forked
Embed:
(wiki syntax)
Show/hide line numbers
PwmOut.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_PWMOUT_H 00017 #define MBED_PWMOUT_H 00018 00019 #include "platform/platform.h" 00020 00021 #if defined (DEVICE_PWMOUT) || defined(DOXYGEN_ONLY) 00022 #include "hal/pwmout_api.h" 00023 #include "platform/mbed_critical.h" 00024 00025 namespace mbed { 00026 /** \addtogroup drivers */ 00027 00028 /** A pulse-width modulation digital output 00029 * 00030 * @note Synchronization level: Interrupt safe 00031 * 00032 * Example 00033 * @code 00034 * // Fade a led on. 00035 * #include "mbed.h" 00036 * 00037 * PwmOut led(LED1); 00038 * 00039 * int main() { 00040 * while(1) { 00041 * led = led + 0.01; 00042 * wait(0.2); 00043 * if(led == 1.0) { 00044 * led = 0; 00045 * } 00046 * } 00047 * } 00048 * @endcode 00049 * @ingroup drivers 00050 */ 00051 class PwmOut { 00052 00053 public: 00054 00055 /** Create a PwmOut connected to the specified pin 00056 * 00057 * @param pin PwmOut pin to connect to 00058 */ 00059 PwmOut(PinName pin) { 00060 core_util_critical_section_enter(); 00061 pwmout_init(&_pwm, pin); 00062 core_util_critical_section_exit(); 00063 } 00064 00065 /** Set the ouput duty-cycle, specified as a percentage (float) 00066 * 00067 * @param value A floating-point value representing the output duty-cycle, 00068 * specified as a percentage. The value should lie between 00069 * 0.0f (representing on 0%) and 1.0f (representing on 100%). 00070 * Values outside this range will be saturated to 0.0f or 1.0f. 00071 */ 00072 void write(float value) { 00073 core_util_critical_section_enter(); 00074 pwmout_write(&_pwm, value); 00075 core_util_critical_section_exit(); 00076 } 00077 00078 /** Return the current output duty-cycle setting, measured as a percentage (float) 00079 * 00080 * @returns 00081 * A floating-point value representing the current duty-cycle being output on the pin, 00082 * measured as a percentage. The returned value will lie between 00083 * 0.0f (representing on 0%) and 1.0f (representing on 100%). 00084 * 00085 * @note 00086 * This value may not match exactly the value set by a previous write(). 00087 */ 00088 float read() { 00089 core_util_critical_section_enter(); 00090 float val = pwmout_read(&_pwm); 00091 core_util_critical_section_exit(); 00092 return val; 00093 } 00094 00095 /** Set the PWM period, specified in seconds (float), keeping the duty cycle the same. 00096 * 00097 * @param seconds Change the period of a PWM signal in seconds (float) without modifying the duty cycle 00098 * @note 00099 * The resolution is currently in microseconds; periods smaller than this 00100 * will be set to zero. 00101 */ 00102 void period(float seconds) { 00103 core_util_critical_section_enter(); 00104 pwmout_period(&_pwm, seconds); 00105 core_util_critical_section_exit(); 00106 } 00107 00108 /** Set the PWM period, specified in milli-seconds (int), keeping the duty cycle the same. 00109 * @param ms Change the period of a PWM signal in milli-seconds without modifying the duty cycle 00110 */ 00111 void period_ms(int ms) { 00112 core_util_critical_section_enter(); 00113 pwmout_period_ms(&_pwm, ms); 00114 core_util_critical_section_exit(); 00115 } 00116 00117 /** Set the PWM period, specified in micro-seconds (int), keeping the duty cycle the same. 00118 * @param us Change the period of a PWM signal in micro-seconds without modifying the duty cycle 00119 */ 00120 void period_us(int us) { 00121 core_util_critical_section_enter(); 00122 pwmout_period_us(&_pwm, us); 00123 core_util_critical_section_exit(); 00124 } 00125 00126 /** Set the PWM pulsewidth, specified in seconds (float), keeping the period the same. 00127 * @param seconds Change the pulse width of a PWM signal specified in seconds (float) 00128 */ 00129 void pulsewidth(float seconds) { 00130 core_util_critical_section_enter(); 00131 pwmout_pulsewidth(&_pwm, seconds); 00132 core_util_critical_section_exit(); 00133 } 00134 00135 /** Set the PWM pulsewidth, specified in milli-seconds (int), keeping the period the same. 00136 * @param ms Change the pulse width of a PWM signal specified in milli-seconds 00137 */ 00138 void pulsewidth_ms(int ms) { 00139 core_util_critical_section_enter(); 00140 pwmout_pulsewidth_ms(&_pwm, ms); 00141 core_util_critical_section_exit(); 00142 } 00143 00144 /** Set the PWM pulsewidth, specified in micro-seconds (int), keeping the period the same. 00145 * @param us Change the pulse width of a PWM signal specified in micro-seconds 00146 */ 00147 void pulsewidth_us(int us) { 00148 core_util_critical_section_enter(); 00149 pwmout_pulsewidth_us(&_pwm, us); 00150 core_util_critical_section_exit(); 00151 } 00152 00153 /** A operator shorthand for write() 00154 * \sa PwmOut::write() 00155 */ 00156 PwmOut& operator= (float value) { 00157 // Underlying call is thread safe 00158 write(value); 00159 return *this; 00160 } 00161 00162 /** A operator shorthand for write() 00163 * \sa PwmOut::write() 00164 */ 00165 PwmOut& operator= (PwmOut& rhs) { 00166 // Underlying call is thread safe 00167 write(rhs.read()); 00168 return *this; 00169 } 00170 00171 /** An operator shorthand for read() 00172 * \sa PwmOut::read() 00173 */ 00174 operator float() { 00175 // Underlying call is thread safe 00176 return read(); 00177 } 00178 00179 protected: 00180 pwmout_t _pwm; 00181 }; 00182 00183 } // namespace mbed 00184 00185 #endif 00186 00187 #endif
Generated on Tue Jul 12 2022 16:02:32 by
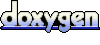