
Workshop example
Dependencies: X_NUCLEO_COMMON ST_INTERFACES
HTS221_driver.c
00001 /** 00002 ****************************************************************************** 00003 * @file HTS221_driver.c 00004 * @author HESA Application Team 00005 * @version V1.1 00006 * @date 10-August-2016 00007 * @brief HTS221 driver file 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Includes ------------------------------------------------------------------*/ 00039 #include "HTS221_driver.h" 00040 00041 #ifdef __cplusplus 00042 extern "C" { 00043 #endif 00044 00045 #ifdef USE_FULL_ASSERT_HTS221 00046 #include <stdio.h> 00047 #endif 00048 00049 00050 /** @addtogroup Environmental_Sensor 00051 * @{ 00052 */ 00053 00054 /** @defgroup HTS221_DRIVER 00055 * @brief HTS221 DRIVER 00056 * @{ 00057 */ 00058 00059 /** @defgroup HTS221_Imported_Function_Prototypes 00060 * @{ 00061 */ 00062 00063 extern uint8_t HTS221_io_write( void *handle, uint8_t WriteAddr, uint8_t *pBuffer, uint16_t nBytesToWrite ); 00064 extern uint8_t HTS221_io_read( void *handle, uint8_t ReadAddr, uint8_t *pBuffer, uint16_t nBytesToRead ); 00065 00066 /** 00067 * @} 00068 */ 00069 00070 /** @defgroup HTS221_Private_Function_Prototypes 00071 * @{ 00072 */ 00073 00074 /** 00075 * @} 00076 */ 00077 00078 /** @defgroup HTS221_Private_Functions 00079 * @{ 00080 */ 00081 00082 /** 00083 * @} 00084 */ 00085 00086 /** @defgroup HTS221_Public_Functions 00087 * @{ 00088 */ 00089 00090 /******************************************************************************* 00091 * Function Name : HTS221_read_reg 00092 * Description : Generic Reading function. It must be fullfilled with either 00093 * : I2C or SPI reading functions 00094 * Input : Register Address 00095 * Output : Data Read 00096 * Return : None 00097 *******************************************************************************/ 00098 HTS221_Error_et HTS221_read_reg( void *handle, uint8_t RegAddr, uint16_t NumByteToRead, uint8_t *Data ) 00099 { 00100 00101 if ( NumByteToRead > 1 ) RegAddr |= 0x80; 00102 00103 if ( HTS221_io_read( handle, RegAddr, Data, NumByteToRead ) ) 00104 return HTS221_ERROR; 00105 else 00106 return HTS221_OK; 00107 } 00108 00109 /******************************************************************************* 00110 * Function Name : HTS221_write_reg 00111 * Description : Generic Writing function. It must be fullfilled with either 00112 * : I2C or SPI writing function 00113 * Input : Register Address, Data to be written 00114 * Output : None 00115 * Return : None 00116 *******************************************************************************/ 00117 HTS221_Error_et HTS221_write_reg( void *handle, uint8_t RegAddr, uint16_t NumByteToWrite, uint8_t *Data ) 00118 { 00119 00120 if ( NumByteToWrite > 1 ) RegAddr |= 0x80; 00121 00122 if ( HTS221_io_write( handle, RegAddr, Data, NumByteToWrite ) ) 00123 return HTS221_ERROR; 00124 else 00125 return HTS221_OK; 00126 } 00127 00128 /** 00129 * @brief Get the version of this driver. 00130 * @param pxVersion pointer to a HTS221_DriverVersion_st structure that contains the version information. 00131 * This parameter is a pointer to @ref HTS221_DriverVersion_st. 00132 * @retval Error code [HTS221_OK, HTS221_ERROR]. 00133 */ 00134 HTS221_Error_et HTS221_Get_DriverVersion(HTS221_DriverVersion_st* version) 00135 { 00136 version->Major = HTS221_DRIVER_VERSION_MAJOR; 00137 version->Minor = HTS221_DRIVER_VERSION_MINOR; 00138 version->Point = HTS221_DRIVER_VERSION_POINT; 00139 00140 return HTS221_OK; 00141 } 00142 00143 /** 00144 * @brief Get device type ID. 00145 * @param *handle Device handle. 00146 * @param deviceid pointer to the returned device type ID. 00147 * @retval Error code [HTS221_OK, HTS221_ERROR]. 00148 */ 00149 HTS221_Error_et HTS221_Get_DeviceID(void *handle, uint8_t* deviceid) 00150 { 00151 if(HTS221_read_reg(handle, HTS221_WHO_AM_I_REG, 1, deviceid)) 00152 return HTS221_ERROR; 00153 00154 return HTS221_OK; 00155 } 00156 00157 /** 00158 * @brief Initializes the HTS221 with the specified parameters in HTS221_Init_st struct. 00159 * @param *handle Device handle. 00160 * @param pxInit pointer to a HTS221_Init_st structure that contains the configuration. 00161 * This parameter is a pointer to @ref HTS221_Init_st. 00162 * @retval Error code [HTS221_OK, HTS221_ERROR]. 00163 */ 00164 HTS221_Error_et HTS221_Set_InitConfig(void *handle, HTS221_Init_st* pxInit) 00165 { 00166 uint8_t buffer[3]; 00167 00168 HTS221_assert_param(IS_HTS221_AVGH(pxInit->avg_h )); 00169 HTS221_assert_param(IS_HTS221_AVGT(pxInit->avg_t )); 00170 HTS221_assert_param(IS_HTS221_ODR(pxInit->odr )); 00171 HTS221_assert_param(IS_HTS221_State(pxInit->bdu_status )); 00172 HTS221_assert_param(IS_HTS221_State(pxInit->heater_status )); 00173 00174 HTS221_assert_param(IS_HTS221_DrdyLevelType(pxInit->irq_level )); 00175 HTS221_assert_param(IS_HTS221_OutputType(pxInit->irq_output_type )); 00176 HTS221_assert_param(IS_HTS221_State(pxInit->irq_enable )); 00177 00178 if(HTS221_read_reg(handle, HTS221_AV_CONF_REG, 1, buffer)) 00179 return HTS221_ERROR; 00180 00181 buffer[0] &= ~(HTS221_AVGH_MASK | HTS221_AVGT_MASK); 00182 buffer[0] |= (uint8_t)pxInit->avg_h ; 00183 buffer[0] |= (uint8_t)pxInit->avg_t ; 00184 00185 if(HTS221_write_reg(handle, HTS221_AV_CONF_REG, 1, buffer)) 00186 return HTS221_ERROR; 00187 00188 if(HTS221_read_reg(handle, HTS221_CTRL_REG1, 3, buffer)) 00189 return HTS221_ERROR; 00190 00191 buffer[0] &= ~(HTS221_BDU_MASK | HTS221_ODR_MASK); 00192 buffer[0] |= (uint8_t)pxInit->odr ; 00193 buffer[0] |= ((uint8_t)pxInit->bdu_status ) << HTS221_BDU_BIT; 00194 00195 buffer[1] &= ~HTS221_HEATHER_BIT; 00196 buffer[1] |= ((uint8_t)pxInit->heater_status ) << HTS221_HEATHER_BIT; 00197 00198 buffer[2] &= ~(HTS221_DRDY_H_L_MASK | HTS221_PP_OD_MASK | HTS221_DRDY_MASK); 00199 buffer[2] |= ((uint8_t)pxInit->irq_level ) << HTS221_DRDY_H_L_BIT; 00200 buffer[2] |= (uint8_t)pxInit->irq_output_type ; 00201 buffer[2] |= ((uint8_t)pxInit->irq_enable ) << HTS221_DRDY_BIT; 00202 00203 if(HTS221_write_reg(handle, HTS221_CTRL_REG1, 3, buffer)) 00204 return HTS221_ERROR; 00205 00206 return HTS221_OK; 00207 } 00208 00209 /** 00210 * @brief Returns a HTS221_Init_st struct with the actual configuration. 00211 * @param *handle Device handle. 00212 * @param pxInit pointer to a HTS221_Init_st structure. 00213 * This parameter is a pointer to @ref HTS221_Init_st. 00214 * @retval Error code [HTS221_OK, HTS221_ERROR]. 00215 */ 00216 HTS221_Error_et HTS221_Get_InitConfig(void *handle, HTS221_Init_st* pxInit) 00217 { 00218 uint8_t buffer[3]; 00219 00220 if(HTS221_read_reg(handle, HTS221_AV_CONF_REG, 1, buffer)) 00221 return HTS221_ERROR; 00222 00223 pxInit->avg_h = (HTS221_Avgh_et)(buffer[0] & HTS221_AVGH_MASK); 00224 pxInit->avg_t = (HTS221_Avgt_et)(buffer[0] & HTS221_AVGT_MASK); 00225 00226 if(HTS221_read_reg(handle, HTS221_CTRL_REG1, 3, buffer)) 00227 return HTS221_ERROR; 00228 00229 pxInit->odr = (HTS221_Odr_et)(buffer[0] & HTS221_ODR_MASK); 00230 pxInit->bdu_status = (HTS221_State_et)((buffer[0] & HTS221_BDU_MASK) >> HTS221_BDU_BIT); 00231 pxInit->heater_status = (HTS221_State_et)((buffer[1] & HTS221_HEATHER_MASK) >> HTS221_HEATHER_BIT); 00232 00233 pxInit->irq_level = (HTS221_DrdyLevel_et)(buffer[2] & HTS221_DRDY_H_L_MASK); 00234 pxInit->irq_output_type = (HTS221_OutputType_et)(buffer[2] & HTS221_PP_OD_MASK); 00235 pxInit->irq_enable = (HTS221_State_et)((buffer[2] & HTS221_DRDY_MASK) >> HTS221_DRDY_BIT); 00236 00237 return HTS221_OK; 00238 } 00239 00240 /** 00241 * @brief De initialization function for HTS221. 00242 * This function put the HTS221 in power down, make a memory boot and clear the data output flags. 00243 * @param *handle Device handle. 00244 * @retval Error code [HTS221_OK, HTS221_ERROR]. 00245 */ 00246 HTS221_Error_et HTS221_DeInit(void *handle) 00247 { 00248 uint8_t buffer[4]; 00249 00250 if(HTS221_read_reg(handle, HTS221_CTRL_REG1, 2, buffer)) 00251 return HTS221_ERROR; 00252 00253 /* HTS221 in power down */ 00254 buffer[0] |= 0x01 << HTS221_PD_BIT; 00255 00256 /* Make HTS221 boot */ 00257 buffer[1] |= 0x01 << HTS221_BOOT_BIT; 00258 00259 if(HTS221_write_reg(handle, HTS221_CTRL_REG1, 2, buffer)) 00260 return HTS221_ERROR; 00261 00262 /* Dump of data output */ 00263 if(HTS221_read_reg(handle, HTS221_HR_OUT_L_REG, 4, buffer)) 00264 return HTS221_ERROR; 00265 00266 return HTS221_OK; 00267 } 00268 00269 /** 00270 * @brief Read HTS221 output registers, and calculate humidity and temperature. 00271 * @param *handle Device handle. 00272 * @param humidity pointer to the returned humidity value that must be divided by 10 to get the value in [%]. 00273 * @param temperature pointer to the returned temperature value that must be divided by 10 to get the value in ['C]. 00274 * @retval Error code [HTS221_OK, HTS221_ERROR]. 00275 */ 00276 HTS221_Error_et HTS221_Get_Measurement(void *handle, uint16_t* humidity, int16_t* temperature) 00277 { 00278 if ( HTS221_Get_Temperature( handle, temperature ) == HTS221_ERROR ) return HTS221_ERROR; 00279 if ( HTS221_Get_Humidity( handle, humidity ) == HTS221_ERROR ) return HTS221_ERROR; 00280 00281 return HTS221_OK; 00282 } 00283 00284 /** 00285 * @brief Read HTS221 output registers. Humidity and temperature. 00286 * @param *handle Device handle. 00287 * @param humidity pointer to the returned humidity raw value. 00288 * @param temperature pointer to the returned temperature raw value. 00289 * @retval Error code [HTS221_OK, HTS221_ERROR]. 00290 */ 00291 HTS221_Error_et HTS221_Get_RawMeasurement(void *handle, int16_t* humidity, int16_t* temperature) 00292 { 00293 uint8_t buffer[4]; 00294 00295 if(HTS221_read_reg(handle, HTS221_HR_OUT_L_REG, 4, buffer)) 00296 return HTS221_ERROR; 00297 00298 *humidity = (int16_t)((((uint16_t)buffer[1]) << 8) | (uint16_t)buffer[0]); 00299 *temperature = (int16_t)((((uint16_t)buffer[3]) << 8) | (uint16_t)buffer[2]); 00300 00301 return HTS221_OK; 00302 } 00303 00304 /** 00305 * @brief Read HTS221 Humidity output registers, and calculate humidity. 00306 * @param *handle Device handle. 00307 * @param Pointer to the returned humidity value that must be divided by 10 to get the value in [%]. 00308 * @retval Error code [HTS221_OK, HTS221_ERROR]. 00309 */ 00310 HTS221_Error_et HTS221_Get_Humidity(void *handle, uint16_t* value) 00311 { 00312 int16_t H0_T0_out, H1_T0_out, H_T_out; 00313 int16_t H0_rh, H1_rh; 00314 uint8_t buffer[2]; 00315 float tmp_f; 00316 00317 if(HTS221_read_reg(handle, HTS221_H0_RH_X2, 2, buffer)) 00318 return HTS221_ERROR; 00319 H0_rh = buffer[0] >> 1; 00320 H1_rh = buffer[1] >> 1; 00321 00322 if(HTS221_read_reg(handle, HTS221_H0_T0_OUT_L, 2, buffer)) 00323 return HTS221_ERROR; 00324 H0_T0_out = (((uint16_t)buffer[1]) << 8) | (uint16_t)buffer[0]; 00325 00326 if(HTS221_read_reg(handle, HTS221_H1_T0_OUT_L, 2, buffer)) 00327 return HTS221_ERROR; 00328 H1_T0_out = (((uint16_t)buffer[1]) << 8) | (uint16_t)buffer[0]; 00329 00330 if(HTS221_read_reg(handle, HTS221_HR_OUT_L_REG, 2, buffer)) 00331 return HTS221_ERROR; 00332 H_T_out = (((uint16_t)buffer[1]) << 8) | (uint16_t)buffer[0]; 00333 00334 tmp_f = (float)(H_T_out - H0_T0_out) * (float)(H1_rh - H0_rh) / (float)(H1_T0_out - H0_T0_out) + H0_rh; 00335 tmp_f *= 10.0f; 00336 00337 *value = ( tmp_f > 1000.0f ) ? 1000 00338 : ( tmp_f < 0.0f ) ? 0 00339 : ( uint16_t )tmp_f; 00340 00341 return HTS221_OK; 00342 } 00343 00344 /** 00345 * @brief Read HTS221 humidity output registers. 00346 * @param *handle Device handle. 00347 * @param Pointer to the returned humidity raw value. 00348 * @retval Error code [HTS221_OK, HTS221_ERROR]. 00349 */ 00350 HTS221_Error_et HTS221_Get_HumidityRaw(void *handle, int16_t* value) 00351 { 00352 uint8_t buffer[2]; 00353 00354 if(HTS221_read_reg(handle, HTS221_HR_OUT_L_REG, 2, buffer)) 00355 return HTS221_ERROR; 00356 00357 *value = (int16_t)((((uint16_t)buffer[1]) << 8) | (uint16_t)buffer[0]); 00358 00359 return HTS221_OK; 00360 } 00361 00362 /** 00363 * @brief Read HTS221 temperature output registers, and calculate temperature. 00364 * @param *handle Device handle. 00365 * @param Pointer to the returned temperature value that must be divided by 10 to get the value in ['C]. 00366 * @retval Error code [HTS221_OK, HTS221_ERROR]. 00367 */ 00368 HTS221_Error_et HTS221_Get_Temperature(void *handle, int16_t *value) 00369 { 00370 int16_t T0_out, T1_out, T_out, T0_degC_x8_u16, T1_degC_x8_u16; 00371 int16_t T0_degC, T1_degC; 00372 uint8_t buffer[4], tmp; 00373 float tmp_f; 00374 00375 if(HTS221_read_reg(handle, HTS221_T0_DEGC_X8, 2, buffer)) 00376 return HTS221_ERROR; 00377 if(HTS221_read_reg(handle, HTS221_T0_T1_DEGC_H2, 1, &tmp)) 00378 return HTS221_ERROR; 00379 00380 T0_degC_x8_u16 = (((uint16_t)(tmp & 0x03)) << 8) | ((uint16_t)buffer[0]); 00381 T1_degC_x8_u16 = (((uint16_t)(tmp & 0x0C)) << 6) | ((uint16_t)buffer[1]); 00382 T0_degC = T0_degC_x8_u16 >> 3; 00383 T1_degC = T1_degC_x8_u16 >> 3; 00384 00385 if(HTS221_read_reg(handle, HTS221_T0_OUT_L, 4, buffer)) 00386 return HTS221_ERROR; 00387 00388 T0_out = (((uint16_t)buffer[1]) << 8) | (uint16_t)buffer[0]; 00389 T1_out = (((uint16_t)buffer[3]) << 8) | (uint16_t)buffer[2]; 00390 00391 if(HTS221_read_reg(handle, HTS221_TEMP_OUT_L_REG, 2, buffer)) 00392 return HTS221_ERROR; 00393 00394 T_out = (((uint16_t)buffer[1]) << 8) | (uint16_t)buffer[0]; 00395 00396 tmp_f = (float)(T_out - T0_out) * (float)(T1_degC - T0_degC) / (float)(T1_out - T0_out) + T0_degC; 00397 tmp_f *= 10.0f; 00398 00399 *value = ( int16_t )tmp_f; 00400 00401 return HTS221_OK; 00402 } 00403 00404 /** 00405 * @brief Read HTS221 temperature output registers. 00406 * @param *handle Device handle. 00407 * @param Pointer to the returned temperature raw value. 00408 * @retval Error code [HTS221_OK, HTS221_ERROR]. 00409 */ 00410 HTS221_Error_et HTS221_Get_TemperatureRaw(void *handle, int16_t* value) 00411 { 00412 uint8_t buffer[2]; 00413 00414 if(HTS221_read_reg(handle, HTS221_TEMP_OUT_L_REG, 2, buffer)) 00415 return HTS221_ERROR; 00416 00417 *value = (int16_t)((((uint16_t)buffer[1]) << 8) | (uint16_t)buffer[0]); 00418 00419 return HTS221_OK; 00420 } 00421 00422 /** 00423 * @brief Get the availability of new data for humidity and temperature. 00424 * @param *handle Device handle. 00425 * @param humidity pointer to the returned humidity data status [HTS221_SET/HTS221_RESET]. 00426 * @param temperature pointer to the returned temperature data status [HTS221_SET/HTS221_RESET]. 00427 * This parameter is a pointer to @ref HTS221_BitStatus_et. 00428 * @retval Error code [HTS221_OK, HTS221_ERROR]. 00429 */ 00430 HTS221_Error_et HTS221_Get_DataStatus(void *handle, HTS221_BitStatus_et* humidity, HTS221_BitStatus_et* temperature) 00431 { 00432 uint8_t tmp; 00433 00434 if(HTS221_read_reg(handle, HTS221_STATUS_REG, 1, &tmp)) 00435 return HTS221_ERROR; 00436 00437 *humidity = (HTS221_BitStatus_et)((tmp & HTS221_HDA_MASK) >> HTS221_H_DA_BIT); 00438 *temperature = (HTS221_BitStatus_et)(tmp & HTS221_TDA_MASK); 00439 00440 return HTS221_OK; 00441 } 00442 00443 /** 00444 * @brief Exit from power down mode. 00445 * @param *handle Device handle. 00446 * @param void. 00447 * @retval Error code [HTS221_OK, HTS221_ERROR]. 00448 */ 00449 HTS221_Error_et HTS221_Activate(void *handle) 00450 { 00451 uint8_t tmp; 00452 00453 if(HTS221_read_reg(handle, HTS221_CTRL_REG1, 1, &tmp)) 00454 return HTS221_ERROR; 00455 00456 tmp |= HTS221_PD_MASK; 00457 00458 if(HTS221_write_reg(handle, HTS221_CTRL_REG1, 1, &tmp)) 00459 return HTS221_ERROR; 00460 00461 return HTS221_OK; 00462 } 00463 00464 /** 00465 * @brief Put the sensor in power down mode. 00466 * @param *handle Device handle. 00467 * @retval Error code [HTS221_OK, HTS221_ERROR]. 00468 */ 00469 HTS221_Error_et HTS221_DeActivate(void *handle) 00470 { 00471 uint8_t tmp; 00472 00473 if(HTS221_read_reg(handle, HTS221_CTRL_REG1, 1, &tmp)) 00474 return HTS221_ERROR; 00475 00476 tmp &= ~HTS221_PD_MASK; 00477 00478 if(HTS221_write_reg(handle, HTS221_CTRL_REG1, 1, &tmp)) 00479 return HTS221_ERROR; 00480 00481 return HTS221_OK; 00482 } 00483 00484 00485 00486 /** 00487 * @brief Check if the single measurement has completed. 00488 * @param *handle Device handle. 00489 * @param tmp is set to 1, when the measure is completed 00490 * @retval Status [HTS221_ERROR, HTS221_OK] 00491 */ 00492 HTS221_Error_et HTS221_IsMeasurementCompleted(void *handle, HTS221_BitStatus_et* Is_Measurement_Completed) 00493 { 00494 uint8_t tmp; 00495 00496 if(HTS221_read_reg(handle, HTS221_STATUS_REG, 1, &tmp)) 00497 return HTS221_ERROR; 00498 00499 if((tmp & (uint8_t)(HTS221_HDA_MASK | HTS221_TDA_MASK)) == (uint8_t)(HTS221_HDA_MASK | HTS221_TDA_MASK)) 00500 *Is_Measurement_Completed = HTS221_SET; 00501 else 00502 *Is_Measurement_Completed = HTS221_RESET; 00503 00504 return HTS221_OK; 00505 } 00506 00507 00508 /** 00509 * @brief Set_ humidity and temperature average mode. 00510 * @param *handle Device handle. 00511 * @param avgh is the average mode for humidity, this parameter is @ref HTS221_Avgh_et. 00512 * @param avgt is the average mode for temperature, this parameter is @ref HTS221_Avgt_et. 00513 * @retval Error code [HTS221_OK, HTS221_ERROR]. 00514 */ 00515 HTS221_Error_et HTS221_Set_AvgHT(void *handle, HTS221_Avgh_et avgh, HTS221_Avgt_et avgt) 00516 { 00517 uint8_t tmp; 00518 00519 HTS221_assert_param(IS_HTS221_AVGH(avgh)); 00520 HTS221_assert_param(IS_HTS221_AVGT(avgt)); 00521 00522 if(HTS221_read_reg(handle, HTS221_AV_CONF_REG, 1, &tmp)) 00523 return HTS221_ERROR; 00524 00525 tmp &= ~(HTS221_AVGH_MASK | HTS221_AVGT_MASK); 00526 tmp |= (uint8_t)avgh; 00527 tmp |= (uint8_t)avgt; 00528 00529 if(HTS221_write_reg(handle, HTS221_AV_CONF_REG, 1, &tmp)) 00530 return HTS221_ERROR; 00531 00532 return HTS221_OK; 00533 } 00534 00535 /** 00536 * @brief Set humidity average mode. 00537 * @param *handle Device handle. 00538 * @param avgh is the average mode for humidity, this parameter is @ref HTS221_Avgh_et. 00539 * @retval Error code [HTS221_OK, HTS221_ERROR]. 00540 */ 00541 HTS221_Error_et HTS221_Set_AvgH(void *handle, HTS221_Avgh_et avgh) 00542 { 00543 uint8_t tmp; 00544 00545 HTS221_assert_param(IS_HTS221_AVGH(avgh)); 00546 00547 if(HTS221_read_reg(handle, HTS221_AV_CONF_REG, 1, &tmp)) 00548 return HTS221_ERROR; 00549 00550 tmp &= ~HTS221_AVGH_MASK; 00551 tmp |= (uint8_t)avgh; 00552 00553 if(HTS221_write_reg(handle, HTS221_AV_CONF_REG, 1, &tmp)) 00554 return HTS221_ERROR; 00555 00556 return HTS221_OK; 00557 } 00558 00559 /** 00560 * @brief Set temperature average mode. 00561 * @param *handle Device handle. 00562 * @param avgt is the average mode for temperature, this parameter is @ref HTS221_Avgt_et. 00563 * @retval Error code [HTS221_OK, HTS221_ERROR]. 00564 */ 00565 HTS221_Error_et HTS221_Set_AvgT(void *handle, HTS221_Avgt_et avgt) 00566 { 00567 uint8_t tmp; 00568 00569 HTS221_assert_param(IS_HTS221_AVGT(avgt)); 00570 00571 if(HTS221_read_reg(handle, HTS221_AV_CONF_REG, 1, &tmp)) 00572 return HTS221_ERROR; 00573 00574 tmp &= ~HTS221_AVGT_MASK; 00575 tmp |= (uint8_t)avgt; 00576 00577 if(HTS221_write_reg(handle, HTS221_AV_CONF_REG, 1, &tmp)) 00578 return HTS221_ERROR; 00579 00580 return HTS221_OK; 00581 } 00582 00583 /** 00584 * @brief Get humidity and temperature average mode. 00585 * @param *handle Device handle. 00586 * @param avgh pointer to the returned value with the humidity average mode. 00587 * @param avgt pointer to the returned value with the temperature average mode. 00588 * @retval Error code [HTS221_OK, HTS221_ERROR]. 00589 */ 00590 HTS221_Error_et HTS221_Get_AvgHT(void *handle, HTS221_Avgh_et* avgh, HTS221_Avgt_et* avgt) 00591 { 00592 uint8_t tmp; 00593 00594 if(HTS221_read_reg(handle, HTS221_AV_CONF_REG, 1, &tmp)) 00595 return HTS221_ERROR; 00596 00597 *avgh = (HTS221_Avgh_et)(tmp & HTS221_AVGH_MASK); 00598 *avgt = (HTS221_Avgt_et)(tmp & HTS221_AVGT_MASK); 00599 00600 return HTS221_OK; 00601 } 00602 00603 /** 00604 * @brief Set block data update mode. 00605 * @param *handle Device handle. 00606 * @param status can be HTS221_ENABLE: enable the block data update, output data registers are updated once both MSB and LSB are read. 00607 * @param status can be HTS221_DISABLE: output data registers are continuously updated. 00608 * This parameter is a @ref HTS221_BitStatus_et. 00609 * @retval Error code [HTS221_OK, HTS221_ERROR]. 00610 */ 00611 HTS221_Error_et HTS221_Set_BduMode(void *handle, HTS221_State_et status) 00612 { 00613 uint8_t tmp; 00614 00615 HTS221_assert_param(IS_HTS221_State(status)); 00616 00617 if(HTS221_read_reg(handle, HTS221_CTRL_REG1, 1, &tmp)) 00618 return HTS221_ERROR; 00619 00620 tmp &= ~HTS221_BDU_MASK; 00621 tmp |= ((uint8_t)status) << HTS221_BDU_BIT; 00622 00623 if(HTS221_write_reg(handle, HTS221_CTRL_REG1, 1, &tmp)) 00624 return HTS221_ERROR; 00625 00626 return HTS221_OK; 00627 } 00628 00629 /** 00630 * @brief Get block data update mode. 00631 * @param *handle Device handle. 00632 * @param Pointer to the returned value with block data update mode status. 00633 * @retval Error code [HTS221_OK, HTS221_ERROR]. 00634 */ 00635 HTS221_Error_et HTS221_Get_BduMode(void *handle, HTS221_State_et* status) 00636 { 00637 uint8_t tmp; 00638 00639 if(HTS221_read_reg(handle, HTS221_CTRL_REG1, 1, &tmp)) 00640 return HTS221_ERROR; 00641 00642 *status = (HTS221_State_et)((tmp & HTS221_BDU_MASK) >> HTS221_BDU_BIT); 00643 00644 return HTS221_OK; 00645 } 00646 00647 /** 00648 * @brief Enter or exit from power down mode. 00649 * @param *handle Device handle. 00650 * @param status can be HTS221_SET: HTS221 in power down mode. 00651 * @param status can be HTS221_REET: HTS221 in active mode. 00652 * This parameter is a @ref HTS221_BitStatus_et. 00653 * @retval Error code [HTS221_OK, HTS221_ERROR]. 00654 */ 00655 HTS221_Error_et HTS221_Set_PowerDownMode(void *handle, HTS221_BitStatus_et status) 00656 { 00657 uint8_t tmp; 00658 00659 HTS221_assert_param(IS_HTS221_BitStatus(status)); 00660 00661 if(HTS221_read_reg(handle, HTS221_CTRL_REG1, 1, &tmp)) 00662 return HTS221_ERROR; 00663 00664 tmp &= ~HTS221_PD_MASK; 00665 tmp |= ((uint8_t)status) << HTS221_PD_BIT; 00666 00667 if(HTS221_write_reg(handle, HTS221_CTRL_REG1, 1, &tmp)) 00668 return HTS221_ERROR; 00669 00670 return HTS221_OK; 00671 } 00672 00673 /** 00674 * @brief Get if HTS221 is in active mode or in power down mode. 00675 * @param *handle Device handle. 00676 * @param Pointer to the returned value with HTS221 status. 00677 * @retval Error code [HTS221_OK, HTS221_ERROR]. 00678 */ 00679 HTS221_Error_et HTS221_Get_PowerDownMode(void *handle, HTS221_BitStatus_et* status) 00680 { 00681 uint8_t tmp; 00682 00683 if(HTS221_read_reg(handle, HTS221_CTRL_REG1, 1, &tmp)) 00684 return HTS221_ERROR; 00685 00686 *status = (HTS221_BitStatus_et)((tmp & HTS221_PD_MASK) >> HTS221_PD_BIT); 00687 00688 return HTS221_OK; 00689 } 00690 00691 /** 00692 * @brief Set the output data rate mode. 00693 * @param *handle Device handle. 00694 * @param odr is the output data rate mode. 00695 * This parameter is a @ref HTS221_Odr_et. 00696 * @retval Error code [HTS221_OK, HTS221_ERROR]. 00697 */ 00698 HTS221_Error_et HTS221_Set_Odr(void *handle, HTS221_Odr_et odr) 00699 { 00700 uint8_t tmp; 00701 00702 HTS221_assert_param(IS_HTS221_ODR(odr)); 00703 00704 if(HTS221_read_reg(handle, HTS221_CTRL_REG1, 1, &tmp)) 00705 return HTS221_ERROR; 00706 00707 tmp &= ~HTS221_ODR_MASK; 00708 tmp |= (uint8_t)odr; 00709 00710 if(HTS221_write_reg(handle, HTS221_CTRL_REG1, 1, &tmp)) 00711 return HTS221_ERROR; 00712 00713 return HTS221_OK; 00714 } 00715 00716 /** 00717 * @brief Get the output data rate mode. 00718 * @param *handle Device handle. 00719 * @param Pointer to the returned value with output data rate mode. 00720 * @retval Error code [HTS221_OK, HTS221_ERROR]. 00721 */ 00722 HTS221_Error_et HTS221_Get_Odr(void *handle, HTS221_Odr_et* odr) 00723 { 00724 uint8_t tmp; 00725 00726 if(HTS221_read_reg(handle, HTS221_CTRL_REG1, 1, &tmp)) 00727 return HTS221_ERROR; 00728 00729 tmp &= HTS221_ODR_MASK; 00730 *odr = (HTS221_Odr_et)tmp; 00731 00732 return HTS221_OK; 00733 } 00734 00735 /** 00736 * @brief Reboot Memory Content. 00737 * @param *handle Device handle. 00738 * @retval Error code [HTS221_OK, HTS221_ERROR]. 00739 */ 00740 HTS221_Error_et HTS221_MemoryBoot(void *handle) 00741 { 00742 uint8_t tmp; 00743 00744 if(HTS221_read_reg(handle, HTS221_CTRL_REG2, 1, &tmp)) 00745 return HTS221_ERROR; 00746 00747 tmp |= HTS221_BOOT_MASK; 00748 00749 if(HTS221_write_reg(handle, HTS221_CTRL_REG2, 1, &tmp)) 00750 return HTS221_ERROR; 00751 00752 return HTS221_OK; 00753 } 00754 00755 /** 00756 * @brief Configure the internal heater. 00757 * @param *handle Device handle. 00758 * @param The status of the internal heater [HTS221_ENABLE/HTS221_DISABLE]. 00759 * This parameter is a @ref HTS221_State_et. 00760 * @retval Error code [HTS221_OK, HTS221_ERROR] 00761 */ 00762 HTS221_Error_et HTS221_Set_HeaterState(void *handle, HTS221_State_et status) 00763 { 00764 uint8_t tmp; 00765 00766 HTS221_assert_param(IS_HTS221_State(status)); 00767 00768 if(HTS221_read_reg(handle, HTS221_CTRL_REG2, 1, &tmp)) 00769 return HTS221_ERROR; 00770 00771 tmp &= ~HTS221_HEATHER_MASK; 00772 tmp |= ((uint8_t)status) << HTS221_HEATHER_BIT; 00773 00774 if(HTS221_write_reg(handle, HTS221_CTRL_REG2, 1, &tmp)) 00775 return HTS221_ERROR; 00776 00777 return HTS221_OK; 00778 } 00779 00780 /** 00781 * @brief Get the internal heater. 00782 * @param *handle Device handle. 00783 * @param Pointer to the returned status of the internal heater [HTS221_ENABLE/HTS221_DISABLE]. 00784 * @retval Error code [HTS221_OK, HTS221_ERROR]. 00785 */ 00786 HTS221_Error_et HTS221_Get_HeaterState(void *handle, HTS221_State_et* status) 00787 { 00788 uint8_t tmp; 00789 00790 if(HTS221_read_reg(handle, HTS221_CTRL_REG2, 1, &tmp)) 00791 return HTS221_ERROR; 00792 00793 *status = (HTS221_State_et)((tmp & HTS221_HEATHER_MASK) >> HTS221_HEATHER_BIT); 00794 00795 return HTS221_OK; 00796 } 00797 00798 /** 00799 * @brief Set ONE_SHOT bit to start a new conversion (ODR mode has to be 00). 00800 * Once the measurement is done, ONE_SHOT bit is self-cleared. 00801 * @param *handle Device handle. 00802 * @retval Error code [HTS221_OK, HTS221_ERROR]. 00803 */ 00804 HTS221_Error_et HTS221_StartOneShotMeasurement(void *handle) 00805 { 00806 uint8_t tmp; 00807 00808 if(HTS221_read_reg(handle, HTS221_CTRL_REG2, 1, &tmp)) 00809 return HTS221_ERROR; 00810 00811 tmp |= HTS221_ONE_SHOT_MASK; 00812 00813 if(HTS221_write_reg(handle, HTS221_CTRL_REG2, 1, &tmp)) 00814 return HTS221_ERROR; 00815 00816 return HTS221_OK; 00817 00818 } 00819 00820 /** 00821 * @brief Set level configuration of the interrupt pin DRDY. 00822 * @param *handle Device handle. 00823 * @param status can be HTS221_LOW_LVL: active level is LOW. 00824 * @param status can be HTS221_HIGH_LVL: active level is HIGH. 00825 * This parameter is a @ref HTS221_State_et. 00826 * @retval Error code [HTS221_OK, HTS221_ERROR]. 00827 */ 00828 HTS221_Error_et HTS221_Set_IrqActiveLevel(void *handle, HTS221_DrdyLevel_et value) 00829 { 00830 uint8_t tmp; 00831 00832 HTS221_assert_param(IS_HTS221_DrdyLevelType(value)); 00833 00834 if(HTS221_read_reg(handle, HTS221_CTRL_REG3, 1, &tmp)) 00835 return HTS221_ERROR; 00836 00837 tmp &= ~HTS221_DRDY_H_L_MASK; 00838 tmp |= (uint8_t)value; 00839 00840 if(HTS221_write_reg(handle, HTS221_CTRL_REG3, 1, &tmp)) 00841 return HTS221_ERROR; 00842 00843 return HTS221_OK; 00844 } 00845 00846 /** 00847 * @brief Get level configuration of the interrupt pin DRDY. 00848 * @param *handle Device handle. 00849 * @param Pointer to the returned status of the level configuration [HTS221_ENABLE/HTS221_DISABLE]. 00850 * @retval Error code [HTS221_OK, HTS221_ERROR]. 00851 */ 00852 HTS221_Error_et HTS221_Get_IrqActiveLevel(void *handle, HTS221_DrdyLevel_et* value) 00853 { 00854 uint8_t tmp; 00855 00856 if(HTS221_read_reg(handle, HTS221_CTRL_REG3, 1, &tmp)) 00857 return HTS221_ERROR; 00858 00859 *value = (HTS221_DrdyLevel_et)(tmp & HTS221_DRDY_H_L_MASK); 00860 00861 return HTS221_OK; 00862 } 00863 00864 /** 00865 * @brief Set Push-pull/open drain configuration for the interrupt pin DRDY. 00866 * @param *handle Device handle. 00867 * @param value is the output type configuration. 00868 * This parameter is a @ref HTS221_OutputType_et. 00869 * @retval Error code [HTS221_OK, HTS221_ERROR]. 00870 */ 00871 HTS221_Error_et HTS221_Set_IrqOutputType(void *handle, HTS221_OutputType_et value) 00872 { 00873 uint8_t tmp; 00874 00875 HTS221_assert_param(IS_HTS221_OutputType(value)); 00876 00877 if(HTS221_read_reg(handle, HTS221_CTRL_REG3, 1, &tmp)) 00878 return HTS221_ERROR; 00879 00880 tmp &= ~HTS221_PP_OD_MASK; 00881 tmp |= (uint8_t)value; 00882 00883 if(HTS221_write_reg(handle, HTS221_CTRL_REG3, 1, &tmp)) 00884 return HTS221_ERROR; 00885 00886 return HTS221_OK; 00887 } 00888 00889 /** 00890 * @brief Get the configuration for the interrupt pin DRDY. 00891 * @param *handle Device handle. 00892 * @param Pointer to the returned value with output type configuration. 00893 * @retval Error code [HTS221_OK, HTS221_ERROR]. 00894 */ 00895 HTS221_Error_et HTS221_Get_IrqOutputType(void *handle, HTS221_OutputType_et* value) 00896 { 00897 uint8_t tmp; 00898 00899 if(HTS221_read_reg(handle, HTS221_CTRL_REG3, 1, &tmp)) 00900 return HTS221_ERROR; 00901 00902 *value = (HTS221_OutputType_et)(tmp & HTS221_PP_OD_MASK); 00903 00904 return HTS221_OK; 00905 } 00906 00907 /** 00908 * @brief Enable/disable the interrupt mode. 00909 * @param *handle Device handle. 00910 * @param status is the enable/disable for the interrupt mode. 00911 * This parameter is a @ref HTS221_State_et. 00912 * @retval Error code [HTS221_OK, HTS221_ERROR]. 00913 */ 00914 HTS221_Error_et HTS221_Set_IrqEnable(void *handle, HTS221_State_et status) 00915 { 00916 uint8_t tmp; 00917 00918 HTS221_assert_param(IS_HTS221_State(status)); 00919 00920 if(HTS221_read_reg(handle, HTS221_CTRL_REG3, 1, &tmp)) 00921 return HTS221_ERROR; 00922 00923 tmp &= ~HTS221_DRDY_MASK; 00924 tmp |= ((uint8_t)status) << HTS221_DRDY_BIT; 00925 00926 if(HTS221_write_reg(handle, HTS221_CTRL_REG3, 1, &tmp)) 00927 return HTS221_ERROR; 00928 00929 return HTS221_OK; 00930 } 00931 00932 /** 00933 * @brief Get the interrupt mode. 00934 * @param *handle Device handle. 00935 * @param Pointer to the returned status of the interrupt mode configuration [HTS221_ENABLE/HTS221_DISABLE]. 00936 * @retval Error code [HTS221_OK, HTS221_ERROR]. 00937 */ 00938 HTS221_Error_et HTS221_Get_IrqEnable(void *handle, HTS221_State_et* status) 00939 { 00940 uint8_t tmp; 00941 00942 if(HTS221_read_reg(handle, HTS221_CTRL_REG3, 1, &tmp)) 00943 return HTS221_ERROR; 00944 00945 *status = (HTS221_State_et)((tmp & HTS221_DRDY_MASK) >> HTS221_DRDY_BIT); 00946 00947 return HTS221_OK; 00948 } 00949 00950 00951 #ifdef USE_FULL_ASSERT_HTS221 00952 /** 00953 * @brief Reports the name of the source file and the source line number 00954 * where the assert_param error has occurred. 00955 * @param file: pointer to the source file name 00956 * @param line: assert_param error line source number 00957 * @retval : None 00958 */ 00959 void HTS221_assert_failed(uint8_t* file, uint32_t line) 00960 { 00961 /* User can add his own implementation to report the file name and line number */ 00962 printf("Wrong parameters value: file %s on line %d\r\n", file, (int)line); 00963 00964 /* Infinite loop */ 00965 while (1) 00966 { 00967 } 00968 } 00969 #endif 00970 00971 #ifdef __cplusplus 00972 } 00973 #endif 00974 00975 /** 00976 * @} 00977 */ 00978 00979 /** 00980 * @} 00981 */ 00982 00983 /** 00984 * @} 00985 */ 00986 00987 /******************* (C) COPYRIGHT 2013 STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 22:34:14 by
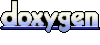