
PID simple
Dependencies: DebouncedIn TextLCD2 mbed
main.cpp
00001 #include "mbed.h" 00002 #include "DebouncedIn.h" 00003 #include "TextLCD.h" 00004 00005 AnalogIn AI(PTC2); 00006 AnalogOut AO(PTE30); 00007 TextLCD lcd(PTB10, PTB11, PTE2, PTE3, PTE4, PTE5); // Rs, E, D4-D7 00008 00009 DigitalOut led1(LED1); 00010 DigitalOut led2(LED2); 00011 DebouncedIn button1(PTC12); 00012 DebouncedIn button2(PTC13); 00013 DebouncedIn button3(PTA1); 00014 DebouncedIn button4(PTC16); 00015 00016 Timer t; 00017 int flag; 00018 00019 00020 /* Definiendo variables Globales */ 00021 00022 int C1=0x0E; // solo muestra el curzor 00023 int C2=0x18; // desplaza izquierda 00024 int C3=0x1A; // desplaza derecha 00025 int C4=0x0C; // Quita cursor bajo 00026 int i; // índice de la variable 00027 int j; 00028 int kp, ki, kd, sp, cycle; 00029 int ap, ai, ad, err, PV, pid, err_v; 00030 /* Programa fundamental*/ 00031 00032 00033 int main() 00034 { 00035 /* Imprimir en Pantalla Los labels y valores=? de 00036 * los parametros del PID 00037 */ 00038 00039 lcd.cls(); //Borra la Pantalla 00040 lcd.printf("Sp%d",sp); 00041 lcd.locate(8,0); //sistema coordenado para posicionar en pantalla 00042 lcd.printf("Kp%d",kp); 00043 lcd.locate(0,1); 00044 lcd.printf("Ki%d",ki); 00045 lcd.locate(8,1); 00046 lcd.printf("Kd%d",kd); 00047 lcd.writeCommand(C1);//escribimos un comando segun el manual del modulo LCD 00048 /* No me cuadra muy bien la 00049 * lectura de los valores (consultar en las otras tareas) 00050 */ 00051 lcd.locate(0,0); 00052 lcd.printf("Sp%d",sp); 00053 00054 /* Ciclo while para alterar los valores de los parametros*/ 00055 while (1) 00056 { 00057 if (button3.falling()) //Boton con flanco de caida 00058 { 00059 ++j; 00060 } 00061 if (j==0) //Este if realiza dos revisiones y actua solo en este valor (SP) 00062 { 00063 lcd.locate(2,0); 00064 lcd.printf("%d",sp); //sigo con la inquietud del write lectura 00065 if (button1.falling()) 00066 { 00067 ++sp; 00068 } 00069 if (button2.falling()) 00070 { 00071 --sp; 00072 } 00073 } 00074 if (j==1) //Verificar si estos if son mas efectivos quetus Whiles Tarea1 00075 { 00076 lcd.locate(10,0); 00077 lcd.printf("%d",kp); 00078 if (button1.falling()) 00079 { 00080 ++kp; 00081 } 00082 if (button2.falling()) 00083 { 00084 --kp; 00085 } 00086 } 00087 if (j==2) 00088 { 00089 lcd.locate(2,1); 00090 lcd.printf("%d",ki); 00091 if (button1.falling()) 00092 { 00093 ++ki; 00094 } 00095 if (button2.falling()) 00096 { 00097 --ki; 00098 } 00099 } 00100 if (j==3) 00101 { 00102 lcd.locate(10,1); 00103 lcd.printf("%d",kd); 00104 if (button1.falling()) 00105 { 00106 ++kd; 00107 } 00108 if (button2.falling()) 00109 { 00110 --kd; 00111 } 00112 } 00113 if (j==4) //Este if realiza dos revisiones y actua solo en este valor (SP) 00114 { 00115 j=0; 00116 } 00117 if (button4.falling()) 00118 { 00119 break; //Salir de este ciclo while 00120 } 00121 } 00122 /* Interaccion y avisos al usuario de sistema listo!!*/ 00123 lcd.writeCommand(C4);//escribimos un comando segun el manual del modulo LCD para quitar cursor bajo 00124 lcd.cls(); 00125 lcd.printf("DATOS GUARDADOS"); 00126 wait(2); 00127 lcd.cls(); 00128 lcd.printf("INICIA EL PID"); 00129 wait(2); 00130 lcd.cls(); 00131 /* Imprimir los labels de algunas variables y parametros de Control en Ejecucion */ 00132 lcd.printf("Er%g",err); 00133 lcd.locate(8,0); 00134 lcd.printf("Me%g",PV); 00135 lcd.locate(0,1); 00136 lcd.printf("SP%d",sp); 00137 lcd.locate(8,1); 00138 lcd.printf("Co%g",pid); 00139 /*Ziegler–Nichols_method*/ 00140 /*kp=((kp*3.3)/(kp*4.5)); 00141 ki=2*kp; 00142 kd=kp/8*/; 00143 wait(.5); 00144 00145 /* Ciclo while contiene el codigo especifico rutiona del PID*/ 00146 while (1) 00147 { 00148 /* pilas con leer el puerto analogo y asignarlo a med*/ 00149 PV = AI.read(); 00150 PV =PV*1000; 00151 err =sp-PV; 00152 err=err/100; 00153 ap=kp*err; //Proporcional incremento en funcion del error Parte P 00154 ai=(ki*err)+ai; //Acumula los errores Parte I 00155 ad=kd*(err-err_v); // tiene en cuenta el delta del error Parte D 00156 pid=ap+ai+ad; // comentar esta y ponerla despues de comentar los ifs 00157 if (ai>999) 00158 { 00159 ai=1000; 00160 } 00161 else if (pid<0) 00162 { 00163 pid=0; 00164 } 00165 AO.write(pid/1000); 00166 if(flag==0) 00167 { 00168 t.start(); 00169 flag=1; 00170 } 00171 00172 //AO=pid; 00173 if (t>0.3) 00174 { 00175 /* Imprimir algunas variables y parametros de Control en Ejecucion */ 00176 lcd.locate(2,0); 00177 printf("%g",err); 00178 lcd.locate(2,1); 00179 printf("%d",sp); 00180 lcd.locate(10,0); 00181 printf("%g",PV); 00182 lcd.locate(10,1); 00183 printf("%g",pid); 00184 t.reset(); 00185 flag=0; 00186 wait(.3); 00187 } 00188 00189 err_v = err; 00190 00191 00192 } 00193 00194 00195 } 00196 00197
Generated on Tue Jul 26 2022 21:24:45 by
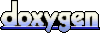