forked
Embed:
(wiki syntax)
Show/hide line numbers
core_cm0plus.h
Go to the documentation of this file.
00001 /**************************************************************************//** 00002 * @file core_cm0plus.h 00003 * @brief CMSIS Cortex-M0+ Core Peripheral Access Layer Header File 00004 * @version V5.0.2 00005 * @date 13. February 2017 00006 ******************************************************************************/ 00007 /* 00008 * Copyright (c) 2009-2017 ARM Limited. All rights reserved. 00009 * 00010 * SPDX-License-Identifier: Apache-2.0 00011 * 00012 * Licensed under the Apache License, Version 2.0 (the License); you may 00013 * not use this file except in compliance with the License. 00014 * You may obtain a copy of the License at 00015 * 00016 * www.apache.org/licenses/LICENSE-2.0 00017 * 00018 * Unless required by applicable law or agreed to in writing, software 00019 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00020 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00021 * See the License for the specific language governing permissions and 00022 * limitations under the License. 00023 */ 00024 00025 #if defined ( __ICCARM__ ) 00026 #pragma system_include /* treat file as system include file for MISRA check */ 00027 #elif defined (__ARMCC_VERSION) && (__ARMCC_VERSION >= 6010050) 00028 #pragma clang system_header /* treat file as system include file */ 00029 #endif 00030 00031 #ifndef __CORE_CM0PLUS_H_GENERIC 00032 #define __CORE_CM0PLUS_H_GENERIC 00033 00034 #include <stdint.h> 00035 00036 #ifdef __cplusplus 00037 extern "C" { 00038 #endif 00039 00040 /** 00041 \page CMSIS_MISRA_Exceptions MISRA-C:2004 Compliance Exceptions 00042 CMSIS violates the following MISRA-C:2004 rules: 00043 00044 \li Required Rule 8.5, object/function definition in header file.<br> 00045 Function definitions in header files are used to allow 'inlining'. 00046 00047 \li Required Rule 18.4, declaration of union type or object of union type: '{...}'.<br> 00048 Unions are used for effective representation of core registers. 00049 00050 \li Advisory Rule 19.7, Function-like macro defined.<br> 00051 Function-like macros are used to allow more efficient code. 00052 */ 00053 00054 00055 /******************************************************************************* 00056 * CMSIS definitions 00057 ******************************************************************************/ 00058 /** 00059 \ingroup Cortex-M0+ 00060 @{ 00061 */ 00062 00063 /* CMSIS CM0+ definitions */ 00064 #define __CM0PLUS_CMSIS_VERSION_MAIN ( 5U) /*!< [31:16] CMSIS HAL main version */ 00065 #define __CM0PLUS_CMSIS_VERSION_SUB ( 0U) /*!< [15:0] CMSIS HAL sub version */ 00066 #define __CM0PLUS_CMSIS_VERSION ((__CM0PLUS_CMSIS_VERSION_MAIN << 16U) | \ 00067 __CM0PLUS_CMSIS_VERSION_SUB ) /*!< CMSIS HAL version number */ 00068 00069 #define __CORTEX_M (0U) /*!< Cortex-M Core */ 00070 00071 /** __FPU_USED indicates whether an FPU is used or not. 00072 This core does not support an FPU at all 00073 */ 00074 #define __FPU_USED 0U 00075 00076 #if defined ( __CC_ARM ) 00077 #if defined __TARGET_FPU_VFP 00078 #error "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00079 #endif 00080 00081 #elif defined (__ARMCC_VERSION) && (__ARMCC_VERSION >= 6010050) 00082 #if defined __ARM_PCS_VFP 00083 #error "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00084 #endif 00085 00086 #elif defined ( __GNUC__ ) 00087 #if defined (__VFP_FP__) && !defined(__SOFTFP__) 00088 #error "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00089 #endif 00090 00091 #elif defined ( __ICCARM__ ) 00092 #if defined __ARMVFP__ 00093 #error "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00094 #endif 00095 00096 #elif defined ( __TI_ARM__ ) 00097 #if defined __TI_VFP_SUPPORT__ 00098 #error "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00099 #endif 00100 00101 #elif defined ( __TASKING__ ) 00102 #if defined __FPU_VFP__ 00103 #error "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00104 #endif 00105 00106 #elif defined ( __CSMC__ ) 00107 #if ( __CSMC__ & 0x400U) 00108 #error "Compiler generates FPU instructions for a device without an FPU (check __FPU_PRESENT)" 00109 #endif 00110 00111 #endif 00112 00113 #include "cmsis_compiler.h" /* CMSIS compiler specific defines */ 00114 00115 00116 #ifdef __cplusplus 00117 } 00118 #endif 00119 00120 #endif /* __CORE_CM0PLUS_H_GENERIC */ 00121 00122 #ifndef __CMSIS_GENERIC 00123 00124 #ifndef __CORE_CM0PLUS_H_DEPENDANT 00125 #define __CORE_CM0PLUS_H_DEPENDANT 00126 00127 #ifdef __cplusplus 00128 extern "C" { 00129 #endif 00130 00131 /* check device defines and use defaults */ 00132 #if defined __CHECK_DEVICE_DEFINES 00133 #ifndef __CM0PLUS_REV 00134 #define __CM0PLUS_REV 0x0000U 00135 #warning "__CM0PLUS_REV not defined in device header file; using default!" 00136 #endif 00137 00138 #ifndef __MPU_PRESENT 00139 #define __MPU_PRESENT 0U 00140 #warning "__MPU_PRESENT not defined in device header file; using default!" 00141 #endif 00142 00143 #ifndef __VTOR_PRESENT 00144 #define __VTOR_PRESENT 0U 00145 #warning "__VTOR_PRESENT not defined in device header file; using default!" 00146 #endif 00147 00148 #ifndef __NVIC_PRIO_BITS 00149 #define __NVIC_PRIO_BITS 2U 00150 #warning "__NVIC_PRIO_BITS not defined in device header file; using default!" 00151 #endif 00152 00153 #ifndef __Vendor_SysTickConfig 00154 #define __Vendor_SysTickConfig 0U 00155 #warning "__Vendor_SysTickConfig not defined in device header file; using default!" 00156 #endif 00157 #endif 00158 00159 /* IO definitions (access restrictions to peripheral registers) */ 00160 /** 00161 \defgroup CMSIS_glob_defs CMSIS Global Defines 00162 00163 <strong>IO Type Qualifiers</strong> are used 00164 \li to specify the access to peripheral variables. 00165 \li for automatic generation of peripheral register debug information. 00166 */ 00167 #ifdef __cplusplus 00168 #define __I volatile /*!< Defines 'read only' permissions */ 00169 #else 00170 #define __I volatile const /*!< Defines 'read only' permissions */ 00171 #endif 00172 #define __O volatile /*!< Defines 'write only' permissions */ 00173 #define __IO volatile /*!< Defines 'read / write' permissions */ 00174 00175 /* following defines should be used for structure members */ 00176 #define __IM volatile const /*! Defines 'read only' structure member permissions */ 00177 #define __OM volatile /*! Defines 'write only' structure member permissions */ 00178 #define __IOM volatile /*! Defines 'read / write' structure member permissions */ 00179 00180 /*@} end of group Cortex-M0+ */ 00181 00182 00183 00184 /******************************************************************************* 00185 * Register Abstraction 00186 Core Register contain: 00187 - Core Register 00188 - Core NVIC Register 00189 - Core SCB Register 00190 - Core SysTick Register 00191 - Core MPU Register 00192 ******************************************************************************/ 00193 /** 00194 \defgroup CMSIS_core_register Defines and Type Definitions 00195 \brief Type definitions and defines for Cortex-M processor based devices. 00196 */ 00197 00198 /** 00199 \ingroup CMSIS_core_register 00200 \defgroup CMSIS_CORE Status and Control Registers 00201 \brief Core Register type definitions. 00202 @{ 00203 */ 00204 00205 /** 00206 \brief Union type to access the Application Program Status Register (APSR). 00207 */ 00208 typedef union 00209 { 00210 struct 00211 { 00212 uint32_t _reserved0:28; /*!< bit: 0..27 Reserved */ 00213 uint32_t V:1; /*!< bit: 28 Overflow condition code flag */ 00214 uint32_t C:1; /*!< bit: 29 Carry condition code flag */ 00215 uint32_t Z:1; /*!< bit: 30 Zero condition code flag */ 00216 uint32_t N:1; /*!< bit: 31 Negative condition code flag */ 00217 } b; /*!< Structure used for bit access */ 00218 uint32_t w; /*!< Type used for word access */ 00219 } APSR_Type; 00220 00221 /* APSR Register Definitions */ 00222 #define APSR_N_Pos 31U /*!< APSR: N Position */ 00223 #define APSR_N_Msk (1UL << APSR_N_Pos) /*!< APSR: N Mask */ 00224 00225 #define APSR_Z_Pos 30U /*!< APSR: Z Position */ 00226 #define APSR_Z_Msk (1UL << APSR_Z_Pos) /*!< APSR: Z Mask */ 00227 00228 #define APSR_C_Pos 29U /*!< APSR: C Position */ 00229 #define APSR_C_Msk (1UL << APSR_C_Pos) /*!< APSR: C Mask */ 00230 00231 #define APSR_V_Pos 28U /*!< APSR: V Position */ 00232 #define APSR_V_Msk (1UL << APSR_V_Pos) /*!< APSR: V Mask */ 00233 00234 00235 /** 00236 \brief Union type to access the Interrupt Program Status Register (IPSR). 00237 */ 00238 typedef union 00239 { 00240 struct 00241 { 00242 uint32_t ISR:9; /*!< bit: 0.. 8 Exception number */ 00243 uint32_t _reserved0:23; /*!< bit: 9..31 Reserved */ 00244 } b; /*!< Structure used for bit access */ 00245 uint32_t w; /*!< Type used for word access */ 00246 } IPSR_Type; 00247 00248 /* IPSR Register Definitions */ 00249 #define IPSR_ISR_Pos 0U /*!< IPSR: ISR Position */ 00250 #define IPSR_ISR_Msk (0x1FFUL /*<< IPSR_ISR_Pos*/) /*!< IPSR: ISR Mask */ 00251 00252 00253 /** 00254 \brief Union type to access the Special-Purpose Program Status Registers (xPSR). 00255 */ 00256 typedef union 00257 { 00258 struct 00259 { 00260 uint32_t ISR:9; /*!< bit: 0.. 8 Exception number */ 00261 uint32_t _reserved0:15; /*!< bit: 9..23 Reserved */ 00262 uint32_t T:1; /*!< bit: 24 Thumb bit (read 0) */ 00263 uint32_t _reserved1:3; /*!< bit: 25..27 Reserved */ 00264 uint32_t V:1; /*!< bit: 28 Overflow condition code flag */ 00265 uint32_t C:1; /*!< bit: 29 Carry condition code flag */ 00266 uint32_t Z:1; /*!< bit: 30 Zero condition code flag */ 00267 uint32_t N:1; /*!< bit: 31 Negative condition code flag */ 00268 } b; /*!< Structure used for bit access */ 00269 uint32_t w; /*!< Type used for word access */ 00270 } xPSR_Type; 00271 00272 /* xPSR Register Definitions */ 00273 #define xPSR_N_Pos 31U /*!< xPSR: N Position */ 00274 #define xPSR_N_Msk (1UL << xPSR_N_Pos) /*!< xPSR: N Mask */ 00275 00276 #define xPSR_Z_Pos 30U /*!< xPSR: Z Position */ 00277 #define xPSR_Z_Msk (1UL << xPSR_Z_Pos) /*!< xPSR: Z Mask */ 00278 00279 #define xPSR_C_Pos 29U /*!< xPSR: C Position */ 00280 #define xPSR_C_Msk (1UL << xPSR_C_Pos) /*!< xPSR: C Mask */ 00281 00282 #define xPSR_V_Pos 28U /*!< xPSR: V Position */ 00283 #define xPSR_V_Msk (1UL << xPSR_V_Pos) /*!< xPSR: V Mask */ 00284 00285 #define xPSR_T_Pos 24U /*!< xPSR: T Position */ 00286 #define xPSR_T_Msk (1UL << xPSR_T_Pos) /*!< xPSR: T Mask */ 00287 00288 #define xPSR_ISR_Pos 0U /*!< xPSR: ISR Position */ 00289 #define xPSR_ISR_Msk (0x1FFUL /*<< xPSR_ISR_Pos*/) /*!< xPSR: ISR Mask */ 00290 00291 00292 /** 00293 \brief Union type to access the Control Registers (CONTROL). 00294 */ 00295 typedef union 00296 { 00297 struct 00298 { 00299 uint32_t nPRIV:1; /*!< bit: 0 Execution privilege in Thread mode */ 00300 uint32_t SPSEL:1; /*!< bit: 1 Stack to be used */ 00301 uint32_t _reserved1:30; /*!< bit: 2..31 Reserved */ 00302 } b; /*!< Structure used for bit access */ 00303 uint32_t w; /*!< Type used for word access */ 00304 } CONTROL_Type; 00305 00306 /* CONTROL Register Definitions */ 00307 #define CONTROL_SPSEL_Pos 1U /*!< CONTROL: SPSEL Position */ 00308 #define CONTROL_SPSEL_Msk (1UL << CONTROL_SPSEL_Pos) /*!< CONTROL: SPSEL Mask */ 00309 00310 #define CONTROL_nPRIV_Pos 0U /*!< CONTROL: nPRIV Position */ 00311 #define CONTROL_nPRIV_Msk (1UL /*<< CONTROL_nPRIV_Pos*/) /*!< CONTROL: nPRIV Mask */ 00312 00313 /*@} end of group CMSIS_CORE */ 00314 00315 00316 /** 00317 \ingroup CMSIS_core_register 00318 \defgroup CMSIS_NVIC Nested Vectored Interrupt Controller (NVIC) 00319 \brief Type definitions for the NVIC Registers 00320 @{ 00321 */ 00322 00323 /** 00324 \brief Structure type to access the Nested Vectored Interrupt Controller (NVIC). 00325 */ 00326 typedef struct 00327 { 00328 __IOM uint32_t ISER[1U]; /*!< Offset: 0x000 (R/W) Interrupt Set Enable Register */ 00329 uint32_t RESERVED0[31U]; 00330 __IOM uint32_t ICER[1U]; /*!< Offset: 0x080 (R/W) Interrupt Clear Enable Register */ 00331 uint32_t RSERVED1[31U]; 00332 __IOM uint32_t ISPR[1U]; /*!< Offset: 0x100 (R/W) Interrupt Set Pending Register */ 00333 uint32_t RESERVED2[31U]; 00334 __IOM uint32_t ICPR[1U]; /*!< Offset: 0x180 (R/W) Interrupt Clear Pending Register */ 00335 uint32_t RESERVED3[31U]; 00336 uint32_t RESERVED4[64U]; 00337 __IOM uint32_t IP[8U]; /*!< Offset: 0x300 (R/W) Interrupt Priority Register */ 00338 } NVIC_Type; 00339 00340 /*@} end of group CMSIS_NVIC */ 00341 00342 00343 /** 00344 \ingroup CMSIS_core_register 00345 \defgroup CMSIS_SCB System Control Block (SCB) 00346 \brief Type definitions for the System Control Block Registers 00347 @{ 00348 */ 00349 00350 /** 00351 \brief Structure type to access the System Control Block (SCB). 00352 */ 00353 typedef struct 00354 { 00355 __IM uint32_t CPUID; /*!< Offset: 0x000 (R/ ) CPUID Base Register */ 00356 __IOM uint32_t ICSR; /*!< Offset: 0x004 (R/W) Interrupt Control and State Register */ 00357 #if defined (__VTOR_PRESENT) && (__VTOR_PRESENT == 1U) 00358 __IOM uint32_t VTOR; /*!< Offset: 0x008 (R/W) Vector Table Offset Register */ 00359 #else 00360 uint32_t RESERVED0; 00361 #endif 00362 __IOM uint32_t AIRCR; /*!< Offset: 0x00C (R/W) Application Interrupt and Reset Control Register */ 00363 __IOM uint32_t SCR; /*!< Offset: 0x010 (R/W) System Control Register */ 00364 __IOM uint32_t CCR; /*!< Offset: 0x014 (R/W) Configuration Control Register */ 00365 uint32_t RESERVED1; 00366 __IOM uint32_t SHP[2U]; /*!< Offset: 0x01C (R/W) System Handlers Priority Registers. [0] is RESERVED */ 00367 __IOM uint32_t SHCSR; /*!< Offset: 0x024 (R/W) System Handler Control and State Register */ 00368 } SCB_Type; 00369 00370 /* SCB CPUID Register Definitions */ 00371 #define SCB_CPUID_IMPLEMENTER_Pos 24U /*!< SCB CPUID: IMPLEMENTER Position */ 00372 #define SCB_CPUID_IMPLEMENTER_Msk (0xFFUL << SCB_CPUID_IMPLEMENTER_Pos) /*!< SCB CPUID: IMPLEMENTER Mask */ 00373 00374 #define SCB_CPUID_VARIANT_Pos 20U /*!< SCB CPUID: VARIANT Position */ 00375 #define SCB_CPUID_VARIANT_Msk (0xFUL << SCB_CPUID_VARIANT_Pos) /*!< SCB CPUID: VARIANT Mask */ 00376 00377 #define SCB_CPUID_ARCHITECTURE_Pos 16U /*!< SCB CPUID: ARCHITECTURE Position */ 00378 #define SCB_CPUID_ARCHITECTURE_Msk (0xFUL << SCB_CPUID_ARCHITECTURE_Pos) /*!< SCB CPUID: ARCHITECTURE Mask */ 00379 00380 #define SCB_CPUID_PARTNO_Pos 4U /*!< SCB CPUID: PARTNO Position */ 00381 #define SCB_CPUID_PARTNO_Msk (0xFFFUL << SCB_CPUID_PARTNO_Pos) /*!< SCB CPUID: PARTNO Mask */ 00382 00383 #define SCB_CPUID_REVISION_Pos 0U /*!< SCB CPUID: REVISION Position */ 00384 #define SCB_CPUID_REVISION_Msk (0xFUL /*<< SCB_CPUID_REVISION_Pos*/) /*!< SCB CPUID: REVISION Mask */ 00385 00386 /* SCB Interrupt Control State Register Definitions */ 00387 #define SCB_ICSR_NMIPENDSET_Pos 31U /*!< SCB ICSR: NMIPENDSET Position */ 00388 #define SCB_ICSR_NMIPENDSET_Msk (1UL << SCB_ICSR_NMIPENDSET_Pos) /*!< SCB ICSR: NMIPENDSET Mask */ 00389 00390 #define SCB_ICSR_PENDSVSET_Pos 28U /*!< SCB ICSR: PENDSVSET Position */ 00391 #define SCB_ICSR_PENDSVSET_Msk (1UL << SCB_ICSR_PENDSVSET_Pos) /*!< SCB ICSR: PENDSVSET Mask */ 00392 00393 #define SCB_ICSR_PENDSVCLR_Pos 27U /*!< SCB ICSR: PENDSVCLR Position */ 00394 #define SCB_ICSR_PENDSVCLR_Msk (1UL << SCB_ICSR_PENDSVCLR_Pos) /*!< SCB ICSR: PENDSVCLR Mask */ 00395 00396 #define SCB_ICSR_PENDSTSET_Pos 26U /*!< SCB ICSR: PENDSTSET Position */ 00397 #define SCB_ICSR_PENDSTSET_Msk (1UL << SCB_ICSR_PENDSTSET_Pos) /*!< SCB ICSR: PENDSTSET Mask */ 00398 00399 #define SCB_ICSR_PENDSTCLR_Pos 25U /*!< SCB ICSR: PENDSTCLR Position */ 00400 #define SCB_ICSR_PENDSTCLR_Msk (1UL << SCB_ICSR_PENDSTCLR_Pos) /*!< SCB ICSR: PENDSTCLR Mask */ 00401 00402 #define SCB_ICSR_ISRPREEMPT_Pos 23U /*!< SCB ICSR: ISRPREEMPT Position */ 00403 #define SCB_ICSR_ISRPREEMPT_Msk (1UL << SCB_ICSR_ISRPREEMPT_Pos) /*!< SCB ICSR: ISRPREEMPT Mask */ 00404 00405 #define SCB_ICSR_ISRPENDING_Pos 22U /*!< SCB ICSR: ISRPENDING Position */ 00406 #define SCB_ICSR_ISRPENDING_Msk (1UL << SCB_ICSR_ISRPENDING_Pos) /*!< SCB ICSR: ISRPENDING Mask */ 00407 00408 #define SCB_ICSR_VECTPENDING_Pos 12U /*!< SCB ICSR: VECTPENDING Position */ 00409 #define SCB_ICSR_VECTPENDING_Msk (0x1FFUL << SCB_ICSR_VECTPENDING_Pos) /*!< SCB ICSR: VECTPENDING Mask */ 00410 00411 #define SCB_ICSR_VECTACTIVE_Pos 0U /*!< SCB ICSR: VECTACTIVE Position */ 00412 #define SCB_ICSR_VECTACTIVE_Msk (0x1FFUL /*<< SCB_ICSR_VECTACTIVE_Pos*/) /*!< SCB ICSR: VECTACTIVE Mask */ 00413 00414 #if defined (__VTOR_PRESENT) && (__VTOR_PRESENT == 1U) 00415 /* SCB Interrupt Control State Register Definitions */ 00416 #define SCB_VTOR_TBLOFF_Pos 8U /*!< SCB VTOR: TBLOFF Position */ 00417 #define SCB_VTOR_TBLOFF_Msk (0xFFFFFFUL << SCB_VTOR_TBLOFF_Pos) /*!< SCB VTOR: TBLOFF Mask */ 00418 #endif 00419 00420 /* SCB Application Interrupt and Reset Control Register Definitions */ 00421 #define SCB_AIRCR_VECTKEY_Pos 16U /*!< SCB AIRCR: VECTKEY Position */ 00422 #define SCB_AIRCR_VECTKEY_Msk (0xFFFFUL << SCB_AIRCR_VECTKEY_Pos) /*!< SCB AIRCR: VECTKEY Mask */ 00423 00424 #define SCB_AIRCR_VECTKEYSTAT_Pos 16U /*!< SCB AIRCR: VECTKEYSTAT Position */ 00425 #define SCB_AIRCR_VECTKEYSTAT_Msk (0xFFFFUL << SCB_AIRCR_VECTKEYSTAT_Pos) /*!< SCB AIRCR: VECTKEYSTAT Mask */ 00426 00427 #define SCB_AIRCR_ENDIANESS_Pos 15U /*!< SCB AIRCR: ENDIANESS Position */ 00428 #define SCB_AIRCR_ENDIANESS_Msk (1UL << SCB_AIRCR_ENDIANESS_Pos) /*!< SCB AIRCR: ENDIANESS Mask */ 00429 00430 #define SCB_AIRCR_SYSRESETREQ_Pos 2U /*!< SCB AIRCR: SYSRESETREQ Position */ 00431 #define SCB_AIRCR_SYSRESETREQ_Msk (1UL << SCB_AIRCR_SYSRESETREQ_Pos) /*!< SCB AIRCR: SYSRESETREQ Mask */ 00432 00433 #define SCB_AIRCR_VECTCLRACTIVE_Pos 1U /*!< SCB AIRCR: VECTCLRACTIVE Position */ 00434 #define SCB_AIRCR_VECTCLRACTIVE_Msk (1UL << SCB_AIRCR_VECTCLRACTIVE_Pos) /*!< SCB AIRCR: VECTCLRACTIVE Mask */ 00435 00436 /* SCB System Control Register Definitions */ 00437 #define SCB_SCR_SEVONPEND_Pos 4U /*!< SCB SCR: SEVONPEND Position */ 00438 #define SCB_SCR_SEVONPEND_Msk (1UL << SCB_SCR_SEVONPEND_Pos) /*!< SCB SCR: SEVONPEND Mask */ 00439 00440 #define SCB_SCR_SLEEPDEEP_Pos 2U /*!< SCB SCR: SLEEPDEEP Position */ 00441 #define SCB_SCR_SLEEPDEEP_Msk (1UL << SCB_SCR_SLEEPDEEP_Pos) /*!< SCB SCR: SLEEPDEEP Mask */ 00442 00443 #define SCB_SCR_SLEEPONEXIT_Pos 1U /*!< SCB SCR: SLEEPONEXIT Position */ 00444 #define SCB_SCR_SLEEPONEXIT_Msk (1UL << SCB_SCR_SLEEPONEXIT_Pos) /*!< SCB SCR: SLEEPONEXIT Mask */ 00445 00446 /* SCB Configuration Control Register Definitions */ 00447 #define SCB_CCR_STKALIGN_Pos 9U /*!< SCB CCR: STKALIGN Position */ 00448 #define SCB_CCR_STKALIGN_Msk (1UL << SCB_CCR_STKALIGN_Pos) /*!< SCB CCR: STKALIGN Mask */ 00449 00450 #define SCB_CCR_UNALIGN_TRP_Pos 3U /*!< SCB CCR: UNALIGN_TRP Position */ 00451 #define SCB_CCR_UNALIGN_TRP_Msk (1UL << SCB_CCR_UNALIGN_TRP_Pos) /*!< SCB CCR: UNALIGN_TRP Mask */ 00452 00453 /* SCB System Handler Control and State Register Definitions */ 00454 #define SCB_SHCSR_SVCALLPENDED_Pos 15U /*!< SCB SHCSR: SVCALLPENDED Position */ 00455 #define SCB_SHCSR_SVCALLPENDED_Msk (1UL << SCB_SHCSR_SVCALLPENDED_Pos) /*!< SCB SHCSR: SVCALLPENDED Mask */ 00456 00457 /*@} end of group CMSIS_SCB */ 00458 00459 00460 /** 00461 \ingroup CMSIS_core_register 00462 \defgroup CMSIS_SysTick System Tick Timer (SysTick) 00463 \brief Type definitions for the System Timer Registers. 00464 @{ 00465 */ 00466 00467 /** 00468 \brief Structure type to access the System Timer (SysTick). 00469 */ 00470 typedef struct 00471 { 00472 __IOM uint32_t CTRL; /*!< Offset: 0x000 (R/W) SysTick Control and Status Register */ 00473 __IOM uint32_t LOAD; /*!< Offset: 0x004 (R/W) SysTick Reload Value Register */ 00474 __IOM uint32_t VAL; /*!< Offset: 0x008 (R/W) SysTick Current Value Register */ 00475 __IM uint32_t CALIB; /*!< Offset: 0x00C (R/ ) SysTick Calibration Register */ 00476 } SysTick_Type; 00477 00478 /* SysTick Control / Status Register Definitions */ 00479 #define SysTick_CTRL_COUNTFLAG_Pos 16U /*!< SysTick CTRL: COUNTFLAG Position */ 00480 #define SysTick_CTRL_COUNTFLAG_Msk (1UL << SysTick_CTRL_COUNTFLAG_Pos) /*!< SysTick CTRL: COUNTFLAG Mask */ 00481 00482 #define SysTick_CTRL_CLKSOURCE_Pos 2U /*!< SysTick CTRL: CLKSOURCE Position */ 00483 #define SysTick_CTRL_CLKSOURCE_Msk (1UL << SysTick_CTRL_CLKSOURCE_Pos) /*!< SysTick CTRL: CLKSOURCE Mask */ 00484 00485 #define SysTick_CTRL_TICKINT_Pos 1U /*!< SysTick CTRL: TICKINT Position */ 00486 #define SysTick_CTRL_TICKINT_Msk (1UL << SysTick_CTRL_TICKINT_Pos) /*!< SysTick CTRL: TICKINT Mask */ 00487 00488 #define SysTick_CTRL_ENABLE_Pos 0U /*!< SysTick CTRL: ENABLE Position */ 00489 #define SysTick_CTRL_ENABLE_Msk (1UL /*<< SysTick_CTRL_ENABLE_Pos*/) /*!< SysTick CTRL: ENABLE Mask */ 00490 00491 /* SysTick Reload Register Definitions */ 00492 #define SysTick_LOAD_RELOAD_Pos 0U /*!< SysTick LOAD: RELOAD Position */ 00493 #define SysTick_LOAD_RELOAD_Msk (0xFFFFFFUL /*<< SysTick_LOAD_RELOAD_Pos*/) /*!< SysTick LOAD: RELOAD Mask */ 00494 00495 /* SysTick Current Register Definitions */ 00496 #define SysTick_VAL_CURRENT_Pos 0U /*!< SysTick VAL: CURRENT Position */ 00497 #define SysTick_VAL_CURRENT_Msk (0xFFFFFFUL /*<< SysTick_VAL_CURRENT_Pos*/) /*!< SysTick VAL: CURRENT Mask */ 00498 00499 /* SysTick Calibration Register Definitions */ 00500 #define SysTick_CALIB_NOREF_Pos 31U /*!< SysTick CALIB: NOREF Position */ 00501 #define SysTick_CALIB_NOREF_Msk (1UL << SysTick_CALIB_NOREF_Pos) /*!< SysTick CALIB: NOREF Mask */ 00502 00503 #define SysTick_CALIB_SKEW_Pos 30U /*!< SysTick CALIB: SKEW Position */ 00504 #define SysTick_CALIB_SKEW_Msk (1UL << SysTick_CALIB_SKEW_Pos) /*!< SysTick CALIB: SKEW Mask */ 00505 00506 #define SysTick_CALIB_TENMS_Pos 0U /*!< SysTick CALIB: TENMS Position */ 00507 #define SysTick_CALIB_TENMS_Msk (0xFFFFFFUL /*<< SysTick_CALIB_TENMS_Pos*/) /*!< SysTick CALIB: TENMS Mask */ 00508 00509 /*@} end of group CMSIS_SysTick */ 00510 00511 #if defined (__MPU_PRESENT) && (__MPU_PRESENT == 1U) 00512 /** 00513 \ingroup CMSIS_core_register 00514 \defgroup CMSIS_MPU Memory Protection Unit (MPU) 00515 \brief Type definitions for the Memory Protection Unit (MPU) 00516 @{ 00517 */ 00518 00519 /** 00520 \brief Structure type to access the Memory Protection Unit (MPU). 00521 */ 00522 typedef struct 00523 { 00524 __IM uint32_t TYPE; /*!< Offset: 0x000 (R/ ) MPU Type Register */ 00525 __IOM uint32_t CTRL; /*!< Offset: 0x004 (R/W) MPU Control Register */ 00526 __IOM uint32_t RNR; /*!< Offset: 0x008 (R/W) MPU Region RNRber Register */ 00527 __IOM uint32_t RBAR; /*!< Offset: 0x00C (R/W) MPU Region Base Address Register */ 00528 __IOM uint32_t RASR; /*!< Offset: 0x010 (R/W) MPU Region Attribute and Size Register */ 00529 } MPU_Type; 00530 00531 /* MPU Type Register Definitions */ 00532 #define MPU_TYPE_IREGION_Pos 16U /*!< MPU TYPE: IREGION Position */ 00533 #define MPU_TYPE_IREGION_Msk (0xFFUL << MPU_TYPE_IREGION_Pos) /*!< MPU TYPE: IREGION Mask */ 00534 00535 #define MPU_TYPE_DREGION_Pos 8U /*!< MPU TYPE: DREGION Position */ 00536 #define MPU_TYPE_DREGION_Msk (0xFFUL << MPU_TYPE_DREGION_Pos) /*!< MPU TYPE: DREGION Mask */ 00537 00538 #define MPU_TYPE_SEPARATE_Pos 0U /*!< MPU TYPE: SEPARATE Position */ 00539 #define MPU_TYPE_SEPARATE_Msk (1UL /*<< MPU_TYPE_SEPARATE_Pos*/) /*!< MPU TYPE: SEPARATE Mask */ 00540 00541 /* MPU Control Register Definitions */ 00542 #define MPU_CTRL_PRIVDEFENA_Pos 2U /*!< MPU CTRL: PRIVDEFENA Position */ 00543 #define MPU_CTRL_PRIVDEFENA_Msk (1UL << MPU_CTRL_PRIVDEFENA_Pos) /*!< MPU CTRL: PRIVDEFENA Mask */ 00544 00545 #define MPU_CTRL_HFNMIENA_Pos 1U /*!< MPU CTRL: HFNMIENA Position */ 00546 #define MPU_CTRL_HFNMIENA_Msk (1UL << MPU_CTRL_HFNMIENA_Pos) /*!< MPU CTRL: HFNMIENA Mask */ 00547 00548 #define MPU_CTRL_ENABLE_Pos 0U /*!< MPU CTRL: ENABLE Position */ 00549 #define MPU_CTRL_ENABLE_Msk (1UL /*<< MPU_CTRL_ENABLE_Pos*/) /*!< MPU CTRL: ENABLE Mask */ 00550 00551 /* MPU Region Number Register Definitions */ 00552 #define MPU_RNR_REGION_Pos 0U /*!< MPU RNR: REGION Position */ 00553 #define MPU_RNR_REGION_Msk (0xFFUL /*<< MPU_RNR_REGION_Pos*/) /*!< MPU RNR: REGION Mask */ 00554 00555 /* MPU Region Base Address Register Definitions */ 00556 #define MPU_RBAR_ADDR_Pos 8U /*!< MPU RBAR: ADDR Position */ 00557 #define MPU_RBAR_ADDR_Msk (0xFFFFFFUL << MPU_RBAR_ADDR_Pos) /*!< MPU RBAR: ADDR Mask */ 00558 00559 #define MPU_RBAR_VALID_Pos 4U /*!< MPU RBAR: VALID Position */ 00560 #define MPU_RBAR_VALID_Msk (1UL << MPU_RBAR_VALID_Pos) /*!< MPU RBAR: VALID Mask */ 00561 00562 #define MPU_RBAR_REGION_Pos 0U /*!< MPU RBAR: REGION Position */ 00563 #define MPU_RBAR_REGION_Msk (0xFUL /*<< MPU_RBAR_REGION_Pos*/) /*!< MPU RBAR: REGION Mask */ 00564 00565 /* MPU Region Attribute and Size Register Definitions */ 00566 #define MPU_RASR_ATTRS_Pos 16U /*!< MPU RASR: MPU Region Attribute field Position */ 00567 #define MPU_RASR_ATTRS_Msk (0xFFFFUL << MPU_RASR_ATTRS_Pos) /*!< MPU RASR: MPU Region Attribute field Mask */ 00568 00569 #define MPU_RASR_XN_Pos 28U /*!< MPU RASR: ATTRS.XN Position */ 00570 #define MPU_RASR_XN_Msk (1UL << MPU_RASR_XN_Pos) /*!< MPU RASR: ATTRS.XN Mask */ 00571 00572 #define MPU_RASR_AP_Pos 24U /*!< MPU RASR: ATTRS.AP Position */ 00573 #define MPU_RASR_AP_Msk (0x7UL << MPU_RASR_AP_Pos) /*!< MPU RASR: ATTRS.AP Mask */ 00574 00575 #define MPU_RASR_TEX_Pos 19U /*!< MPU RASR: ATTRS.TEX Position */ 00576 #define MPU_RASR_TEX_Msk (0x7UL << MPU_RASR_TEX_Pos) /*!< MPU RASR: ATTRS.TEX Mask */ 00577 00578 #define MPU_RASR_S_Pos 18U /*!< MPU RASR: ATTRS.S Position */ 00579 #define MPU_RASR_S_Msk (1UL << MPU_RASR_S_Pos) /*!< MPU RASR: ATTRS.S Mask */ 00580 00581 #define MPU_RASR_C_Pos 17U /*!< MPU RASR: ATTRS.C Position */ 00582 #define MPU_RASR_C_Msk (1UL << MPU_RASR_C_Pos) /*!< MPU RASR: ATTRS.C Mask */ 00583 00584 #define MPU_RASR_B_Pos 16U /*!< MPU RASR: ATTRS.B Position */ 00585 #define MPU_RASR_B_Msk (1UL << MPU_RASR_B_Pos) /*!< MPU RASR: ATTRS.B Mask */ 00586 00587 #define MPU_RASR_SRD_Pos 8U /*!< MPU RASR: Sub-Region Disable Position */ 00588 #define MPU_RASR_SRD_Msk (0xFFUL << MPU_RASR_SRD_Pos) /*!< MPU RASR: Sub-Region Disable Mask */ 00589 00590 #define MPU_RASR_SIZE_Pos 1U /*!< MPU RASR: Region Size Field Position */ 00591 #define MPU_RASR_SIZE_Msk (0x1FUL << MPU_RASR_SIZE_Pos) /*!< MPU RASR: Region Size Field Mask */ 00592 00593 #define MPU_RASR_ENABLE_Pos 0U /*!< MPU RASR: Region enable bit Position */ 00594 #define MPU_RASR_ENABLE_Msk (1UL /*<< MPU_RASR_ENABLE_Pos*/) /*!< MPU RASR: Region enable bit Disable Mask */ 00595 00596 /*@} end of group CMSIS_MPU */ 00597 #endif 00598 00599 00600 /** 00601 \ingroup CMSIS_core_register 00602 \defgroup CMSIS_CoreDebug Core Debug Registers (CoreDebug) 00603 \brief Cortex-M0+ Core Debug Registers (DCB registers, SHCSR, and DFSR) are only accessible over DAP and not via processor. 00604 Therefore they are not covered by the Cortex-M0+ header file. 00605 @{ 00606 */ 00607 /*@} end of group CMSIS_CoreDebug */ 00608 00609 00610 /** 00611 \ingroup CMSIS_core_register 00612 \defgroup CMSIS_core_bitfield Core register bit field macros 00613 \brief Macros for use with bit field definitions (xxx_Pos, xxx_Msk). 00614 @{ 00615 */ 00616 00617 /** 00618 \brief Mask and shift a bit field value for use in a register bit range. 00619 \param[in] field Name of the register bit field. 00620 \param[in] value Value of the bit field. This parameter is interpreted as an uint32_t type. 00621 \return Masked and shifted value. 00622 */ 00623 #define _VAL2FLD(field, value) (((uint32_t)(value) << field ## _Pos) & field ## _Msk) 00624 00625 /** 00626 \brief Mask and shift a register value to extract a bit filed value. 00627 \param[in] field Name of the register bit field. 00628 \param[in] value Value of register. This parameter is interpreted as an uint32_t type. 00629 \return Masked and shifted bit field value. 00630 */ 00631 #define _FLD2VAL(field, value) (((uint32_t)(value) & field ## _Msk) >> field ## _Pos) 00632 00633 /*@} end of group CMSIS_core_bitfield */ 00634 00635 00636 /** 00637 \ingroup CMSIS_core_register 00638 \defgroup CMSIS_core_base Core Definitions 00639 \brief Definitions for base addresses, unions, and structures. 00640 @{ 00641 */ 00642 00643 /* Memory mapping of Core Hardware */ 00644 #define SCS_BASE (0xE000E000UL) /*!< System Control Space Base Address */ 00645 #define SysTick_BASE (SCS_BASE + 0x0010UL) /*!< SysTick Base Address */ 00646 #define NVIC_BASE (SCS_BASE + 0x0100UL) /*!< NVIC Base Address */ 00647 #define SCB_BASE (SCS_BASE + 0x0D00UL) /*!< System Control Block Base Address */ 00648 00649 #define SCB ((SCB_Type *) SCB_BASE ) /*!< SCB configuration struct */ 00650 #define SysTick ((SysTick_Type *) SysTick_BASE ) /*!< SysTick configuration struct */ 00651 #define NVIC ((NVIC_Type *) NVIC_BASE ) /*!< NVIC configuration struct */ 00652 00653 #if defined (__MPU_PRESENT) && (__MPU_PRESENT == 1U) 00654 #define MPU_BASE (SCS_BASE + 0x0D90UL) /*!< Memory Protection Unit */ 00655 #define MPU ((MPU_Type *) MPU_BASE ) /*!< Memory Protection Unit */ 00656 #endif 00657 00658 /*@} */ 00659 00660 00661 00662 /******************************************************************************* 00663 * Hardware Abstraction Layer 00664 Core Function Interface contains: 00665 - Core NVIC Functions 00666 - Core SysTick Functions 00667 - Core Register Access Functions 00668 ******************************************************************************/ 00669 /** 00670 \defgroup CMSIS_Core_FunctionInterface Functions and Instructions Reference 00671 */ 00672 00673 00674 00675 /* ########################## NVIC functions #################################### */ 00676 /** 00677 \ingroup CMSIS_Core_FunctionInterface 00678 \defgroup CMSIS_Core_NVICFunctions NVIC Functions 00679 \brief Functions that manage interrupts and exceptions via the NVIC. 00680 @{ 00681 */ 00682 00683 #ifdef CMSIS_NVIC_VIRTUAL 00684 #ifndef CMSIS_NVIC_VIRTUAL_HEADER_FILE 00685 #define CMSIS_NVIC_VIRTUAL_HEADER_FILE "cmsis_nvic_virtual.h" 00686 #endif 00687 #include CMSIS_NVIC_VIRTUAL_HEADER_FILE 00688 #else 00689 /*#define NVIC_SetPriorityGrouping __NVIC_SetPriorityGrouping not available for Cortex-M0+ */ 00690 /*#define NVIC_GetPriorityGrouping __NVIC_GetPriorityGrouping not available for Cortex-M0+ */ 00691 #define NVIC_EnableIRQ __NVIC_EnableIRQ 00692 #define NVIC_GetEnableIRQ __NVIC_GetEnableIRQ 00693 #define NVIC_DisableIRQ __NVIC_DisableIRQ 00694 #define NVIC_GetPendingIRQ __NVIC_GetPendingIRQ 00695 #define NVIC_SetPendingIRQ __NVIC_SetPendingIRQ 00696 #define NVIC_ClearPendingIRQ __NVIC_ClearPendingIRQ 00697 /*#define NVIC_GetActive __NVIC_GetActive not available for Cortex-M0+ */ 00698 #define NVIC_SetPriority __NVIC_SetPriority 00699 #define NVIC_GetPriority __NVIC_GetPriority 00700 #define NVIC_SystemReset __NVIC_SystemReset 00701 #endif /* CMSIS_NVIC_VIRTUAL */ 00702 00703 #ifdef CMSIS_VECTAB_VIRTUAL 00704 #ifndef CMSIS_VECTAB_VIRTUAL_HEADER_FILE 00705 #define CMSIS_VECTAB_VIRTUAL_HEADER_FILE "cmsis_vectab_virtual.h" 00706 #endif 00707 #include CMSIS_VECTAB_VIRTUAL_HEADER_FILE 00708 #else 00709 #define NVIC_SetVector __NVIC_SetVector 00710 #define NVIC_GetVector __NVIC_GetVector 00711 #endif /* (CMSIS_VECTAB_VIRTUAL) */ 00712 00713 #define NVIC_USER_IRQ_OFFSET 16 00714 00715 00716 /* Interrupt Priorities are WORD accessible only under ARMv6M */ 00717 /* The following MACROS handle generation of the register offset and byte masks */ 00718 #define _BIT_SHIFT(IRQn) ( ((((uint32_t)(int32_t)(IRQn)) ) & 0x03UL) * 8UL) 00719 #define _SHP_IDX(IRQn) ( (((((uint32_t)(int32_t)(IRQn)) & 0x0FUL)-8UL) >> 2UL) ) 00720 #define _IP_IDX(IRQn) ( (((uint32_t)(int32_t)(IRQn)) >> 2UL) ) 00721 00722 00723 /** 00724 \brief Enable Interrupt 00725 \details Enables a device specific interrupt in the NVIC interrupt controller. 00726 \param [in] IRQn Device specific interrupt number. 00727 \note IRQn must not be negative. 00728 */ 00729 __STATIC_INLINE void __NVIC_EnableIRQ(IRQn_Type IRQn) 00730 { 00731 if ((int32_t)(IRQn) >= 0) 00732 { 00733 NVIC->ISER[0U] = (uint32_t)(1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL)); 00734 } 00735 } 00736 00737 00738 /** 00739 \brief Get Interrupt Enable status 00740 \details Returns a device specific interrupt enable status from the NVIC interrupt controller. 00741 \param [in] IRQn Device specific interrupt number. 00742 \return 0 Interrupt is not enabled. 00743 \return 1 Interrupt is enabled. 00744 \note IRQn must not be negative. 00745 */ 00746 __STATIC_INLINE uint32_t __NVIC_GetEnableIRQ(IRQn_Type IRQn) 00747 { 00748 if ((int32_t)(IRQn) >= 0) 00749 { 00750 return((uint32_t)(((NVIC->ISER[0U] & (1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL))) != 0UL) ? 1UL : 0UL)); 00751 } 00752 else 00753 { 00754 return(0U); 00755 } 00756 } 00757 00758 00759 /** 00760 \brief Disable Interrupt 00761 \details Disables a device specific interrupt in the NVIC interrupt controller. 00762 \param [in] IRQn Device specific interrupt number. 00763 \note IRQn must not be negative. 00764 */ 00765 __STATIC_INLINE void __NVIC_DisableIRQ(IRQn_Type IRQn) 00766 { 00767 if ((int32_t)(IRQn) >= 0) 00768 { 00769 NVIC->ICER[0U] = (uint32_t)(1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL)); 00770 __DSB(); 00771 __ISB(); 00772 } 00773 } 00774 00775 00776 /** 00777 \brief Get Pending Interrupt 00778 \details Reads the NVIC pending register and returns the pending bit for the specified device specific interrupt. 00779 \param [in] IRQn Device specific interrupt number. 00780 \return 0 Interrupt status is not pending. 00781 \return 1 Interrupt status is pending. 00782 \note IRQn must not be negative. 00783 */ 00784 __STATIC_INLINE uint32_t __NVIC_GetPendingIRQ(IRQn_Type IRQn) 00785 { 00786 if ((int32_t)(IRQn) >= 0) 00787 { 00788 return((uint32_t)(((NVIC->ISPR[0U] & (1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL))) != 0UL) ? 1UL : 0UL)); 00789 } 00790 else 00791 { 00792 return(0U); 00793 } 00794 } 00795 00796 00797 /** 00798 \brief Set Pending Interrupt 00799 \details Sets the pending bit of a device specific interrupt in the NVIC pending register. 00800 \param [in] IRQn Device specific interrupt number. 00801 \note IRQn must not be negative. 00802 */ 00803 __STATIC_INLINE void __NVIC_SetPendingIRQ(IRQn_Type IRQn) 00804 { 00805 if ((int32_t)(IRQn) >= 0) 00806 { 00807 NVIC->ISPR[0U] = (uint32_t)(1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL)); 00808 } 00809 } 00810 00811 00812 /** 00813 \brief Clear Pending Interrupt 00814 \details Clears the pending bit of a device specific interrupt in the NVIC pending register. 00815 \param [in] IRQn Device specific interrupt number. 00816 \note IRQn must not be negative. 00817 */ 00818 __STATIC_INLINE void __NVIC_ClearPendingIRQ(IRQn_Type IRQn) 00819 { 00820 if ((int32_t)(IRQn) >= 0) 00821 { 00822 NVIC->ICPR[0U] = (uint32_t)(1UL << (((uint32_t)(int32_t)IRQn) & 0x1FUL)); 00823 } 00824 } 00825 00826 00827 /** 00828 \brief Set Interrupt Priority 00829 \details Sets the priority of a device specific interrupt or a processor exception. 00830 The interrupt number can be positive to specify a device specific interrupt, 00831 or negative to specify a processor exception. 00832 \param [in] IRQn Interrupt number. 00833 \param [in] priority Priority to set. 00834 \note The priority cannot be set for every processor exception. 00835 */ 00836 __STATIC_INLINE void __NVIC_SetPriority(IRQn_Type IRQn, uint32_t priority) 00837 { 00838 if ((int32_t)(IRQn) >= 0) 00839 { 00840 NVIC->IP[_IP_IDX(IRQn)] = ((uint32_t)(NVIC->IP[_IP_IDX(IRQn)] & ~(0xFFUL << _BIT_SHIFT(IRQn))) | 00841 (((priority << (8U - __NVIC_PRIO_BITS)) & (uint32_t)0xFFUL) << _BIT_SHIFT(IRQn))); 00842 } 00843 else 00844 { 00845 SCB->SHP[_SHP_IDX(IRQn)] = ((uint32_t)(SCB->SHP[_SHP_IDX(IRQn)] & ~(0xFFUL << _BIT_SHIFT(IRQn))) | 00846 (((priority << (8U - __NVIC_PRIO_BITS)) & (uint32_t)0xFFUL) << _BIT_SHIFT(IRQn))); 00847 } 00848 } 00849 00850 00851 /** 00852 \brief Get Interrupt Priority 00853 \details Reads the priority of a device specific interrupt or a processor exception. 00854 The interrupt number can be positive to specify a device specific interrupt, 00855 or negative to specify a processor exception. 00856 \param [in] IRQn Interrupt number. 00857 \return Interrupt Priority. 00858 Value is aligned automatically to the implemented priority bits of the microcontroller. 00859 */ 00860 __STATIC_INLINE uint32_t __NVIC_GetPriority(IRQn_Type IRQn) 00861 { 00862 00863 if ((int32_t)(IRQn) >= 0) 00864 { 00865 return((uint32_t)(((NVIC->IP[ _IP_IDX(IRQn)] >> _BIT_SHIFT(IRQn) ) & (uint32_t)0xFFUL) >> (8U - __NVIC_PRIO_BITS))); 00866 } 00867 else 00868 { 00869 return((uint32_t)(((SCB->SHP[_SHP_IDX(IRQn)] >> _BIT_SHIFT(IRQn) ) & (uint32_t)0xFFUL) >> (8U - __NVIC_PRIO_BITS))); 00870 } 00871 } 00872 00873 00874 /** 00875 \brief Set Interrupt Vector 00876 \details Sets an interrupt vector in SRAM based interrupt vector table. 00877 The interrupt number can be positive to specify a device specific interrupt, 00878 or negative to specify a processor exception. 00879 VTOR must been relocated to SRAM before. 00880 If VTOR is not present address 0 must be mapped to SRAM. 00881 \param [in] IRQn Interrupt number 00882 \param [in] vector Address of interrupt handler function 00883 */ 00884 __STATIC_INLINE void __NVIC_SetVector(IRQn_Type IRQn, uint32_t vector) 00885 { 00886 #if defined (__VTOR_PRESENT) && (__VTOR_PRESENT == 1U) 00887 uint32_t *vectors = (uint32_t *)SCB->VTOR; 00888 #else 00889 uint32_t *vectors = (uint32_t *)0x0U; 00890 #endif 00891 vectors[(int32_t)IRQn + NVIC_USER_IRQ_OFFSET] = vector; 00892 } 00893 00894 00895 /** 00896 \brief Get Interrupt Vector 00897 \details Reads an interrupt vector from interrupt vector table. 00898 The interrupt number can be positive to specify a device specific interrupt, 00899 or negative to specify a processor exception. 00900 \param [in] IRQn Interrupt number. 00901 \return Address of interrupt handler function 00902 */ 00903 __STATIC_INLINE uint32_t __NVIC_GetVector(IRQn_Type IRQn) 00904 { 00905 #if defined (__VTOR_PRESENT) && (__VTOR_PRESENT == 1U) 00906 uint32_t *vectors = (uint32_t *)SCB->VTOR; 00907 #else 00908 uint32_t *vectors = (uint32_t *)0x0U; 00909 #endif 00910 return vectors[(int32_t)IRQn + NVIC_USER_IRQ_OFFSET]; 00911 00912 } 00913 00914 00915 /** 00916 \brief System Reset 00917 \details Initiates a system reset request to reset the MCU. 00918 */ 00919 __STATIC_INLINE void __NVIC_SystemReset(void) 00920 { 00921 __DSB(); /* Ensure all outstanding memory accesses included 00922 buffered write are completed before reset */ 00923 SCB->AIRCR = ((0x5FAUL << SCB_AIRCR_VECTKEY_Pos) | 00924 SCB_AIRCR_SYSRESETREQ_Msk); 00925 __DSB(); /* Ensure completion of memory access */ 00926 00927 for(;;) /* wait until reset */ 00928 { 00929 __NOP(); 00930 } 00931 } 00932 00933 /*@} end of CMSIS_Core_NVICFunctions */ 00934 00935 00936 /* ########################## FPU functions #################################### */ 00937 /** 00938 \ingroup CMSIS_Core_FunctionInterface 00939 \defgroup CMSIS_Core_FpuFunctions FPU Functions 00940 \brief Function that provides FPU type. 00941 @{ 00942 */ 00943 00944 /** 00945 \brief get FPU type 00946 \details returns the FPU type 00947 \returns 00948 - \b 0: No FPU 00949 - \b 1: Single precision FPU 00950 - \b 2: Double + Single precision FPU 00951 */ 00952 __STATIC_INLINE uint32_t SCB_GetFPUType(void) 00953 { 00954 return 0U; /* No FPU */ 00955 } 00956 00957 00958 /*@} end of CMSIS_Core_FpuFunctions */ 00959 00960 00961 00962 /* ################################## SysTick function ############################################ */ 00963 /** 00964 \ingroup CMSIS_Core_FunctionInterface 00965 \defgroup CMSIS_Core_SysTickFunctions SysTick Functions 00966 \brief Functions that configure the System. 00967 @{ 00968 */ 00969 00970 #if defined (__Vendor_SysTickConfig) && (__Vendor_SysTickConfig == 0U) 00971 00972 /** 00973 \brief System Tick Configuration 00974 \details Initializes the System Timer and its interrupt, and starts the System Tick Timer. 00975 Counter is in free running mode to generate periodic interrupts. 00976 \param [in] ticks Number of ticks between two interrupts. 00977 \return 0 Function succeeded. 00978 \return 1 Function failed. 00979 \note When the variable <b>__Vendor_SysTickConfig</b> is set to 1, then the 00980 function <b>SysTick_Config</b> is not included. In this case, the file <b><i>device</i>.h</b> 00981 must contain a vendor-specific implementation of this function. 00982 */ 00983 __STATIC_INLINE uint32_t SysTick_Config(uint32_t ticks) 00984 { 00985 if ((ticks - 1UL) > SysTick_LOAD_RELOAD_Msk) 00986 { 00987 return (1UL); /* Reload value impossible */ 00988 } 00989 00990 SysTick->LOAD = (uint32_t)(ticks - 1UL); /* set reload register */ 00991 NVIC_SetPriority (SysTick_IRQn, (1UL << __NVIC_PRIO_BITS) - 1UL); /* set Priority for Systick Interrupt */ 00992 SysTick->VAL = 0UL; /* Load the SysTick Counter Value */ 00993 SysTick->CTRL = SysTick_CTRL_CLKSOURCE_Msk | 00994 SysTick_CTRL_TICKINT_Msk | 00995 SysTick_CTRL_ENABLE_Msk; /* Enable SysTick IRQ and SysTick Timer */ 00996 return (0UL); /* Function successful */ 00997 } 00998 00999 #endif 01000 01001 /*@} end of CMSIS_Core_SysTickFunctions */ 01002 01003 01004 01005 01006 #ifdef __cplusplus 01007 } 01008 #endif 01009 01010 #endif /* __CORE_CM0PLUS_H_DEPENDANT */ 01011 01012 #endif /* __CMSIS_GENERIC */
Generated on Tue Jul 12 2022 16:02:32 by
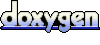