
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
unity_fixture_Test.c
00001 //- Copyright (c) 2010 James Grenning and Contributed to Unity Project 00002 /* ========================================== 00003 Unity Project - A Test Framework for C 00004 Copyright (c) 2007 Mike Karlesky, Mark VanderVoord, Greg Williams 00005 [Released under MIT License. Please refer to license.txt for details] 00006 ========================================== */ 00007 00008 #include "unity_fixture.h" 00009 #include "unity_output_Spy.h" 00010 #include <stdlib.h> 00011 #include <string.h> 00012 00013 TEST_GROUP(UnityFixture); 00014 00015 TEST_SETUP(UnityFixture) 00016 { 00017 } 00018 00019 TEST_TEAR_DOWN(UnityFixture) 00020 { 00021 } 00022 00023 static int* pointer1 = 0; 00024 static int* pointer2 = (int*)2; 00025 static int* pointer3 = (int*)3; 00026 static int int1; 00027 static int int2; 00028 static int int3; 00029 static int int4; 00030 00031 TEST(UnityFixture, PointerSetting) 00032 { 00033 TEST_ASSERT_POINTERS_EQUAL(pointer1, 0); 00034 UT_PTR_SET(pointer1, &int1); 00035 UT_PTR_SET(pointer2, &int2); 00036 UT_PTR_SET(pointer3, &int3); 00037 TEST_ASSERT_POINTERS_EQUAL(pointer1, &int1); 00038 TEST_ASSERT_POINTERS_EQUAL(pointer2, &int2); 00039 TEST_ASSERT_POINTERS_EQUAL(pointer3, &int3); 00040 UT_PTR_SET(pointer1, &int4); 00041 UnityPointer_UndoAllSets(); 00042 TEST_ASSERT_POINTERS_EQUAL(pointer1, 0); 00043 TEST_ASSERT_POINTERS_EQUAL(pointer2, (int*)2); 00044 TEST_ASSERT_POINTERS_EQUAL(pointer3, (int*)3); 00045 } 00046 00047 TEST(UnityFixture, ForceMallocFail) 00048 { 00049 void* m; 00050 void* mfails; 00051 UnityMalloc_MakeMallocFailAfterCount(1); 00052 m = malloc(10); 00053 CHECK(m); 00054 mfails = malloc(10); 00055 TEST_ASSERT_POINTERS_EQUAL(0, mfails); 00056 free(m); 00057 } 00058 00059 TEST(UnityFixture, ReallocSmallerIsUnchanged) 00060 { 00061 void* m1 = malloc(10); 00062 void* m2 = realloc(m1, 5); 00063 TEST_ASSERT_POINTERS_EQUAL(m1, m2); 00064 free(m2); 00065 } 00066 00067 TEST(UnityFixture, ReallocSameIsUnchanged) 00068 { 00069 void* m1 = malloc(10); 00070 void* m2 = realloc(m1, 10); 00071 TEST_ASSERT_POINTERS_EQUAL(m1, m2); 00072 free(m2); 00073 } 00074 00075 TEST(UnityFixture, ReallocLargerNeeded) 00076 { 00077 void* m1 = malloc(10); 00078 void* m2; 00079 CHECK(m1); 00080 strcpy((char*)m1, "123456789"); 00081 m2 = realloc(m1, 15); 00082 // CHECK(m1 != m2); //Depends on implementation 00083 STRCMP_EQUAL("123456789", m2); 00084 free(m2); 00085 } 00086 00087 TEST(UnityFixture, ReallocNullPointerIsLikeMalloc) 00088 { 00089 void* m = realloc(0, 15); 00090 CHECK(m != 0); 00091 free(m); 00092 } 00093 00094 TEST(UnityFixture, ReallocSizeZeroFreesMemAndReturnsNullPointer) 00095 { 00096 void* m1 = malloc(10); 00097 void* m2 = realloc(m1, 0); 00098 TEST_ASSERT_POINTERS_EQUAL(0, m2); 00099 } 00100 00101 TEST(UnityFixture, CallocFillsWithZero) 00102 { 00103 void* m = calloc(3, sizeof(char)); 00104 char* s = (char*)m; 00105 CHECK(m); 00106 TEST_ASSERT_BYTES_EQUAL(0, s[0]); 00107 TEST_ASSERT_BYTES_EQUAL(0, s[1]); 00108 TEST_ASSERT_BYTES_EQUAL(0, s[2]); 00109 free(m); 00110 } 00111 00112 static char *p1; 00113 static char *p2; 00114 00115 TEST(UnityFixture, PointerSet) 00116 { 00117 char c1; 00118 char c2; 00119 char newC1; 00120 char newC2; 00121 p1 = &c1; 00122 p2 = &c2; 00123 00124 UnityPointer_Init(); 00125 UT_PTR_SET(p1, &newC1); 00126 UT_PTR_SET(p2, &newC2); 00127 TEST_ASSERT_POINTERS_EQUAL(&newC1, p1); 00128 TEST_ASSERT_POINTERS_EQUAL(&newC2, p2); 00129 UnityPointer_UndoAllSets(); 00130 TEST_ASSERT_POINTERS_EQUAL(&c1, p1); 00131 TEST_ASSERT_POINTERS_EQUAL(&c2, p2); 00132 } 00133 00134 TEST(UnityFixture, FreeNULLSafety) 00135 { 00136 free(NULL); 00137 } 00138 00139 TEST(UnityFixture, ConcludeTestIncrementsFailCount) 00140 { 00141 _U_UINT savedFails = Unity.TestFailures; 00142 _U_UINT savedIgnores = Unity.TestIgnores; 00143 UnityOutputCharSpy_Enable(1); 00144 Unity.CurrentTestFailed = 1; 00145 UnityConcludeFixtureTest(); // Resets TestFailed for this test to pass 00146 Unity.CurrentTestIgnored = 1; 00147 UnityConcludeFixtureTest(); // Resets TestIgnored 00148 UnityOutputCharSpy_Enable(0); 00149 TEST_ASSERT_EQUAL(savedFails + 1, Unity.TestFailures); 00150 TEST_ASSERT_EQUAL(savedIgnores + 1, Unity.TestIgnores); 00151 Unity.TestFailures = savedFails; 00152 Unity.TestIgnores = savedIgnores; 00153 } 00154 00155 //------------------------------------------------------------ 00156 00157 TEST_GROUP(UnityCommandOptions); 00158 00159 static int savedVerbose; 00160 static unsigned int savedRepeat; 00161 static const char* savedName; 00162 static const char* savedGroup; 00163 00164 TEST_SETUP(UnityCommandOptions) 00165 { 00166 savedVerbose = UnityFixture.Verbose; 00167 savedRepeat = UnityFixture.RepeatCount; 00168 savedName = UnityFixture.NameFilter; 00169 savedGroup = UnityFixture.GroupFilter; 00170 } 00171 00172 TEST_TEAR_DOWN(UnityCommandOptions) 00173 { 00174 UnityFixture.Verbose = savedVerbose; 00175 UnityFixture.RepeatCount= savedRepeat; 00176 UnityFixture.NameFilter = savedName; 00177 UnityFixture.GroupFilter = savedGroup; 00178 } 00179 00180 00181 static const char* noOptions[] = { 00182 "testrunner.exe" 00183 }; 00184 00185 TEST(UnityCommandOptions, DefaultOptions) 00186 { 00187 UnityGetCommandLineOptions(1, noOptions); 00188 TEST_ASSERT_EQUAL(0, UnityFixture.Verbose); 00189 TEST_ASSERT_POINTERS_EQUAL(0, UnityFixture.GroupFilter); 00190 TEST_ASSERT_POINTERS_EQUAL(0, UnityFixture.NameFilter); 00191 TEST_ASSERT_EQUAL(1, UnityFixture.RepeatCount); 00192 } 00193 00194 static const char* verbose[] = { 00195 "testrunner.exe", 00196 "-v" 00197 }; 00198 00199 TEST(UnityCommandOptions, OptionVerbose) 00200 { 00201 TEST_ASSERT_EQUAL(0, UnityGetCommandLineOptions(2, verbose)); 00202 TEST_ASSERT_EQUAL(1, UnityFixture.Verbose); 00203 } 00204 00205 static const char* group[] = { 00206 "testrunner.exe", 00207 "-g", "groupname" 00208 }; 00209 00210 TEST(UnityCommandOptions, OptionSelectTestByGroup) 00211 { 00212 TEST_ASSERT_EQUAL(0, UnityGetCommandLineOptions(3, group)); 00213 STRCMP_EQUAL("groupname", UnityFixture.GroupFilter); 00214 } 00215 00216 static const char* name[] = { 00217 "testrunner.exe", 00218 "-n", "testname" 00219 }; 00220 00221 TEST(UnityCommandOptions, OptionSelectTestByName) 00222 { 00223 TEST_ASSERT_EQUAL(0, UnityGetCommandLineOptions(3, name)); 00224 STRCMP_EQUAL("testname", UnityFixture.NameFilter); 00225 } 00226 00227 static const char* repeat[] = { 00228 "testrunner.exe", 00229 "-r", "99" 00230 }; 00231 00232 TEST(UnityCommandOptions, OptionSelectRepeatTestsDefaultCount) 00233 { 00234 TEST_ASSERT_EQUAL(0, UnityGetCommandLineOptions(2, repeat)); 00235 TEST_ASSERT_EQUAL(2, UnityFixture.RepeatCount); 00236 } 00237 00238 TEST(UnityCommandOptions, OptionSelectRepeatTestsSpecificCount) 00239 { 00240 TEST_ASSERT_EQUAL(0, UnityGetCommandLineOptions(3, repeat)); 00241 TEST_ASSERT_EQUAL(99, UnityFixture.RepeatCount); 00242 } 00243 00244 static const char* multiple[] = { 00245 "testrunner.exe", 00246 "-v", 00247 "-g", "groupname", 00248 "-n", "testname", 00249 "-r", "98" 00250 }; 00251 00252 TEST(UnityCommandOptions, MultipleOptions) 00253 { 00254 TEST_ASSERT_EQUAL(0, UnityGetCommandLineOptions(8, multiple)); 00255 TEST_ASSERT_EQUAL(1, UnityFixture.Verbose); 00256 STRCMP_EQUAL("groupname", UnityFixture.GroupFilter); 00257 STRCMP_EQUAL("testname", UnityFixture.NameFilter); 00258 TEST_ASSERT_EQUAL(98, UnityFixture.RepeatCount); 00259 } 00260 00261 static const char* dashRNotLast[] = { 00262 "testrunner.exe", 00263 "-v", 00264 "-g", "gggg", 00265 "-r", 00266 "-n", "tttt", 00267 }; 00268 00269 TEST(UnityCommandOptions, MultipleOptionsDashRNotLastAndNoValueSpecified) 00270 { 00271 TEST_ASSERT_EQUAL(0, UnityGetCommandLineOptions(7, dashRNotLast)); 00272 TEST_ASSERT_EQUAL(1, UnityFixture.Verbose); 00273 STRCMP_EQUAL("gggg", UnityFixture.GroupFilter); 00274 STRCMP_EQUAL("tttt", UnityFixture.NameFilter); 00275 TEST_ASSERT_EQUAL(2, UnityFixture.RepeatCount); 00276 } 00277 00278 static const char* unknownCommand[] = { 00279 "testrunner.exe", 00280 "-v", 00281 "-g", "groupname", 00282 "-n", "testname", 00283 "-r", "98", 00284 "-z" 00285 }; 00286 TEST(UnityCommandOptions, UnknownCommandIsIgnored) 00287 { 00288 TEST_ASSERT_EQUAL(0, UnityGetCommandLineOptions(9, unknownCommand)); 00289 TEST_ASSERT_EQUAL(1, UnityFixture.Verbose); 00290 STRCMP_EQUAL("groupname", UnityFixture.GroupFilter); 00291 STRCMP_EQUAL("testname", UnityFixture.NameFilter); 00292 TEST_ASSERT_EQUAL(98, UnityFixture.RepeatCount); 00293 } 00294 00295 TEST(UnityCommandOptions, GroupOrNameFilterWithoutStringFails) 00296 { 00297 TEST_ASSERT_EQUAL(1, UnityGetCommandLineOptions(3, unknownCommand)); 00298 TEST_ASSERT_EQUAL(1, UnityGetCommandLineOptions(5, unknownCommand)); 00299 TEST_ASSERT_EQUAL(1, UnityMain(3, unknownCommand, NULL)); 00300 } 00301 00302 TEST(UnityCommandOptions, GroupFilterReallyFilters) 00303 { 00304 _U_UINT saved = Unity.NumberOfTests; 00305 TEST_ASSERT_EQUAL(0, UnityGetCommandLineOptions(4, unknownCommand)); 00306 UnityIgnoreTest(NULL, "non-matching", NULL); 00307 TEST_ASSERT_EQUAL(saved, Unity.NumberOfTests); 00308 } 00309 00310 IGNORE_TEST(UnityCommandOptions, TestShouldBeIgnored) 00311 { 00312 TEST_FAIL_MESSAGE("This test should not run!"); 00313 } 00314 00315 //------------------------------------------------------------ 00316 00317 TEST_GROUP(LeakDetection); 00318 00319 TEST_SETUP(LeakDetection) 00320 { 00321 #ifdef UNITY_EXCLUDE_STDLIB_MALLOC 00322 UnityOutputCharSpy_Create(200); 00323 #else 00324 UnityOutputCharSpy_Create(1000); 00325 #endif 00326 } 00327 00328 TEST_TEAR_DOWN(LeakDetection) 00329 { 00330 UnityOutputCharSpy_Destroy(); 00331 } 00332 00333 #define EXPECT_ABORT_BEGIN \ 00334 { \ 00335 jmp_buf TestAbortFrame; \ 00336 memcpy(TestAbortFrame, Unity.AbortFrame, sizeof(jmp_buf)); \ 00337 if (TEST_PROTECT()) \ 00338 { 00339 00340 #define EXPECT_ABORT_END \ 00341 } \ 00342 memcpy(Unity.AbortFrame, TestAbortFrame, sizeof(jmp_buf)); \ 00343 } 00344 00345 // This tricky set of defines lets us see if we are using the Spy, returns 1 if true 00346 #ifdef __STDC_VERSION__ 00347 00348 #if __STDC_VERSION__ >= 199901L 00349 #define USING_SPY_AS(a) EXPAND_AND_USE_2ND(ASSIGN_VALUE(a), 0) 00350 #define ASSIGN_VALUE(a) VAL_##a 00351 #define VAL_UnityOutputCharSpy_OutputChar 0, 1 00352 #define EXPAND_AND_USE_2ND(a, b) SECOND_PARAM(a, b, throwaway) 00353 #define SECOND_PARAM(a, b, ...) b 00354 #if USING_SPY_AS(UNITY_OUTPUT_CHAR) 00355 #define USING_OUTPUT_SPY // UNITY_OUTPUT_CHAR = UnityOutputCharSpy_OutputChar 00356 #endif 00357 #endif // >= 199901 00358 00359 #else // __STDC_VERSION__ else 00360 #define UnityOutputCharSpy_OutputChar 42 00361 #if UNITY_OUTPUT_CHAR == UnityOutputCharSpy_OutputChar // Works if no -Wundef -Werror 00362 #define USING_OUTPUT_SPY 00363 #endif 00364 #undef UnityOutputCharSpy_OutputChar 00365 #endif // __STDC_VERSION__ 00366 00367 TEST(LeakDetection, DetectsLeak) 00368 { 00369 #ifndef USING_OUTPUT_SPY 00370 TEST_IGNORE_MESSAGE("Build with '-D UNITY_OUTPUT_CHAR=UnityOutputCharSpy_OutputChar' to enable tests"); 00371 #else 00372 void* m = malloc(10); 00373 TEST_ASSERT_NOT_NULL(m); 00374 UnityOutputCharSpy_Enable(1); 00375 EXPECT_ABORT_BEGIN 00376 UnityMalloc_EndTest(); 00377 EXPECT_ABORT_END 00378 UnityOutputCharSpy_Enable(0); 00379 Unity.CurrentTestFailed = 0; 00380 CHECK(strstr(UnityOutputCharSpy_Get(), "This test leaks!")); 00381 free(m); 00382 #endif 00383 } 00384 00385 TEST(LeakDetection, BufferOverrunFoundDuringFree) 00386 { 00387 #ifndef USING_OUTPUT_SPY 00388 TEST_IGNORE(); 00389 #else 00390 void* m = malloc(10); 00391 char* s = (char*)m; 00392 TEST_ASSERT_NOT_NULL(m); 00393 s[10] = (char)0xFF; 00394 UnityOutputCharSpy_Enable(1); 00395 EXPECT_ABORT_BEGIN 00396 free(m); 00397 EXPECT_ABORT_END 00398 UnityOutputCharSpy_Enable(0); 00399 Unity.CurrentTestFailed = 0; 00400 CHECK(strstr(UnityOutputCharSpy_Get(), "Buffer overrun detected during free()")); 00401 #endif 00402 } 00403 00404 TEST(LeakDetection, BufferOverrunFoundDuringRealloc) 00405 { 00406 #ifndef USING_OUTPUT_SPY 00407 TEST_IGNORE(); 00408 #else 00409 void* m = malloc(10); 00410 char* s = (char*)m; 00411 TEST_ASSERT_NOT_NULL(m); 00412 s[10] = (char)0xFF; 00413 UnityOutputCharSpy_Enable(1); 00414 EXPECT_ABORT_BEGIN 00415 m = realloc(m, 100); 00416 EXPECT_ABORT_END 00417 UnityOutputCharSpy_Enable(0); 00418 Unity.CurrentTestFailed = 0; 00419 CHECK(strstr(UnityOutputCharSpy_Get(), "Buffer overrun detected during realloc()")); 00420 #endif 00421 } 00422 00423 TEST(LeakDetection, BufferGuardWriteFoundDuringFree) 00424 { 00425 #ifndef USING_OUTPUT_SPY 00426 TEST_IGNORE(); 00427 #else 00428 void* m = malloc(10); 00429 char* s = (char*)m; 00430 TEST_ASSERT_NOT_NULL(m); 00431 s[-1] = (char)0x00; // Will not detect 0 00432 s[-2] = (char)0x01; 00433 UnityOutputCharSpy_Enable(1); 00434 EXPECT_ABORT_BEGIN 00435 free(m); 00436 EXPECT_ABORT_END 00437 UnityOutputCharSpy_Enable(0); 00438 Unity.CurrentTestFailed = 0; 00439 CHECK(strstr(UnityOutputCharSpy_Get(), "Buffer overrun detected during free()")); 00440 #endif 00441 } 00442 00443 TEST(LeakDetection, BufferGuardWriteFoundDuringRealloc) 00444 { 00445 #ifndef USING_OUTPUT_SPY 00446 TEST_IGNORE(); 00447 #else 00448 void* m = malloc(10); 00449 char* s = (char*)m; 00450 TEST_ASSERT_NOT_NULL(m); 00451 s[-1] = (char)0x0A; 00452 UnityOutputCharSpy_Enable(1); 00453 EXPECT_ABORT_BEGIN 00454 m = realloc(m, 100); 00455 EXPECT_ABORT_END 00456 UnityOutputCharSpy_Enable(0); 00457 Unity.CurrentTestFailed = 0; 00458 CHECK(strstr(UnityOutputCharSpy_Get(), "Buffer overrun detected during realloc()")); 00459 #endif 00460 } 00461 00462 TEST(LeakDetection, PointerSettingMax) 00463 { 00464 #ifndef USING_OUTPUT_SPY 00465 TEST_IGNORE(); 00466 #else 00467 int i; 00468 for (i = 0; i < 50; i++) UT_PTR_SET(pointer1, &int1); 00469 UnityOutputCharSpy_Enable(1); 00470 EXPECT_ABORT_BEGIN 00471 UT_PTR_SET(pointer1, &int1); 00472 EXPECT_ABORT_END 00473 UnityOutputCharSpy_Enable(0); 00474 Unity.CurrentTestFailed = 0; 00475 CHECK(strstr(UnityOutputCharSpy_Get(), "Too many pointers set")); 00476 #endif 00477 } 00478 00479 //------------------------------------------------------------ 00480 00481 TEST_GROUP(InternalMalloc); 00482 00483 TEST_SETUP(InternalMalloc) { } 00484 TEST_TEAR_DOWN(InternalMalloc) { } 00485 00486 TEST(InternalMalloc, MallocPastBufferFails) 00487 { 00488 #ifdef UNITY_EXCLUDE_STDLIB_MALLOC 00489 void* m = malloc(UNITY_INTERNAL_HEAP_SIZE_BYTES/2 + 1); 00490 void* n = malloc(UNITY_INTERNAL_HEAP_SIZE_BYTES/2); 00491 TEST_ASSERT_NOT_NULL(m); 00492 TEST_ASSERT_NULL(n); 00493 free(m); 00494 #endif 00495 } 00496 00497 TEST(InternalMalloc, CallocPastBufferFails) 00498 { 00499 #ifdef UNITY_EXCLUDE_STDLIB_MALLOC 00500 void* m = calloc(1, UNITY_INTERNAL_HEAP_SIZE_BYTES/2 + 1); 00501 void* n = calloc(1, UNITY_INTERNAL_HEAP_SIZE_BYTES/2); 00502 TEST_ASSERT_NOT_NULL(m); 00503 TEST_ASSERT_NULL(n); 00504 free(m); 00505 #endif 00506 } 00507 00508 TEST(InternalMalloc, MallocThenReallocGrowsMemoryInPlace) 00509 { 00510 #ifdef UNITY_EXCLUDE_STDLIB_MALLOC 00511 void* m = malloc(UNITY_INTERNAL_HEAP_SIZE_BYTES/2 + 1); 00512 void* n = realloc(m, UNITY_INTERNAL_HEAP_SIZE_BYTES/2 + 9); 00513 TEST_ASSERT_NOT_NULL(m); 00514 TEST_ASSERT_EQUAL(m, n); 00515 free(n); 00516 #endif 00517 } 00518 00519 TEST(InternalMalloc, ReallocFailDoesNotFreeMem) 00520 { 00521 #ifdef UNITY_EXCLUDE_STDLIB_MALLOC 00522 void* m = malloc(UNITY_INTERNAL_HEAP_SIZE_BYTES/2); 00523 void* n1 = malloc(10); 00524 void* out_of_mem = realloc(n1, UNITY_INTERNAL_HEAP_SIZE_BYTES/2 + 1); 00525 void* n2 = malloc(10); 00526 TEST_ASSERT_NOT_NULL(m); 00527 TEST_ASSERT_NULL(out_of_mem); 00528 TEST_ASSERT_NOT_EQUAL(n2, n1); 00529 free(n2); 00530 free(n1); 00531 free(m); 00532 #endif 00533 }
Generated on Tue Jul 12 2022 19:12:17 by
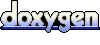