reverted HTTPCLient debug back to defaulted off
Fork of MTS-Socket by
TCPSocketConnection.cpp
00001 #include "mbed.h" 00002 #include "TCPSocketConnection.h" 00003 #include <algorithm> 00004 00005 #include "MTSLog.h" 00006 00007 using namespace mts; 00008 00009 TCPSocketConnection::TCPSocketConnection() 00010 { 00011 } 00012 00013 int TCPSocketConnection::connect(const char* host, const int port) 00014 { 00015 if(!ip) { 00016 logError("IPstack object pointer is NULL"); 00017 return -1; 00018 } 00019 if (!ip->open(host, port, IPStack::TCP)) { 00020 return -1; 00021 } 00022 return 0; 00023 } 00024 00025 bool TCPSocketConnection::is_connected(void) 00026 { 00027 return ip->isOpen(); 00028 } 00029 00030 int TCPSocketConnection::send(char* data, int length) 00031 { 00032 Timer tmr; 00033 00034 if (!_blocking) { 00035 tmr.start(); 00036 while (tmr.read_ms() < _timeout) { 00037 if (ip->writeable()) 00038 break; 00039 } 00040 if (tmr.read_ms() >= _timeout) { 00041 return -1; 00042 } 00043 } 00044 return ip->write(data, length, 0); 00045 } 00046 00047 // -1 if unsuccessful, else number of bytes written 00048 int TCPSocketConnection::send_all(char* data, int length) 00049 { 00050 if (_blocking) { 00051 return ip->write(data, length, -1); 00052 } else { 00053 return ip->write(data, length, _timeout); 00054 } 00055 } 00056 00057 // -1 if unsuccessful, else number of bytes received 00058 int TCPSocketConnection::receive(char* data, int length) 00059 { 00060 Timer tmr; 00061 int bytes = 0; 00062 int totalbytes = 0; 00063 00064 if (_blocking) { 00065 return ip->read(data, length, _timeout); 00066 } else { 00067 tmr.start(); 00068 do { 00069 bytes = ip->read(data + totalbytes, length - totalbytes, 250); 00070 if (bytes < 0) { 00071 return -1; 00072 } 00073 totalbytes += bytes; 00074 } while ( tmr.read() < _timeout && totalbytes < length); 00075 return totalbytes; 00076 } 00077 } 00078 00079 // -1 if unsuccessful, else number of bytes received 00080 int TCPSocketConnection::receive_all(char* data, int length) 00081 { 00082 if (_blocking) { 00083 return ip->read(data, length, -1); 00084 } else { 00085 return ip->read(data, length, _timeout); 00086 } 00087 }
Generated on Wed Jul 13 2022 10:29:47 by
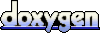