
Fork for workshops
Embed:
(wiki syntax)
Show/hide line numbers
m2mconfig.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef M2MCONFIG_H 00017 #define M2MCONFIG_H 00018 00019 /*! \file m2mconfig.h 00020 * \brief File defining all system build time configuration used by mbed-client. 00021 */ 00022 00023 #include "mbed-client/m2mstring.h" 00024 00025 #include <stddef.h> 00026 00027 using namespace m2m; 00028 00029 /** 00030 * \def MBED_CLIENT_RECONNECTION_COUNT 00031 * 00032 * \brief Sets Reconnection count for mbed Client 00033 * to attempt a reconnection re-tries until 00034 * reaches the defined value either by the application 00035 * or the default value set in Client. 00036 * By default, the value is 3. 00037 */ 00038 #undef MBED_CLIENT_RECONNECTION_COUNT /* 3 */ 00039 00040 /** 00041 * \def MBED_CLIENT_RECONNECTION_INTERVAL 00042 * 00043 * \brief Sets Reconnection interval (in seconds) for 00044 * mbed Client to attempt a reconnection re-tries. 00045 * By default, the value is 5 seconds. 00046 */ 00047 #undef MBED_CLIENT_RECONNECTION_INTERVAL /* 5 */ 00048 00049 /** 00050 * \def MBED_CLIENT_TCP_KEEPALIVE_INTERVAL 00051 * 00052 * \brief The number of seconds between CoAP ping messages. 00053 * By default, the value is 90 seconds. 00054 */ 00055 #undef MBED_CLIENT_TCP_KEEPALIVE_INTERVAL /* 90 */ 00056 00057 /** 00058 * \def MBED_CLIENT_EVENT_LOOP_SIZE 00059 * 00060 * \brief Defines the size of memory allocated for 00061 * event loop (in bytes) for timer and network event 00062 * handling of mbed Client. 00063 * By default, this value is 1024 bytes.This memory is 00064 * allocated from heap 00065 */ 00066 #undef MBED_CLIENT_EVENT_LOOP_SIZE /* 1024 */ 00067 00068 #ifdef YOTTA_CFG_RECONNECTION_COUNT 00069 #define MBED_CLIENT_RECONNECTION_COUNT YOTTA_CFG_RECONNECTION_COUNT 00070 #elif defined MBED_CONF_MBED_CLIENT_RECONNECTION_COUNT 00071 #define MBED_CLIENT_RECONNECTION_COUNT MBED_CONF_MBED_CLIENT_RECONNECTION_COUNT 00072 #endif 00073 00074 #ifdef YOTTA_CFG_RECONNECTION_INTERVAL 00075 #define MBED_CLIENT_RECONNECTION_INTERVAL YOTTA_CFG_RECONNECTION_INTERVAL 00076 #elif defined MBED_CONF_MBED_CLIENT_RECONNECTION_INTERVAL 00077 #define MBED_CLIENT_RECONNECTION_INTERVAL MBED_CONF_MBED_CLIENT_RECONNECTION_INTERVAL 00078 #endif 00079 00080 #ifdef YOTTA_CFG_TCP_KEEPALIVE_INTERVAL 00081 #define MBED_CLIENT_TCP_KEEPALIVE_INTERVAL YOTTA_CFG_TCP_KEEPALIVE_INTERVAL 00082 #elif defined MBED_CONF_MBED_CLIENT_TCP_KEEPALIVE_INTERVAL 00083 #define MBED_CLIENT_TCP_KEEPALIVE_INTERVAL MBED_CONF_MBED_CLIENT_TCP_KEEPALIVE_INTERVAL 00084 #endif 00085 00086 #ifdef YOTTA_CFG_DISABLE_BOOTSTRAP_FEATURE 00087 #define MBED_CLIENT_DISABLE_BOOTSTRAP_FEATURE YOTTA_CFG_DISABLE_BOOTSTRAP_FEATURE 00088 #elif defined MBED_CONF_MBED_CLIENT_DISABLE_BOOTSTRAP_FEATURE 00089 #define MBED_CLIENT_DISABLE_BOOTSTRAP_FEATURE MBED_CONF_MBED_CLIENT_DISABLE_BOOTSTRAP_FEATURE 00090 #endif 00091 00092 #ifdef YOTTA_CFG_COAP_MAX_INCOMING_BLOCK_MESSAGE_SIZE 00093 #define SN_COAP_MAX_INCOMING_MESSAGE_SIZE YOTTA_CFG_COAP_MAX_INCOMING_BLOCK_MESSAGE_SIZE 00094 #elif defined MBED_CONF_MBED_CLIENT_SN_COAP_MAX_INCOMING_MESSAGE_SIZE 00095 #define SN_COAP_MAX_INCOMING_MESSAGE_SIZE MBED_CONF_MBED_CLIENT_SN_COAP_MAX_INCOMING_MESSAGE_SIZE 00096 #endif 00097 00098 #ifdef MBED_CONF_MBED_CLIENT_EVENT_LOOP_SIZE 00099 #define MBED_CLIENT_EVENT_LOOP_SIZE MBED_CONF_MBED_CLIENT_EVENT_LOOP_SIZE 00100 #endif 00101 00102 #ifdef YOTTA_CFG_DISABLE_INTERFACE_DESCRIPTION 00103 #define DISABLE_INTERFACE_DESCRIPTION YOTTA_CFG_DISABLE_INTERFACE_DESCRIPTION 00104 #elif defined MBED_CONF_MBED_CLIENT_DISABLE_INTERFACE_DESCRIPTION 00105 #define DISABLE_INTERFACE_DESCRIPTION MBED_CONF_MBED_CLIENT_DISABLE_INTERFACE_DESCRIPTION 00106 #endif 00107 00108 #ifdef YOTTA_CFG_DISABLE_RESOURCE_TYPE 00109 #define DISABLE_RESOURCE_TYPE YOTTA_CFG_DISABLE_RESOURCE_TYPE 00110 #elif defined MBED_CONF_MBED_CLIENT_DISABLE_RESOURCE_TYPE 00111 #define DISABLE_RESOURCE_TYPE MBED_CONF_MBED_CLIENT_DISABLE_RESOURCE_TYPE 00112 #endif 00113 00114 #ifdef YOTTA_CFG_DISABLE_DELAYED_RESPONSE 00115 #define DISABLE_DELAYED_RESPONSE YOTTA_CFG_DISABLE_DELAYED_RESPONSE 00116 #elif defined MBED_CONF_MBED_CLIENT_DISABLE_DELAYED_RESPONSE 00117 #define DISABLE_DELAYED_RESPONSE MBED_CONF_MBED_CLIENT_DISABLE_DELAYED_RESPONSE 00118 #endif 00119 00120 #ifdef YOTTA_CFG_DISABLE_BLOCK_MESSAGE 00121 #define DISABLE_BLOCK_MESSAGE YOTTA_CFG_DISABLE_BLOCK_MESSAGE 00122 #elif defined MBED_CONF_MBED_CLIENT_DISABLE_BLOCK_MESSAGE 00123 #define DISABLE_BLOCK_MESSAGE MBED_CONF_MBED_CLIENT_DISABLE_BLOCK_MESSAGE 00124 #endif 00125 00126 #ifdef MBED_CONF_MBED_CLIENT_DTLS_PEER_MAX_TIMEOUT 00127 #define MBED_CLIENT_DTLS_PEER_MAX_TIMEOUT MBED_CONF_MBED_CLIENT_DTLS_PEER_MAX_TIMEOUT 00128 #endif 00129 00130 00131 #if defined (__ICCARM__) 00132 #define m2m_deprecated 00133 #else 00134 #define m2m_deprecated __attribute__ ((deprecated)) 00135 #endif 00136 00137 // This is valid for mbed-client-mbedtls 00138 // For other SSL implementation there 00139 // can be other 00140 00141 /* 00142 *\brief A callback function for a random number 00143 * required by the mbed-client-mbedtls module. 00144 */ 00145 typedef uint32_t (*random_number_cb)(void) ; 00146 00147 /* 00148 *\brief An entropy structure for an mbedtls entropy source. 00149 * \param entropy_source_ptr The entropy function. 00150 * \param p_source The function data. 00151 * \param threshold A minimum required from the source before entropy is released 00152 * (with mbedtls_entropy_func()) (in bytes). 00153 * \param strong MBEDTLS_ENTROPY_SOURCE_STRONG = 1 or 00154 * MBEDTSL_ENTROPY_SOURCE_WEAK = 0. 00155 * At least one strong source needs to be added. 00156 * Weaker sources (such as the cycle counter) can be used as 00157 * a complement. 00158 */ 00159 typedef struct mbedtls_entropy { 00160 int (*entropy_source_ptr)(void *, unsigned char *,size_t , size_t *); 00161 void *p_source; 00162 size_t threshold; 00163 int strong; 00164 }entropy_cb; 00165 00166 #ifdef MBED_CLIENT_USER_CONFIG_FILE 00167 #include MBED_CLIENT_USER_CONFIG_FILE 00168 #endif 00169 00170 #ifndef MBED_CLIENT_RECONNECTION_COUNT 00171 #define MBED_CLIENT_RECONNECTION_COUNT 3 00172 #endif 00173 00174 #ifndef MBED_CLIENT_RECONNECTION_INTERVAL 00175 #define MBED_CLIENT_RECONNECTION_INTERVAL 5 00176 #endif 00177 00178 #ifndef MBED_CLIENT_TCP_KEEPALIVE_INTERVAL 00179 #define MBED_CLIENT_TCP_KEEPALIVE_INTERVAL 90 00180 #endif 00181 00182 #ifndef MBED_CLIENT_EVENT_LOOP_SIZE 00183 #define MBED_CLIENT_EVENT_LOOP_SIZE 1024 00184 #endif 00185 00186 #ifndef SN_COAP_MAX_INCOMING_MESSAGE_SIZE 00187 #define SN_COAP_MAX_INCOMING_MESSAGE_SIZE UINT16_MAX 00188 #endif 00189 00190 #ifndef MBED_CLIENT_DTLS_PEER_MAX_TIMEOUT 00191 #define MBED_CLIENT_DTLS_PEER_MAX_TIMEOUT 80000 00192 #endif 00193 00194 #endif // M2MCONFIG_H
Generated on Tue Jul 12 2022 16:24:13 by
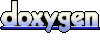