
Pull request for i.a. sensor buffer template
Dependencies: BLE_API MPU6050 mbed nRF51822
main.cpp
00001 #include "mbed.h" 00002 #include "mbed_mem_trace.h" 00003 #include "nrf51.h" 00004 #include "nrf51_bitfields.h" 00005 #include "MPU6050.h" 00006 00007 #include "BLE.h" 00008 #include "DFUService.h" 00009 #include "UARTService.h" 00010 #include "BatteryService.h" 00011 #include "DeviceInformationService.h" 00012 #include "TemperatureService.h" 00013 #include "AccelerationService.h" 00014 00015 #include "ReadIntervals.h" 00016 #include "Util.h" 00017 #include "IO.h" 00018 #include "MeasurementBufferTemplate.h" 00019 00020 //https://os.mbed.com/handbook/SDFileSystem 00021 // http://os.mbed.com/users/mbed_official/code/SDFileSystem/ 00022 // needs: https://os.mbed.com/teams/mbed-official/code/FATFileSystem/pull-request/4 00023 // #include "SDFileSystem.h" 00024 //https://os.mbed.com/cookbook/SD-Card-File-System 00025 00026 00027 /////////////////////////////////////// 00028 // DEFINES // 00029 /////////////////////////////////////// 00030 00031 // Starting sampling rate. 00032 #define DEFAULT_MPU_HZ (100) 00033 00034 // --- Device Information --- // 00035 #define MANUFACTURER "PM @ ALTEN" 00036 #define MODELNUMBER "IoT BLE" 00037 #define SERIALNUMBER "123456" 00038 #define HARDWAREREVISION "Seeed demo IoT" 00039 #define FIRMWAREREVISION "1.0" 00040 #define SOFTWAREREVISION "1.0" 00041 00042 ///////////////////////////////////////// 00043 // CONSTANTS // 00044 ///////////////////////////////////////// 00045 00046 // --- UUIDs --- // 00047 const char acceleration128bitUUIDlist[] = { 0x53, 0xF8, 0x0E, 0x12, 0xAD, 0xBB, 0xF1, 0x38, 0xAC, 0x07, 0xD2, 0x3D, 0xF0, 0xE2, 0x34, 0x5D }; 00048 const UUID uuidTempBulkService("11111111-1000-2222-3333-444455556666"); 00049 const UUID uuidTempBulkCharRead("11111111-1001-2222-3333-444455556666"); 00050 00051 // --- Temperature Buffer Data --- // 00052 const unsigned int TemperatureBufferSize = 100; 00053 const unsigned int TemperatureBulkUpdateSize = 6; 00054 // https://devzone.nordicsemi.com/f/nordic-q-a/519/reading-a-subset-of-data-on-a-ble-server 00055 // characteristic data max 512 bytes 00056 // https://github.com/pieterm/bledemo#step-8-add-callback-function-on-data-written-event 00057 // GattWriteCallbackParams 00058 00059 00060 ///////////////////////////////////////////////////// 00061 // FUNCTION DECLARATIONS // 00062 ///////////////////////////////////////////////////// 00063 00064 uint16_t readTemperature(); 00065 00066 //////////////////////////////////////// 00067 // TYPEDEFS // 00068 //////////////////////////////////////// 00069 00070 typedef MeasurementBufferTemplate<uint16_t, TemperatureBufferSize, TemperatureBulkUpdateSize, ReadIntervals::TemperatureSensorPeriod, readTemperature> TemperatureBuffer; 00071 00072 ///////////////////////////////////////// 00073 // VARIABLES // 00074 ///////////////////////////////////////// 00075 00076 // --- Buffers --- // 00077 TemperatureBuffer temperatureBuffer; 00078 TemperatureBuffer::BulkUpdate tempUpdate; 00079 00080 // --- Timing --- // 00081 Ticker ticker; 00082 00083 // --- BLE --- // 00084 GattCharacteristic * tempBulkUpdateCharacteristic; 00085 00086 // --- Flags --- // 00087 volatile bool batteryVoltageChanged = false; 00088 volatile bool tempUpdateFlag = false; 00089 volatile bool startMeasure = false; 00090 00091 ///////////////////////////////////////// 00092 // FUNCTIONS // 00093 ///////////////////////////////////////// 00094 00095 00096 uint16_t readTemperature(){ 00097 //read temperature (raw) 00098 int16_t tempRaw = mpu.getTempRaw(); 00099 // convert into 0.1 degrees Celsius 00100 tempRaw *= 10; 00101 tempRaw += 5210; 00102 tempRaw /= 340; 00103 tempRaw += 350; 00104 00105 return tempRaw; 00106 } 00107 00108 00109 void connectionCallback(const Gap::ConnectionCallbackParams_t *params) 00110 { 00111 LOG("Connected!\n"); 00112 // bleIsConnected = true; 00113 } 00114 00115 void disconnectionCallback(const Gap::DisconnectionCallbackParams_t *cbParams) 00116 { 00117 LOG("Disconnected!\n"); 00118 LOG("Restarting the advertising process\n"); 00119 BLE & ble = BLE::Instance(BLE::DEFAULT_INSTANCE); 00120 ble.gap().startAdvertising(); 00121 // bleIsConnected = false; 00122 } 00123 00124 void tick(void) 00125 { 00126 static int tickerSleepTime=0; 00127 blue = !blue; 00128 00129 //update time 00130 ReadIntervals::updateTimeLeft(tickerSleepTime); 00131 00132 //perform actions 00133 if(ReadIntervals::temperatureSensorPeriodPassed()){ 00134 green = !green; 00135 startMeasure = true; // notify the main-loop to start measuring the MPU6050 00136 } 00137 if(ReadIntervals::batteryMonitorPeriodPassed()){ 00138 red = !red; 00139 batteryVoltageChanged = true; 00140 } 00141 00142 //prep next ticker-sleep 00143 tickerSleepTime = ReadIntervals::getTickerSleepTime(); 00144 ticker.attach(tick, tickerSleepTime); 00145 } 00146 00147 // void detect(void) 00148 // { 00149 // LOG("Button pressed\n"); 00150 // blue = !blue; 00151 // } 00152 00153 void setAdvertisingPayload(BLE & ble){ 00154 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED); 00155 ble.gap().setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00156 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LIST_128BIT_SERVICE_IDS, 00157 (const uint8_t *)acceleration128bitUUIDlist, sizeof(acceleration128bitUUIDlist)); 00158 } 00159 00160 void setScanResponsePayload(BLE & ble){ 00161 uint8_t name[] = "iot aabbccddeeff"; 00162 Gap::AddressType_t addr_type; 00163 Gap::Address_t address; 00164 ble_error_t error = ble.gap().getAddress(&addr_type, address); 00165 if(error == BLE_ERROR_NONE){ 00166 for(int i = 5; i >= 0; i--){ 00167 char buffer[3]; 00168 sprintf(buffer, "%02x", address[i]); 00169 name[4 + ((5-i)*2)] = buffer[0]; 00170 name[4 + ((5-i)*2) + 1] = buffer[1]; 00171 } 00172 } 00173 00174 ble.gap().accumulateScanResponse(GapAdvertisingData::COMPLETE_LOCAL_NAME, 00175 name, sizeof(name)); 00176 } 00177 00178 00179 void onDataWritten(const GattWriteCallbackParams * context) 00180 { 00181 if(context->handle == tempBulkUpdateCharacteristic->getValueHandle()){ 00182 const time_t & newSelectedTime = *(time_t*)context->data; 00183 tempUpdate = temperatureBuffer.getBulkUpdate(newSelectedTime); 00184 tempUpdateFlag = true; 00185 } 00186 } 00187 00188 int main(void) 00189 { 00190 ///Start global timer 00191 AppTime::init(); 00192 00193 ///Initialize led values 00194 blue = 0; 00195 green = 1; 00196 red = 1; 00197 00198 wait(1); 00199 00200 #ifdef USE_SERIAL 00201 pc.baud(115200); 00202 #endif /* USE_SERIAL */ 00203 00204 LOG("---- Seeed Tiny BLE ----\n"); 00205 00206 ///Initialize MPU6050 00207 LOG("MPU6050 testConnection \n"); 00208 bool mpu6050TestResult = mpu.testConnection(); 00209 if(mpu6050TestResult) { 00210 LOG("MPU6050 test passed \n"); 00211 } else { 00212 LOG("MPU6050 test failed \n"); 00213 } 00214 00215 ///Initialize temperature buffer & update 00216 uint16_t temperature = temperatureBuffer.performMeasurement(); 00217 tempUpdate = temperatureBuffer.getBulkUpdate(0); 00218 00219 ///Initialize ticker 00220 ticker.attach(tick, ReadIntervals::TemperatureSensorPeriod); 00221 00222 // button.fall(detect); 00223 00224 ///Initialize BLE 00225 LOG("Initialising the nRF51822\n"); 00226 BLE & ble = BLE::Instance(BLE::DEFAULT_INSTANCE); 00227 ble.init(); 00228 ble.gap().onDisconnection(disconnectionCallback); 00229 ble.gap().onConnection(connectionCallback); 00230 00231 // -> Initialize BLE - custom services 00232 tempBulkUpdateCharacteristic = new GattCharacteristic(uuidTempBulkCharRead, NULL, 1, sizeof(tempUpdate), 00233 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE_WITHOUT_RESPONSE | GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE); 00234 GattService tempBulkService(uuidTempBulkService, &tempBulkUpdateCharacteristic, 1); 00235 ble.gattServer().addService(tempBulkService); 00236 ble.gattServer().onDataWritten(onDataWritten); 00237 00238 // -> Initialize BLE - advertising and scan payloads 00239 setAdvertisingPayload(ble); 00240 setScanResponsePayload(ble); 00241 00242 // -> Initialize BLE - generic services 00243 DFUService dfu(ble); 00244 UARTService uartService(ble); 00245 BatteryService batteryService(ble); 00246 DeviceInformationService deviceInfo(ble, MANUFACTURER, MODELNUMBER, SERIALNUMBER, HARDWAREREVISION, FIRMWAREREVISION, SOFTWAREREVISION); 00247 TemperatureService tempService(ble); 00248 AccelerationService accelerationService(ble); 00249 00250 // -> Initialize BLE - start advertising 00251 ble.gap().setAdvertisingInterval(160); // 100ms; in multiples of 0.625ms. 00252 ble.gap().startAdvertising(); 00253 00254 tempService.updateTemperature(temperature); 00255 00256 while(true) { 00257 ble.waitForEvent(); 00258 00259 // update battery level after the level is measured 00260 if(batteryVoltageChanged == true) { 00261 float batteryVoltage = battery.read(); 00262 // LOG("VBat: %4.3f, ADC: %4.3f, Vadc: %4.3f\n", batteryVoltage*2.0f, batteryVoltage, batteryVoltage*3.3f); 00263 LOG("VBat: %s, ADC: %s, Vadc: %s\n", floatToCharArray(batteryVoltage*2.0f), floatToCharArray(batteryVoltage), floatToCharArray(batteryVoltage*3.3f)); 00264 batteryService.updateBatteryLevel((uint8_t)(batteryVoltage*100.0f)); // input is 0-1.0 of 3.3V -> *100 = percentage of 3.3V 00265 batteryVoltageChanged = false; 00266 } 00267 00268 if(startMeasure == true) { 00269 //uartService.write("test\n", 5); 00270 //float a[3]; 00271 //mpu.getAccelero(a); 00272 //LOG("Acceleration %.2f;%.2f;%.2f\n", a[0], a[1], a[2]); 00273 00274 ///Acceleration 00275 //read accelerometer data 00276 int a[3]; 00277 mpu.getAcceleroRaw(a); 00278 //convert to format accepted by accelerionService 00279 int16_t a_16[3]; 00280 castArray(a, a_16, 3); //cannot simply cast 'a' to int16_t*, memory won't move accordingly 00281 // update BLE acceleration 00282 accelerationService.addAcceleration(a_16); 00283 LOG("Acceleration 0x%x;0x%x;0x%x\n", a[0], a[1], a[2]); 00284 00285 ///Temperature 00286 uint16_t temperature = temperatureBuffer.performMeasurement(); 00287 tempService.updateTemperature(temperature); 00288 00289 ///Reset measure flag 00290 startMeasure = false; 00291 } 00292 00293 if(tempUpdateFlag){ 00294 ble_error_t err = ble.gattServer().write(tempBulkUpdateCharacteristic->getValueHandle(), (const uint8_t*)&tempUpdate, sizeof(tempUpdate)); 00295 LOG("err:[%d]\n", err); 00296 LOG("rT:[%d]\n", tempUpdate.requestedTime); 00297 LOG("iT:[%d]\n", tempUpdate.initialTime); 00298 LOG("nR:[%d]\n", tempUpdate.numberOfReadings); 00299 LOG("pT:[%s]\n", floatToCharArray(tempUpdate.sensorReadingInterval)); 00300 // LOG("pT:[%f]\n", tempUpdate.sensorReadingInterval); 00301 for(unsigned int i=0; i < tempUpdate.numberOfReadings; i++){ 00302 LOG("%#010x\n", tempUpdate.readings[i]); 00303 } 00304 tempUpdateFlag = false; 00305 } 00306 } 00307 } 00308 00309
Generated on Sat Jul 23 2022 08:14:02 by
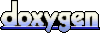