
Pull request for i.a. sensor buffer template
Dependencies: BLE_API MPU6050 mbed nRF51822
AccelerationService.h
00001 #ifndef __ACCELERATION_SERVICE_H__ 00002 #define __ACCELERATION_SERVICE_H__ 00003 00004 #include "ble/BLE.h" 00005 00006 #define UUID_ACCELERATION_X_CHAR "5D34E2F0-3DD2-07AC-38F2-BBAD120EF853" 00007 #define UUID_ACCELERATION_Y_CHAR "5D34E2F0-3DD2-07AC-38F3-BBAD120EF853" 00008 #define UUID_ACCELERATION_Z_CHAR "5D34E2F0-3DD2-07AC-38F4-BBAD120EF853" 00009 #define UUID_ACCELERATION_SERVICE "5D34E2F0-3DD2-07AC-38F1-BBAD120EF853" 00010 00011 /** 00012 * @class AccelerationService 00013 * @brief BLE Acceleration Service. This service displays the acceleration 00014 */ 00015 class AccelerationService { 00016 public: 00017 /** 00018 * @param[in] _ble 00019 * BLE object for the underlying controller. 00020 */ 00021 AccelerationService (BLE &_ble) : 00022 ble(_ble), 00023 accelerationIndex(0), 00024 accelerationXCharacteristic(UUID(UUID_ACCELERATION_X_CHAR), &temp, GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY), 00025 accelerationYCharacteristic(UUID(UUID_ACCELERATION_Y_CHAR), &temp, GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY), 00026 accelerationZCharacteristic(UUID(UUID_ACCELERATION_Z_CHAR), &temp, GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY) { 00027 00028 GattCharacteristic *charTable[] = {&accelerationXCharacteristic, &accelerationYCharacteristic, &accelerationZCharacteristic}; 00029 GattService AccelerationService (UUID(UUID_ACCELERATION_SERVICE), charTable, sizeof(charTable) / sizeof(GattCharacteristic *)); 00030 00031 ble.addService(AccelerationService); 00032 } 00033 00034 /** 00035 * @brief Update the temperature with a new value. Valid values lie between -2732 and above. 00036 * 00037 * @param newTemp 00038 * Update to temperature. 00039 */ 00040 void addAcceleration(int16_t *newAccel) { 00041 temp = newAccel[0]; 00042 ble.gattServer().write(accelerationXCharacteristic.getValueHandle(), (const uint8_t*)&temp, sizeof(temp)); 00043 temp = newAccel[1]; 00044 ble.gattServer().write(accelerationYCharacteristic.getValueHandle(), (const uint8_t*)&temp, sizeof(temp)); 00045 temp = newAccel[2]; 00046 ble.gattServer().write(accelerationZCharacteristic.getValueHandle(), (const uint8_t*)&temp, sizeof(temp)); 00047 } 00048 00049 00050 protected: 00051 /** 00052 * A reference to the underlying BLE instance that this object is attached to. 00053 * The services and characteristics will be registered in this BLE instance. 00054 */ 00055 BLE &ble; 00056 00057 /** 00058 * The current temperature represented as 0.1 degrees Celsius. 00059 */ 00060 //int16_t acceleration[30][3]; 00061 int16_t temp; 00062 00063 uint16_t accelerationIndex; 00064 /** 00065 * A ReadOnlyGattCharacteristic that allows access to the peer device to the 00066 * temperature value through BLE. 00067 */ 00068 ReadOnlyGattCharacteristic<int16_t> accelerationXCharacteristic; 00069 ReadOnlyGattCharacteristic<int16_t> accelerationYCharacteristic; 00070 ReadOnlyGattCharacteristic<int16_t> accelerationZCharacteristic; 00071 }; 00072 00073 #endif /* #ifndef __ACCELERATION_SERVICE_H__*/
Generated on Sat Jul 23 2022 08:14:02 by
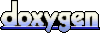