
ADT7410 sample
Dependencies: ADT7410 BLE_API mbed nRF51822
Fork of BLENano_SimpleTemplate_temp_170802 by
main.cpp
00001 /* 00002 00003 Copyright (c) 2012-2014 RedBearLab 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00006 and associated documentation files (the "Software"), to deal in the Software without restriction, 00007 including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, 00009 subject to the following conditions: 00010 The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. 00011 00012 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, 00013 INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR 00014 PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE 00015 FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00016 ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 00018 */ 00019 00020 /* 00021 * The application works with the BlueJelly.js 00022 * 00023 * http://jellyware.jp/ 00024 * 00025 */ 00026 00027 //====================================================================== 00028 //Grobal 00029 //====================================================================== 00030 //------------------------------------------------------------ 00031 //Include Header Files 00032 //------------------------------------------------------------ 00033 #include "mbed.h" 00034 #include "ble/BLE.h" 00035 #include "ADT7410.h" 00036 00037 //------------------------------------------------------------ 00038 //Definition 00039 //------------------------------------------------------------ 00040 #define TXRX_BUF_LEN 20 //max 20[byte] 00041 #define DEVICE_LOCAL_NAME "ADT7410 Temperature" //Change Device name 00042 #define ADVERTISING_INTERVAL 160 //160 * 0.625[ms] = 100[ms] 00043 #define TICKER_TIME 200000 //200000[us] = 200[ms] 00044 #define DIGITAL_OUT_PIN P0_9 00045 //#define ANALOG_IN_PIN P0_4 00046 00047 00048 //------------------------------------------------------------ 00049 //Object generation 00050 //------------------------------------------------------------ 00051 BLE ble; 00052 DigitalOut LED_SET(DIGITAL_OUT_PIN); 00053 //AnalogIn ANALOG(ANALOG_IN_PIN); 00054 00055 //I2C Pin setting P0_4=SDA, P0_5=SCL 00056 ADT7410 temp(P0_4, P0_5, 0x90, 10000); 00057 00058 //------------------------------------------------------------ 00059 //Service & Characteristic Setting 00060 //------------------------------------------------------------ 00061 //Service UUID 00062 static const uint8_t base_uuid[] = { 0x71, 0x3D, 0x00, 0x00, 0x50, 0x3E, 0x4C, 0x75, 0xBA, 0x94, 0x31, 0x48, 0xF1, 0x8D, 0x94, 0x1E } ; 00063 00064 //Characteristic UUID 00065 static const uint8_t tx_uuid[] = { 0x71, 0x3D, 0x00, 0x03, 0x50, 0x3E, 0x4C, 0x75, 0xBA, 0x94, 0x31, 0x48, 0xF1, 0x8D, 0x94, 0x1E } ; 00066 static const uint8_t rx_uuid[] = { 0x71, 0x3D, 0x00, 0x02, 0x50, 0x3E, 0x4C, 0x75, 0xBA, 0x94, 0x31, 0x48, 0xF1, 0x8D, 0x94, 0x1E } ; 00067 00068 //Characteristic Value 00069 uint8_t txPayload[TXRX_BUF_LEN] = {0,}; 00070 uint8_t rxPayload[TXRX_BUF_LEN] = {0,}; 00071 00072 //Characteristic Property Setting etc 00073 GattCharacteristic txCharacteristic (tx_uuid, txPayload, 1, TXRX_BUF_LEN, GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE | GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ); 00074 GattCharacteristic rxCharacteristic (rx_uuid, rxPayload, 1, TXRX_BUF_LEN, GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY| GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ); 00075 GattCharacteristic *myChars[] = {&txCharacteristic, &rxCharacteristic}; 00076 00077 //Service Setting 00078 GattService myService(base_uuid, myChars, sizeof(myChars) / sizeof(GattCharacteristic *)); 00079 00080 00081 //====================================================================== 00082 //onDisconnection 00083 //====================================================================== 00084 void disconnectionCallback(const Gap::DisconnectionCallbackParams_t *params) 00085 { 00086 ble.startAdvertising(); 00087 } 00088 00089 00090 //====================================================================== 00091 //onDataWritten 00092 //====================================================================== 00093 void WrittenHandler(const GattWriteCallbackParams *Handler) 00094 { 00095 uint8_t buf[TXRX_BUF_LEN]; 00096 uint16_t bytesRead; 00097 00098 if (Handler->handle == txCharacteristic.getValueAttribute().getHandle()) 00099 { 00100 ble.readCharacteristicValue(txCharacteristic.getValueAttribute().getHandle(), buf, &bytesRead); 00101 memset(txPayload, 0, TXRX_BUF_LEN); 00102 memcpy(txPayload, buf, TXRX_BUF_LEN); 00103 00104 if(buf[0] == 1) 00105 LED_SET = 1; 00106 else 00107 LED_SET = 0; 00108 } 00109 } 00110 00111 00112 //====================================================================== 00113 //onTimeout 00114 //====================================================================== 00115 00116 00117 void m_status_check_handle(void) 00118 { 00119 uint8_t buf[2]; 00120 float tempadt; 00121 00122 // get temperature every two seconds 00123 tempadt = temp.getTemp(); 00124 00125 int16_t value0 = tempadt; //Get integer value 00126 int16_t value1 = (tempadt - value0)*100; //Get decimal value 00127 buf[0] = value0; 00128 buf[1] = value1; 00129 00130 //Send out 00131 ble.updateCharacteristicValue(rxCharacteristic.getValueAttribute().getHandle(), buf, 2); 00132 00133 } 00134 00135 //====================================================================== 00136 //convert reverse UUID 00137 //====================================================================== 00138 void reverseUUID(const uint8_t* src, uint8_t* dst) 00139 { 00140 int i; 00141 00142 for(i=0;i<16;i++) 00143 dst[i] = src[15 - i]; 00144 } 00145 00146 00147 //====================================================================== 00148 //main 00149 //====================================================================== 00150 int main(void) 00151 { 00152 uint8_t base_uuid_rev[16]; 00153 00154 //Timer Setting [us] 00155 Ticker ticker; 00156 ticker.attach_us(m_status_check_handle, TICKER_TIME); 00157 00158 // reset sensor to default values 00159 temp.reset(); 00160 00161 // reduce sample rate to save power 00162 temp.setConfig(ONE_SPS_MODE); 00163 00164 //BLE init 00165 ble.init(); 00166 00167 //EventListener 00168 ble.onDisconnection(disconnectionCallback); 00169 ble.onDataWritten(WrittenHandler); 00170 00171 //------------------------------------------------------------ 00172 //setup advertising 00173 //------------------------------------------------------------ 00174 //Classic BT not support 00175 ble.accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED); 00176 00177 //Connectable to Central 00178 ble.setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00179 00180 //Local Name 00181 ble.accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LOCAL_NAME, 00182 (const uint8_t *)DEVICE_LOCAL_NAME, sizeof(DEVICE_LOCAL_NAME) - 1); 00183 00184 //GAP AdvertisingData 00185 reverseUUID(base_uuid, base_uuid_rev); 00186 ble.accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LIST_128BIT_SERVICE_IDS, 00187 (uint8_t *)base_uuid_rev, sizeof(base_uuid)); 00188 00189 //Advertising Interval 00190 ble.setAdvertisingInterval(ADVERTISING_INTERVAL); 00191 00192 //Add Service 00193 ble.addService(myService); 00194 00195 //Start Advertising 00196 ble.startAdvertising(); 00197 00198 //------------------------------------------------------------ 00199 //Loop 00200 //------------------------------------------------------------ 00201 while(1) 00202 { 00203 ble.waitForEvent(); 00204 } 00205 }
Generated on Mon Jul 18 2022 01:11:42 by
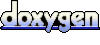