Fork of https://os.mbed.com/teams/mbed_example/code/WebSocketClient/ Update to MbedOS6
Embed:
(wiki syntax)
Show/hide line numbers
Websocket.h
00001 /** 00002 * @author Samuel Mokrani 00003 * 00004 * @section LICENSE 00005 * 00006 * Copyright (c) 2011 mbed 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy 00009 * of this software and associated documentation files (the "Software"), to deal 00010 * in the Software without restriction, including without limitation the rights 00011 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00012 * copies of the Software, and to permit persons to whom the Software is 00013 * furnished to do so, subject to the following conditions: 00014 * 00015 * The above copyright notice and this permission notice shall be included in 00016 * all copies or substantial portions of the Software. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00019 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00020 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00021 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00022 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00023 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00024 * THE SOFTWARE. 00025 * 00026 * @section DESCRIPTION 00027 * Simple websocket client 00028 * 00029 */ 00030 00031 #ifndef WEBSOCKET_H 00032 #define WEBSOCKET_H 00033 00034 #include "mbed.h" 00035 00036 /** Websocket client Class. 00037 * 00038 * Example (ethernet network): 00039 * @code 00040 * #include "mbed.h" 00041 * #include "EthernetInterface.h" 00042 * #include "Websocket.h" 00043 * 00044 * int main() { 00045 * EthernetInterface eth; 00046 * eth.connect(); 00047 * SocketAddress ip; 00048 * eth.get_ip_address(&ip); 00049 * const char *p_ip = ip.get_ip_address(); 00050 * printf("IP address: %s\n", p_ip ? p_ip : "None"); 00051 * 00052 * Websocket ws("ws://echo.websocket.org/"); 00053 * ws.connect(); 00054 * char recv[20]; 00055 * while (1) { 00056 * int res = ws.send((char*)"WebSocket Hello World!"); 00057 * printf("Error: %d\n", res); 00058 * if (ws.read(recv)) { 00059 * printf("rcv: %s\r\n", recv); 00060 * } 00061 * 00062 * thread_sleep_for(100ms); 00063 * } 00064 * } 00065 * @endcode 00066 */ 00067 00068 class Websocket 00069 { 00070 public: 00071 /** 00072 * Constructor 00073 * 00074 * @param url The Websocket url in the form "ws://ip_domain[:port]/path" (by default: port = 80) 00075 * @param iface The NetworkInterface 00076 * @param ca TLS certificate (root_ca_cert) 00077 */ 00078 Websocket(char * url, NetworkInterface * iface, const char* ca = nullptr); 00079 00080 /** 00081 * Connect to the websocket url 00082 * 00083 *@return true if the connection is established, false otherwise 00084 */ 00085 bool connect(); 00086 00087 /** 00088 * Send a string according to the websocket format (see rfc 6455) 00089 * 00090 * @param str string to be sent 00091 * 00092 * @returns the number of bytes sent 00093 */ 00094 int send(char * str); 00095 00096 /** 00097 * Read a websocket message 00098 * 00099 * @param message pointer to the string to be read (null if drop frame) 00100 * 00101 * @return true if a websocket frame has been read 00102 */ 00103 bool read(char * message); 00104 00105 /** 00106 * Close the websocket connection 00107 * 00108 * @return true if the connection has been closed, false otherwise 00109 */ 00110 bool close(); 00111 00112 /* 00113 * Accessor: get path from the websocket url 00114 * 00115 * @return path 00116 */ 00117 char* getPath(); 00118 00119 private: 00120 void fillFields(char * url); 00121 int parseURL(const char* url, char* scheme, size_t maxSchemeLen, char* host, size_t maxHostLen, uint16_t* port, char* path, size_t maxPathLen); //Parse URL 00122 int sendOpcode(uint8_t opcode, char * msg); 00123 int sendLength(uint32_t len, char * msg); 00124 int sendMask(char * msg); 00125 int readChar(char * pC, bool block = true); 00126 00127 char scheme[8]; 00128 uint16_t port; 00129 char host[32]; 00130 char path[64]; 00131 bool secured; 00132 00133 Socket* _socket; 00134 00135 int read(char * buf, int len, int min_len = -1); 00136 int write(char * buf, int len); 00137 }; 00138 00139 #endif
Generated on Tue Jul 19 2022 14:17:08 by
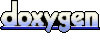