
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
uriqueryparser.h
00001 /* 00002 * Copyright (c) 2017 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifdef __cplusplus 00018 extern "C" { 00019 #endif 00020 00021 #ifndef URIQUERYPARSER_H_ 00022 #define URIQUERYPARSER_H_ 00023 00024 00025 /** 00026 * @brief Parse query parameter from uri and return size of parameter value and pointer to value within 00027 * uri. 00028 * 00029 * Example usage: 00030 * char *value_ptr = NULL; 00031 * ssize_t value_len = parse_query_parameter_value_from_uri("http://www.myquery.com?someparameter=value", "someparameter", &value_ptr); 00032 * will result in value_len = 5 and value_ptr = "value" 00033 * 00034 * @param uri uri to parse. 00035 * @param parameter_name, parameter name to parse from query. 00036 * @param parameter_value[OUT], pointer to parameter value, NULL if parameter does not exist. 00037 * @return size of parameter value, -1 if parameter does not exist in uri 00038 */ 00039 int parse_query_parameter_value_from_uri(const char *uri, const char *parameter_name, const char **parameter_value); 00040 00041 /** 00042 * @brief Parse query parameter from query and return size of parameter value and pointer to value within 00043 * query. 00044 * 00045 * Example usage: 00046 * char *value_ptr = NULL; 00047 * ssize_t value_len = parse_query_parameter_value("someparameter=value¶m2=second", "param2", &value_ptr); 00048 * will result in value_len = 6 and value_ptr = "second" 00049 * 00050 * @param query query to parse. 00051 * @param parameter_name, parameter name to parse from query. 00052 * @param parameter_value[OUT], pointer to parameter value, NULL if parameter does not exist. 00053 * @return size of parameter value, -1 if parameter does not exist in query 00054 */ 00055 int parse_query_parameter_value_from_query(const char *uri, const char *parameter_name, const char **parameter_value); 00056 00057 00058 #endif /* URIQUERYPARSER_H_ */ 00059 00060 #ifdef __cplusplus 00061 } 00062 #endif
Generated on Tue Jul 12 2022 19:12:17 by
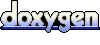