
Program to satellite, send variables of temperature, pressure, RH, GPS
Dependencies: mbed nRF24L01P BMP180 DHT11 GPS
Sensores.cpp
00001 #include "Sensores.h" 00002 #include "mbed.h" 00003 #include "Dht11.h" 00004 #include "BMP180.h" 00005 #include "GPS.h" 00006 #include "nRF24L01P.h" 00007 00008 00009 Serial BT(Rx,Tx); 00010 Dht11 sensor(SENSOR); 00011 I2C i2c(SDA, SCL); //SDA,SCL 00012 BMP180 bmp180(&i2c); 00013 GPS ark(ARK_Rx, ARK_Tx); 00014 DigitalOut myled(LED); 00015 Ticker mTicker; 00016 nRF24L01P my_nrf24l01p(MOSI, MISO, SCK, CSN, CE, IRQ); // mosi, miso, sck, csn, ce, irq 00017 00018 00019 int Temperatura,Humedad, Presion, Altura, Lat, Long; 00020 float Longitud, Latitud, temp; 00021 long UTC,muestreo_sensores=0;; 00022 char hora,minuto,segundo; 00023 int count=0; 00024 int count_enviar=0; 00025 00026 00027 00028 char tab_control[TRANSFER_SIZE]={'T','H','P','A','L','D','U'}; 00029 char valor[TRANSFER_SIZE]; 00030 int variables[7]; 00031 00032 00033 void ticker_callback(){ 00034 count++; 00035 muestreo_sensores++; 00036 count_enviar++; 00037 00038 if(count>10){ 00039 // Temperatura=sensor.getFahrenheit(); 00040 if( ark.sample() == 1) { 00041 // myled=0; 00042 Latitud=ark.latitude; 00043 Longitud=ark.longitude; 00044 UTC=ark.utc+50000; 00045 Altura=ark.alt; 00046 00047 } 00048 // else myled=1; 00049 count=0; 00050 } 00051 00052 if(muestreo_sensores>200) { 00053 sensor.read(); 00054 Humedad=sensor.getHumidity(); 00055 muestreo_sensores=0; 00056 } 00057 00058 if(count_enviar>5) { 00059 enviar_datos(); 00060 myled=!myled; 00061 count_enviar=0; 00062 } 00063 00064 } 00065 00066 //////////////////////////////////////////////////////////////////////////////// 00067 void init_serial(void){ 00068 BT.baud(115200); 00069 } 00070 00071 void init_transmisor(void){ 00072 00073 my_nrf24l01p.powerUp(); 00074 my_nrf24l01p.setTransferSize( TRANSFER_SIZE ); 00075 my_nrf24l01p.setTransmitMode(); 00076 my_nrf24l01p.enable(); 00077 } 00078 00079 void init_sensores(void){ 00080 mTicker.attach_us(&ticker_callback,10000); //(funtion(),tiempo de interrupcion(seg)) 00081 bmp180.init(); 00082 } 00083 00084 void actualizar(void){ 00085 00086 bmp180.startTemperature(); 00087 wait_ms(5); 00088 if(bmp180.getTemperature(&temp) != 0) { 00089 // BT.printf("Error getting temperature\n"); 00090 } 00091 00092 bmp180.startPressure(BMP180::ULTRA_LOW_POWER); 00093 wait_ms(10); // Wait for conversion to complete 00094 if(bmp180.getPressure(&Presion) != 0) { 00095 // BT.printf("Error getting pressure\n"); 00096 } 00097 00098 00099 00100 } 00101 00102 void enviar_datos(void){ 00103 presion(); 00104 sensor_dht11(); 00105 global(); 00106 transmitir(); 00107 } 00108 00109 ////////////////////////////////////////////////////////////////////////////////Enviar data 00110 00111 void presion(void){ 00112 00113 00114 Temperatura=temp*100; 00115 variables[0]=Temperatura; 00116 variables[2]=Presion; 00117 /* 00118 sprintf(valor,"T%06d",Temperatura); 00119 00120 BT.printf(valor); 00121 BT.printf("\n");*/ 00122 } 00123 00124 void sensor_dht11(void){ 00125 variables[1]=Humedad; 00126 } 00127 00128 void global(void){ 00129 00130 Lat=Latitud*1000; 00131 Long=Longitud*100; 00132 00133 variables[3]=Altura; 00134 variables[4]=Lat; 00135 variables[5]=Long; 00136 variables[6]=UTC; 00137 00138 } 00139 00140 //////////////////////////////////////////////////////////////////////////////// 00141 void transmitir(void){ 00142 for(int i=0; i<=6; i++){ 00143 00144 sprintf(valor,"%c%6d",tab_control[i],variables[i]); 00145 00146 // If the transmit buffer is full 00147 if ( TRANSFER_SIZE >= sizeof( valor) ) { 00148 BT.printf("Sending Message... "); 00149 // Send the transmitbuffer via the nRF24L01+ 00150 my_nrf24l01p.write( NRF24L01P_PIPE_P0,valor, TRANSFER_SIZE ); 00151 BT.printf("Message Sent\n"); 00152 } 00153 } 00154 } 00155 00156 00157 00158
Generated on Tue Jul 19 2022 09:12:18 by
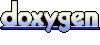