
Touch screen drivers control dashboard for miniature locomotive. Features meters for speed, volts, power. Switches for lights, horns. Drives multiple STM3_ESC brushless motor controllers for complete brushless loco system as used in "The Brute" - www.jons-workshop.com
Dependencies: TS_DISCO_F746NG mbed Servo LCD_DISCO_F746NG BSP_DISCO_F746NG QSPI_DISCO_F746NG AsyncSerial FastPWM
Electric_Loco.h
00001 #include "mbed.h" 00002 #include "movingcoilmeter.h" 00003 #include <cctype> 00004 /* Updated January 2019 00005 Jon Freeman 00006 00007 5" and 7.25" gauge Electric Locomotive Controller - ST DISCO-F746NG 00008 Uses built in display and touch screen. 00009 00010 Display shows 'analogue' moving coil meter movements for : 00011 Locomotive speed Miles per Hour 00012 System voltage (range 10v - 63v or thereabouts) 00013 Power Watts delivered to drive motors or dumped. 00014 00015 Touch screen has three 'buttons', and are where the meter movements show. 00016 Two smaller meter buttons for two horns, larger speedo button currently unsused 00017 00018 Display has 'slider' touch control. This drives the loco. 00019 Control in central position when not driving or drfting. 00020 Moving towards bottom of screen applies regenerative braking - move further down applies harder braking. 00021 Moving towards top of screen powers drive motors, move further up applies more torque (current controller implemented) 00022 Take finger off and control drifts down to central 'neutral' position. 00023 */ 00024 00025 00026 #define MAX_TOUCHES 6 // Touch screen can decode up to this many simultaneous finger press positions 00027 #define NEUTRAL_VAL 150 // Number of pixels 00028 00029 #define SLIDERX 418 // slider graphic x position 00030 #define SLIDERY 2 // slider graphic y position 00031 #define SLIDERW 50 // pixel width of slider 00032 #define SLIDERH 268 // pixel height of slider 00033 00034 #define VOLTMETER_X 68 // Voltmeter screen position 00035 #define VOLTMETER_Y 68 00036 #define AMMETER_X 68 // Ammeter screen position - Now replaced by Power meter 00037 #define AMMETER_Y 202 00038 #define SPEEDO_X 274 // Speedometer screen position 00039 #define SPEEDO_Y 135 00040 00041 #define V_A_SIZE 56 // Size of voltmeter and ammeter 00042 #define SPEEDO_SIZE 114 00043 00044 #define SPEEDO_BODY_COLOUR LCD_COLOR_BLACK 00045 #define SPEEDO_DIAL_COLOUR LCD_COLOR_WHITE 00046 #define SPEEDO_TEXT_COLOUR LCD_COLOR_BLUE 00047 00048 #define VMETER_BODY_COLOUR LCD_COLOR_BLACK 00049 #define VMETER_DIAL_COLOUR LCD_COLOR_WHITE 00050 #define VMETER_TEXT_COLOUR LCD_COLOR_BLUE 00051 00052 #define AMETER_BODY_COLOUR LCD_COLOR_BLACK 00053 #define AMETER_DIAL_COLOUR LCD_COLOR_WHITE 00054 #define AMETER_TEXT_COLOUR LCD_COLOR_BLUE 00055 const int 00056 BUTTON_RAD = (SLIDERW / 2) - 4, // radius of circular 'knob' in slider control 00057 MIN_POS = BUTTON_RAD + 5, // top of screen 00058 MAX_POS = SLIDERH - (BUTTON_RAD + 1), // bottom of screen 00059 CIRC_CTR = SLIDERX + BUTTON_RAD + 4; 00060 00061 static const double PI = 4.0 * atan(1.0); 00062 static const double TWO_PI = 8.0 * atan(1.0); 00063 00064 enum {HI_HORN, LO_HORN}; 00065 enum {NO_DPS, ONE_DP}; 00066 // Assign unique number to every button we may use, and keep count of total number of them 00067 enum {ENTER, SLIDER_BUTTON, SPEEDO_BUTTON, VMETER_BUTTON, AMETER_BUTTON,NUMOF_BUTTONS} ; // button names 00068 enum {STATES, RUN, NEUTRAL_DRIFT, REGEN_BRAKE, RUN_DOWN, INTO_RUN, INTO_REGEN_BRAKE, INTO_NEUTRAL_DRIFT}; 00069 enum {SLIDER_PRESS, SLIDER_RELEASE, SLIDER_AUTOREP}; 00070 00071 struct point { int x; int y; } ; 00072 struct keystr { int keynum; int x; int y; } ; 00073 struct ky_bd { int count, slider_y; keystr key[MAX_TOUCHES + 1]; bool sli; } ; 00074 00075 class screen_touch_handler 00076 { 00077 ky_bd kybd_a, kybd_b; // alternating present - previous keyboard structures 00078 ky_bd * present_kybd, * previous_kybd; // pointers 00079 bool in_list (struct ky_bd & , int ) ; 00080 void motor_power () ; 00081 void flush () ; 00082 int viscous_drag (int, double, double) ; 00083 int position; 00084 int oldpos; 00085 int next_state; 00086 public: 00087 int direction; 00088 void DrawSlider () ; 00089 void HandleFingerInput () ; 00090 screen_touch_handler () ; // default constructor 00091 } ; 00092 00093 const int MAX_PARAMS = 30; 00094 const int MAX_CMD_LINE_LEN = 180; 00095 const int MAX_ESCS = 12; 00096 00097 struct parameters { 00098 int32_t numof_menu_items, numof_cl_values_read; 00099 double dbl[MAX_PARAMS]; 00100 } ; 00101 00102 enum { 00103 FAULT_0, 00104 FAULT_BOARD_ID_IN_MSG, 00105 FAULT_TS, 00106 FAULT_PC, 00107 FAULT_COM, 00108 FAULT_COM_NO_MATCH, 00109 FAULT_COM_LINE_LEN, 00110 FAULT_QSPI, 00111 FAULT_ODOMETER, 00112 FAULT_MAX, 00113 NUMOF_REPORTABLE_TS_ERRORS 00114 } ; 00115 00116 class error_handling_Jan_2019 00117 { 00118 int32_t TS_fault[NUMOF_REPORTABLE_TS_ERRORS] ; // Some number of reportable error codes, accessible through set and read members 00119 public: 00120 error_handling_Jan_2019 () { // default constructor 00121 for (int i = 0; i < (sizeof(TS_fault) / sizeof(int32_t)); i++) 00122 TS_fault[i] = 0; 00123 } 00124 void set (uint32_t, int32_t) ; 00125 void clr (uint32_t) ; 00126 uint32_t read (uint32_t) ; 00127 bool all_good () ; 00128 void report_any (bool) ; 00129 } ; 00130 00131 class command_line_interpreter_core { 00132 parameters a ; // as opposed to clicore(¶meters) 00133 struct kb_command const * clist; 00134 int32_t portio, cl_index, target_unit; 00135 char cmd_line[MAX_CMD_LINE_LEN]; 00136 char * cmd_line_ptr; 00137 int clreadable (); 00138 int clgetc (); 00139 void clputc (int); 00140 public: 00141 command_line_interpreter_core () {}; // default constructor 00142 command_line_interpreter_core (int, int, struct kb_command const * ) ; //{ // constructor including io port id value 00143 void sniff () ; // The function to call often to sniff out commands from command line and act upon them 00144 } ; 00145 00146 class STM3_ESC_Interface 00147 { 00148 int board_IDs [MAX_ESCS], // allow up to 4 boards in practical system, size for 10+ as 10 discrete ID nums exist 00149 board_count, 00150 reqno; 00151 public: 00152 double last_V, last_I, mph, esc_speeds[MAX_ESCS]; 00153 STM3_ESC_Interface () { // Constructor 00154 board_count = 0; 00155 reqno = 0; 00156 last_V = last_I = mph = 0.0; 00157 for (int i = 0; i < MAX_ESCS; i++) { 00158 board_IDs[i] = 0; 00159 esc_speeds[i] = 0.0; 00160 } 00161 } 00162 bool request_mph () ; // Issue "'n'mph\r" to one particular STM3_ESC board to request MPH 22/06/2018 00163 void mph_update (double) ; // touched 08/01/2019 00164 void set_board_ID (int) ; // called in response to 'whon' coming back from a STM3_ESC 00165 void search_for_escs () ; // one-off call during startup to identify all connected STM3_ESCs 00166 void get_boards_list (int *) ; // copies board list 00167 void set_V_limit (double) ; // Set max motor voltage 00168 void set_I_limit (double) ; // Set max motor current 00169 void message (char *) ; // Broadcast message to all STM3_ESCs 00170 void message (int, char *) ; // Send message to one individual STM3_ESC 00171 } ; 00172 00173 00174
Generated on Thu Jul 14 2022 06:50:34 by
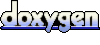