Library to use remote control Spektrum AR6210
Embed:
(wiki syntax)
Show/hide line numbers
AR6210.h
00001 #pragma once 00002 // Original code: https://mbed.org/users/BlazeX/code/AR8000/ 00003 // Modified by Joram Querner for Spektrum AR6210 00004 00005 // Interrupt callback 00006 #define AR6210_RISE_FALL(Ch)\ 00007 void Rise##Ch()\ 00008 {\ 00009 LastRise[Ch]= Time.read_us();\ 00010 }\ 00011 void Fall##Ch()\ 00012 {\ 00013 int dT= Time.read_us() - LastRise[Ch];\ 00014 if(dT > 900 && dT < 2100)\ 00015 dTime[Ch]= dT;\ 00016 } 00017 00018 00019 class AR6210 00020 { 00021 private: 00022 InterruptIn ChInt0; 00023 InterruptIn ChInt1; 00024 InterruptIn ChInt2; 00025 InterruptIn ChInt3; 00026 InterruptIn ChInt4; 00027 InterruptIn ChInt5; 00028 00029 Timer Time; 00030 volatile int LastRise[6]; //Zeitpunkt der letzten steigende Flanke 00031 volatile int dTime[6]; //Pulsdauer in us [1000...2000] 00032 00033 float map(int x, int in_min, int in_max, float out_min, float out_max); 00034 00035 public: 00036 int RawChannels[6]; //Rohdaten [1000...2000] 00037 00038 //Die Steuerbefehle 00039 float Throttle; //0=Aus, 1=Vollgas 00040 float Aileron; //-1=Links, 0=Nichts, +1=Rechts 00041 float Elevator; //-1=Sinken, 0=Nichts, +1=Steigen 00042 float Rudder; //-1=Links, 0=Nichts, +1=Rechts 00043 00044 float Gear; //-1...+1 Left Trim 00045 float Aux; //-1...+1 Right Trim 00046 00047 //Initialisieren 00048 AR6210(); 00049 void init(); 00050 void update(); 00051 00052 //Interrupt-Callbacks definieren 00053 AR6210_RISE_FALL(0); 00054 AR6210_RISE_FALL(1); 00055 AR6210_RISE_FALL(2); 00056 AR6210_RISE_FALL(3); 00057 AR6210_RISE_FALL(4); 00058 AR6210_RISE_FALL(5); 00059 };
Generated on Thu Jul 14 2022 13:37:52 by
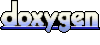