
Workshop example
Dependencies: X_NUCLEO_COMMON ST_INTERFACES
LPS22HB_driver.h
00001 /** 00002 ****************************************************************************** 00003 * @file LPS22HB_driver.h 00004 * @author HESA Application Team 00005 * @version V1.1 00006 * @date 10-August-2016 00007 * @brief LPS22HB driver header file 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Define to prevent recursive inclusion -------------------------------------*/ 00039 #ifndef __LPS22HB_DRIVER__H 00040 #define __LPS22HB_DRIVER__H 00041 00042 #include <stdint.h> 00043 00044 #ifdef __cplusplus 00045 extern "C" { 00046 #endif 00047 00048 // the user must include the proper file where HAL_read_reg and HAL_write_reg are implemented 00049 //#include "HAL_EnvSensors.h" 00050 00051 /* Uncomment the line below to expanse the "assert_param" macro in the drivers code */ 00052 //#define USE_FULL_ASSERT_LPS22HB 00053 00054 /* Exported macro ------------------------------------------------------------*/ 00055 #ifdef USE_FULL_ASSERT_LPS22HB 00056 00057 /** 00058 * @brief The assert_param macro is used for function's parameters check. 00059 * @param expr: If expr is false, it calls assert_failed function which reports 00060 * the name of the source file and the source line number of the call 00061 * that failed. If expr is true, it returns no value. 00062 * @retval None 00063 */ 00064 #define LPS22HB_assert_param(expr) ((expr) ? (void)0 : LPS22HB_assert_failed((uint8_t *)__FILE__, __LINE__)) 00065 /* Exported functions ------------------------------------------------------- */ 00066 void LPS22HB_assert_failed(uint8_t* file, uint32_t line); 00067 #else 00068 #define LPS22HB_assert_param(expr) ((void)0) 00069 #endif /* USE_FULL_ASSERT_LPS22HB */ 00070 00071 /** @addtogroup Environmental_Sensor 00072 * @{ 00073 */ 00074 00075 /** @addtogroup LPS22HB_DRIVER 00076 * @{ 00077 */ 00078 00079 /* Exported Types -------------------------------------------------------------*/ 00080 /** @defgroup LPS22HB_Exported_Types 00081 * @{ 00082 */ 00083 00084 /** 00085 * @brief Error type. 00086 */ 00087 typedef enum {LPS22HB_OK = (uint8_t)0, LPS22HB_ERROR = !LPS22HB_OK} LPS22HB_Error_et; 00088 00089 /** 00090 * @brief Enable/Disable type. 00091 */ 00092 typedef enum {LPS22HB_DISABLE = (uint8_t)0, LPS22HB_ENABLE = !LPS22HB_DISABLE} LPS22HB_State_et; 00093 #define IS_LPS22HB_State(MODE) ((MODE == LPS22HB_ENABLE) || (MODE == LPS22HB_DISABLE) ) 00094 00095 /** 00096 * @brief Bit status type. 00097 */ 00098 typedef enum {LPS22HB_RESET = (uint8_t)0, LPS22HB_SET = !LPS22HB_RESET} LPS22HB_BitStatus_et; 00099 #define IS_LPS22HB_BitStatus(MODE) ((MODE == LPS22HB_RESET) || (MODE == LPS22HB_SET)) 00100 00101 /*RES_CONF see LC_EN bit*/ 00102 /** 00103 * @brief LPS22HB Power/Noise Mode configuration. 00104 */ 00105 typedef enum { 00106 LPS22HB_LowNoise = (uint8_t)0x00, /*!< Low Noise mode */ 00107 LPS22HB_LowPower = (uint8_t)0x01 /*!< Low Current mode */ 00108 } LPS22HB_PowerMode_et; 00109 00110 #define IS_LPS22HB_PowerMode(MODE) ((MODE == LPS22HB_LowNoise) || (MODE == LPS22HB_LowPower)) 00111 00112 /** 00113 * @brief Output data rate configuration. 00114 */ 00115 typedef enum { 00116 00117 LPS22HB_ODR_ONE_SHOT = (uint8_t)0x00, /*!< Output Data Rate: one shot */ 00118 LPS22HB_ODR_1HZ = (uint8_t)0x10, /*!< Output Data Rate: 1Hz */ 00119 LPS22HB_ODR_10HZ = (uint8_t)0x20, /*!< Output Data Rate: 10Hz */ 00120 LPS22HB_ODR_25HZ = (uint8_t)0x30, /*!< Output Data Rate: 25Hz */ 00121 LPS22HB_ODR_50HZ = (uint8_t)0x40, /*!< Output Data Rate: 50Hz */ 00122 LPS22HB_ODR_75HZ = (uint8_t)0x50 /*!< Output Data Rate: 75Hz */ 00123 } LPS22HB_Odr_et; 00124 00125 #define IS_LPS22HB_ODR(ODR) ((ODR == LPS22HB_ODR_ONE_SHOT) || (ODR == LPS22HB_ODR_1HZ) || \ 00126 (ODR == LPS22HB_ODR_10HZ) || (ODR == LPS22HB_ODR_25HZ)|| (ODR == LPS22HB_ODR_50HZ) || (ODR == LPS22HB_ODR_75HZ)) 00127 00128 /** 00129 * @brief Low Pass Filter Cutoff Configuration. 00130 */ 00131 typedef enum { 00132 00133 LPS22HB_ODR_9 = (uint8_t)0x00, /*!< Filter Cutoff ODR/9 */ 00134 LPS22HB_ODR_20 = (uint8_t)0x04 /*!< Filter Cutoff ODR/20 */ 00135 } LPS22HB_LPF_Cutoff_et; 00136 00137 #define IS_LPS22HB_LPF_Cutoff(CUTOFF) ((CUTOFF == LPS22HB_ODR_9) || (CUTOFF == LPS22HB_ODR_20) ) 00138 00139 /** 00140 * @brief Block data update. 00141 */ 00142 00143 typedef enum { 00144 LPS22HB_BDU_CONTINUOUS_UPDATE = (uint8_t)0x00, /*!< Data updated continuously */ 00145 LPS22HB_BDU_NO_UPDATE = (uint8_t)0x02 /*!< Data updated after a read operation */ 00146 } LPS22HB_Bdu_et; 00147 #define IS_LPS22HB_BDUMode(MODE) ((MODE == LPS22HB_BDU_CONTINUOUS_UPDATE) || (MODE == LPS22HB_BDU_NO_UPDATE)) 00148 00149 /** 00150 * @brief LPS22HB Spi Mode configuration. 00151 */ 00152 typedef enum { 00153 LPS22HB_SPI_4_WIRE = (uint8_t)0x00, 00154 LPS22HB_SPI_3_WIRE = (uint8_t)0x01 00155 } LPS22HB_SPIMode_et; 00156 00157 #define IS_LPS22HB_SPIMode(MODE) ((MODE == LPS22HB_SPI_4_WIRE) || (MODE == LPS22HB_SPI_3_WIRE)) 00158 00159 00160 /** 00161 * @brief LPS22HB Interrupt Active Level Configuration (on High or Low) 00162 */ 00163 typedef enum 00164 { 00165 LPS22HB_ActiveHigh = (uint8_t)0x00, 00166 LPS22HB_ActiveLow = (uint8_t)0x80 00167 }LPS22HB_InterruptActiveLevel_et; 00168 #define IS_LPS22HB_InterruptActiveLevel(MODE) ((MODE == LPS22HB_ActiveHigh) || (MODE == LPS22HB_ActiveLow)) 00169 00170 /** 00171 * @brief LPS22HB Push-pull/Open Drain selection on Interrupt pads. 00172 */ 00173 typedef enum 00174 { 00175 LPS22HB_PushPull = (uint8_t)0x00, 00176 LPS22HB_OpenDrain = (uint8_t)0x40 00177 }LPS22HB_OutputType_et; 00178 #define IS_LPS22HB_OutputType(MODE) ((MODE == LPS22HB_PushPull) || (MODE == LPS22HB_OpenDrain)) 00179 00180 00181 /** 00182 * @brief Data Signal on INT pad control bits. 00183 */ 00184 typedef enum 00185 { 00186 LPS22HB_DATA = (uint8_t)0x00, 00187 LPS22HB_P_HIGH = (uint8_t)0x01, 00188 LPS22HB_P_LOW = (uint8_t)0x02, 00189 LPS22HB_P_LOW_HIGH = (uint8_t)0x03 00190 }LPS22HB_OutputSignalConfig_et; 00191 #define IS_LPS22HB_OutputSignal(MODE) ((MODE == LPS22HB_DATA) || (MODE == LPS22HB_P_HIGH)||\ 00192 (MODE == LPS22HB_P_LOW) || (MODE == LPS22HB_P_LOW_HIGH)) 00193 00194 00195 00196 /** 00197 * @brief LPS22HB Interrupt Differential Status. 00198 */ 00199 00200 typedef struct 00201 { 00202 uint8_t PH ; /*!< High Differential Pressure event occured */ 00203 uint8_t PL ; /*!< Low Differential Pressure event occured */ 00204 uint8_t IA ; /*!< One or more interrupt events have been generated.Interrupt Active */ 00205 uint8_t BOOT ; /*!< i '1' indicates that the Boot (Reboot) phase is running */ 00206 }LPS22HB_InterruptDiffStatus_st; 00207 00208 00209 /** 00210 * @brief LPS22HB Pressure and Temperature data status. 00211 */ 00212 typedef struct 00213 { 00214 uint8_t TempDataAvailable ; /*!< Temperature data available bit */ 00215 uint8_t PressDataAvailable ; /*!< Pressure data available bit */ 00216 uint8_t TempDataOverrun ; /*!< Temperature data over-run bit */ 00217 uint8_t PressDataOverrun ; /*!< Pressure data over-run bit */ 00218 }LPS22HB_DataStatus_st; 00219 00220 00221 /** 00222 * @brief LPS22HB Clock Tree configuration. 00223 */ 00224 typedef enum { 00225 LPS22HB_CTE_NotBalanced = (uint8_t)0x00, 00226 LPS22HB_CTE_Balanced = (uint8_t)0x20 00227 } LPS22HB_CTE_et; 00228 00229 #define IS_LPS22HB_CTE(MODE) ((MODE == LPS22HB_CTE_NotBalanced) || (MODE == LPS22HB_CTE_Balanced)) 00230 00231 /** 00232 * @brief LPS22HB Fifo Mode. 00233 */ 00234 00235 typedef enum { 00236 LPS22HB_FIFO_BYPASS_MODE = (uint8_t)0x00, /*!< The FIFO is disabled and empty. The pressure is read directly*/ 00237 LPS22HB_FIFO_MODE = (uint8_t)0x20, /*!< Stops collecting data when full */ 00238 LPS22HB_FIFO_STREAM_MODE = (uint8_t)0x40, /*!< Keep the newest measurements in the FIFO*/ 00239 LPS22HB_FIFO_TRIGGER_STREAMTOFIFO_MODE = (uint8_t)0x60, /*!< STREAM MODE until trigger deasserted, then change to FIFO MODE*/ 00240 LPS22HB_FIFO_TRIGGER_BYPASSTOSTREAM_MODE = (uint8_t)0x80, /*!< BYPASS MODE until trigger deasserted, then STREAM MODE*/ 00241 LPS22HB_FIFO_TRIGGER_BYPASSTOFIFO_MODE = (uint8_t)0xE0 /*!< BYPASS mode until trigger deasserted, then FIFO MODE*/ 00242 } LPS22HB_FifoMode_et; 00243 00244 #define IS_LPS22HB_FifoMode(MODE) ((MODE == LPS22HB_FIFO_BYPASS_MODE) || (MODE ==LPS22HB_FIFO_MODE)||\ 00245 (MODE == LPS22HB_FIFO_STREAM_MODE) || (MODE == LPS22HB_FIFO_TRIGGER_STREAMTOFIFO_MODE)||\ 00246 (MODE == LPS22HB_FIFO_TRIGGER_BYPASSTOSTREAM_MODE) || (MODE == LPS22HB_FIFO_TRIGGER_BYPASSTOFIFO_MODE)) 00247 00248 00249 /** 00250 * @brief LPS22HB Fifo Satus. 00251 */ 00252 typedef struct { 00253 uint8_t FIFO_LEVEL ; /*!< FIFO Stored data level: 00000: FIFO empty; 10000: FIFO is FULL and ha 32 unread samples */ 00254 uint8_t FIFO_EMPTY ; /*!< Empty FIFO Flag .1 FIFO is empty (see FIFO_level) */ 00255 uint8_t FIFO_FULL ; /*!< Full FIFO flag.1 FIFO is Full (see FIFO_level) */ 00256 uint8_t FIFO_OVR ; /*!< Overrun bit status. 1 FIFO is full and at least one sample in the FIFO has been overwritten */ 00257 uint8_t FIFO_FTH ; /*!< FIFO Threshold (Watermark) Status. 1 FIFO filling is equal or higher then FTH (wtm) level.*/ 00258 }LPS22HB_FifoStatus_st; 00259 00260 00261 00262 /** 00263 * @brief LPS22HB Configuration structure definition. 00264 */ 00265 typedef struct 00266 { 00267 LPS22HB_PowerMode_et PowerMode ; /*!< Enable Low Current Mode (low Power) or Low Noise Mode*/ 00268 LPS22HB_Odr_et OutputDataRate ; /*!< Output Data Rate */ 00269 LPS22HB_Bdu_et BDU ; /*!< Enable to inhibit the output registers update between the reading of upper and lower register parts.*/ 00270 LPS22HB_State_et LowPassFilter ; /*!< Enable/ Disable Low Pass Filter */ 00271 LPS22HB_LPF_Cutoff_et LPF_Cutoff ; /*!< Low Pass Filter Configuration */ 00272 LPS22HB_SPIMode_et Sim ; /*!< SPI Serial Interface Mode selection */ 00273 LPS22HB_State_et IfAddInc ; /*!< Enable/Disable Register address automatically inceremented during a multiple byte access */ 00274 }LPS22HB_ConfigTypeDef_st; 00275 00276 00277 /** 00278 * @brief LPS22HB Interrupt structure definition . 00279 */ 00280 typedef struct { 00281 LPS22HB_InterruptActiveLevel_et INT_H_L ; /*!< Interrupt active high, low. Default value: 0 */ 00282 LPS22HB_OutputType_et PP_OD ; /*!< Push-pull/open drain selection on interrupt pads. Default value: 0 */ 00283 LPS22HB_OutputSignalConfig_et OutputSignal_INT ; /*!< Data signal on INT Pad: Data,Pressure High, Preessure Low,P High or Low*/ 00284 LPS22HB_State_et DRDY ; /*!< Enable/Disable Data Ready Interrupt on INT_DRDY Pin*/ 00285 LPS22HB_State_et FIFO_OVR ; /*!< Enable/Disable FIFO Overrun Interrupt on INT_DRDY Pin*/ 00286 LPS22HB_State_et FIFO_FTH ; /*!< Enable/Disable FIFO threshold (Watermark) interrupt on INT_DRDY pin.*/ 00287 LPS22HB_State_et FIFO_FULL ; /*!< Enable/Disable FIFO FULL interrupt on INT_DRDY pin.*/ 00288 LPS22HB_State_et LatchIRQ ; /*!< Latch Interrupt request in to INT_SOURCE reg*/ 00289 int16_t THS_threshold ; /*!< Threshold value for pressure interrupt generation*/ 00290 LPS22HB_State_et AutoRifP ; /*!< Enable/Disable AutoRifP function */ 00291 LPS22HB_State_et AutoZero ; /*!< Enable/Disable AutoZero function */ 00292 }LPS22HB_InterruptTypeDef_st; 00293 00294 /** 00295 * @brief LPS22HB FIFO structure definition. 00296 */ 00297 typedef struct { 00298 LPS22HB_FifoMode_et FIFO_MODE ; /*!< Fifo Mode Selection */ 00299 LPS22HB_State_et WTM_INT ; /*!< Enable/Disable the watermark interrupt*/ 00300 uint8_t WTM_LEVEL ; /*!< FIFO threshold/Watermark level selection*/ 00301 }LPS22HB_FIFOTypeDef_st; 00302 00303 #define IS_LPS22HB_WtmLevel(LEVEL) ((LEVEL > 0) && (LEVEL <=31)) 00304 /** 00305 * @brief LPS22HB Measure Type definition. 00306 */ 00307 typedef struct { 00308 int16_t Tout; 00309 int32_t Pout; 00310 }LPS22HB_MeasureTypeDef_st; 00311 00312 00313 /** 00314 * @brief LPS22HB Driver Version Info structure definition. 00315 */ 00316 typedef struct { 00317 uint8_t Major; 00318 uint8_t Minor; 00319 uint8_t Point; 00320 }LPS22HB_driverVersion_st; 00321 00322 00323 /** 00324 * @brief Bitfield positioning. 00325 */ 00326 #define LPS22HB_BIT(x) ((uint8_t)x) 00327 00328 /** 00329 * @brief I2C address. 00330 */ 00331 /* SD0/SA0(pin 5) is connected to the voltage supply */ 00332 #define LPS22HB_ADDRESS_HIGH 0xBA /**< LPS22HB I2C Address High */ 00333 /* SDO/SA0 (pin5) is connected to the GND */ 00334 #define LPS22HB_ADDRESS_LOW 0xB8 /**< LPS22HB I2C Address Low */ 00335 00336 /** 00337 * @brief Set the LPS22HB driver version. 00338 */ 00339 00340 #define LPS22HB_driverVersion_Major (uint8_t)1 00341 #define LPS22HB_driverVersion_Minor (uint8_t)0 00342 #define LPS22HB_driverVersion_Point (uint8_t)0 00343 00344 /** 00345 * @} 00346 */ 00347 00348 00349 /* Exported Constants ---------------------------------------------------------*/ 00350 /** @defgroup LPS22HB_Exported_Constants 00351 * @{ 00352 */ 00353 00354 00355 /** 00356 * @addtogroup LPS22HB_Register 00357 * @{ 00358 */ 00359 00360 00361 00362 /** 00363 * @brief Device Identification register. 00364 * \code 00365 * Read 00366 * Default value: 0xB1 00367 * 7:0 This read-only register contains the device identifier that, for LPS22HB, is set to B1h. 00368 * \endcode 00369 */ 00370 00371 #define LPS22HB_WHO_AM_I_REG (uint8_t)0x0F 00372 00373 /** 00374 * @brief Device Identification value. 00375 */ 00376 #define LPS22HB_WHO_AM_I_VAL (uint8_t)0xB1 00377 00378 00379 /** 00380 * @brief Reference Pressure Register(LSB data) 00381 * \code 00382 * Read/write 00383 * Default value: 0x00 00384 * 7:0 REFL7-0: Lower part of the reference pressure value that 00385 * is sum to the sensor output pressure. 00386 * \endcode 00387 */ 00388 #define LPS22HB_REF_P_XL_REG (uint8_t)0x15 00389 00390 00391 /** 00392 * @brief Reference Pressure Register (Middle data) 00393 * \code 00394 * Read/write 00395 * Default value: 0x00 00396 * 7:0 REFL15-8: Middle part of the reference pressure value that 00397 * is sum to the sensor output pressure. 00398 * \endcode 00399 */ 00400 #define LPS22HB_REF_P_L_REG (uint8_t)0x16 00401 00402 /** 00403 * @brief Reference Pressure Register (MSB data) 00404 * \code 00405 * Read/write 00406 * Default value: 0x00 00407 * 7:0 REFL23-16 Higest part of the reference pressure value that 00408 * is sum to the sensor output pressure. 00409 * \endcode 00410 */ 00411 #define LPS22HB_REF_P_H_REG (uint8_t)0x17 00412 00413 00414 /** 00415 * @brief Pressure and temperature resolution mode Register 00416 * \code 00417 * Read/write 00418 * Default value: 0x05 00419 * 7:2 These bits must be set to 0 for proper operation of the device 00420 * 1: Reserved 00421 * 0 LC_EN: Low Current Mode Enable. Default 0 00422 * \endcode 00423 */ 00424 #define LPS22HB_RES_CONF_REG (uint8_t)0x1A 00425 00426 #define LPS22HB_LCEN_MASK (uint8_t)0x01 00427 00428 /** 00429 * @brief Control Register 1 00430 * \code 00431 * Read/write 00432 * Default value: 0x00 00433 * 7: This bit must be set to 0 for proper operation of the device 00434 * 6:4 ODR2, ODR1, ODR0: output data rate selection.Default 000 00435 * ODR2 | ODR1 | ODR0 | Pressure output data-rate(Hz) | Pressure output data-rate(Hz) 00436 * ---------------------------------------------------------------------------------- 00437 * 0 | 0 | 0 | one shot | one shot 00438 * 0 | 0 | 1 | 1 | 1 00439 * 0 | 1 | 0 | 10 | 10 00440 * 0 | 1 | 1 | 25 | 25 00441 * 1 | 0 | 0 | 50 | 50 00442 * 1 | 0 | 1 | 75 | 75 00443 * 1 | 1 | 0 | Reserved | Reserved 00444 * 1 | 1 | 1 | Reserved | Reserved 00445 * 00446 * 3 EN_LPFP: Enable Low Pass filter on Pressure data. Default value:0 00447 * 2:LPF_CFG Low-pass configuration register. (0: Filter cutoff is ODR/9; 1: filter cutoff is ODR/20) 00448 * 1 BDU: block data update. 0 - continuous update; 1 - output registers not updated until MSB and LSB reading. 00449 * 0 SIM: SPI Serial Interface Mode selection. 0 - SPI 4-wire; 1 - SPI 3-wire 00450 * \endcode 00451 */ 00452 #define LPS22HB_CTRL_REG1 (uint8_t)0x10 00453 00454 #define LPS22HB_ODR_MASK (uint8_t)0x70 00455 #define LPS22HB_LPFP_MASK (uint8_t)0x08 00456 #define LPS22HB_LPFP_CUTOFF_MASK (uint8_t)0x04 00457 #define LPS22HB_BDU_MASK (uint8_t)0x02 00458 #define LPS22HB_SIM_MASK (uint8_t)0x01 00459 00460 #define LPS22HB_LPFP_BIT LPS22HB_BIT(3) 00461 00462 00463 /** 00464 * @brief Control Register 2 00465 * \code 00466 * Read/write 00467 * Default value: 0x10 00468 * 7 BOOT: Reboot memory content. 0: normal mode; 1: reboot memory content. Self-clearing upon completation 00469 * 6 FIFO_EN: FIFO Enable. 0: disable; 1: enable 00470 * 5 STOP_ON_FTH: Stop on FIFO Threshold FIFO Watermark level use. 0: disable; 1: enable 00471 * 4 IF_ADD_INC: Register address automatically incrementeed during a multiple byte access with a serial interface (I2C or SPI). Default value 1.( 0: disable; 1: enable) 00472 * 3 I2C DIS: Disable I2C interface 0: I2C Enabled; 1: I2C disabled 00473 * 2 SWRESET: Software reset. 0: normal mode; 1: SW reset. Self-clearing upon completation 00474 * 1 AUTO_ZERO: Autozero enable. 0: normal mode; 1: autozero enable. 00475 * 0 ONE_SHOT: One shot enable. 0: waiting for start of conversion; 1: start for a new dataset 00476 * \endcode 00477 */ 00478 #define LPS22HB_CTRL_REG2 (uint8_t)0x11 00479 00480 #define LPS22HB_BOOT_BIT LPS22HB_BIT(7) 00481 #define LPS22HB_FIFO_EN_BIT LPS22HB_BIT(6) 00482 #define LPS22HB_WTM_EN_BIT LPS22HB_BIT(5) 00483 #define LPS22HB_ADD_INC_BIT LPS22HB_BIT(4) 00484 #define LPS22HB_I2C_BIT LPS22HB_BIT(3) 00485 #define LPS22HB_SW_RESET_BIT LPS22HB_BIT(2) 00486 00487 #define LPS22HB_FIFO_EN_MASK (uint8_t)0x40 00488 #define LPS22HB_WTM_EN_MASK (uint8_t)0x20 00489 #define LPS22HB_ADD_INC_MASK (uint8_t)0x10 00490 #define LPS22HB_I2C_MASK (uint8_t)0x08 00491 #define LPS22HB_ONE_SHOT_MASK (uint8_t)0x01 00492 00493 00494 /** 00495 * @brief CTRL Reg3 Interrupt Control Register 00496 * \code 00497 * Read/write 00498 * Default value: 0x00 00499 * 7 INT_H_L: Interrupt active high, low. 0:active high; 1: active low. 00500 * 6 PP_OD: Push-Pull/OpenDrain selection on interrupt pads. 0: Push-pull; 1: open drain. 00501 * 5 F_FSS5: FIFO full flag on INT_DRDY pin. Defaul value: 0. (0: Diasable; 1 : Enable). 00502 * 4 F_FTH: FIFO threshold (watermark) status on INT_DRDY pin. Defaul value: 0. (0: Diasable; 1 : Enable). 00503 * 3 F_OVR: FIFO overrun interrupt on INT_DRDY pin. Defaul value: 0. (0: Diasable; 1 : Enable). 00504 * 2 DRDY: Data-ready signal on INT_DRDY pin. Defaul value: 0. (0: Diasable; 1 : Enable). 00505 * 1:0 INT_S2, INT_S1: data signal on INT pad control bits. 00506 * INT_S2 | INT_S1 | INT pin 00507 * ------------------------------------------------------ 00508 * 0 | 0 | Data signal( in order of priority:PTH_DRDY or F_FTH or F_OVR_or F_FSS5 00509 * 0 | 1 | Pressure high (P_high) 00510 * 1 | 0 | Pressure low (P_low) 00511 * 1 | 1 | P_low OR P_high 00512 * \endcode 00513 */ 00514 #define LPS22HB_CTRL_REG3 (uint8_t)0x12 00515 00516 #define LPS22HB_PP_OD_BIT LPS22HB_BIT(6) 00517 #define LPS22HB_FIFO_FULL_BIT LPS22HB_BIT(5) 00518 #define LPS22HB_FIFO_FTH_BIT LPS22HB_BIT(4) 00519 #define LPS22HB_FIFO_OVR_BIT LPS22HB_BIT(3) 00520 #define LPS22HB_DRDY_BIT LPS22HB_BIT(2) 00521 00522 00523 #define LPS22HB_INT_H_L_MASK (uint8_t)0x80 00524 #define LPS22HB_PP_OD_MASK (uint8_t)0x40 00525 #define LPS22HB_FIFO_FULL_MASK (uint8_t)0x20 00526 #define LPS22HB_FIFO_FTH_MASK (uint8_t)0x10 00527 #define LPS22HB_FIFO_OVR_MASK (uint8_t)0x08 00528 #define LPS22HB_DRDY_MASK (uint8_t)0x04 00529 #define LPS22HB_INT_S12_MASK (uint8_t)0x03 00530 00531 00532 /** 00533 * @brief Interrupt Differential configuration Register 00534 * \code 00535 * Read/write 00536 * Default value: 0x00. 00537 * 7 AUTORIFP: AutoRifP Enable ?? 00538 * 6 RESET_ARP: Reset AutoRifP function 00539 * 4 AUTOZERO: Autozero enabled 00540 * 5 RESET_AZ: Reset Autozero Function 00541 * 3 DIFF_EN: Interrupt generation enable 00542 * 2 LIR: Latch Interrupt request into INT_SOURCE register. 0 - interrupt request not latched; 1 - interrupt request latched 00543 * 1 PL_E: Enable interrupt generation on differential pressure low event. 0 - disable; 1 - enable 00544 * 0 PH_E: Enable interrupt generation on differential pressure high event. 0 - disable; 1 - enable 00545 * \endcode 00546 */ 00547 #define LPS22HB_INTERRUPT_CFG_REG (uint8_t)0x0B 00548 00549 #define LPS22HB_DIFF_EN_BIT LPS22HB_BIT(3) 00550 #define LPS22HB_LIR_BIT LPS22HB_BIT(2) 00551 #define LPS22HB_PLE_BIT LPS22HB_BIT(1) 00552 #define LPS22HB_PHE_BIT LPS22HB_BIT(0) 00553 00554 #define LPS22HB_AUTORIFP_MASK (uint8_t)0x80 00555 #define LPS22HB_RESET_ARP_MASK (uint8_t)0x40 00556 #define LPS22HB_AUTOZERO_MASK (uint8_t)0x20 00557 #define LPS22HB_RESET_AZ_MASK (uint8_t)0x10 00558 #define LPS22HB_DIFF_EN_MASK (uint8_t)0x08 00559 #define LPS22HB_LIR_MASK (uint8_t)0x04 00560 #define LPS22HB_PLE_MASK (uint8_t)0x02 00561 #define LPS22HB_PHE_MASK (uint8_t)0x01 00562 00563 00564 00565 /** 00566 * @brief Interrupt source Register (It is cleared by reading it) 00567 * \code 00568 * Read 00569 * Default value: ----. 00570 * 7 BOOT_STATUS: If 1 indicates that the Boot (Reboot) phase is running. 00571 * 6:3 Reserved: Keep these bits at 0 00572 * 2 IA: Interrupt Active.0: no interrupt has been generated; 1: one or more interrupt events have been generated. 00573 * 1 PL: Differential pressure Low. 0: no interrupt has been generated; 1: Low differential pressure event has occurred. 00574 * 0 PH: Differential pressure High. 0: no interrupt has been generated; 1: High differential pressure event has occurred. 00575 * \endcode 00576 */ 00577 #define LPS22HB_INTERRUPT_SOURCE_REG (uint8_t)0x25 00578 00579 #define LPS22HB_BOOT_STATUS_BIT LPS22HB_BIT(7) 00580 #define LPS22HB_IA_BIT LPS22HB_BIT(2) 00581 #define LPS22HB_PL_BIT LPS22HB_BIT(1) 00582 #define LPS22HB_PH_BIT LPS22HB_BIT(0) 00583 00584 #define LPS22HB_BOOT_STATUS_MASK (uint8_t)0x80 00585 #define LPS22HB_IA_MASK (uint8_t)0x04 00586 #define LPS22HB_PL_MASK (uint8_t)0x02 00587 #define LPS22HB_PH_MASK (uint8_t)0x01 00588 00589 00590 /** 00591 * @brief Status Register 00592 * \code 00593 * Read 00594 * Default value: --- 00595 * 7:6 Reserved: 0 00596 * 5 T_OR: Temperature data overrun. 0: no overrun has occurred; 1: a new data for temperature has overwritten the previous one. 00597 * 4 P_OR: Pressure data overrun. 0: no overrun has occurred; 1: new data for pressure has overwritten the previous one. 00598 * 3:2 Reserved: 0 00599 * 1 T_DA: Temperature data available. 0: new data for temperature is not yet available; 1: new data for temperature is available. 00600 * 0 P_DA: Pressure data available. 0: new data for pressure is not yet available; 1: new data for pressure is available. 00601 * \endcode 00602 */ 00603 #define LPS22HB_STATUS_REG (uint8_t)0x27 00604 00605 #define LPS22HB_TOR_BIT LPS22HB_BIT(5) 00606 #define LPS22HB_POR_BIT LPS22HB_BIT(4) 00607 #define LPS22HB_TDA_BIT LPS22HB_BIT(1) 00608 #define LPS22HB_PDA_BIT LPS22HB_BIT(0) 00609 00610 #define LPS22HB_TOR_MASK (uint8_t)0x20 00611 #define LPS22HB_POR_MASK (uint8_t)0x10 00612 #define LPS22HB_TDA_MASK (uint8_t)0x02 00613 #define LPS22HB_PDA_MASK (uint8_t)0x01 00614 00615 00616 00617 /** 00618 * @brief Pressure data (LSB) register. 00619 * \code 00620 * Read 00621 * Default value: 0x00.(To be verified) 00622 * POUT7 - POUT0: Pressure data LSB (2's complement). 00623 * Pressure output data: Pout(hPA)=(PRESS_OUT_H & PRESS_OUT_L & 00624 * PRESS_OUT_XL)[dec]/4096. 00625 * \endcode 00626 */ 00627 00628 #define LPS22HB_PRESS_OUT_XL_REG (uint8_t)0x28 00629 /** 00630 * @brief Pressure data (Middle part) register. 00631 * \code 00632 * Read 00633 * Default value: 0x80. 00634 * POUT15 - POUT8: Pressure data middle part (2's complement). 00635 * Pressure output data: Pout(hPA)=(PRESS_OUT_H & PRESS_OUT_L & 00636 * PRESS_OUT_XL)[dec]/4096. 00637 * \endcode 00638 */ 00639 #define LPS22HB_PRESS_OUT_L_REG (uint8_t)0x29 00640 00641 /** 00642 * @brief Pressure data (MSB) register. 00643 * \code 00644 * Read 00645 * Default value: 0x2F. 00646 * POUT23 - POUT16: Pressure data MSB (2's complement). 00647 * Pressure output data: Pout(hPA)=(PRESS_OUT_H & PRESS_OUT_L & 00648 * PRESS_OUT_XL)[dec]/4096. 00649 * \endcode 00650 */ 00651 #define LPS22HB_PRESS_OUT_H_REG (uint8_t)0x2A 00652 00653 /** 00654 * @brief Temperature data (LSB) register. 00655 * \code 00656 * Read 00657 * Default value: 0x00. 00658 * TOUT7 - TOUT0: temperature data LSB. 00659 * Tout(degC)=TEMP_OUT/100 00660 * \endcode 00661 */ 00662 #define LPS22HB_TEMP_OUT_L_REG (uint8_t)0x2B 00663 00664 /** 00665 * @brief Temperature data (MSB) register. 00666 * \code 00667 * Read 00668 * Default value: 0x00. 00669 * TOUT15 - TOUT8: temperature data MSB. 00670 * Tout(degC)=TEMP_OUT/100 00671 * \endcode 00672 */ 00673 #define LPS22HBH_TEMP_OUT_H_REG (uint8_t)0x2C 00674 00675 /** 00676 * @brief Threshold pressure (LSB) register. 00677 * \code 00678 * Read/write 00679 * Default value: 0x00. 00680 * 7:0 THS7-THS0: LSB Threshold pressure Low part of threshold value for pressure interrupt 00681 * generation. The complete threshold value is given by THS_P_H & THS_P_L and is 00682 * expressed as unsigned number. P_ths(hPA)=(THS_P_H & THS_P_L)[dec]/16. 00683 * \endcode 00684 */ 00685 #define LPS22HB_THS_P_LOW_REG (uint8_t)0x0C 00686 00687 /** 00688 * @brief Threshold pressure (MSB) 00689 * \code 00690 * Read/write 00691 * Default value: 0x00. 00692 * 7:0 THS15-THS8: MSB Threshold pressure. High part of threshold value for pressure interrupt 00693 * generation. The complete threshold value is given by THS_P_H & THS_P_L and is 00694 * expressed as unsigned number. P_ths(mbar)=(THS_P_H & THS_P_L)[dec]/16. 00695 * \endcode 00696 */ 00697 #define LPS22HB_THS_P_HIGH_REG (uint8_t)0x0D 00698 00699 /** 00700 * @brief FIFO control register 00701 * \code 00702 * Read/write 00703 * Default value: 0x00 00704 * 7:5 F_MODE2, F_MODE1, F_MODE0: FIFO mode selection. 00705 * FM2 | FM1 | FM0 | FIFO MODE 00706 * --------------------------------------------------- 00707 * 0 | 0 | 0 | BYPASS MODE 00708 * 0 | 0 | 1 | FIFO MODE. Stops collecting data when full 00709 * 0 | 1 | 0 | STREAM MODE: Keep the newest measurements in the FIFO 00710 * 0 | 1 | 1 | STREAM MODE until trigger deasserted, then change to FIFO MODE 00711 * 1 | 0 | 0 | BYPASS MODE until trigger deasserted, then STREAM MODE 00712 * 1 | 0 | 1 | Reserved for future use 00713 * 1 | 1 | 0 | Reserved 00714 * 1 | 1 | 1 | BYPASS mode until trigger deasserted, then FIFO MODE 00715 * 00716 * 4:0 WTM_POINT4-0 : FIFO Watermark level selection (0-31) 00717 */ 00718 #define LPS22HB_CTRL_FIFO_REG (uint8_t)0x14 00719 00720 #define LPS22HB_FIFO_MODE_MASK (uint8_t)0xE0 00721 #define LPS22HB_WTM_POINT_MASK (uint8_t)0x1F 00722 00723 00724 /** 00725 * @brief FIFO Status register 00726 * \code 00727 * Read 00728 * Default value: ---- 00729 * 7 FTH_FIFO: FIFO threshold status. 0:FIFO filling is lower than FTH level; 1: FIFO is equal or higher than FTH level. 00730 * 6 OVR: Overrun bit status. 0 - FIFO not full; 1 -FIFO is full and at least one sample in the FIFO has been overwritten. 00731 * 5:0 FSS: FIFO Stored data level. 000000: FIFO empty, 100000: FIFO is full and has 32 unread samples. 00732 * \endcode 00733 */ 00734 #define LPS22HB_STATUS_FIFO_REG (uint8_t)0x26 00735 00736 #define LPS22HB_FTH_FIFO_BIT LPS22HB_BIT(7) 00737 #define LPS22HB_OVR_FIFO_BIT LPS22HB_BIT(6) 00738 00739 #define LPS22HB_FTH_FIFO_MASK (uint8_t)0x80 00740 #define LPS22HB_OVR_FIFO_MASK (uint8_t)0x40 00741 #define LPS22HB_LEVEL_FIFO_MASK (uint8_t)0x3F 00742 #define LPS22HB_FIFO_EMPTY (uint8_t)0x00 00743 #define LPS22HB_FIFO_FULL (uint8_t)0x20 00744 00745 00746 00747 /** 00748 * @brief Pressure offset register (LSB) 00749 * \code 00750 * Read/write 00751 * Default value: 0x00 00752 * 7:0 RPDS7-0:Pressure Offset for 1 point calibration (OPC) after soldering. 00753 * This register contains the low part of the pressure offset value after soldering,for 00754 * differential pressure computing. The complete value is given by RPDS_L & RPDS_H 00755 * and is expressed as signed 2 complement value. 00756 * \endcode 00757 */ 00758 #define LPS22HB_RPDS_L_REG (uint8_t)0x18 00759 00760 /** 00761 * @brief Pressure offset register (MSB) 00762 * \code 00763 * Read/write 00764 * Default value: 0x00 00765 * 7:0 RPDS15-8:Pressure Offset for 1 point calibration (OPC) after soldering. 00766 * This register contains the high part of the pressure offset value after soldering (see description RPDS_L) 00767 * \endcode 00768 */ 00769 #define LPS22HB_RPDS_H_REG (uint8_t)0x19 00770 00771 00772 /** 00773 * @brief Clock Tree Configuration register 00774 * \code 00775 * Read/write 00776 * Default value: 0x00 00777 * 7:6 Reserved. 00778 * 5: CTE: Clock Tree Enhancement 00779 * \endcode 00780 */ 00781 00782 #define LPS22HB_CLOCK_TREE_CONFIGURATION (uint8_t)0x43 00783 00784 #define LPS22HB_CTE_MASK (uint8_t)0x20 00785 00786 /** 00787 * @} 00788 */ 00789 00790 00791 /** 00792 * @} 00793 */ 00794 00795 00796 /* Exported Functions -------------------------------------------------------------*/ 00797 /** @defgroup LPS22HB_Exported_Functions 00798 * @{ 00799 */ 00800 00801 LPS22HB_Error_et LPS22HB_read_reg( void *handle, uint8_t RegAddr, uint16_t NumByteToRead, uint8_t *Data ); 00802 LPS22HB_Error_et LPS22HB_write_reg( void *handle, uint8_t RegAddr, uint16_t NumByteToWrite, uint8_t *Data ); 00803 00804 /** 00805 * @brief Init the HAL layer. 00806 * @param None 00807 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 00808 */ 00809 #define LPS22HB_HalInit (LPS22HB_Error_et)HAL_Init_I2C 00810 00811 /** 00812 * @brief DeInit the HAL layer. 00813 * @param None 00814 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 00815 */ 00816 #define LPS22HB_HalDeInit (LPS22HB_Error_et)HAL_DeInit_I2C 00817 00818 00819 /** 00820 * @brief Get the LPS22HB driver version. 00821 * @param None 00822 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 00823 */ 00824 LPS22HB_Error_et LPS22HB_Get_DriverVersion(LPS22HB_driverVersion_st *Version); 00825 00826 /** 00827 * @brief Initialization function for LPS22HB. 00828 * This function make a memory boot. 00829 * Init the sensor with a standard basic confifuration. 00830 * Low Power, ODR 25 Hz, Low Pass Filter disabled; BDU enabled; I2C enabled; 00831 * NO FIFO; NO Interrupt Enabled. 00832 * @param None. 00833 * @retval Error code[LPS22HB_ERROR, LPS22HB_OK] 00834 */ 00835 LPS22HB_Error_et LPS22HB_Init(void *handle); 00836 00837 /** 00838 * @brief DeInit the LPS2Hb driver. 00839 * @param None 00840 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 00841 */ 00842 00843 LPS22HB_Error_et LPS22HB_DeInit(void *handle); 00844 00845 00846 /** 00847 * @brief Read identification code by WHO_AM_I register 00848 * @param Buffer to empty by Device identification Value. 00849 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 00850 */ 00851 LPS22HB_Error_et LPS22HB_Get_DeviceID(void *handle, uint8_t* deviceid); 00852 00853 00854 /** 00855 * @brief Set LPS22HB Low Power or Low Noise Mode Configuration 00856 * @param LPS22HB_LowNoise or LPS22HB_LowPower mode 00857 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 00858 */ 00859 LPS22HB_Error_et LPS22HB_Set_PowerMode(void *handle, LPS22HB_PowerMode_et mode); 00860 00861 /** 00862 * @brief Get LPS22HB Power Mode 00863 * @param Buffer to empty with Mode: Low Noise or Low Current 00864 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 00865 */ 00866 LPS22HB_Error_et LPS22HB_Get_PowerMode(void *handle, LPS22HB_PowerMode_et* mode); 00867 00868 00869 /** 00870 * @brief Set LPS22HB Output Data Rate 00871 * @param Output Data Rate 00872 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 00873 */ 00874 LPS22HB_Error_et LPS22HB_Set_Odr(void *handle, LPS22HB_Odr_et odr); 00875 00876 00877 /** 00878 * @brief Get LPS22HB Output Data Rate 00879 * @param Buffer to empty with Output Data Rate 00880 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 00881 */ 00882 LPS22HB_Error_et LPS22HB_Get_Odr(void *handle, LPS22HB_Odr_et* odr); 00883 00884 /** 00885 * @brief Enable/Disale low-pass filter on LPS22HB pressure data 00886 * @param state: enable or disable 00887 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 00888 */ 00889 LPS22HB_Error_et LPS22HB_Set_LowPassFilter(void *handle, LPS22HB_State_et state); 00890 00891 00892 /** 00893 * @brief Set low-pass filter cutoff configuration on LPS22HB pressure data 00894 * @param Filter Cutoff ODR/9 or ODR/20 00895 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 00896 */ 00897 LPS22HB_Error_et LPS22HB_Set_LowPassFilterCutoff(void *handle, LPS22HB_LPF_Cutoff_et cutoff); 00898 00899 /** 00900 * @brief Set Block Data Update mode 00901 * @param LPS22HB_BDU_CONTINUOS_UPDATE/ LPS22HB_BDU_NO_UPDATE 00902 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 00903 */ 00904 LPS22HB_Error_et LPS22HB_Set_Bdu(void *handle, LPS22HB_Bdu_et bdu); 00905 00906 00907 /** 00908 * @brief Get Block Data Update mode 00909 * @param Buffer to empty whit the bdu mode read from sensor 00910 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 00911 */ 00912 LPS22HB_Error_et LPS22HB_Get_Bdu(void *handle, LPS22HB_Bdu_et* bdu); 00913 00914 /** 00915 * @brief Set SPI mode: 3 Wire Interface OR 4 Wire Interface 00916 * @param LPS22HB_SPI_4_WIRE/LPS22HB_SPI_3_WIRE 00917 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 00918 */ 00919 LPS22HB_Error_et LPS22HB_Set_SpiInterface(void *handle, LPS22HB_SPIMode_et spimode); 00920 00921 /** 00922 * @brief Get SPI mode: 3 Wire Interface OR 4 Wire Interface 00923 * @param buffer to empty with SPI mode 00924 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 00925 */ 00926 LPS22HB_Error_et LPS22HB_Get_SpiInterface(void *handle, LPS22HB_SPIMode_et* spimode); 00927 00928 /** 00929 * @brief Software Reset 00930 * @param void 00931 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 00932 */ 00933 LPS22HB_Error_et LPS22HB_SwReset(void *handle); 00934 00935 /** 00936 * @brief Reboot Memory Content. 00937 * @param void 00938 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 00939 */ 00940 LPS22HB_Error_et LPS22HB_MemoryBoot(void *handle); 00941 00942 /** 00943 * @brief Software Reset ann BOOT 00944 * @param void 00945 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 00946 */ 00947 LPS22HB_Error_et LPS22HB_SwResetAndMemoryBoot(void *handle); 00948 00949 00950 /** 00951 * @brief Enable or Disable FIFO 00952 * @param LPS22HB_ENABLE/LPS22HB_DISABLE 00953 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 00954 */ 00955 LPS22HB_Error_et LPS22HB_Set_FifoModeUse(void *handle, LPS22HB_State_et status); 00956 00957 /** 00958 * @brief Enable or Disable FIFO Watermark level use. Stop on FIFO Threshold 00959 * @param LPS22HB_ENABLE/LPS22HB_DISABLE 00960 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 00961 */ 00962 LPS22HB_Error_et LPS22HB_Set_FifoWatermarkLevelUse(void *handle, LPS22HB_State_et status); 00963 00964 /** 00965 * @brief Enable or Disable the Automatic increment register address during a multiple byte access with a serial interface (I2C or SPI) 00966 * @param LPS22HB_ENABLE/LPS22HB_DISABLE. Default is LPS22HB_ENABLE 00967 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 00968 */ 00969 LPS22HB_Error_et LPS22HB_Set_AutomaticIncrementRegAddress(void *handle, LPS22HB_State_et status); 00970 00971 00972 /** 00973 * @brief Set One Shot bit to start a new conversion (ODR mode has to be 000) 00974 * @param void 00975 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 00976 */ 00977 LPS22HB_Error_et LPS22HB_StartOneShotMeasurement(void *handle); 00978 00979 /** 00980 * @brief Enable/Disable I2C 00981 * @param State. Enable (reset bit)/ Disable (set bit) 00982 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 00983 */ 00984 LPS22HB_Error_et LPS22HB_Set_I2C(void *handle, LPS22HB_State_et i2cstate); 00985 00986 00987 /*CTRL_REG3 Interrupt Control*/ 00988 /** 00989 * @brief Set Interrupt Active on High or Low Level 00990 * @param LPS22HB_ActiveHigh/LPS22HB_ActiveLow 00991 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 00992 */ 00993 LPS22HB_Error_et LPS22HB_Set_InterruptActiveLevel(void *handle, LPS22HB_InterruptActiveLevel_et mode); 00994 00995 /** 00996 * @brief Set Push-pull/open drain selection on interrupt pads. 00997 * @param LPS22HB_PushPull/LPS22HB_OpenDrain 00998 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 00999 */ 01000 LPS22HB_Error_et LPS22HB_Set_InterruptOutputType(void *handle, LPS22HB_OutputType_et output); 01001 01002 /** 01003 * @brief Set Data signal on INT1 pad control bits. 01004 * @param LPS22HB_DATA,LPS22HB_P_HIGH_LPS22HB_P_LOW,LPS22HB_P_LOW_HIGH 01005 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 01006 */ 01007 LPS22HB_Error_et LPS22HB_Set_InterruptControlConfig(void *handle, LPS22HB_OutputSignalConfig_et config); 01008 01009 01010 /** 01011 * @brief Enable/Disable Data-ready signal on INT_DRDY pin. 01012 * @param LPS22HB_ENABLE/LPS22HB_DISABLE 01013 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 01014 */ 01015 LPS22HB_Error_et LPS22HB_Set_DRDYInterrupt(void *handle, LPS22HB_State_et status); 01016 01017 /** 01018 * @brief Enable/Disable FIFO overrun interrupt on INT_DRDY pin. 01019 * @param LPS22HB_ENABLE/LPS22HB_DISABLE 01020 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 01021 */ 01022 LPS22HB_Error_et LPS22HB_Set_FIFO_OVR_Interrupt(void *handle, LPS22HB_State_et status); 01023 01024 /** 01025 * @brief Enable/Disable FIFO threshold (Watermark) interrupt on INT_DRDY pin. 01026 * @param LPS22HB_ENABLE/LPS22HB_DISABLE 01027 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 01028 */ 01029 LPS22HB_Error_et LPS22HB_Set_FIFO_FTH_Interrupt(void *handle, LPS22HB_State_et status); 01030 01031 /** 01032 * @brief Enable/Disable FIFO FULL interrupt on INT_DRDY pin. 01033 * @param LPS22HB_ENABLE/LPS22HB_DISABLE 01034 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 01035 */ 01036 LPS22HB_Error_et LPS22HB_Set_FIFO_FULL_Interrupt(void *handle, LPS22HB_State_et status); 01037 01038 /** 01039 * @brief Enable AutoRifP function 01040 * @param none 01041 * @detail When this function is enabled, an internal register is set with the current pressure values 01042 * and the content is subtracted from the pressure output value and result is used for the interrupt generation. 01043 * the AutoRifP is slf creared. 01044 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 01045 */ 01046 LPS22HB_Error_et LPS22HB_Set_AutoRifP(void *handle); 01047 01048 /** 01049 * @brief Disable AutoRifP 01050 * @param none 01051 * @detail the RESET_ARP bit is used to disable the AUTORIFP function. This bis i is selfdleared 01052 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 01053 */ 01054 LPS22HB_Error_et LPS22HB_ResetAutoRifP(void *handle); 01055 01056 /**????? 01057 * @brief Set AutoZero Function bit 01058 * @detail When set to ‘1’, the actual pressure output is copied in the REF_P reg (@0x15..0x17) 01059 * @param None 01060 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 01061 */ 01062 LPS22HB_Error_et LPS22HB_Set_AutoZeroFunction(void *handle); 01063 01064 /**??? 01065 * @brief Set ResetAutoZero Function bit 01066 * @details REF_P reg (@0x015..17) set pressure reference to default value RPDS reg (0x18/19). 01067 * @param None 01068 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 01069 */ 01070 LPS22HB_Error_et LPS22HB_ResetAutoZeroFunction(void *handle); 01071 01072 01073 /** 01074 * @brief Enable/ Disable the computing of differential pressure output (Interrupt Generation) 01075 * @param LPS22HB_ENABLE,LPS22HB_DISABLE 01076 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 01077 */ 01078 LPS22HB_Error_et LPS22HB_Set_InterruptDifferentialGeneration(void *handle, LPS22HB_State_et diff_en) ; 01079 01080 01081 01082 /** 01083 * @brief Get the DIFF_EN bit value 01084 * @param buffer to empty with the read value of DIFF_EN bit 01085 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 01086 */ 01087 LPS22HB_Error_et LPS22HB_Get_InterruptDifferentialGeneration(void *handle, LPS22HB_State_et* diff_en); 01088 01089 01090 /** 01091 * @brief Latch Interrupt request to the INT_SOURCE register. 01092 * @param LPS22HB_ENABLE/LPS22HB_DISABLE 01093 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 01094 */ 01095 LPS22HB_Error_et LPS22HB_LatchInterruptRequest(void *handle, LPS22HB_State_et status); 01096 01097 /** 01098 * @brief Enable\Disable Interrupt Generation on differential pressure Low event 01099 * @param LPS22HB_ENABLE/LPS22HB_DISABLE 01100 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 01101 */ 01102 LPS22HB_Error_et LPS22HB_Set_PLE(void *handle, LPS22HB_State_et status); 01103 01104 /** 01105 * @brief Enable\Disable Interrupt Generation on differential pressure High event 01106 * @param LPS22HB_ENABLE/LPS22HB_DISABLE 01107 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 01108 */ 01109 LPS22HB_Error_et LPS22HB_Set_PHE(void *handle, LPS22HB_State_et status); 01110 01111 /** 01112 * @brief Get the Interrupt Generation on differential pressure status event and the Boot Status. 01113 * @detail The INT_SOURCE register is cleared by reading it. 01114 * @param Status Event Flag: BOOT, PH,PL,IA 01115 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 01116 */ 01117 LPS22HB_Error_et LPS22HB_Get_InterruptDifferentialEventStatus(void *handle, LPS22HB_InterruptDiffStatus_st* interruptsource); 01118 01119 01120 /** 01121 * @brief Get the status of Pressure and Temperature data 01122 * @param Data Status Flag: TempDataAvailable, TempDataOverrun, PressDataAvailable, PressDataOverrun 01123 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 01124 */ 01125 LPS22HB_Error_et LPS22HB_Get_DataStatus(void *handle, LPS22HB_DataStatus_st* datastatus); 01126 01127 01128 /** 01129 * @brief Get the LPS22HB raw presure value 01130 * @param The buffer to empty with the pressure raw value 01131 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 01132 */ 01133 LPS22HB_Error_et LPS22HB_Get_RawPressure(void *handle, int32_t *raw_press); 01134 01135 /** 01136 * @brief Get the LPS22HB Pressure value in hPA. 01137 * @param The buffer to empty with the pressure value that must be divided by 100 to get the value in hPA 01138 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 01139 */ 01140 LPS22HB_Error_et LPS22HB_Get_Pressure(void *handle, int32_t* Pout); 01141 01142 /** 01143 * @brief Read LPS22HB output register, and calculate the raw temperature. 01144 * @param The buffer to empty with the temperature raw value 01145 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 01146 */ 01147 LPS22HB_Error_et LPS22HB_Get_RawTemperature(void *handle, int16_t *raw_data); 01148 01149 /** 01150 * @brief Read the Temperature value in °C. 01151 * @param The buffer to empty with the temperature value that must be divided by 10 to get the value in ['C] 01152 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 01153 */ 01154 LPS22HB_Error_et LPS22HB_Get_Temperature(void *handle, int16_t* Tout); 01155 01156 /** 01157 * @brief Get the threshold value used for pressure interrupt generation. 01158 * @param The buffer to empty with the temperature value 01159 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 01160 */ 01161 LPS22HB_Error_et LPS22HB_Get_PressureThreshold(void *handle, int16_t *P_ths); 01162 01163 /** 01164 * @brief Set the threshold value used for pressure interrupt generation. 01165 * @param The buffer to empty with the temperature value 01166 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 01167 */ 01168 LPS22HB_Error_et LPS22HB_Set_PressureThreshold(void *handle, int16_t P_ths); 01169 01170 /** 01171 * @brief Set Fifo Mode. 01172 * @param Fifo Mode struct 01173 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 01174 */ 01175 LPS22HB_Error_et LPS22HB_Set_FifoMode(void *handle, LPS22HB_FifoMode_et fifomode); 01176 /** 01177 * @brief Get Fifo Mode. 01178 * @param Buffer to empty with fifo mode value 01179 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 01180 */ 01181 LPS22HB_Error_et LPS22HB_Get_FifoMode(void *handle, LPS22HB_FifoMode_et* fifomode); 01182 01183 /** 01184 * @brief Set Fifo Watermark Level. 01185 * @param Watermark level value [0 31] 01186 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 01187 */ 01188 LPS22HB_Error_et LPS22HB_Set_FifoWatermarkLevel(void *handle, uint8_t wtmlevel); 01189 01190 /** 01191 * @brief Get FIFO Watermark Level 01192 * @param buffer to empty with watermak level[0,31] value read from sensor 01193 * @retval Status [LPS22HB_ERROR, LPS22HB_OK] 01194 */ 01195 LPS22HB_Error_et LPS22HB_Get_FifoWatermarkLevel(void *handle, uint8_t *wtmlevel); 01196 01197 01198 /** 01199 * @brief Get Fifo Status. 01200 * @param Buffer to empty with fifo status 01201 * @retval Status [LPS22HB_ERROR, LPS22HB_OK] 01202 */ 01203 LPS22HB_Error_et LPS22HB_Get_FifoStatus(void *handle, LPS22HB_FifoStatus_st* status); 01204 01205 01206 /** 01207 * @brief Get the reference pressure after soldering for computing differential pressure (hPA) 01208 * @param buffer to empty with the he pressure value (hPA) 01209 * @retval Status [LPS22HB_ERROR, LPS22HB_OK] 01210 */ 01211 LPS22HB_Error_et LPS22HB_Get_PressureOffsetValue(void *handle, int16_t *pressoffset); 01212 01213 /** 01214 * @brief Get the Reference Pressure value 01215 * @detail It is a 24-bit data added to the sensor output measurement to detect a measured pressure beyond programmed limits. 01216 * @param Buffer to empty with reference pressure value 01217 * @retval Status [LPS22HB_ERROR, LPS22HB_OK] 01218 */ 01219 LPS22HB_Error_et LPS22HB_Get_ReferencePressure(void *handle, int32_t* RefP); 01220 01221 01222 /** 01223 * @brief Check if the single measurement has completed. 01224 * @param the returned value is set to 1, when the measurement is completed 01225 * @retval Status [LPS22HB_ERROR, LPS22HB_OK] 01226 */ 01227 LPS22HB_Error_et LPS22HB_IsMeasurementCompleted(void *handle, uint8_t* Is_Measurement_Completed); 01228 01229 01230 /** 01231 * @brief Get the values of the last single measurement. 01232 * @param Pressure and temperature value 01233 * @retvalStatus [LPS22HB_ERROR, LPS22HB_OK] 01234 */ 01235 LPS22HB_Error_et LPS22HB_Get_Measurement(void *handle, LPS22HB_MeasureTypeDef_st *Measurement_Value); 01236 01237 01238 /** 01239 * @brief Set Generic Configuration 01240 * @param Struct to empty with the chosen values 01241 * @retval Error code[LPS22HB_ERROR, LPS22HB_OK] 01242 */ 01243 LPS22HB_Error_et LPS22HB_Set_GenericConfig(void *handle, LPS22HB_ConfigTypeDef_st* pxLPS22HBInit); 01244 01245 /** 01246 * @brief Get Generic configuration 01247 * @param Struct to empty with configuration values 01248 * @retval Error code[LPS22HB_ERROR, LPS22HB_OK] 01249 */ 01250 LPS22HB_Error_et LPS22HB_Get_GenericConfig(void *handle, LPS22HB_ConfigTypeDef_st* pxLPS22HBInit); 01251 01252 /** 01253 * @brief Set Interrupt configuration 01254 * @param Struct holding the configuration values 01255 * @retval Error code[LPS22HB_ERROR, LPS22HB_OK] 01256 */ 01257 LPS22HB_Error_et LPS22HB_Set_InterruptConfig(void *handle, LPS22HB_InterruptTypeDef_st* pLPS22HBInt); 01258 01259 /** 01260 * @brief LPS22HBGet_InterruptConfig 01261 * @param Struct to empty with configuration values 01262 * @retval S Error code[LPS22HB_ERROR, LPS22HB_OK] 01263 */ 01264 LPS22HB_Error_et LPS22HB_Get_InterruptConfig(void *handle, LPS22HB_InterruptTypeDef_st* pLPS22HBInt); 01265 01266 /** 01267 * @brief Set Fifo configuration 01268 * @param Struct holding the configuration values 01269 * @retval Error code[LPS22HB_ERROR, LPS22HB_OK] 01270 */ 01271 LPS22HB_Error_et LPS22HB_Set_FifoConfig(void *handle, LPS22HB_FIFOTypeDef_st* pLPS22HBFIFO); 01272 01273 /** 01274 * @brief Get Fifo configuration 01275 * @param Struct to empty with the configuration values 01276 * @retval Error code[LPS22HB_ERROR, LPS22HB_OK] 01277 */ 01278 LPS22HB_Error_et LPS22HB_Get_FifoConfig(void *handle, LPS22HB_FIFOTypeDef_st* pLPS22HBFIFO); 01279 01280 /** 01281 * @brief Clock Tree Confoguration 01282 * @param LPS22HB_CTE_NotBalanced, LPS22HB_CTE_ABalanced 01283 * @retval Error Code [LPS22HB_ERROR, LPS22HB_OK] 01284 */ 01285 LPS22HB_Error_et LPS22HB_Set_ClockTreeConfifuration(void *handle, LPS22HB_CTE_et mode); 01286 01287 /** 01288 * @} 01289 */ 01290 01291 /** 01292 * @} 01293 */ 01294 01295 /** 01296 * @} 01297 */ 01298 01299 #ifdef __cplusplus 01300 } 01301 #endif 01302 01303 #endif /* __LPS22HB_DRIVER__H */ 01304 01305 /******************* (C) COPYRIGHT 2013 STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 22:34:14 by
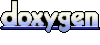