
Workshop example
Dependencies: X_NUCLEO_COMMON ST_INTERFACES
MySystemStorage.cpp
00001 /* 00002 * Copyright (c) 2018 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "BlockDevice.h" 00017 #include "FileSystem.h" 00018 #include "FATFileSystem.h" 00019 #include "LittleFileSystem.h" 00020 00021 #if COMPONENT_SPIF 00022 #include "SPIFBlockDevice.h" 00023 #endif 00024 00025 #if COMPONENT_QSPIF 00026 #include "QSPIFBlockDevice.h" 00027 #endif 00028 00029 #if COMPONENT_DATAFLASH 00030 #include "DataFlashBlockDevice.h" 00031 #endif 00032 00033 #if COMPONENT_SD 00034 #include "SDBlockDevice.h" 00035 #endif 00036 00037 #if COMPONENT_FLASHIAP 00038 #include "FlashIAPBlockDevice.h" 00039 #endif 00040 00041 #if COMPONENT_NUSD 00042 #include "NuSDBlockDevice.h" 00043 #endif 00044 00045 using namespace mbed; 00046 00047 // Align a value to a specified size. 00048 // Parameters : 00049 // val - [IN] Value. 00050 // size - [IN] Size. 00051 // Return : Aligned value. 00052 static inline uint32_t align_up(uint32_t val, uint32_t size) 00053 { 00054 return (((val - 1) / size) + 1) * size; 00055 } 00056 00057 BlockDevice *BlockDevice::get_default_instance() 00058 { 00059 #if COMPONENT_SPIF 00060 00061 static SPIFBlockDevice default_bd( 00062 MBED_CONF_SPIF_DRIVER_SPI_MOSI, 00063 MBED_CONF_SPIF_DRIVER_SPI_MISO, 00064 MBED_CONF_SPIF_DRIVER_SPI_CLK, 00065 MBED_CONF_SPIF_DRIVER_SPI_CS, 00066 MBED_CONF_SPIF_DRIVER_SPI_FREQ 00067 ); 00068 00069 return &default_bd; 00070 00071 #elif COMPONENT_QSPIF 00072 00073 static QSPIFBlockDevice default_bd( 00074 MBED_CONF_QSPIF_QSPI_IO0, 00075 MBED_CONF_QSPIF_QSPI_IO1, 00076 MBED_CONF_QSPIF_QSPI_IO2, 00077 MBED_CONF_QSPIF_QSPI_IO3, 00078 MBED_CONF_QSPIF_QSPI_SCK, 00079 MBED_CONF_QSPIF_QSPI_CSN, 00080 MBED_CONF_QSPIF_QSPI_POLARITY_MODE, 00081 MBED_CONF_QSPIF_QSPI_FREQ 00082 ); 00083 00084 return &default_bd; 00085 00086 #elif COMPONENT_DATAFLASH 00087 00088 static DataFlashBlockDevice default_bd( 00089 MBED_CONF_DATAFLASH_SPI_MOSI, 00090 MBED_CONF_DATAFLASH_SPI_MISO, 00091 MBED_CONF_DATAFLASH_SPI_CLK, 00092 MBED_CONF_DATAFLASH_SPI_CS 00093 ); 00094 00095 return &default_bd; 00096 00097 #elif COMPONENT_SD 00098 00099 static SDBlockDevice default_bd( 00100 MBED_CONF_SD_SPI_MOSI, 00101 MBED_CONF_SD_SPI_MISO, 00102 MBED_CONF_SD_SPI_CLK, 00103 MBED_CONF_SD_SPI_CS 00104 ); 00105 00106 return &default_bd; 00107 00108 #elif COMPONENT_NUSD 00109 00110 static NuSDBlockDevice default_bd; 00111 00112 return &default_bd; 00113 00114 #elif COMPONENT_FLASHIAP 00115 00116 #if (MBED_CONF_FLASHIAP_BLOCK_DEVICE_SIZE == 0) && (MBED_CONF_FLASHIAP_BLOCK_DEVICE_BASE_ADDRESS == 0xFFFFFFFF) 00117 00118 size_t flash_size; 00119 uint32_t start_address; 00120 uint32_t bottom_address; 00121 FlashIAP flash; 00122 00123 int ret = flash.init(); 00124 if (ret != 0) { 00125 return 0; 00126 } 00127 00128 //Find the start of first sector after text area 00129 bottom_address = align_up(FLASHIAP_ROM_END, flash.get_sector_size(FLASHIAP_ROM_END)); 00130 start_address = flash.get_flash_start(); 00131 flash_size = flash.get_flash_size(); 00132 00133 ret = flash.deinit(); 00134 00135 static FlashIAPBlockDevice default_bd(bottom_address, start_address + flash_size - bottom_address); 00136 00137 #else 00138 00139 static FlashIAPBlockDevice default_bd; 00140 00141 #endif 00142 00143 return &default_bd; 00144 00145 #else 00146 00147 return NULL; 00148 00149 #endif 00150 00151 } 00152 00153 FileSystem *FileSystem::get_default_instance() 00154 { 00155 #if COMPONENT_SPIF || COMPONENT_QSPIF || COMPONENT_DATAFLASH || COMPONENT_NUSD 00156 00157 static LittleFileSystem flash("flash", BlockDevice::get_default_instance()); 00158 flash.set_as_default(); 00159 00160 return &flash; 00161 00162 #elif COMPONENT_SD 00163 00164 static FATFileSystem sdcard("sd", BlockDevice::get_default_instance()); 00165 sdcard.set_as_default(); 00166 00167 return &sdcard; 00168 00169 #elif COMPONENT_FLASHIAP 00170 00171 static LittleFileSystem flash("flash", BlockDevice::get_default_instance()); 00172 flash.set_as_default(); 00173 00174 return &flash; 00175 00176 #else 00177 00178 return NULL; 00179 00180 #endif 00181 00182 }
Generated on Tue Jul 12 2022 22:34:14 by
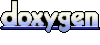