Logibotの動作用の基本ライブラリです
Dependents: Logibot_Temp Motor_Temp
beep_sound.cpp
00001 #include "beep_sound.h" 00002 #include "math.h" 00003 #include <queue> 00004 00005 00006 beep_sound::beep_sound(PinName pwm) 00007 { 00008 frequency = 0; 00009 playing = false; 00010 teishi = false; 00011 m_pwm = new PwmOut(pwm); 00012 m_pwm->write(0.0f); 00013 } 00014 00015 beep_sound::~beep_sound() 00016 { 00017 delete m_pwm; 00018 } 00019 00020 void beep_sound::stop(){ 00021 m_pwm->write(0.0f); 00022 // キューが空でなければ次の音へ 00023 if(otoQue.empty() == true){ 00024 playing = false; 00025 }else if(otoQue.front().time_s != -1){ 00026 playing = true; 00027 beep_sound::playContinue(); 00028 }else if(otoQue.front().time_s == -1){ 00029 otoQue.pop(); 00030 playing = false; 00031 }else{ 00032 playing = false; 00033 } 00034 } 00035 00036 // 音の再生 00037 void beep_sound::NoteOn(int octave, int note) 00038 { 00039 playing = true; 00040 frequency = frequencyTable[note] << octave; 00041 m_pwm->period(1.0f/(float)frequency); 00042 m_pwm->write(0.5f); 00043 } 00044 00045 // 1音ずつ音符情報をキューに追加 00046 void beep_sound::setGakuhu(int argOc, int argNo, float argTi) 00047 { 00048 bufG.octave = argOc; 00049 bufG.note = argNo; 00050 bufG.time_s = argTi; 00051 otoQue.push(bufG); 00052 } 00053 00054 // 休符をキューに追加 00055 void beep_sound::setKyuhu(float argTi) 00056 { 00057 bufG.octave = 10; 00058 bufG.note = 0; 00059 bufG.time_s = argTi; 00060 otoQue.push(bufG); 00061 } 00062 00063 // 楽譜キューを再生、音停止の割り込み予約 00064 // Timeoutの日本語リファレンスには書いていないが、 00065 // thisを引数にしないとコンパイルエラーになるので注意 00066 void beep_sound::playGakuhu() 00067 { 00068 if(playing == true){ 00069 while(otoQue.front().time_s != -1) {otoQue.pop();} 00070 otoQue.pop(); //最後のフラグを削除 00071 // 最後のフラグを挿入 00072 bufG.time_s = -1; 00073 otoQue.push(bufG); 00074 beep_sound::stop(); 00075 }else{ 00076 // 最後のフラグを挿入 00077 bufG.time_s = -1; 00078 otoQue.push(bufG); 00079 playing = true; 00080 beep_sound::NoteOn(otoQue.front().octave, otoQue.front().note); 00081 interruptStop.attach(this, &beep_sound::stop, otoQue.front().time_s); 00082 otoQue.pop(); 00083 } 00084 } 00085 void beep_sound::playContinue() 00086 { 00087 beep_sound::NoteOn(otoQue.front().octave, otoQue.front().note); 00088 interruptStop.attach(this, &beep_sound::stop, otoQue.front().time_s); 00089 otoQue.pop(); 00090 } 00091 00092 void beep_sound::SetFrequency(int octave, int note, bool on_off) 00093 { 00094 static int preFrequency = 0; 00095 if(on_off == true){ 00096 int frequency = frequencyTable[note] << octave; 00097 if(frequency != preFrequency){ 00098 m_pwm->period(1.0f/(float)frequency); 00099 m_pwm->write(0.5f); 00100 } 00101 preFrequency = frequency; 00102 }else{ 00103 m_pwm->write(0.0f); 00104 preFrequency = 0; 00105 } 00106 } 00107 void beep_sound::onpu(int octave, int note, float time_s) 00108 { 00109 playing = true; 00110 beep_sound::NoteOn(octave, note); 00111 interruptStop.attach(this, &beep_sound::stop, time_s); 00112 } 00113 void beep_sound::onpu_stop(int octave, int note, float time_s) 00114 { 00115 playing = true; 00116 beep_sound::NoteOn(octave, note); 00117 wait(time_s); 00118 m_pwm->write(0.0f); 00119 } 00120 void beep_sound::sinwave(float center,int speed,int width) 00121 { 00122 for(float i=0.0f;i<6.28f;i+=0.01*speed) 00123 { 00124 m_pwm->period(1.0f/(center+width*(float)sin(i))); 00125 m_pwm->write(0.5f); 00126 wait_ms(2); 00127 } 00128 } 00129 void beep_sound::setwave(float frequency,float duty,float wait) 00130 { 00131 m_pwm->period(1.0f/(frequency)); 00132 m_pwm->write(duty); 00133 wait_ms(wait); 00134 } 00135 00136 00137 //以下サンプル音集 00138 //引数の回数だけ鳴らす 00139 void beep_sound::boot() 00140 { 00141 beep_sound::setGakuhu(4,8,0.03); 00142 beep_sound::setGakuhu(0,8,0.03); 00143 beep_sound::setGakuhu(1,8,0.03); 00144 beep_sound::setKyuhu(0.14); 00145 beep_sound::setGakuhu(2,8,0.08); 00146 beep_sound::setGakuhu(3,1,0.16); 00147 beep_sound::playGakuhu(); 00148 } 00149 00150 void beep_sound::beep_right(int nTimes) 00151 { 00152 for(int i=0; i<nTimes; i++){ 00153 beep_sound::setGakuhu(3,11,0.1); 00154 beep_sound::setGakuhu(3,7,0.35); 00155 beep_sound::setKyuhu(0.1); 00156 } 00157 beep_sound::playGakuhu(); 00158 } 00159 void beep_sound::beep_wrong(int nTimes) 00160 { 00161 for (int i = 0; i < nTimes; i++) { 00162 beep_sound::setGakuhu(0, 12, 0.1); 00163 beep_sound::setKyuhu(0.1); 00164 beep_sound::setGakuhu(0, 12, 0.35); 00165 beep_sound::setKyuhu(0.1); 00166 } 00167 beep_sound::playGakuhu(); 00168 } 00169 void beep_sound::beep_readyGo(int nTimes) 00170 { 00171 //for (int i = 0; i < nTimes; i++) { 00172 beep_sound::onpu_stop(1,0,0.5); 00173 wait(0.5); 00174 beep_sound::onpu_stop(1,0,0.5); 00175 wait(0.5); 00176 beep_sound::onpu_stop(1,0,0.5); 00177 wait(0.5); 00178 beep_sound::onpu(2,0,1); 00179 //} 00180 } 00181 void beep_sound::beep_notif1(int nTimes){ 00182 for (int i = 0; i < nTimes; i++) { 00183 beep_sound::setGakuhu(3,5,0.1); 00184 beep_sound::setGakuhu(3,10,0.1); 00185 beep_sound::setGakuhu(4,2,0.1); 00186 } 00187 beep_sound::playGakuhu(); 00188 } 00189 void beep_sound::beep_notif2(int nTimes){ 00190 for (int i = 0; i < nTimes; i++) { 00191 beep_sound::setGakuhu(1,2,0.1); 00192 beep_sound::setGakuhu(1,9,0.1); 00193 beep_sound::setGakuhu(2,2,0.2); 00194 } 00195 beep_sound::playGakuhu(); 00196 }
Generated on Wed Jul 20 2022 11:45:10 by
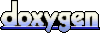