A library for LPM013M126A – JDI Color Memory LCD. This library is an inherited class from the “GraphicDisplay”, and Its functions are similar to “C12832_lcd” or ”SPI_TFT” library.
Dependents: Test_ColorMemLCD rIoTwear_LCD
ColorMemLCD.h
00001 /* 00002 * mbed library for the JDI color memory LCD LPM013M126A 00003 * derived from C12832_lcd 00004 * Copyright (c) 2016 Tadayuki Okamoto 00005 * Released under the MIT License: http://mbed.org/license/mit 00006 * 00007 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00008 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00009 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00010 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00011 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00012 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00013 * THE SOFTWARE. 00014 */ 00015 00016 #ifndef MBED_COLOR_MEM_LCD_H 00017 #define MBED_COLOR_MEM_LCD_H 00018 00019 #include "mbed.h" 00020 #include "GraphicsDisplay.h" 00021 00022 00023 /** @def 00024 * Device define 00025 */ 00026 #define LCD_DEVICE_WIDTH (176) 00027 #define LCD_DEVICE_HEIGHT (176) 00028 00029 /** @def 00030 * window system define 00031 */ 00032 #define LCD_DISP_WIDTH (176) 00033 #define LCD_DISP_HEIGHT (176) 00034 #define LCD_DISP_HEIGHT_MAX_BUF (44) 00035 00036 00037 /** @def 00038 * some RGB color definitions 00039 */ 00040 /* R, G, B */ 00041 #define LCD_COLOR_BLACK (0x00) /* 0 0 0 0 */ 00042 #define LCD_COLOR_BLUE (0x02) /* 0 0 1 0 */ 00043 #define LCD_COLOR_GREEN (0x04) /* 0 1 0 0 */ 00044 #define LCD_COLOR_CYAN (0x06) /* 0 1 1 0 */ 00045 #define LCD_COLOR_RED (0x08) /* 1 0 0 0 */ 00046 #define LCD_COLOR_MAGENTA (0x0a) /* 1 0 1 0 */ 00047 #define LCD_COLOR_YELLOW (0x0c) /* 1 1 0 0 */ 00048 #define LCD_COLOR_WHITE (0x0e) /* 1 1 1 0 */ 00049 00050 /** @def 00051 * ID for setTransMode 00052 */ 00053 #define LCD_TRANSMODE_OPAQUE (0x00) //!< BackGroud is Opaque 00054 #define LCD_TRANSMODE_TRANSPARENT (0x01) //!< BackGroud is Transparent 00055 #define LCD_TRANSMODE_TRANSLUCENT (0x02) //!< BackGroud is Translucent 00056 00057 /** @def 00058 *ID for setBlinkMode 00059 */ 00060 #define LCD_BLINKMODE_NONE (0x00) //!< Blinking None 00061 #define LCD_BLINKMODE_WHITE (0x01) //!< Blinking White 00062 #define LCD_BLINKMODE_BLACK (0x02) //!< Blinking Black 00063 #define LCD_BLINKMODE_INVERSE (0x03) //!< Inversion Mode 00064 00065 /** A class for Color Memory LCD Library 00066 */ 00067 class ColorMemLCD : public GraphicsDisplay { 00068 public: 00069 00070 /** Create a SPI_LCD object connected to SPI 00071 * 00072 * @param mosi pin connected to SDO of display 00073 * @param miso pin connected to SDI of display 00074 * @param sclk pin connected to RS of display 00075 * @param cs pin connected to CS of display 00076 * @param disp pin connected to DISP of display 00077 * @param power pin connected to POWER of display 00078 */ 00079 ColorMemLCD( PinName mosi, PinName miso, PinName sclk, PinName cs, PinName disp, PinName power, const char* name ="TFT" ); 00080 00081 /** Get the width of the screen in pixel 00082 * 00083 * @return width of screen in pixel 00084 * 00085 */ 00086 virtual int width(); 00087 00088 /** Get the height of the screen in pixel 00089 * 00090 * @return height of screen in pixel 00091 * 00092 */ 00093 virtual int height(); 00094 00095 /** Set window region 00096 * 00097 * @param x horizontal position 00098 * @param y vertical position 00099 * @param w window width in pixel 00100 * @param h window height in pixels 00101 */ 00102 virtual void window( int x, int y, int w, int h ); 00103 00104 /** Set a pixel on the window memory 00105 * 00106 * @param x horizontal position 00107 * @param y vertical position 00108 * @param color 4 bit pixel color 00109 */ 00110 virtual void pixel(int x, int y,int colour); 00111 00112 /** Set a pixel on the window memory (alpha blend) 00113 * 00114 * @param x horizontal position 00115 * @param y vertical position 00116 * @param color 4 bit pixel color 00117 */ 00118 virtual void pixel_alpha(int x, int y,int colour); 00119 00120 /** Fill the window memory with background color 00121 * 00122 */ 00123 virtual void cls (void); 00124 00125 /** draw a circle 00126 * 00127 * @param x0,y0 center 00128 * @param r radius 00129 * @param color 4 bit color 00130 * 00131 */ 00132 void circle(int x, int y, int r, int colour); 00133 00134 /** draw a filled circle 00135 * 00136 * @param x0,y0 center 00137 * @param r radius 00138 * @param color 4 bit color 00139 */ 00140 void fillcircle(int x, int y, int r, int colour); 00141 00142 /** draw a horizontal line 00143 * 00144 * @param x0 horizontal start 00145 * @param x1 horizontal stop 00146 * @param y vertical position 00147 * @param color 4 bit color 00148 * 00149 */ 00150 void hline(int x0, int x1, int y, int colour); 00151 00152 /** draw a vertical line 00153 * 00154 * @param x horizontal position 00155 * @param y0 vertical start 00156 * @param y1 vertical stop 00157 * @param color 4 bit color 00158 */ 00159 void vline(int y0, int y1, int x, int colour); 00160 00161 /** draw a 1 pixel line 00162 * 00163 * @param x0,y0 start point 00164 * @param x1,y1 stop point 00165 * @param color 4 bit color 00166 * 00167 */ 00168 void line(int x0, int y0, int x1, int y1, int colour); 00169 00170 /** draw a rect 00171 * 00172 * @param x0,y0 top left corner 00173 * @param x1,y1 down right corner 00174 * @param color 4 bit color 00175 * 00176 */ 00177 void rect(int x0, int y0, int x1, int y1, int colour); 00178 00179 /** draw a filled rect 00180 * 00181 * @param x0,y0 top left corner 00182 * @param x1,y1 down right corner 00183 * @param color 4 bit color 00184 * 00185 */ 00186 void fillrect(int x0, int y0, int x1, int y1, int colour); 00187 00188 /** setup cursor position 00189 * 00190 * @param x x-position (top left) 00191 * @param y y-position 00192 */ 00193 virtual void locate(int x, int y); 00194 00195 /** calculate the max number of char in a line 00196 * 00197 * @return max columns depends on actual font size 00198 * 00199 */ 00200 virtual int columns(void); 00201 00202 /** calculate the max number of columns 00203 * 00204 * @return max columndepends on actual font size 00205 * 00206 */ 00207 virtual int rows(void); 00208 00209 /** put a char on the screen 00210 * 00211 * @param value char to print 00212 * @return printed char 00213 * 00214 */ 00215 virtual int _putc(int value); 00216 00217 /** draw a character of selected font 00218 * 00219 * @param x x-position of char (top left) 00220 * @param y y-position 00221 * @param c char to print 00222 * 00223 */ 00224 virtual void character(int x, int y, int c); 00225 00226 /** select the font 00227 * 00228 * @param f pointer to font array 00229 * 00230 */ 00231 void set_font(unsigned char* f); 00232 00233 /** set transpalent effect 00234 * 00235 * @param mode trans mode 00236 * 00237 */ 00238 void setTransMode( char mode ); 00239 00240 /** set a bitmap on the window buffer 00241 * 00242 * @param x,y upper left corner 00243 * @param w width of bitmap 00244 * @param h high of bitmap 00245 * @param *bitmap pointer to the bitmap data 00246 * 00247 * @remarks bitmap format 4 bit R1 G1 B1 00248 * 00249 */ 00250 void Bitmap4bit( int x, int y, int w, int h, unsigned char *bitmap); 00251 00252 /** set a bitmap on the window buffer 00253 * 00254 * @param x,y upper left corner 00255 * @param w width of draw rect 00256 * @param h high of draw rect 00257 * @param *bitmap pointer to the bitmap data 00258 * @param bmp_x,bmp_y upper left corner at BMP files 00259 * @param bmp_w width of bitmap 00260 * @param bmp_h high of bitmap 00261 * 00262 * @remarks bitmap format 4 bit R1 G1 B1 00263 * 00264 */ 00265 void Bitmap4bit( int x, int y, int w, int h, unsigned char *bitmap, int bmp_x, int bmp_y, int bmp_w, int bmp_h ); 00266 00267 /** set a bitmap on the window buffer 00268 * 00269 * @param x,y upper left corner 00270 * @param w width of bitmap 00271 * @param h high of bitmap 00272 * @param *bitmap pointer to the bitmap data 00273 * 00274 * @remarks bitmap format 1 bit 00275 * 00276 */ 00277 void Bitmap1bit( int x, int y, int w, int h, unsigned char *bitmap); 00278 00279 /** set a bitmap on the window buffer 00280 * 00281 * @param x,y upper left corner 00282 * @param w width of draw rect 00283 * @param h high of draw rect 00284 * @param *bitmap pointer to the bitmap data 00285 * @param bmp_x,bmp_y upper left corner at BMP files 00286 * @param bmp_w width of bitmap 00287 * @param bmp_h high of bitmap 00288 * 00289 * @remarks bitmap format 1 bit 00290 * 00291 */ 00292 void Bitmap1bit( int x, int y, int w, int h, unsigned char *bitmap, int bmp_x, int bmp_y, int bmp_w, int bmp_h ); 00293 00294 /** Transfer to the LCD from diaply buffer 00295 * 00296 */ 00297 void update(); 00298 00299 /** Transfer a 24 bit BMP to the LCD from filesytem 00300 * 00301 * @param x,y position of upper left corner 00302 * @param *filenameBMP name of the BMP file 00303 * 00304 * @retval 1 succes 00305 * @retval 0 error 00306 * 00307 * @remarks bitmap format 24 bit R8 G8 B8 00308 * 00309 */ 00310 int BMP_24( int x, int y, const char *filenameBMP ); 00311 00312 /** Command for setting the state of LCD 00313 * 00314 */ 00315 void command_SetState(); 00316 00317 /** Command to clear whole the LCD 00318 * 00319 */ 00320 void command_AllClear(); 00321 00322 /** Command to blink 00323 * @param mode Blinking Mode or Inversion Mode 00324 * 00325 */ 00326 void setBlinkMode( char mode ); 00327 00328 /** Toggle the LCD polarity 00329 * 00330 */ 00331 void polling(); 00332 00333 void symbol(unsigned int x, unsigned int y, unsigned char *symbol); 00334 00335 protected: 00336 00337 /* Instance of inteface */ 00338 SPI _spi; 00339 DigitalOut _cs; 00340 DigitalOut _disp; 00341 DigitalOut _power; 00342 00343 /* polarity variable */ 00344 char polarity; 00345 char blink_cmd; 00346 00347 /* trans mode variable */ 00348 char trans_mode; 00349 00350 /* data for character variable */ 00351 unsigned char* font; 00352 int char_x; 00353 int char_y; 00354 00355 /* window variable */ 00356 int window_x; 00357 int window_y; 00358 int window_w; 00359 int window_h; 00360 00361 /* temporary buffer */ 00362 char cmd_buf[LCD_DISP_WIDTH/2]; /* for sending command */ 00363 char disp_buf[(LCD_DISP_WIDTH/2)*LCD_DISP_HEIGHT_MAX_BUF]; /* display buffer */ 00364 char file_buf[118]; /* for reading files */ 00365 00366 /** send command 00367 * 00368 * @param line_cmd cmannd data 00369 * @param line line of display 00370 * 00371 */ 00372 void sendLineCommand( char* line_cmd, int line ); 00373 }; 00374 00375 #endif /* MBED_COLOR_MEM_LCD_H */
Generated on Sun Jul 24 2022 21:55:57 by
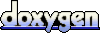