCB->VTOR; 02437 return vectors[(int32_t)IRQn + NVIC_USER_IRQ_OFFSET]; 02438 } 02439 02440 02441 /** 02442 \brief System Reset 02443 \details Initiates a system reset request to reset the MCU. 02444 */ 02445 __STATIC_INLINE void __NVIC_SystemReset(void) 02446 { 02447 __DSB(); /* Ensure all outstanding memory accesses included 02448 buffered write are completed before reset */ 02449 SCB->AIRCR = (uint32_t)((0x5FAUL << SCB_AIRCR_VECTKEY_Pos) | 02450 (SCB->AIRCR & SCB_AIRCR_PRIGROUP_Msk) | 02451 SCB_AIRCR_SYSRESETREQ_Msk ); /* Keep priority group unchanged */ 02452 __DSB(); /* Ensure completion of memory access */ 02453 02454 for(;;) /* wait until reset */ 02455 { 02456 __NOP(); 02457 } 02458 } 02459 02460 #if defined (__ARM_FEATURE_CMSE) && (__ARM_FEATURE_CMSE == 3U) 02461 /** 02462 \brief Set Priority Grouping (non-secure) 02463 \details Sets the non-secure priority grouping field when in secure state using the required unlock sequence. 02464 The parameter PriorityGroup is assigned to the field SCB->AIRCR [10:8] PRIGROUP field. 02465 Only values from 0..7 are used. 02466 In case of a conflict between priority grouping and available 02467 priority bits (__NVIC_PRIO_BITS), the smallest possible priority group is set. 02468 \param [in] PriorityGroup Priority grouping field. 02469 */ 02470 __STATIC_INLINE void TZ_NVIC_SetPriorityGrouping_NS(uint32_t PriorityGroup) 02471 { 02472 uint32_t reg_value; 02473 uint32_t PriorityGroupTmp = (PriorityGroup & (uint32_t)0x07UL); /* only values 0..7 are used */ 02474 02475 reg_value = SCB_NS->AIRCR; /* read old register configuration */ 02476 reg_value &= ~((uint32_t)(SCB_AIRCR_VECTKEY_Msk | SCB_AIRCR_PRIGROUP_Msk)); /* clear bits to change */ 02477 reg_value = (reg_value | 02478 ((uint32_t)0x5FAUL << SCB_AIRCR_VECTKEY_Pos) | 02479 (PriorityGroupTmp << 8U) ); /* Insert write key and priorty group */ 02480 SCB_NS->AIRCR = reg_value; 02481 } 02482 02483 02484 /** 02485 \brief Get Priority Grouping (non-secure) 02486 \details Reads the priority grouping field from the non-secure NVIC when in secure state. 02487 \return Priority grouping field (SCB->AIRCR [10:8] PRIGROUP field). 02488 */ 02489 __STATIC_INLINE uint32_t TZ_NVIC_GetPriorityGrouping_NS(void) 02490 { 02491 return ((uint32_t)((SCB_NS->AIRCR & SCB_AIRCR_PRIGROUP_Msk) >> SCB_AIRCR_PRIGROUP_Pos)); 02492 } 02493 02494 02495 /** 02496 \brief Enable Interrupt (non-secure) 02497 \details Enables a device specific interrupt in the non-secure NVIC interrupt controller when in secure state. 02498 \param [in] IRQn Device specific interrupt number. 02499 \note IRQn must not be negative. 02500 */ 02501 __STATIC_INLINE void TZ_NVIC_EnableIRQ_NS(IRQn_Type IRQn) 02502 { 02503 if ((int32_t)(IRQn) >= 0) 02504 { 02505 NVIC_NS->ISER[(((uint32_t)(int32_t)IRQn) >> 5UL)] = (uint32_t)(1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL)); 02506 } 02507 } 02508 02509 02510 /** 02511 \brief Get Interrupt Enable status (non-secure) 02512 \details Returns a device specific interrupt enable status from the non-secure NVIC interrupt controller when in secure state. 02513 \param [in] IRQn Device specific interrupt number. 02514 \return 0 Interrupt is not enabled. 02515 \return 1 Interrupt is enabled. 02516 \note IRQn must not be negative. 02517 */ 02518 __STATIC_INLINE uint32_t TZ_NVIC_GetEnableIRQ_NS(IRQn_Type IRQn) 02519 { 02520 if ((int32_t)(IRQn) >= 0) 02521 { 02522 return((uint32_t)(((NVIC_NS->ISER[(((uint32_t)(int32_t)IRQn) >> 5UL)] & (1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL))) != 0UL) ? 1UL : 0UL)); 02523 } 02524 else 02525 { 02526 return(0U); 02527 } 02528 } 02529 02530 02531 /** 02532 \brief Disable Interrupt (non-secure) 02533 \details Disables a device specific interrupt in the non-secure NVIC interrupt controller when in secure state. 02534 \param [in] IRQn Device specific interrupt number. 02535 \note IRQn must not be negative. 02536 */ 02537 __STATIC_INLINE void TZ_NVIC_DisableIRQ_NS(IRQn_Type IRQn) 02538 { 02539 if ((int32_t)(IRQn) >= 0) 02540 { 02541 NVIC_NS->ICER[(((uint32_t)(int32_t)IRQn) >> 5UL)] = (uint32_t)(1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL)); 02542 } 02543 } 02544 02545 02546 /** 02547 \brief Get Pending Interrupt (non-secure) 02548 \details Reads the NVIC pending register in the non-secure NVIC when in secure state and returns the pending bit for the specified device specific interrupt. 02549 \param [in] IRQn Device specific interrupt number. 02550 \return 0 Interrupt status is not pending. 02551 \return 1 Interrupt status is pending. 02552 \note IRQn must not be negative. 02553 */ 02554 __STATIC_INLINE uint32_t TZ_NVIC_GetPendingIRQ_NS(IRQn_Type IRQn) 02555 { 02556 if ((int32_t)(IRQn) >= 0) 02557 { 02558 return((uint32_t)(((NVIC_NS->ISPR[(((uint32_t)(int32_t)IRQn) >> 5UL)] & (1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL))) != 0UL) ? 1UL : 0UL)); 02559 } 02560 } 02561 02562 02563 /** 02564 \brief Set Pending Interrupt (non-secure) 02565 \details Sets the pending bit of a device specific interrupt in the non-secure NVIC pending register when in secure state. 02566 \param [in] IRQn Device specific interrupt number. 02567 \note IRQn must not be negative. 02568 */ 02569 __STATIC_INLINE void TZ_NVIC_SetPendingIRQ_NS(IRQn_Type IRQn) 02570 { 02571 if ((int32_t)(IRQn) >= 0) 02572 { 02573 NVIC_NS->ISPR[(((uint32_t)(int32_t)IRQn) >> 5UL)] = (uint32_t)(1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL)); 02574 } 02575 } 02576 02577 02578 /** 02579 \brief Clear Pending Interrupt (non-secure) 02580 \details Clears the pending bit of a device specific interrupt in the non-secure NVIC pending register when in secure state. 02581 \param [in] IRQn Device specific interrupt number. 02582 \note IRQn must not be negative. 02583 */ 02584 __STATIC_INLINE void TZ_NVIC_ClearPendingIRQ_NS(IRQn_Type IRQn) 02585 { 02586 if ((int32_t)(IRQn) >= 0) 02587 { 02588 NVIC_NS->ICPR[(((uint32_t)(int32_t)IRQn) >> 5UL)] = (uint32_t)(1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL)); 02589 } 02590 } 02591 02592 02593 /** 02594 \brief Get Active Interrupt (non-secure) 02595 \details Reads the active register in non-secure NVIC when in secure state and returns the active bit for the device specific interrupt. 02596 \param [in] IRQn Device specific interrupt number. 02597 \return 0 Interrupt status is not active. 02598 \return 1 Interrupt status is active. 02599 \note IRQn must not be negative. 02600 */ 02601 __STATIC_INLINE uint32_t TZ_NVIC_GetActive_NS(IRQn_Type IRQn) 02602 { 02603 if ((int32_t)(IRQn) >= 0) 02604 { 02605 return((uint32_t)(((NVIC_NS->IABR[(((uint32_t)(int32_t)IRQn) >> 5UL)] & (1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL))) != 0UL) ? 1UL : 0UL)); 02606 } 02607 else 02608 { 02609 return(0U); 02610 } 02611 } 02612 02613 02614 /** 02615 \brief Set Interrupt Priority (non-secure) 02616 \details Sets the priority of a non-secure device specific interrupt or a non-secure processor exception when in secure state. 02617 The interrupt number can be positive to specify a device specific interrupt, 02618 or negative to specify a processor exception. 02619 \param [in] IRQn Interrupt number. 02620 \param [in] priority Priority to set. 02621 \note The priority cannot be set for every non-secure processor exception. 02622 */ 02623 __STATIC_INLINE void TZ_NVIC_SetPriority_NS(IRQn_Type IRQn, uint32_t priority) 02624 { 02625 if ((int32_t)(IRQn) >= 0) 02626 { 02627 NVIC_NS->IPR[((uint32_t)(int32_t)IRQn)] = (uint8_t)((priority << (8U - __NVIC_PRIO_BITS)) & (uint32_t)0xFFUL); 02628 } 02629 else 02630 { 02631 SCB_NS->SHPR[(((uint32_t)(int32_t)IRQn) & 0xFUL)-4UL] = (uint8_t)((priority << (8U - __NVIC_PRIO_BITS)) & (uint32_t)0xFFUL); 02632 } 02633 } 02634 02635 02636 /** 02637 \brief Get Interrupt Priority (non-secure) 02638 \details Reads the priority of a non-secure device specific interrupt or a non-secure processor exception when in secure state. 02639 The interrupt number can be positive to specify a device specific interrupt, 02640 or negative to specify a processor exception. 02641 \param [in] IRQn Interrupt number. 02642 \return Interrupt Priority. Value is aligned automatically to the implemented priority bits of the microcontroller. 02643 */ 02644 __STATIC_INLINE uint32_t TZ_NVIC_GetPriority_NS(IRQn_Type IRQn) 02645 { 02646 02647 if ((int32_t)(IRQn) >= 0) 02648 { 02649 return(((uint32_t)NVIC_NS->IPR[((uint32_t)(int32_t)IRQn)] >> (8U - __NVIC_PRIO_BITS))); 02650 } 02651 else 02652 { 02653 return(((uint32_t)SCB_NS->SHPR[(((uint32_t)(int32_t)IRQn) & 0xFUL)-4UL] >> (8U - __NVIC_PRIO_BITS))); 02654 } 02655 } 02656 #endif /* defined (__ARM_FEATURE_CMSE) &&(__ARM_FEATURE_CMSE == 3U) */ 02657 02658 /*@} end of CMSIS_Core_NVICFunctions */ 02659 02660 02661 /* ########################## FPU functions #################################### */ 02662 /** 02663 \ingroup CMSIS_Core_FunctionInterface 02664 \defgroup CMSIS_Core_FpuFunctions FPU Functions 02665 \brief Function that provides FPU type. 02666 @{ 02667 */ 02668 02669 /** 02670 \brief get FPU type 02671 \details returns the FPU type 02672 \returns 02673 - \b 0: No FPU 02674 - \b 1: Single precision FPU 02675 - \b 2: Double + Single precision FPU 02676 */ 02677 __STATIC_INLINE uint32_t SCB_GetFPUType(void) 02678 { 02679 uint32_t mvfr0; 02680 02681 mvfr0 = FPU->MVFR0; 02682 if ((mvfr0 & (FPU_MVFR0_Single_precision_Msk | FPU_MVFR0_Double_precision_Msk)) == 0x220U) 02683 { 02684 return 2U; /* Double + Single precision FPU */ 02685 } 02686 else if ((mvfr0 & (FPU_MVFR0_Single_precision_Msk | FPU_MVFR0_Double_precision_Msk)) == 0x020U) 02687 { 02688 return 1U; /* Single precision FPU */ 02689 } 02690 else 02691 { 02692 return 0U; /* No FPU */ 02693 } 02694 } 02695 02696 02697 /*@} end of CMSIS_Core_FpuFunctions */ 02698 02699 02700 02701 /* ########################## SAU functions #################################### */ 02702 /** 02703 \ingroup CMSIS_Core_FunctionInterface 02704 \defgroup CMSIS_Core_SAUFunctions SAU Functions 02705 \brief Functions that configure the SAU. 02706 @{ 02707 */ 02708 02709 #if defined (__ARM_FEATURE_CMSE) && (__ARM_FEATURE_CMSE == 3U) 02710 02711 /** 02712 \brief Enable SAU 02713 \details Enables the Security Attribution Unit (SAU). 02714 */ 02715 __STATIC_INLINE void TZ_SAU_Enable(void) 02716 { 02717 SAU->CTRL |= (SAU_CTRL_ENABLE_Msk); 02718 } 02719 02720 02721 02722 /** 02723 \brief Disable SAU 02724 \details Disables the Security Attribution Unit (SAU). 02725 */ 02726 __STATIC_INLINE void TZ_SAU_Disable(void) 02727 { 02728 SAU->CTRL &= ~(SAU_CTRL_ENABLE_Msk); 02729 } 02730 02731 #endif /* defined (__ARM_FEATURE_CMSE) && (__ARM_FEATURE_CMSE == 3U) */ 02732 02733 /*@} end of CMSIS_Core_SAUFunctions */ 02734 02735 02736 02737 02738 /* ################################## SysTick function ############################################ */ 02739 /** 02740 \ingroup CMSIS_Core_FunctionInterface 02741 \defgroup CMSIS_Core_SysTickFunctions SysTick Functions 02742 \brief Functions that configure the System. 02743 @{ 02744 */ 02745 02746 #if defined (__Vendor_SysTickConfig) && (__Vendor_SysTickConfig == 0U) 02747 02748 /** 02749 \brief System Tick Configuration 02750 \details Initializes the System Timer and its interrupt, and starts the System Tick Timer. 02751 Counter is in free running mode to generate periodic interrupts. 02752 \param [in] ticks Number of ticks between two interrupts. 02753 \return 0 Function succeeded. 02754 \return 1 Function failed. 02755 \note When the variable <b>__Vendor_SysTickConfig</b> is set to 1, then the 02756 function <b>SysTick_Config</b> is not included. In this case, the file <b><i>device</i>.h</b> 02757 must contain a vendor-specific implementation of this function. 02758 */ 02759 __STATIC_INLINE uint32_t SysTick_Config(uint32_t ticks) 02760 { 02761 if ((ticks - 1UL) > SysTick_LOAD_RELOAD_Msk) 02762 { 02763 return (1UL); /* Reload value impossible */ 02764 } 02765 02766 SysTick->LOAD = (uint32_t)(ticks - 1UL); /* set reload register */ 02767 NVIC_SetPriority (SysTick_IRQn, (1UL << __NVIC_PRIO_BITS) - 1UL); /* set Priority for Systick Interrupt */ 02768 SysTick->VAL = 0UL; /* Load the SysTick Counter Value */ 02769 SysTick->CTRL = SysTick_CTRL_CLKSOURCE_Msk | 02770 SysTick_CTRL_TICKINT_Msk | 02771 SysTick_CTRL_ENABLE_Msk; /* Enable SysTick IRQ and SysTick Timer */ 02772 return (0UL); /* Function successful */ 02773 } 02774 02775 #if defined (__ARM_FEATURE_CMSE) && (__ARM_FEATURE_CMSE == 3U) 02776 /** 02777 \brief System Tick Configuration (non-secure) 02778 \details Initializes the non-secure System Timer and its interrupt when in secure state, and starts the System Tick Timer. 02779 Counter is in free running mode to generate periodic interrupts. 02780 \param [in] ticks Number of ticks between two interrupts. 02781 \return 0 Function succeeded. 02782 \return 1 Function failed. 02783 \note When the variable <b>__Vendor_SysTickConfig</b> is set to 1, then the 02784 function <b>TZ_SysTick_Config_NS</b> is not included. In this case, the file <b><i>device</i>.h</b> 02785 must contain a vendor-specific implementation of this function. 02786 02787 */ 02788 __STATIC_INLINE uint32_t TZ_SysTick_Config_NS(uint32_t ticks) 02789 { 02790 if ((ticks - 1UL) > SysTick_LOAD_RELOAD_Msk) 02791 { 02792 return (1UL); /* Reload value impossible */ 02793 } 02794 02795 SysTick_NS->LOAD = (uint32_t)(ticks - 1UL); /* set reload register */ 02796 TZ_NVIC_SetPriority_NS (SysTick_IRQn, (1UL << __NVIC_PRIO_BITS) - 1UL); /* set Priority for Systick Interrupt */ 02797 SysTick_NS->VAL = 0UL; /* Load the SysTick Counter Value */ 02798 SysTick_NS->CTRL = SysTick_CTRL_CLKSOURCE_Msk | 02799 SysTick_CTRL_TICKINT_Msk | 02800 SysTick_CTRL_ENABLE_Msk; /* Enable SysTick IRQ and SysTick Timer */ 02801 return (0UL); /* Function successful */ 02802 } 02803 #endif /* defined (__ARM_FEATURE_CMSE) && (__ARM_FEATURE_CMSE == 3U) */ 02804 02805 #endif 02806 02807 /*@} end of CMSIS_Core_SysTickFunctions */ 02808 02809 02810 02811 /* ##################################### Debug In/Output function ########################################### */ 02812 /** 02813 \ingroup CMSIS_Core_FunctionInterface 02814 \defgroup CMSIS_core_DebugFunctions ITM Functions 02815 \brief Functions that access the ITM debug interface. 02816 @{ 02817 */ 02818 02819 extern volatile int32_t ITM_RxBuffer; /*!< External variable to receive characters. */ 02820 #define ITM_RXBUFFER_EMPTY ((int32_t)0x5AA55AA5U) /*!< Value identifying \ref ITM_RxBuffer is ready for next character. */ 02821 02822 02823 /** 02824 \brief ITM Send Character 02825 \details Transmits a character via the ITM channel 0, and 02826 \li Just returns when no debugger is connected that has booked the output. 02827 \li Is blocking when a debugger is connected, but the previous character sent has not been transmitted. 02828 \param [in] ch Character to transmit. 02829 \returns Character to transmit. 02830 */ 02831 __STATIC_INLINE uint32_t ITM_SendChar (uint32_t ch) 02832 { 02833 if (((ITM->TCR & ITM_TCR_ITMENA_Msk) != 0UL) && /* ITM enabled */ 02834 ((ITM->TER & 1UL ) != 0UL) ) /* ITM Port #0 enabled */ 02835 { 02836 while (ITM->PORT[0U].u32 == 0UL) 02837 { 02838 __NOP(); 02839 } 02840 ITM->PORT[0U].u8 = (uint8_t)ch; 02841 } 02842 return (ch); 02843 } 02844 02845 02846 /** 02847 \brief ITM Receive Character 02848 \details Inputs a character via the external variable \ref ITM_RxBuffer. 02849 \return Received character. 02850 \return -1 No character pending. 02851 */ 02852 __STATIC_INLINE int32_t ITM_ReceiveChar (void) 02853 { 02854 int32_t ch = -1; /* no character available */ 02855 02856 if (ITM_RxBuffer != ITM_RXBUFFER_EMPTY) 02857 { 02858 ch = ITM_RxBuffer; 02859 ITM_RxBuffer = ITM_RXBUFFER_EMPTY; /* ready for next character */ 02860 } 02861 02862 return (ch); 02863 } 02864 02865 02866 /** 02867 \brief ITM Check Character 02868 \details Checks whether a character is pending for reading in the variable \ref ITM_RxBuffer. 02869 \return 0 No character available. 02870 \return 1 Character available. 02871 */ 02872 __STATIC_INLINE int32_t ITM_CheckChar (void) 02873 { 02874 02875 if (ITM_RxBuffer == ITM_RXBUFFER_EMPTY) 02876 { 02877 return (0); /* no character available */ 02878 } 02879 else 02880 { 02881 return (1); /* character available */ 02882 } 02883 } 02884 02885 /*@} end of CMSIS_core_DebugFunctions */ 02886 02887 02888 02889 02890 #ifdef __cplusplus 02891 } 02892 #endif 02893 02894 #endif /* __CORE_CM33_H_DEPENDANT */ 02895 02896 #endif /* __CMSIS_GENERIC */
Generated on Tue Jul 12 2022 16:02:32 by
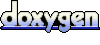