
Dual Brushless Motor ESC, 10-62V, up to 50A per motor. Motors ganged or independent, multiple control input methods, cycle-by-cycle current limit, speed mode and torque mode control. Motors tiny to kW. Speed limit and other parameters easily set in firmware. As used in 'The Brushless Brutalist' locomotive - www.jons-workshop.com. See also Model Engineer magazine June-October 2019.
Dependencies: mbed BufferedSerial Servo PCT2075 FastPWM
error_handler.cpp
00001 /* 00002 STM3_ESC Electronic Speed Controller board, drives Two Brushless Motors, full Four Quadrant Control. 00003 Jon Freeman B. Eng Hons 00004 2015 - 2019 00005 */ 00006 #include "mbed.h" 00007 #include "STM3_ESC.h" 00008 #include "BufferedSerial.h" 00009 extern BufferedSerial pc; 00010 00011 /*class error_handling_Jan_2019 00012 { 00013 int32_t TS_fault[NUMOF_REPORTABLE_TS_ERRORS] ; // Some number of reportable error codes, accessible through set and read members 00014 public: 00015 error_handling_Jan_2019 () { // default constructor 00016 for (int i = 0; i < (sizeof(TS_fault) / sizeof(int32_t)); i++) 00017 TS_fault[i] = 0; 00018 } 00019 void set (uint32_t, int32_t) ; 00020 uint32_t read (uint32_t) ; 00021 bool all_good () ; 00022 void report_any () ; 00023 } ; 00024 */ 00025 00026 const char * FaultList[] = { 00027 /* 00028 FAULT_0, 00029 FAULT_EEPROM, 00030 FAULT_BOARD_ID, 00031 FAULT_COM_LINE_LEN, 00032 FAULT_COM_LINE_NOMATCH, 00033 FAULT_COM_LINE_LEN_PC, 00034 FAULT_COM_LINE_LEN_TS, 00035 FAULT_COM_LINE_NOMATCH_PC, 00036 FAULT_COM_LINE_NOMATCH_TS, 00037 FAULT_MAX, 00038 NUMOF_REPORTABLE_TS_ERRORS 00039 */ 00040 "Zero", 00041 "EEPROM", 00042 "board ID", 00043 "com line len", 00044 "com line nomatch", 00045 "com line len", 00046 "com line len", 00047 "com no match", 00048 "com no match", 00049 "max", 00050 "endoflist", 00051 " ", 00052 } ; 00053 00054 bool error_handling_Jan_2019::all_good () { 00055 for (int i = 0; i < NUMOF_REPORTABLE_TS_ERRORS; i++) 00056 if (ESC_fault[i]) 00057 return false; 00058 return true; 00059 } 00060 00061 /**void error_handling_Jan_2019::set (uint32_t err_no, int32_t bits_to_set) { 00062 Used to set bits in error int 00063 Uses OR to set new bits without clearing other bits set previously 00064 */ 00065 void error_handling_Jan_2019::set (uint32_t err_no, int32_t bits_to_set) { 00066 if (bits_to_set) { 00067 pc.printf ("At Error.set, err_no %d, bits %lx\r\n", err_no, bits_to_set); 00068 ESC_fault[err_no] |= bits_to_set; // Uses OR to set new bits without clearing other bits set previously 00069 } 00070 } 00071 00072 /**void error_handling_Jan_2019::clr (uint32_t err_no) { 00073 Used to clear all bits in error int 00074 */ 00075 void error_handling_Jan_2019::clr (uint32_t err_no) { 00076 ESC_fault[err_no] = 0; 00077 } 00078 00079 uint32_t error_handling_Jan_2019::read (uint32_t err_no) { 00080 return ESC_fault[err_no]; 00081 } 00082 00083 void error_handling_Jan_2019::report_any (bool retain) { 00084 for (int i = 0; i < NUMOF_REPORTABLE_TS_ERRORS; i++) { 00085 if (ESC_fault[i]) { 00086 pc.printf ("Error report, number %d, value %d, %s\r\n", i, ESC_fault[i], FaultList[i]); 00087 if (!retain) 00088 ESC_fault[i] = 0; 00089 } 00090 } 00091 } 00092
Generated on Tue Jul 19 2022 12:28:36 by
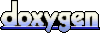