
Pull request for i.a. sensor buffer template
Dependencies: BLE_API MPU6050 mbed nRF51822
Util.h
00001 #ifndef UTIL_H 00002 #define UTIL_H 00003 00004 #include <string> 00005 // #include <vector> 00006 00007 template<typename T_FromType, typename T_ToType> 00008 inline void castArray(T_FromType * fromArray, T_ToType * toArray, const unsigned int & length){ 00009 for(unsigned int i=0; i < length; i++){ 00010 toArray[i] = fromArray[i]; 00011 } 00012 } 00013 00014 // inline std::vector<std::string> split(std::string receivedMessage, const char & delimiter = ' '){ 00015 // std::vector<std::string> ret; 00016 // size_t wordEnd = receivedMessage.find_first_of(delimiter); 00017 // while(wordEnd != std::string::npos){ 00018 // std::string word = receivedMessage.substr(0, wordEnd); 00019 // if(!word.empty()){ 00020 // ret.push_back(word); 00021 // } 00022 // receivedMessage = receivedMessage.substr(wordEnd+1); 00023 // wordEnd = receivedMessage.find_first_of(delimiter); 00024 // } 00025 // if(!receivedMessage.empty()){ 00026 // ret.push_back(receivedMessage); 00027 // } 00028 00029 // return ret; 00030 // } 00031 00032 00033 inline char digitToChar(uint8_t digit){ 00034 return ('0' + digit); //ascii value + digit value = ascii value 00035 } 00036 00037 inline std::string floatToString(float value, int maximumNumberOfDecimals = 5){ //Temporary solution to solve logging of float values 00038 //First make sure buffer is big enough 00039 std::string ret; 00040 int integersLength = 0; 00041 int valueInt = static_cast<int>(value); 00042 00043 //integer part 00044 if(valueInt){ 00045 integersLength = 1; 00046 } 00047 while(valueInt /= 10){ //assuming decimal system 00048 integersLength++; 00049 value /= 10.0f; 00050 } 00051 00052 for(int i=0; i < integersLength; i++){ 00053 uint8_t digit = (uint8_t) value; 00054 ret += digitToChar(digit); 00055 value -= digit; 00056 value *= 10.0f; 00057 } 00058 00059 //decimal point 00060 ret += "."; 00061 00062 //decimal part 00063 int decimalLength = 0; 00064 while((value != 0.0f) && (decimalLength < maximumNumberOfDecimals)){ 00065 uint8_t digit = (uint8_t) value; 00066 ret += digitToChar(digit); 00067 decimalLength++; 00068 value -= digit; 00069 value *= 10.0f; 00070 } 00071 00072 if((decimalLength == 0) && (decimalLength < maximumNumberOfDecimals)){ 00073 ret += "0"; 00074 } 00075 ret += "f"; 00076 00077 return ret; 00078 } 00079 00080 #define floatToCharArray(...) floatToString(__VA_ARGS__).c_str() 00081 00082 #endif /* UTIL_H */
Generated on Sat Jul 23 2022 08:14:02 by
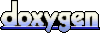