
A stripped down simple seven segement example for RedBED
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /************************************************************************** 00002 * * 00003 * A stripped down simple seven segement example for RedBED * 00004 * * 00005 * The LED block actually has 8 illuminable sections * 00006 * segments 'a' to 'g' for the main display and 'p' for a decimal point * 00007 * _________ * 00008 * <____a____> * 00009 * / \ / \ * 00010 * | | | | * 00011 * |f| |b| * 00012 * | | | | * 00013 * \ /_________ \ / * 00014 * <____g____> * 00015 * / \ / \ * 00016 * | | | | * 00017 * |e| |c| * 00018 * | | | | * 00019 * \ /_________ \ / * 00020 * <____d____> |p| * 00021 * * 00022 **************************************************************************/ 00023 00024 #include "mbed.h" 00025 00026 uint8_t left = 0; 00027 uint8_t right = 1; 00028 00029 //The 8 segment outputs and com to switch between the LEDs 00030 DigitalOut seg_a(P1_23), seg_b(P1_28), seg_c(P0_16), seg_d(P1_31), seg_e(P1_13), seg_f(P1_16), seg_g(P1_19), seg_p(P0_23), com(P1_25); 00031 00032 /****************************************************** 00033 * Set each of the segments for a desired LED * 00034 ******************************************************/ 00035 void SetSegments(uint8_t led, uint8_t a, uint8_t b, uint8_t c, uint8_t d, uint8_t e, uint8_t f, uint8_t g, uint8_t p) 00036 { 00037 //Set desired LED to update 00038 com = led; 00039 00040 //Update the segments to the desired values. 00041 //the loop ensures the segments are on for an amount 00042 //of time before being switched off again. 00043 for(uint8_t i = 0; i < 100; i++) 00044 { 00045 seg_a = 1 - a; //setting a segment to 1 means off 00046 seg_b = 1 - b; //so invert all requested segment values 00047 seg_c = 1 - c; 00048 seg_d = 1 - d; 00049 seg_e = 1 - e; 00050 seg_f = 1 - f; 00051 seg_g = 1 - g; 00052 seg_p = 1 - p; 00053 } 00054 00055 //Finally switch off all segments again, we must do this otherwise when 00056 //switching com the segments will be momentarily duplicated from this led. 00057 seg_a = seg_b = seg_c = seg_d = seg_e = seg_f = seg_g = seg_p = 1; 00058 } 00059 00060 /****************************************************** 00061 * Main loop repeatedly updates each of the LEDs * 00062 ******************************************************/ 00063 int main() 00064 { 00065 for(;;) 00066 { 00067 //Set the display to read '42' 00068 SetSegments(left, 0,1,1,0,0,1,1,0); //4 00069 SetSegments(right, 1,1,0,1,1,0,1,0); //2 00070 } 00071 } 00072
Generated on Tue Jul 26 2022 18:28:02 by
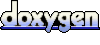