reverted HTTPCLient debug back to defaulted off
Fork of MTS-Socket by
TestTCPSocketConnection.h
00001 #ifndef TESTTCPSOCKETCONNECTION_H 00002 #define TESTTCPSOCKETCONNECTION_H 00003 00004 #include "mtsas.h" 00005 #include <string> 00006 00007 using namespace mts; 00008 00009 class TestTCPSocketConnection : public TestCollection 00010 { 00011 public: 00012 TestTCPSocketConnection(); 00013 virtual void run(); 00014 00015 private: 00016 bool runIteration(); 00017 MTSSerialFlowControl* io; 00018 Cellular* radio; 00019 TCPSocketConnection* sock; 00020 }; 00021 00022 const char PATTERN_LINE1[] = "abcdefghijklmnopqrstuvwzyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890!@#$%^&*()[]{}|"; 00023 const char PATTERN[] = "abcdefghijklmnopqrstuvwzyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890!@#$%^&*()[]{}|\r\n" 00024 "abcdefghijklmnopqrstuvwzyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890!@#$%^&*()[]{}/\r\n" 00025 "abcdefghijklmnopqrstuvwzyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890!@#$%^&*()[]{}-\r\n" 00026 "abcdefghijklmnopqrstuvwzyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890!@#$%^&*()[]{}\\\r\n" 00027 "abcdefghijklmnopqrstuvwzyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890!@#$%^&*()[]{}|\r\n" 00028 "abcdefghijklmnopqrstuvwzyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890!@#$%^&*()[]{}/\r\n" 00029 "abcdefghijklmnopqrstuvwzyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890!@#$%^&*()[]{}-\r\n" 00030 "abcdefghijklmnopqrstuvwzyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890!@#$%^&*()[]{}\\\r\n" 00031 "abcdefghijklmnopqrstuvwzyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890!@#$%^&*()[]{}*\r\n"; 00032 00033 const char MENU_LINE1[] = "send ascii pattern until keypress"; 00034 const char MENU[] = "1 send ascii pattern until keypress" 00035 "2 send ascii pattern (numbered)" 00036 "3 send pattern and close socket" 00037 "4 send [ETX] and wait for keypress" 00038 "5 send [DLE] and wait for keypress" 00039 "6 send all hex values (00-FF)" 00040 "q quit" 00041 ">:"; 00042 00043 const char TCP_TEST_SERVER[] = "204.26.122.5"; 00044 const int TCP_TEST_PORT = 7000; 00045 00046 TestTCPSocketConnection::TestTCPSocketConnection() : TestCollection("TestTCPSocketConnection") {} 00047 00048 void TestTCPSocketConnection::run() 00049 { 00050 00051 MTSLog::setLogLevel(MTSLog::TRACE_LEVEL); 00052 Test::start("Setup"); 00053 io = new MTSSerialFlowControl(D8, D2, D3, D6); 00054 io->baud(115200); 00055 radio = CellularFactory::create(io); 00056 if (! radio) { 00057 Test::assertTrue(false); 00058 } 00059 radio->configureSignals(D4, D7, RESET); 00060 Transport::setTransport(radio); 00061 00062 for (int i = 0; i < 10; i++) { 00063 if (i >= 10) { 00064 Test::assertTrue(false); 00065 } 00066 if (radio->setApn(APN) == MTS_SUCCESS) { 00067 break; 00068 } else { 00069 wait(1); 00070 } 00071 } 00072 for (int i = 0; i < 3; i++) { 00073 if (i >= 3) { 00074 Test::assertTrue(false); 00075 } 00076 if (radio->connect()) { 00077 break; 00078 } else { 00079 wait(1); 00080 } 00081 } 00082 00083 for (int i = 0; i < 5; i++) { 00084 if (i >= 5) { 00085 Test::assertTrue(false); 00086 } 00087 if (radio->ping()) { 00088 break; 00089 } else { 00090 wait(1); 00091 } 00092 } 00093 00094 sock = new TCPSocketConnection(); 00095 sock->set_blocking(false, 2); 00096 Test::end(); 00097 00098 for (int i = 0; i < 10; i++) { 00099 Test::start("Test TCP"); 00100 Test::assertTrue(runIteration()); 00101 Test::end(); 00102 } 00103 00104 radio->disconnect(); 00105 } 00106 00107 bool TestTCPSocketConnection::runIteration() 00108 { 00109 Timer tmr; 00110 int bytesRead = 0; 00111 const int readSize = 1024; 00112 char buffer[readSize] = {0}; 00113 string result; 00114 00115 for (int i = 0; i < 5; i++) { 00116 if (i >= 5) { 00117 return false; 00118 } 00119 if (! sock->connect(TCP_TEST_SERVER, TCP_TEST_PORT)) { 00120 break; 00121 } else { 00122 wait(1); 00123 } 00124 } 00125 00126 logInfo("Receiving Menu"); 00127 tmr.reset(); 00128 tmr.start(); 00129 do { 00130 bytesRead = sock->receive(buffer, readSize); 00131 if (bytesRead > 0) { 00132 result.append(buffer, bytesRead); 00133 } 00134 logInfo("Total Bytes Read: %d", result.size()); 00135 if(result.find(MENU) != std::string::npos) { 00136 break; 00137 } 00138 } while(tmr.read() <= 40); 00139 00140 logInfo("Received: [%d] [%s]", result.size(), result.c_str()); 00141 00142 size_t pos = result.find(MENU_LINE1); 00143 if(pos != string::npos) { 00144 logInfo("Found Menu 1st Line"); 00145 } else { 00146 logError("Failed To Find Menu 1st Line"); 00147 sock->close(); 00148 return false; 00149 } 00150 00151 result.clear(); 00152 00153 logInfo("Writing To Socket: 2"); 00154 if(sock->send("2\r\n", 3) == 3) { 00155 logInfo("Successfully Wrote '2'"); 00156 } else { 00157 logError("Failed To Write '2'"); 00158 sock->close(); 00159 return false; 00160 } 00161 logInfo("Expecting 'how many ? >:'"); 00162 tmr.reset(); 00163 tmr.start(); 00164 do { 00165 bytesRead = sock->receive(buffer, readSize); 00166 if (bytesRead > 0) { 00167 result.append(buffer, bytesRead); 00168 } 00169 logInfo("Total Bytes Read: %d", result.size()); 00170 if(result.find("how many") != std::string::npos) { 00171 break; 00172 } 00173 } while(tmr.read() <= 40); 00174 00175 logInfo("Received: [%d] [%s]", result.size(), result.c_str()); 00176 00177 if(result.find("how many") != std::string::npos) { 00178 logInfo("Successfully Found 'how many'"); 00179 logInfo("Writing To Socket: 2"); 00180 if(sock->send("2\r\n", 3) == 3) { 00181 logInfo("Successfully wrote '2'"); 00182 } else { 00183 logError("Failed to write '2'"); 00184 sock->close(); 00185 return false; 00186 } 00187 } else { 00188 logError("didn't receive 'how many'"); 00189 sock->close(); 00190 return false; 00191 } 00192 00193 result.clear(); 00194 00195 logInfo("Receiving Data"); 00196 tmr.reset(); 00197 tmr.start(); 00198 do { 00199 bytesRead = sock->receive(buffer, readSize); 00200 if (bytesRead > 0) { 00201 result.append(buffer, bytesRead); 00202 } 00203 logInfo("Total Bytes Read: %d", result.size()); 00204 if(result.size() >= 1645) { 00205 break; 00206 } 00207 } while(tmr.read() <= 40); 00208 00209 logInfo("Received Data: [%d] [%s]", result.size(), result.c_str()); 00210 00211 pos = result.find(PATTERN_LINE1); 00212 if(pos != string::npos) { 00213 int patternSize = sizeof(PATTERN) - 1; 00214 const char* ptr = &result.data()[pos]; 00215 bool match = true; 00216 for(int i = 0; i < patternSize; i++) { 00217 if(PATTERN[i] != ptr[i]) { 00218 logError("1st Pattern Doesn't Match At [%d]", i); 00219 logError("Pattern [%02X] Buffer [%02X]", PATTERN[i], ptr[i]); 00220 match = false; 00221 break; 00222 } 00223 } 00224 if(match) { 00225 logInfo("Found 1st Pattern"); 00226 } else { 00227 logError("Failed To Find 1st Pattern"); 00228 sock->close(); 00229 return false; 00230 } 00231 00232 pos = result.find(PATTERN_LINE1, pos + patternSize); 00233 if(pos != std::string::npos) { 00234 ptr = &result.data()[pos]; 00235 match = true; 00236 for(int i = 0; i < patternSize; i++) { 00237 if(PATTERN[i] != ptr[i]) { 00238 logError("2nd Pattern Doesn't Match At [%d]", i); 00239 logError("Pattern [%02X] Buffer [%02X]", PATTERN[i], ptr[i]); 00240 match = false; 00241 break; 00242 } 00243 } 00244 if(match) { 00245 logInfo("Found 2nd Pattern"); 00246 } else { 00247 logError("Failed To Find 2nd Pattern"); 00248 sock->close(); 00249 return false; 00250 } 00251 } 00252 } else { 00253 logError("Failed To Find Pattern 1st Line"); 00254 sock->close(); 00255 return false; 00256 } 00257 00258 result.clear(); 00259 sock->close(); 00260 radio->disconnect(); 00261 return true; 00262 } 00263 00264 #endif
Generated on Wed Jul 13 2022 10:29:47 by
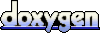