
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
unity_fixture.c
00001 //- Copyright (c) 2010 James Grenning and Contributed to Unity Project 00002 /* ========================================== 00003 Unity Project - A Test Framework for C 00004 Copyright (c) 2007 Mike Karlesky, Mark VanderVoord, Greg Williams 00005 [Released under MIT License. Please refer to license.txt for details] 00006 ========================================== */ 00007 00008 #include <string.h> 00009 #include "unity_fixture.h" 00010 #include "unity_internals.h" 00011 00012 #define UNITY_RESULTS_TAGS_TEST_START "<***UnityTest***>" 00013 #define UNITY_RESULTS_TAGS_TEST_END "</***UnityTest***>" 00014 00015 #define UNITY_RESULTS_TAGS_RESULT_START "<***UnityResult***>" 00016 #define UNITY_RESULTS_TAGS_RESULT_END "</***UnityResult***>" 00017 00018 #define UNITY_RESULTS_TAGS_IGNORE_START "<***UnityIgnoredTest***>" 00019 #define UNITY_RESULTS_TAGS_IGNORE_END "</***UnityIgnoredTest***>" 00020 00021 struct _UnityFixture UnityFixture; 00022 00023 //If you decide to use the function pointer approach. 00024 //Build with -D UNITY_OUTPUT_CHAR=outputChar and include <stdio.h> 00025 //int (*outputChar)(int) = putchar; 00026 00027 #if !defined(UNITY_WEAK_ATTRIBUTE) && !defined(UNITY_WEAK_PRAGMA) 00028 void setUp(void) { /*does nothing*/ } 00029 void tearDown(void) { /*does nothing*/ } 00030 #endif 00031 00032 static void announceTestRun(unsigned int runNumber) 00033 { 00034 UnityPrint("Unity test run "); 00035 UnityPrintNumberUnsigned(runNumber+1); 00036 UnityPrint(" of "); 00037 UnityPrintNumberUnsigned(UnityFixture.RepeatCount); 00038 UNITY_PRINT_EOL(); 00039 } 00040 00041 int UnityMain(int argc, const char* argv[], void (*runAllTests)(void)) 00042 { 00043 int result = UnityGetCommandLineOptions(argc, argv); 00044 unsigned int r; 00045 if (result != 0) 00046 return result; 00047 00048 for (r = 0; r < UnityFixture.RepeatCount; r++) 00049 { 00050 UnityBegin(argv[0]); 00051 announceTestRun(r); 00052 runAllTests(); 00053 UNITY_PRINT_EOL(); 00054 UnityEnd(); 00055 } 00056 00057 return (int)Unity.TestFailures; 00058 } 00059 00060 static int selected(const char* filter, const char* name) 00061 { 00062 if (filter == 0) 00063 return 1; 00064 return strstr(name, filter) ? 1 : 0; 00065 } 00066 00067 static int testSelected(const char* test) 00068 { 00069 return selected(UnityFixture.NameFilter, test); 00070 } 00071 00072 static int groupSelected(const char* group) 00073 { 00074 return selected(UnityFixture.GroupFilter, group); 00075 } 00076 00077 void UnityTestRunner(unityfunction* setup, 00078 unityfunction* testBody, 00079 unityfunction* teardown, 00080 const char* printableName, 00081 const char* group, 00082 const char* name, 00083 const char* file, unsigned int line) 00084 { 00085 if (testSelected(name) && groupSelected(group)) 00086 { 00087 Unity.TestFile = file; 00088 Unity.CurrentTestName = printableName; 00089 Unity.CurrentTestLineNumber = line; 00090 if (!UnityFixture.Verbose) 00091 UNITY_OUTPUT_CHAR('.'); 00092 else 00093 { 00094 /* SA_PATCH: Output results using easy to parse tags. */ 00095 UnityPrint(UNITY_RESULTS_TAGS_TEST_START); 00096 UnityPrint(printableName); 00097 /* SA_PATCH: Output results using easy to parse tags. */ 00098 UnityPrint(UNITY_RESULTS_TAGS_TEST_END); 00099 //UnityPrint(printableName); 00100 } 00101 00102 Unity.NumberOfTests++; 00103 UnityMalloc_StartTest(); 00104 UnityPointer_Init(); 00105 00106 if (TEST_PROTECT()) 00107 { 00108 setup(); 00109 testBody(); 00110 } 00111 if (TEST_PROTECT()) 00112 { 00113 teardown(); 00114 } 00115 if (TEST_PROTECT()) 00116 { 00117 UnityPointer_UndoAllSets(); 00118 if (!Unity.CurrentTestFailed) 00119 UnityMalloc_EndTest(); 00120 } 00121 UnityConcludeFixtureTest(); 00122 } 00123 } 00124 00125 void UnityIgnoreTest(const char* printableName, const char* group, const char* name) 00126 { 00127 if (testSelected(name) && groupSelected(group)) 00128 { 00129 Unity.NumberOfTests++; 00130 Unity.TestIgnores++; 00131 if (!UnityFixture.Verbose) 00132 UNITY_OUTPUT_CHAR('!'); 00133 else 00134 { 00135 /* SA_PATCH: Output results using easy to parse tags. */ 00136 UnityPrint(UNITY_RESULTS_TAGS_IGNORE_START); 00137 UnityPrint(printableName); 00138 /* SA_PATCH: Output results using easy to parse tags. */ 00139 UnityPrint(UNITY_RESULTS_TAGS_IGNORE_END); 00140 //UnityPrint(printableName); 00141 //UNITY_PRINT_EOL(); 00142 } 00143 } 00144 } 00145 00146 00147 //------------------------------------------------- 00148 //Malloc and free stuff 00149 // 00150 #define MALLOC_DONT_FAIL -1 00151 static int malloc_count; 00152 static int malloc_fail_countdown = MALLOC_DONT_FAIL; 00153 00154 void UnityMalloc_StartTest(void) 00155 { 00156 malloc_count = 0; 00157 malloc_fail_countdown = MALLOC_DONT_FAIL; 00158 } 00159 00160 void UnityMalloc_EndTest(void) 00161 { 00162 malloc_fail_countdown = MALLOC_DONT_FAIL; 00163 if (malloc_count != 0) 00164 { 00165 UNITY_TEST_FAIL(Unity.CurrentTestLineNumber, "This test leaks!"); 00166 } 00167 } 00168 00169 void UnityMalloc_MakeMallocFailAfterCount(int countdown) 00170 { 00171 malloc_fail_countdown = countdown; 00172 } 00173 00174 // These definitions are always included from unity_fixture_malloc_overrides.h 00175 // We undef to use them or avoid conflict with <stdlib.h> per the C standard 00176 #undef malloc 00177 #undef free 00178 #undef calloc 00179 #undef realloc 00180 00181 #ifdef UNITY_EXCLUDE_STDLIB_MALLOC 00182 static unsigned char unity_heap[UNITY_INTERNAL_HEAP_SIZE_BYTES]; 00183 static size_t heap_index; 00184 #else 00185 #include <stdlib.h> 00186 #endif 00187 00188 typedef struct GuardBytes 00189 { 00190 size_t size; 00191 size_t guard_space; 00192 } Guard; 00193 00194 00195 static const char end[] = "END"; 00196 00197 void* unity_malloc(size_t size) 00198 { 00199 char* mem; 00200 Guard* guard; 00201 size_t total_size = size + sizeof(Guard) + sizeof(end); 00202 00203 if (malloc_fail_countdown != MALLOC_DONT_FAIL) 00204 { 00205 if (malloc_fail_countdown == 0) 00206 return NULL; 00207 malloc_fail_countdown--; 00208 } 00209 00210 if (size == 0) return NULL; 00211 #ifdef UNITY_EXCLUDE_STDLIB_MALLOC 00212 if (heap_index + total_size > UNITY_INTERNAL_HEAP_SIZE_BYTES) 00213 { 00214 guard = NULL; 00215 } 00216 else 00217 { 00218 guard = (Guard*) &unity_heap[heap_index]; 00219 heap_index += total_size; 00220 } 00221 #else 00222 guard = (Guard*)UNITY_FIXTURE_MALLOC(total_size); 00223 #endif 00224 if (guard == NULL) return NULL; 00225 malloc_count++; 00226 guard->size = size; 00227 guard->guard_space = 0; 00228 mem = (char*)&(guard[1]); 00229 memcpy(&mem[size], end, sizeof(end)); 00230 00231 return (void*)mem; 00232 } 00233 00234 static int isOverrun(void* mem) 00235 { 00236 Guard* guard = (Guard*)mem; 00237 char* memAsChar = (char*)mem; 00238 guard--; 00239 00240 return guard->guard_space != 0 || strcmp(&memAsChar[guard->size], end) != 0; 00241 } 00242 00243 static void release_memory(void* mem) 00244 { 00245 Guard* guard = (Guard*)mem; 00246 guard--; 00247 00248 malloc_count--; 00249 #ifdef UNITY_EXCLUDE_STDLIB_MALLOC 00250 if (mem == unity_heap + heap_index - guard->size - sizeof(end)) 00251 { 00252 heap_index -= (guard->size + sizeof(Guard) + sizeof(end)); 00253 } 00254 #else 00255 UNITY_FIXTURE_FREE(guard); 00256 #endif 00257 } 00258 00259 void unity_free(void* mem) 00260 { 00261 int overrun; 00262 00263 if (mem == NULL) 00264 { 00265 return; 00266 } 00267 00268 overrun = isOverrun(mem); 00269 release_memory(mem); 00270 if (overrun) 00271 { 00272 UNITY_TEST_FAIL(Unity.CurrentTestLineNumber, "Buffer overrun detected during free()"); 00273 } 00274 } 00275 00276 void* unity_calloc(size_t num, size_t size) 00277 { 00278 void* mem = unity_malloc(num * size); 00279 if (mem == NULL) return NULL; 00280 memset(mem, 0, num * size); 00281 return mem; 00282 } 00283 00284 void* unity_realloc(void* oldMem, size_t size) 00285 { 00286 Guard* guard = (Guard*)oldMem; 00287 void* newMem; 00288 00289 if (oldMem == NULL) return unity_malloc(size); 00290 00291 guard--; 00292 if (isOverrun(oldMem)) 00293 { 00294 release_memory(oldMem); 00295 UNITY_TEST_FAIL(Unity.CurrentTestLineNumber, "Buffer overrun detected during realloc()"); 00296 } 00297 00298 if (size == 0) 00299 { 00300 release_memory(oldMem); 00301 return NULL; 00302 } 00303 00304 if (guard->size >= size) return oldMem; 00305 00306 #ifdef UNITY_EXCLUDE_STDLIB_MALLOC // Optimization if memory is expandable 00307 if (oldMem == unity_heap + heap_index - guard->size - sizeof(end) && 00308 heap_index + size - guard->size <= UNITY_INTERNAL_HEAP_SIZE_BYTES) 00309 { 00310 release_memory(oldMem); // Not thread-safe, like unity_heap generally 00311 return unity_malloc(size); // No memcpy since data is in place 00312 } 00313 #endif 00314 newMem = unity_malloc(size); 00315 if (newMem == NULL) return NULL; // Do not release old memory 00316 memcpy(newMem, oldMem, guard->size); 00317 release_memory(oldMem); 00318 return newMem; 00319 } 00320 00321 00322 //-------------------------------------------------------- 00323 //Automatic pointer restoration functions 00324 struct PointerPair 00325 { 00326 void** pointer; 00327 void* old_value; 00328 }; 00329 00330 enum { MAX_POINTERS = 50 }; 00331 static struct PointerPair pointer_store[MAX_POINTERS]; 00332 static int pointer_index = 0; 00333 00334 void UnityPointer_Init(void) 00335 { 00336 pointer_index = 0; 00337 } 00338 00339 void UnityPointer_Set(void** pointer, void* newValue, UNITY_LINE_TYPE line) 00340 { 00341 if (pointer_index >= MAX_POINTERS) 00342 { 00343 UNITY_TEST_FAIL(line, "Too many pointers set"); 00344 } 00345 else 00346 { 00347 pointer_store[pointer_index].pointer = pointer; 00348 pointer_store[pointer_index].old_value = *pointer; 00349 *pointer = newValue; 00350 pointer_index++; 00351 } 00352 } 00353 00354 void UnityPointer_UndoAllSets(void) 00355 { 00356 while (pointer_index > 0) 00357 { 00358 pointer_index--; 00359 *(pointer_store[pointer_index].pointer) = 00360 pointer_store[pointer_index].old_value; 00361 } 00362 } 00363 00364 int UnityGetCommandLineOptions(int argc, const char* argv[]) 00365 { 00366 int i; 00367 UnityFixture.Verbose = 0; 00368 UnityFixture.GroupFilter = 0; 00369 UnityFixture.NameFilter = 0; 00370 UnityFixture.RepeatCount = 1; 00371 00372 if (argc == 1) 00373 return 0; 00374 00375 for (i = 1; i < argc; ) 00376 { 00377 if (strcmp(argv[i], "-v") == 0) 00378 { 00379 UnityFixture.Verbose = 1; 00380 i++; 00381 } 00382 else if (strcmp(argv[i], "-g") == 0) 00383 { 00384 i++; 00385 if (i >= argc) 00386 return 1; 00387 UnityFixture.GroupFilter = argv[i]; 00388 i++; 00389 } 00390 else if (strcmp(argv[i], "-n") == 0) 00391 { 00392 i++; 00393 if (i >= argc) 00394 return 1; 00395 UnityFixture.NameFilter = argv[i]; 00396 i++; 00397 } 00398 else if (strcmp(argv[i], "-r") == 0) 00399 { 00400 UnityFixture.RepeatCount = 2; 00401 i++; 00402 if (i < argc) 00403 { 00404 if (*(argv[i]) >= '0' && *(argv[i]) <= '9') 00405 { 00406 unsigned int digit = 0; 00407 UnityFixture.RepeatCount = 0; 00408 while (argv[i][digit] >= '0' && argv[i][digit] <= '9') 00409 { 00410 UnityFixture.RepeatCount *= 10; 00411 UnityFixture.RepeatCount += (unsigned int)argv[i][digit++] - '0'; 00412 } 00413 i++; 00414 } 00415 } 00416 } else { 00417 // ignore unknown parameter 00418 i++; 00419 } 00420 } 00421 return 0; 00422 } 00423 00424 void UnityConcludeFixtureTest(void) 00425 { 00426 if (Unity.CurrentTestIgnored) 00427 { 00428 Unity.TestIgnores++; 00429 UNITY_PRINT_EOL(); 00430 } 00431 else if (!Unity.CurrentTestFailed) 00432 { 00433 if (UnityFixture.Verbose) 00434 { 00435 UnityPrint(" "); 00436 /* SA_PATCH: Output results using easy to parse tags. */ 00437 UnityPrint(UNITY_RESULTS_TAGS_RESULT_START); 00438 UnityPrint("PASS"); 00439 /* SA_PATCH: Output results using easy to parse tags. */ 00440 UnityPrint(UNITY_RESULTS_TAGS_RESULT_END); 00441 UNITY_OUTPUT_CHAR('\n'); 00442 } 00443 // { 00444 // UnityPrint(" PASS"); 00445 // UNITY_PRINT_EOL(); 00446 // } 00447 } 00448 else // Unity.CurrentTestFailed 00449 { 00450 Unity.TestFailures++; 00451 UNITY_PRINT_EOL(); 00452 } 00453 00454 Unity.CurrentTestFailed = 0; 00455 Unity.CurrentTestIgnored = 0; 00456 }
Generated on Tue Jul 12 2022 19:12:17 by
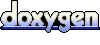