fork
Fork of cpputest by
Embed:
(wiki syntax)
Show/hide line numbers
CommandLineTestRunner.cpp
00001 /* 00002 * Copyright (c) 2007, Michael Feathers, James Grenning and Bas Vodde 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without 00006 * modification, are permitted provided that the following conditions are met: 00007 * * Redistributions of source code must retain the above copyright 00008 * notice, this list of conditions and the following disclaimer. 00009 * * Redistributions in binary form must reproduce the above copyright 00010 * notice, this list of conditions and the following disclaimer in the 00011 * documentation and/or other materials provided with the distribution. 00012 * * Neither the name of the <organization> nor the 00013 * names of its contributors may be used to endorse or promote products 00014 * derived from this software without specific prior written permission. 00015 * 00016 * THIS SOFTWARE IS PROVIDED BY THE EARLIER MENTIONED AUTHORS ``AS IS'' AND ANY 00017 * EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00018 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00019 * DISCLAIMED. IN NO EVENT SHALL <copyright holder> BE LIABLE FOR ANY 00020 * DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00021 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00022 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00023 * ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00024 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00025 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00026 */ 00027 00028 #include "CppUTest/TestHarness.h" 00029 #include "CppUTest/CommandLineTestRunner.h" 00030 #include "CppUTest/TestOutput.h" 00031 #include "CppUTest/JUnitTestOutput.h" 00032 #include "CppUTest/TestRegistry.h" 00033 00034 CommandLineTestRunner::CommandLineTestRunner(int ac, const char** av, TestOutput* output, TestRegistry* registry) : 00035 output_(output), jUnitOutput_(NULL), arguments_(NULL), registry_(registry) 00036 { 00037 arguments_ = new CommandLineArguments(ac, av); 00038 } 00039 00040 CommandLineTestRunner::~CommandLineTestRunner() 00041 { 00042 delete arguments_; 00043 delete jUnitOutput_; 00044 } 00045 00046 int CommandLineTestRunner::RunAllTests(int ac, char** av) 00047 { 00048 return RunAllTests(ac, const_cast<const char**> (av)); 00049 } 00050 00051 int CommandLineTestRunner::RunAllTests(int ac, const char** av) 00052 { 00053 int result = 0; 00054 ConsoleTestOutput output; 00055 00056 MemoryLeakWarningPlugin memLeakWarn(DEF_PLUGIN_MEM_LEAK); 00057 memLeakWarn.destroyGlobalDetectorAndTurnOffMemoryLeakDetectionInDestructor(true); 00058 TestRegistry::getCurrentRegistry()->installPlugin(&memLeakWarn); 00059 00060 { 00061 CommandLineTestRunner runner(ac, av, &output, TestRegistry::getCurrentRegistry()); 00062 result = runner.runAllTestsMain(); 00063 } 00064 00065 if (result == 0) { 00066 output << memLeakWarn.FinalReport(0); 00067 } 00068 TestRegistry::getCurrentRegistry()->removePluginByName(DEF_PLUGIN_MEM_LEAK); 00069 return result; 00070 } 00071 00072 int CommandLineTestRunner::runAllTestsMain() 00073 { 00074 int testResult = 0; 00075 00076 SetPointerPlugin pPlugin(DEF_PLUGIN_SET_POINTER); 00077 registry_->installPlugin(&pPlugin); 00078 00079 if (parseArguments(registry_->getFirstPlugin())) 00080 testResult = runAllTests(); 00081 00082 registry_->removePluginByName(DEF_PLUGIN_SET_POINTER); 00083 return testResult; 00084 } 00085 00086 void CommandLineTestRunner::initializeTestRun() 00087 { 00088 registry_->groupFilter(arguments_->getGroupFilter()); 00089 registry_->nameFilter(arguments_->getNameFilter()); 00090 if (arguments_->isVerbose()) output_->verbose(); 00091 if (arguments_->runTestsInSeperateProcess()) registry_->setRunTestsInSeperateProcess(); 00092 } 00093 00094 int CommandLineTestRunner::runAllTests() 00095 { 00096 initializeTestRun(); 00097 int loopCount = 0; 00098 int failureCount = 0; 00099 int repeat_ = arguments_->getRepeatCount(); 00100 00101 while (loopCount++ < repeat_) { 00102 output_->printTestRun(loopCount, repeat_); 00103 TestResult tr(*output_); 00104 registry_->runAllTests(tr); 00105 failureCount += tr.getFailureCount(); 00106 } 00107 00108 return failureCount; 00109 } 00110 00111 bool CommandLineTestRunner::parseArguments(TestPlugin* plugin) 00112 { 00113 if (arguments_->parse(plugin)) { 00114 if (arguments_->isJUnitOutput()) { 00115 output_ = jUnitOutput_ = new JUnitTestOutput; 00116 if (jUnitOutput_ != NULL) { 00117 jUnitOutput_->setPackageName(arguments_->getPackageName()); 00118 } 00119 } 00120 return true; 00121 } 00122 else { 00123 output_->print(arguments_->usage()); 00124 return false; 00125 } 00126 } 00127 00128 bool CommandLineTestRunner::isVerbose() 00129 { 00130 return arguments_->isVerbose(); 00131 } 00132 00133 int CommandLineTestRunner::getRepeatCount() 00134 { 00135 return arguments_->getRepeatCount(); 00136 } 00137 00138 TestFilter CommandLineTestRunner::getGroupFilter() 00139 { 00140 return arguments_->getGroupFilter(); 00141 } 00142 00143 TestFilter CommandLineTestRunner::getNameFilter() 00144 { 00145 return arguments_->getNameFilter(); 00146 }
Generated on Tue Jul 12 2022 21:37:56 by
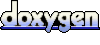