
Pull request for i.a. sensor buffer template
Dependencies: BLE_API MPU6050 mbed nRF51822
TemperatureService.h
00001 #ifndef __TEMPERATURE_SERVICE_H__ 00002 #define __TEMPERATURE_SERVICE_H__ 00003 00004 #include "ble/BLE.h" 00005 00006 #define UUID_TEMPERATURE_CELSIUS_CHAR 0x2A1F 00007 #define UUID_TEMPERATURE_SERVICE "5D34E2F0-3DD2-07AC-38F1-BBAD120EF852" 00008 00009 00010 /** 00011 * @class TemperatureService 00012 * @brief BLE Temperature Service. This service displays the temperature, represented as an 16bit number. 00013 */ 00014 class TemperatureService { 00015 public: 00016 /** 00017 * @param[in] _ble 00018 * BLE object for the underlying controller. 00019 * @param[in] temperature 00020 * 16bit temperature in 0.1 degrees Celsius 00021 */ 00022 TemperatureService (BLE &_ble, int16_t temperature = 200) : 00023 ble(_ble), 00024 temperature(temperature), 00025 temperatureCharacteristic(UUID_TEMPERATURE_CELSIUS_CHAR, &temperature, GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY) { 00026 00027 GattCharacteristic *charTable[] = {&temperatureCharacteristic}; 00028 GattService TemperatureService (UUID(UUID_TEMPERATURE_SERVICE), charTable, sizeof(charTable) / sizeof(GattCharacteristic *)); 00029 00030 ble.addService(TemperatureService); 00031 } 00032 00033 /** 00034 * @brief Update the temperature with a new value. Valid values lie between -2732 and above. 00035 * 00036 * @param newTemp 00037 * Update to temperature. 00038 */ 00039 void updateTemperature(int16_t newTemp) { 00040 temperature = newTemp; 00041 uint16_t temp = (uint16_t)temperature; 00042 ble.gattServer().write(temperatureCharacteristic.getValueHandle(), (const uint8_t*)&temp, sizeof(temperature)); 00043 } 00044 00045 protected: 00046 /** 00047 * A reference to the underlying BLE instance that this object is attached to. 00048 * The services and characteristics will be registered in this BLE instance. 00049 */ 00050 BLE &ble; 00051 00052 /** 00053 * The current temperature represented as 0.1 degrees Celsius. 00054 */ 00055 int16_t temperature; 00056 /** 00057 * A ReadOnlyGattCharacteristic that allows access to the peer device to the 00058 * temperature value through BLE. 00059 */ 00060 ReadOnlyGattCharacteristic<int16_t> temperatureCharacteristic; 00061 }; 00062 00063 #endif /* #ifndef __TEMPERATURE_SERVICE_H__*/
Generated on Sat Jul 23 2022 08:14:02 by
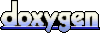