No changes made
Fork of BSP_DISCO_F746NG by
Embed:
(wiki syntax)
Show/hide line numbers
stm32746g_discovery.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32746g_discovery.c 00004 * @author MCD Application Team 00005 * @version V2.0.0 00006 * @date 30-December-2016 00007 * @brief This file provides a set of firmware functions to manage LEDs, 00008 * push-buttons and COM ports available on STM32746G-Discovery 00009 * board(MB1191) from STMicroelectronics. 00010 ****************************************************************************** 00011 * @attention 00012 * 00013 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00014 * 00015 * Redistribution and use in source and binary forms, with or without modification, 00016 * are permitted provided that the following conditions are met: 00017 * 1. Redistributions of source code must retain the above copyright notice, 00018 * this list of conditions and the following disclaimer. 00019 * 2. Redistributions in binary form must reproduce the above copyright notice, 00020 * this list of conditions and the following disclaimer in the documentation 00021 * and/or other materials provided with the distribution. 00022 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00023 * may be used to endorse or promote products derived from this software 00024 * without specific prior written permission. 00025 * 00026 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00027 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00028 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00029 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00030 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00031 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00032 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00033 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00034 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00035 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00036 * 00037 ****************************************************************************** 00038 */ 00039 00040 /* Includes ------------------------------------------------------------------*/ 00041 #include "stm32746g_discovery.h" 00042 00043 void wait_ms(int ms); // MBED to replace HAL_Delay function 00044 00045 /** @addtogroup BSP 00046 * @{ 00047 */ 00048 00049 /** @addtogroup STM32746G_DISCOVERY 00050 * @{ 00051 */ 00052 00053 /** @defgroup STM32746G_DISCOVERY_LOW_LEVEL STM32746G_DISCOVERY_LOW_LEVEL 00054 * @{ 00055 */ 00056 00057 /** @defgroup STM32746G_DISCOVERY_LOW_LEVEL_Private_TypesDefinitions STM32746G_DISCOVERY_LOW_LEVEL Private Types Definitions 00058 * @{ 00059 */ 00060 /** 00061 * @} 00062 */ 00063 00064 /** @defgroup STM32746G_DISCOVERY_LOW_LEVEL_Private_Defines STM32746G_DISCOVERY_LOW_LEVEL Private Defines 00065 * @{ 00066 */ 00067 /** 00068 * @brief STM32746G DISCOVERY BSP Driver version number V2.0.0 00069 */ 00070 #define __STM32746G_DISCO_BSP_VERSION_MAIN (0x02) /*!< [31:24] main version */ 00071 #define __STM32746G_DISCO_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00072 #define __STM32746G_DISCO_BSP_VERSION_SUB2 (0x00) /*!< [15:8] sub2 version */ 00073 #define __STM32746G_DISCO_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00074 #define __STM32746G_DISCO_BSP_VERSION ((__STM32746G_DISCO_BSP_VERSION_MAIN << 24)\ 00075 |(__STM32746G_DISCO_BSP_VERSION_SUB1 << 16)\ 00076 |(__STM32746G_DISCO_BSP_VERSION_SUB2 << 8 )\ 00077 |(__STM32746G_DISCO_BSP_VERSION_RC)) 00078 /** 00079 * @} 00080 */ 00081 00082 /** @defgroup STM32746G_DISCOVERY_LOW_LEVEL_Private_Macros STM32746G_DISCOVERY_LOW_LEVEL Private Macros 00083 * @{ 00084 */ 00085 /** 00086 * @} 00087 */ 00088 00089 /** @defgroup STM32746G_DISCOVERY_LOW_LEVEL_Private_Variables STM32746G_DISCOVERY_LOW_LEVEL Private Variables 00090 * @{ 00091 */ 00092 00093 const uint32_t GPIO_PIN[LEDn] = {LED1_PIN}; 00094 00095 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {WAKEUP_BUTTON_GPIO_PORT, 00096 TAMPER_BUTTON_GPIO_PORT, 00097 KEY_BUTTON_GPIO_PORT}; 00098 00099 const uint16_t BUTTON_PIN[BUTTONn] = {WAKEUP_BUTTON_PIN, 00100 TAMPER_BUTTON_PIN, 00101 KEY_BUTTON_PIN}; 00102 00103 const uint16_t BUTTON_IRQn[BUTTONn] = {WAKEUP_BUTTON_EXTI_IRQn, 00104 TAMPER_BUTTON_EXTI_IRQn, 00105 KEY_BUTTON_EXTI_IRQn}; 00106 00107 USART_TypeDef* COM_USART[COMn] = {DISCOVERY_COM1}; 00108 00109 GPIO_TypeDef* COM_TX_PORT[COMn] = {DISCOVERY_COM1_TX_GPIO_PORT}; 00110 00111 GPIO_TypeDef* COM_RX_PORT[COMn] = {DISCOVERY_COM1_RX_GPIO_PORT}; 00112 00113 const uint16_t COM_TX_PIN[COMn] = {DISCOVERY_COM1_TX_PIN}; 00114 00115 const uint16_t COM_RX_PIN[COMn] = {DISCOVERY_COM1_RX_PIN}; 00116 00117 const uint16_t COM_TX_AF[COMn] = {DISCOVERY_COM1_TX_AF}; 00118 00119 const uint16_t COM_RX_AF[COMn] = {DISCOVERY_COM1_RX_AF}; 00120 00121 static I2C_HandleTypeDef hI2cAudioHandler = {0}; 00122 static I2C_HandleTypeDef hI2cExtHandler = {0}; 00123 00124 /** 00125 * @} 00126 */ 00127 00128 /** @defgroup STM32746G_DISCOVERY_LOW_LEVEL_Private_FunctionPrototypes STM32746G_DISCOVERY_LOW_LEVEL Private Function Prototypes 00129 * @{ 00130 */ 00131 static void I2Cx_MspInit(I2C_HandleTypeDef *i2c_handler); 00132 static void I2Cx_Init(I2C_HandleTypeDef *i2c_handler); 00133 00134 static HAL_StatusTypeDef I2Cx_ReadMultiple(I2C_HandleTypeDef *i2c_handler, uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00135 static HAL_StatusTypeDef I2Cx_WriteMultiple(I2C_HandleTypeDef *i2c_handler, uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00136 static HAL_StatusTypeDef I2Cx_IsDeviceReady(I2C_HandleTypeDef *i2c_handler, uint16_t DevAddress, uint32_t Trials); 00137 static void I2Cx_Error(I2C_HandleTypeDef *i2c_handler, uint8_t Addr); 00138 00139 /* AUDIO IO functions */ 00140 void AUDIO_IO_Init(void); 00141 void AUDIO_IO_DeInit(void); 00142 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value); 00143 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg); 00144 void AUDIO_IO_Delay(uint32_t Delay); 00145 00146 /* TOUCHSCREEN IO functions */ 00147 void TS_IO_Init(void); 00148 void TS_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00149 uint8_t TS_IO_Read(uint8_t Addr, uint8_t Reg); 00150 void TS_IO_Delay(uint32_t Delay); 00151 00152 /* CAMERA IO functions */ 00153 void CAMERA_IO_Init(void); 00154 void CAMERA_Delay(uint32_t Delay); 00155 void CAMERA_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00156 uint8_t CAMERA_IO_Read(uint8_t Addr, uint8_t Reg); 00157 00158 /* I2C EEPROM IO function */ 00159 void EEPROM_IO_Init(void); 00160 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00161 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00162 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00163 /** 00164 * @} 00165 */ 00166 00167 /** @defgroup STM32746G_DISCOVERY_LOW_LEVEL_Exported_Functions STM32746G_DISCOVERY_LOW_LEVELSTM32746G_DISCOVERY_LOW_LEVEL Exported Functions 00168 * @{ 00169 */ 00170 00171 /** 00172 * @brief This method returns the STM32746G DISCOVERY BSP Driver revision 00173 * @retval version: 0xXYZR (8bits for each decimal, R for RC) 00174 */ 00175 uint32_t BSP_GetVersion(void) 00176 { 00177 return __STM32746G_DISCO_BSP_VERSION; 00178 } 00179 00180 /** 00181 * @brief Configures LED on GPIO. 00182 * @param Led: LED to be configured. 00183 * This parameter can be one of the following values: 00184 * @arg LED1 00185 * @retval None 00186 */ 00187 void BSP_LED_Init(Led_TypeDef Led) 00188 { 00189 GPIO_InitTypeDef gpio_init_structure; 00190 GPIO_TypeDef* gpio_led; 00191 00192 if (Led == DISCO_LED1) // MBED 00193 { 00194 gpio_led = LED1_GPIO_PORT; 00195 /* Enable the GPIO_LED clock */ 00196 LED1_GPIO_CLK_ENABLE(); 00197 00198 /* Configure the GPIO_LED pin */ 00199 gpio_init_structure.Pin = GPIO_PIN[Led]; 00200 gpio_init_structure.Mode = GPIO_MODE_OUTPUT_PP; 00201 gpio_init_structure.Pull = GPIO_PULLUP; 00202 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00203 00204 HAL_GPIO_Init(gpio_led, &gpio_init_structure); 00205 00206 /* By default, turn off LED */ 00207 HAL_GPIO_WritePin(gpio_led, GPIO_PIN[Led], GPIO_PIN_RESET); 00208 } 00209 } 00210 00211 /** 00212 * @brief DeInit LEDs. 00213 * @param Led: LED to be configured. 00214 * This parameter can be one of the following values: 00215 * @arg LED1 00216 * @note Led DeInit does not disable the GPIO clock 00217 * @retval None 00218 */ 00219 void BSP_LED_DeInit(Led_TypeDef Led) 00220 { 00221 GPIO_InitTypeDef gpio_init_structure; 00222 GPIO_TypeDef* gpio_led; 00223 00224 if (Led == DISCO_LED1) // MBED 00225 { 00226 gpio_led = LED1_GPIO_PORT; 00227 /* Turn off LED */ 00228 HAL_GPIO_WritePin(gpio_led, GPIO_PIN[Led], GPIO_PIN_RESET); 00229 /* Configure the GPIO_LED pin */ 00230 gpio_init_structure.Pin = GPIO_PIN[Led]; 00231 HAL_GPIO_DeInit(gpio_led, gpio_init_structure.Pin); 00232 } 00233 } 00234 00235 /** 00236 * @brief Turns selected LED On. 00237 * @param Led: LED to be set on 00238 * This parameter can be one of the following values: 00239 * @arg LED1 00240 * @retval None 00241 */ 00242 void BSP_LED_On(Led_TypeDef Led) 00243 { 00244 GPIO_TypeDef* gpio_led; 00245 00246 if (Led == DISCO_LED1) /* Switch On LED connected to GPIO */ // MBED 00247 { 00248 gpio_led = LED1_GPIO_PORT; 00249 HAL_GPIO_WritePin(gpio_led, GPIO_PIN[Led], GPIO_PIN_SET); 00250 } 00251 } 00252 00253 /** 00254 * @brief Turns selected LED Off. 00255 * @param Led: LED to be set off 00256 * This parameter can be one of the following values: 00257 * @arg LED1 00258 * @retval None 00259 */ 00260 void BSP_LED_Off(Led_TypeDef Led) 00261 { 00262 GPIO_TypeDef* gpio_led; 00263 00264 if (Led == DISCO_LED1) /* Switch Off LED connected to GPIO */ // MBED 00265 { 00266 gpio_led = LED1_GPIO_PORT; 00267 HAL_GPIO_WritePin(gpio_led, GPIO_PIN[Led], GPIO_PIN_RESET); 00268 } 00269 } 00270 00271 /** 00272 * @brief Toggles the selected LED. 00273 * @param Led: LED to be toggled 00274 * This parameter can be one of the following values: 00275 * @arg LED1 00276 * @retval None 00277 */ 00278 void BSP_LED_Toggle(Led_TypeDef Led) 00279 { 00280 GPIO_TypeDef* gpio_led; 00281 00282 if (Led == DISCO_LED1) /* Toggle LED connected to GPIO */ // MBED 00283 { 00284 gpio_led = LED1_GPIO_PORT; 00285 HAL_GPIO_TogglePin(gpio_led, GPIO_PIN[Led]); 00286 } 00287 } 00288 00289 /** 00290 * @brief Configures button GPIO and EXTI Line. 00291 * @param Button: Button to be configured 00292 * This parameter can be one of the following values: 00293 * @arg BUTTON_WAKEUP: Wakeup Push Button 00294 * @arg BUTTON_TAMPER: Tamper Push Button 00295 * @arg BUTTON_KEY: Key Push Button 00296 * @param ButtonMode: Button mode 00297 * This parameter can be one of the following values: 00298 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00299 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line 00300 * with interrupt generation capability 00301 * @note On STM32746G-Discovery board, the three buttons (Wakeup, Tamper and key buttons) 00302 * are mapped on the same push button named "User" 00303 * on the board serigraphy. 00304 * @retval None 00305 */ 00306 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode) 00307 { 00308 GPIO_InitTypeDef gpio_init_structure; 00309 00310 /* Enable the BUTTON clock */ 00311 BUTTONx_GPIO_CLK_ENABLE(Button); 00312 00313 if(ButtonMode == BUTTON_MODE_GPIO) 00314 { 00315 /* Configure Button pin as input */ 00316 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00317 gpio_init_structure.Mode = GPIO_MODE_INPUT; 00318 gpio_init_structure.Pull = GPIO_NOPULL; 00319 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00320 HAL_GPIO_Init(BUTTON_PORT[Button], &gpio_init_structure); 00321 } 00322 00323 if(ButtonMode == BUTTON_MODE_EXTI) 00324 { 00325 /* Configure Button pin as input with External interrupt */ 00326 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00327 gpio_init_structure.Pull = GPIO_NOPULL; 00328 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00329 00330 if(Button != BUTTON_WAKEUP) 00331 { 00332 gpio_init_structure.Mode = GPIO_MODE_IT_FALLING; 00333 } 00334 else 00335 { 00336 gpio_init_structure.Mode = GPIO_MODE_IT_RISING; 00337 } 00338 00339 HAL_GPIO_Init(BUTTON_PORT[Button], &gpio_init_structure); 00340 00341 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00342 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0x00); 00343 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00344 } 00345 } 00346 00347 /** 00348 * @brief Push Button DeInit. 00349 * @param Button: Button to be configured 00350 * This parameter can be one of the following values: 00351 * @arg BUTTON_WAKEUP: Wakeup Push Button 00352 * @arg BUTTON_TAMPER: Tamper Push Button 00353 * @arg BUTTON_KEY: Key Push Button 00354 * @note On STM32746G-Discovery board, the three buttons (Wakeup, Tamper and key buttons) 00355 * are mapped on the same push button named "User" 00356 * on the board serigraphy. 00357 * @note PB DeInit does not disable the GPIO clock 00358 * @retval None 00359 */ 00360 void BSP_PB_DeInit(Button_TypeDef Button) 00361 { 00362 GPIO_InitTypeDef gpio_init_structure; 00363 00364 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00365 HAL_NVIC_DisableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00366 HAL_GPIO_DeInit(BUTTON_PORT[Button], gpio_init_structure.Pin); 00367 } 00368 00369 00370 /** 00371 * @brief Returns the selected button state. 00372 * @param Button: Button to be checked 00373 * This parameter can be one of the following values: 00374 * @arg BUTTON_WAKEUP: Wakeup Push Button 00375 * @arg BUTTON_TAMPER: Tamper Push Button 00376 * @arg BUTTON_KEY: Key Push Button 00377 * @note On STM32746G-Discovery board, the three buttons (Wakeup, Tamper and key buttons) 00378 * are mapped on the same push button named "User" 00379 * on the board serigraphy. 00380 * @retval The Button GPIO pin value 00381 */ 00382 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00383 { 00384 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00385 } 00386 00387 /** 00388 * @brief Configures COM port. 00389 * @param COM: COM port to be configured. 00390 * This parameter can be one of the following values: 00391 * @arg COM1 00392 * @arg COM2 00393 * @param huart: Pointer to a UART_HandleTypeDef structure that contains the 00394 * configuration information for the specified USART peripheral. 00395 * @retval None 00396 */ 00397 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef *huart) 00398 { 00399 GPIO_InitTypeDef gpio_init_structure; 00400 00401 /* Enable GPIO clock */ 00402 DISCOVERY_COMx_TX_GPIO_CLK_ENABLE(COM); 00403 DISCOVERY_COMx_RX_GPIO_CLK_ENABLE(COM); 00404 00405 /* Enable USART clock */ 00406 DISCOVERY_COMx_CLK_ENABLE(COM); 00407 00408 /* Configure USART Tx as alternate function */ 00409 gpio_init_structure.Pin = COM_TX_PIN[COM]; 00410 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00411 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00412 gpio_init_structure.Pull = GPIO_PULLUP; 00413 gpio_init_structure.Alternate = COM_TX_AF[COM]; 00414 HAL_GPIO_Init(COM_TX_PORT[COM], &gpio_init_structure); 00415 00416 /* Configure USART Rx as alternate function */ 00417 gpio_init_structure.Pin = COM_RX_PIN[COM]; 00418 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00419 gpio_init_structure.Alternate = COM_RX_AF[COM]; 00420 HAL_GPIO_Init(COM_RX_PORT[COM], &gpio_init_structure); 00421 00422 /* USART configuration */ 00423 huart->Instance = COM_USART[COM]; 00424 HAL_UART_Init(huart); 00425 } 00426 00427 /** 00428 * @brief DeInit COM port. 00429 * @param COM: COM port to be configured. 00430 * This parameter can be one of the following values: 00431 * @arg COM1 00432 * @arg COM2 00433 * @param huart: Pointer to a UART_HandleTypeDef structure that contains the 00434 * configuration information for the specified USART peripheral. 00435 * @retval None 00436 */ 00437 void BSP_COM_DeInit(COM_TypeDef COM, UART_HandleTypeDef *huart) 00438 { 00439 /* USART configuration */ 00440 huart->Instance = COM_USART[COM]; 00441 HAL_UART_DeInit(huart); 00442 00443 /* Enable USART clock */ 00444 DISCOVERY_COMx_CLK_DISABLE(COM); 00445 00446 /* DeInit GPIO pins can be done in the application 00447 (by surcharging this __weak function) */ 00448 00449 /* GPIO pins clock, DMA clock can be shut down in the application 00450 by surcharging this __weak function */ 00451 } 00452 00453 /******************************************************************************* 00454 BUS OPERATIONS 00455 *******************************************************************************/ 00456 00457 /******************************* I2C Routines *********************************/ 00458 /** 00459 * @brief Initializes I2C MSP. 00460 * @param i2c_handler : I2C handler 00461 * @retval None 00462 */ 00463 static void I2Cx_MspInit(I2C_HandleTypeDef *i2c_handler) 00464 { 00465 GPIO_InitTypeDef gpio_init_structure; 00466 00467 if (i2c_handler == (I2C_HandleTypeDef*)(&hI2cAudioHandler)) 00468 { 00469 /* AUDIO and LCD I2C MSP init */ 00470 00471 /*** Configure the GPIOs ***/ 00472 /* Enable GPIO clock */ 00473 DISCOVERY_AUDIO_I2Cx_SCL_SDA_GPIO_CLK_ENABLE(); 00474 00475 /* Configure I2C Tx as alternate function */ 00476 gpio_init_structure.Pin = DISCOVERY_AUDIO_I2Cx_SCL_PIN; 00477 gpio_init_structure.Mode = GPIO_MODE_AF_OD; 00478 gpio_init_structure.Pull = GPIO_NOPULL; 00479 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00480 gpio_init_structure.Alternate = DISCOVERY_AUDIO_I2Cx_SCL_SDA_AF; 00481 HAL_GPIO_Init(DISCOVERY_AUDIO_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00482 00483 /* Configure I2C Rx as alternate function */ 00484 gpio_init_structure.Pin = DISCOVERY_AUDIO_I2Cx_SDA_PIN; 00485 HAL_GPIO_Init(DISCOVERY_AUDIO_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00486 00487 /*** Configure the I2C peripheral ***/ 00488 /* Enable I2C clock */ 00489 DISCOVERY_AUDIO_I2Cx_CLK_ENABLE(); 00490 00491 /* Force the I2C peripheral clock reset */ 00492 DISCOVERY_AUDIO_I2Cx_FORCE_RESET(); 00493 00494 /* Release the I2C peripheral clock reset */ 00495 DISCOVERY_AUDIO_I2Cx_RELEASE_RESET(); 00496 00497 /* Enable and set I2Cx Interrupt to a lower priority */ 00498 HAL_NVIC_SetPriority(DISCOVERY_AUDIO_I2Cx_EV_IRQn, 0x0F, 0); 00499 HAL_NVIC_EnableIRQ(DISCOVERY_AUDIO_I2Cx_EV_IRQn); 00500 00501 /* Enable and set I2Cx Interrupt to a lower priority */ 00502 HAL_NVIC_SetPriority(DISCOVERY_AUDIO_I2Cx_ER_IRQn, 0x0F, 0); 00503 HAL_NVIC_EnableIRQ(DISCOVERY_AUDIO_I2Cx_ER_IRQn); 00504 } 00505 else 00506 { 00507 /* External, camera and Arduino connector I2C MSP init */ 00508 00509 /*** Configure the GPIOs ***/ 00510 /* Enable GPIO clock */ 00511 DISCOVERY_EXT_I2Cx_SCL_SDA_GPIO_CLK_ENABLE(); 00512 00513 /* Configure I2C Tx as alternate function */ 00514 gpio_init_structure.Pin = DISCOVERY_EXT_I2Cx_SCL_PIN; 00515 gpio_init_structure.Mode = GPIO_MODE_AF_OD; 00516 gpio_init_structure.Pull = GPIO_NOPULL; 00517 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00518 gpio_init_structure.Alternate = DISCOVERY_EXT_I2Cx_SCL_SDA_AF; 00519 HAL_GPIO_Init(DISCOVERY_EXT_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00520 00521 /* Configure I2C Rx as alternate function */ 00522 gpio_init_structure.Pin = DISCOVERY_EXT_I2Cx_SDA_PIN; 00523 HAL_GPIO_Init(DISCOVERY_EXT_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00524 00525 /*** Configure the I2C peripheral ***/ 00526 /* Enable I2C clock */ 00527 DISCOVERY_EXT_I2Cx_CLK_ENABLE(); 00528 00529 /* Force the I2C peripheral clock reset */ 00530 DISCOVERY_EXT_I2Cx_FORCE_RESET(); 00531 00532 /* Release the I2C peripheral clock reset */ 00533 DISCOVERY_EXT_I2Cx_RELEASE_RESET(); 00534 00535 /* Enable and set I2Cx Interrupt to a lower priority */ 00536 HAL_NVIC_SetPriority(DISCOVERY_EXT_I2Cx_EV_IRQn, 0x0F, 0); 00537 HAL_NVIC_EnableIRQ(DISCOVERY_EXT_I2Cx_EV_IRQn); 00538 00539 /* Enable and set I2Cx Interrupt to a lower priority */ 00540 HAL_NVIC_SetPriority(DISCOVERY_EXT_I2Cx_ER_IRQn, 0x0F, 0); 00541 HAL_NVIC_EnableIRQ(DISCOVERY_EXT_I2Cx_ER_IRQn); 00542 } 00543 } 00544 00545 /** 00546 * @brief Initializes I2C HAL. 00547 * @param i2c_handler : I2C handler 00548 * @retval None 00549 */ 00550 static void I2Cx_Init(I2C_HandleTypeDef *i2c_handler) 00551 { 00552 if(HAL_I2C_GetState(i2c_handler) == HAL_I2C_STATE_RESET) 00553 { 00554 if (i2c_handler == (I2C_HandleTypeDef*)(&hI2cAudioHandler)) 00555 { 00556 /* Audio and LCD I2C configuration */ 00557 i2c_handler->Instance = DISCOVERY_AUDIO_I2Cx; 00558 } 00559 else 00560 { 00561 /* External, camera and Arduino connector I2C configuration */ 00562 i2c_handler->Instance = DISCOVERY_EXT_I2Cx; 00563 } 00564 i2c_handler->Init.Timing = DISCOVERY_I2Cx_TIMING; 00565 i2c_handler->Init.OwnAddress1 = 0; 00566 i2c_handler->Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00567 i2c_handler->Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00568 i2c_handler->Init.OwnAddress2 = 0; 00569 i2c_handler->Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00570 i2c_handler->Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00571 00572 /* Init the I2C */ 00573 I2Cx_MspInit(i2c_handler); 00574 HAL_I2C_Init(i2c_handler); 00575 } 00576 } 00577 00578 /** 00579 * @brief Reads multiple data. 00580 * @param i2c_handler : I2C handler 00581 * @param Addr: I2C address 00582 * @param Reg: Reg address 00583 * @param MemAddress: Memory address 00584 * @param Buffer: Pointer to data buffer 00585 * @param Length: Length of the data 00586 * @retval Number of read data 00587 */ 00588 static HAL_StatusTypeDef I2Cx_ReadMultiple(I2C_HandleTypeDef *i2c_handler, 00589 uint8_t Addr, 00590 uint16_t Reg, 00591 uint16_t MemAddress, 00592 uint8_t *Buffer, 00593 uint16_t Length) 00594 { 00595 HAL_StatusTypeDef status = HAL_OK; 00596 00597 status = HAL_I2C_Mem_Read(i2c_handler, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, 1000); 00598 00599 /* Check the communication status */ 00600 if(status != HAL_OK) 00601 { 00602 /* I2C error occurred */ 00603 I2Cx_Error(i2c_handler, Addr); 00604 } 00605 return status; 00606 } 00607 00608 /** 00609 * @brief Writes a value in a register of the device through BUS in using DMA mode. 00610 * @param i2c_handler : I2C handler 00611 * @param Addr: Device address on BUS Bus. 00612 * @param Reg: The target register address to write 00613 * @param MemAddress: Memory address 00614 * @param Buffer: The target register value to be written 00615 * @param Length: buffer size to be written 00616 * @retval HAL status 00617 */ 00618 static HAL_StatusTypeDef I2Cx_WriteMultiple(I2C_HandleTypeDef *i2c_handler, 00619 uint8_t Addr, 00620 uint16_t Reg, 00621 uint16_t MemAddress, 00622 uint8_t *Buffer, 00623 uint16_t Length) 00624 { 00625 HAL_StatusTypeDef status = HAL_OK; 00626 00627 status = HAL_I2C_Mem_Write(i2c_handler, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, 1000); 00628 00629 /* Check the communication status */ 00630 if(status != HAL_OK) 00631 { 00632 /* Re-Initiaize the I2C Bus */ 00633 I2Cx_Error(i2c_handler, Addr); 00634 } 00635 return status; 00636 } 00637 00638 /** 00639 * @brief Checks if target device is ready for communication. 00640 * @note This function is used with Memory devices 00641 * @param i2c_handler : I2C handler 00642 * @param DevAddress: Target device address 00643 * @param Trials: Number of trials 00644 * @retval HAL status 00645 */ 00646 static HAL_StatusTypeDef I2Cx_IsDeviceReady(I2C_HandleTypeDef *i2c_handler, uint16_t DevAddress, uint32_t Trials) 00647 { 00648 return (HAL_I2C_IsDeviceReady(i2c_handler, DevAddress, Trials, 1000)); 00649 } 00650 00651 /** 00652 * @brief Manages error callback by re-initializing I2C. 00653 * @param i2c_handler : I2C handler 00654 * @param Addr: I2C Address 00655 * @retval None 00656 */ 00657 static void I2Cx_Error(I2C_HandleTypeDef *i2c_handler, uint8_t Addr) 00658 { 00659 /* De-initialize the I2C communication bus */ 00660 HAL_I2C_DeInit(i2c_handler); 00661 00662 /* Re-Initialize the I2C communication bus */ 00663 I2Cx_Init(i2c_handler); 00664 } 00665 00666 /******************************************************************************* 00667 LINK OPERATIONS 00668 *******************************************************************************/ 00669 00670 /********************************* LINK AUDIO *********************************/ 00671 00672 /** 00673 * @brief Initializes Audio low level. 00674 * @retval None 00675 */ 00676 void AUDIO_IO_Init(void) 00677 { 00678 I2Cx_Init(&hI2cAudioHandler); 00679 } 00680 00681 /** 00682 * @brief Deinitializes Audio low level. 00683 * @retval None 00684 */ 00685 void AUDIO_IO_DeInit(void) 00686 { 00687 } 00688 00689 /** 00690 * @brief Writes a single data. 00691 * @param Addr: I2C address 00692 * @param Reg: Reg address 00693 * @param Value: Data to be written 00694 * @retval None 00695 */ 00696 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value) 00697 { 00698 uint16_t tmp = Value; 00699 00700 Value = ((uint16_t)(tmp >> 8) & 0x00FF); 00701 00702 Value |= ((uint16_t)(tmp << 8)& 0xFF00); 00703 00704 I2Cx_WriteMultiple(&hI2cAudioHandler, Addr, Reg, I2C_MEMADD_SIZE_16BIT,(uint8_t*)&Value, 2); 00705 } 00706 00707 /** 00708 * @brief Reads a single data. 00709 * @param Addr: I2C address 00710 * @param Reg: Reg address 00711 * @retval Data to be read 00712 */ 00713 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg) 00714 { 00715 uint16_t read_value = 0, tmp = 0; 00716 00717 I2Cx_ReadMultiple(&hI2cAudioHandler, Addr, Reg, I2C_MEMADD_SIZE_16BIT, (uint8_t*)&read_value, 2); 00718 00719 tmp = ((uint16_t)(read_value >> 8) & 0x00FF); 00720 00721 tmp |= ((uint16_t)(read_value << 8)& 0xFF00); 00722 00723 read_value = tmp; 00724 00725 return read_value; 00726 } 00727 00728 /** 00729 * @brief AUDIO Codec delay 00730 * @param Delay: Delay in ms 00731 * @retval None 00732 */ 00733 void AUDIO_IO_Delay(uint32_t Delay) 00734 { 00735 //HAL_Delay(Delay); // MBED 00736 wait_ms(Delay); // MBED 00737 } 00738 00739 /********************************* LINK CAMERA ********************************/ 00740 00741 /** 00742 * @brief Initializes Camera low level. 00743 * @retval None 00744 */ 00745 void CAMERA_IO_Init(void) 00746 { 00747 I2Cx_Init(&hI2cExtHandler); 00748 } 00749 00750 /** 00751 * @brief Camera writes single data. 00752 * @param Addr: I2C address 00753 * @param Reg: Register address 00754 * @param Value: Data to be written 00755 * @retval None 00756 */ 00757 void CAMERA_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00758 { 00759 I2Cx_WriteMultiple(&hI2cExtHandler, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT,(uint8_t*)&Value, 1); 00760 } 00761 00762 /** 00763 * @brief Camera reads single data. 00764 * @param Addr: I2C address 00765 * @param Reg: Register address 00766 * @retval Read data 00767 */ 00768 uint8_t CAMERA_IO_Read(uint8_t Addr, uint8_t Reg) 00769 { 00770 uint8_t read_value = 0; 00771 00772 I2Cx_ReadMultiple(&hI2cExtHandler, Addr, Reg, I2C_MEMADD_SIZE_8BIT, (uint8_t*)&read_value, 1); 00773 00774 return read_value; 00775 } 00776 00777 /** 00778 * @brief Camera delay 00779 * @param Delay: Delay in ms 00780 * @retval None 00781 */ 00782 void CAMERA_Delay(uint32_t Delay) 00783 { 00784 //HAL_Delay(Delay); // MBED 00785 wait_ms(Delay); // MBED 00786 } 00787 00788 /******************************** LINK I2C EEPROM *****************************/ 00789 00790 /** 00791 * @brief Initializes peripherals used by the I2C EEPROM driver. 00792 * @retval None 00793 */ 00794 void EEPROM_IO_Init(void) 00795 { 00796 I2Cx_Init(&hI2cExtHandler); 00797 } 00798 00799 /** 00800 * @brief Write data to I2C EEPROM driver in using DMA channel. 00801 * @param DevAddress: Target device address 00802 * @param MemAddress: Internal memory address 00803 * @param pBuffer: Pointer to data buffer 00804 * @param BufferSize: Amount of data to be sent 00805 * @retval HAL status 00806 */ 00807 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 00808 { 00809 return (I2Cx_WriteMultiple(&hI2cExtHandler, DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 00810 } 00811 00812 /** 00813 * @brief Read data from I2C EEPROM driver in using DMA channel. 00814 * @param DevAddress: Target device address 00815 * @param MemAddress: Internal memory address 00816 * @param pBuffer: Pointer to data buffer 00817 * @param BufferSize: Amount of data to be read 00818 * @retval HAL status 00819 */ 00820 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 00821 { 00822 return (I2Cx_ReadMultiple(&hI2cExtHandler, DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 00823 } 00824 00825 /** 00826 * @brief Checks if target device is ready for communication. 00827 * @note This function is used with Memory devices 00828 * @param DevAddress: Target device address 00829 * @param Trials: Number of trials 00830 * @retval HAL status 00831 */ 00832 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 00833 { 00834 return (I2Cx_IsDeviceReady(&hI2cExtHandler, DevAddress, Trials)); 00835 } 00836 00837 /********************************* LINK TOUCHSCREEN *********************************/ 00838 00839 /** 00840 * @brief Initializes Touchscreen low level. 00841 * @retval None 00842 */ 00843 void TS_IO_Init(void) 00844 { 00845 I2Cx_Init(&hI2cAudioHandler); 00846 } 00847 00848 /** 00849 * @brief Writes a single data. 00850 * @param Addr: I2C address 00851 * @param Reg: Reg address 00852 * @param Value: Data to be written 00853 * @retval None 00854 */ 00855 void TS_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00856 { 00857 I2Cx_WriteMultiple(&hI2cAudioHandler, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT,(uint8_t*)&Value, 1); 00858 } 00859 00860 /** 00861 * @brief Reads a single data. 00862 * @param Addr: I2C address 00863 * @param Reg: Reg address 00864 * @retval Data to be read 00865 */ 00866 uint8_t TS_IO_Read(uint8_t Addr, uint8_t Reg) 00867 { 00868 uint8_t read_value = 0; 00869 00870 I2Cx_ReadMultiple(&hI2cAudioHandler, Addr, Reg, I2C_MEMADD_SIZE_8BIT, (uint8_t*)&read_value, 1); 00871 00872 return read_value; 00873 } 00874 00875 /** 00876 * @brief TS delay 00877 * @param Delay: Delay in ms 00878 * @retval None 00879 */ 00880 void TS_IO_Delay(uint32_t Delay) 00881 { 00882 //HAL_Delay(Delay); // MBED 00883 wait_ms(Delay); // MBED 00884 } 00885 00886 /** 00887 * @} 00888 */ 00889 00890 /** 00891 * @} 00892 */ 00893 00894 /** 00895 * @} 00896 */ 00897 00898 /** 00899 * @} 00900 */ 00901 00902 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 19:15:26 by
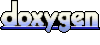