
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
unity.c
00001 /* ========================================================================= 00002 Unity Project - A Test Framework for C 00003 Copyright (c) 2007-14 Mike Karlesky, Mark VanderVoord, Greg Williams 00004 [Released under MIT License. Please refer to license.txt for details] 00005 ============================================================================ */ 00006 00007 #include "unity.h" 00008 #include <stddef.h> 00009 00010 /* If omitted from header, declare overrideable prototypes here so they're ready for use */ 00011 #ifdef UNITY_OMIT_OUTPUT_CHAR_HEADER_DECLARATION 00012 void UNITY_OUTPUT_CHAR(int); 00013 #endif 00014 00015 /* Helpful macros for us to use here */ 00016 #define UNITY_FAIL_AND_BAIL { Unity.CurrentTestFailed = 1; longjmp(Unity.AbortFrame, 1); } 00017 #define UNITY_IGNORE_AND_BAIL { Unity.CurrentTestIgnored = 1; longjmp(Unity.AbortFrame, 1); } 00018 00019 /* return prematurely if we are already in failure or ignore state */ 00020 #define UNITY_SKIP_EXECUTION { if ((Unity.CurrentTestFailed != 0) || (Unity.CurrentTestIgnored != 0)) {return;} } 00021 00022 struct _Unity Unity; 00023 00024 static const char UnityStrOk[] = "OK"; 00025 static const char UnityStrPass[] = "PASS"; 00026 static const char UnityStrFail[] = "FAIL"; 00027 static const char UnityStrIgnore[] = "IGNORE"; 00028 static const char UnityStrNull[] = "NULL"; 00029 static const char UnityStrSpacer[] = ". "; 00030 static const char UnityStrExpected[] = " Expected "; 00031 static const char UnityStrWas[] = " Was "; 00032 static const char UnityStrElement[] = " Element "; 00033 static const char UnityStrByte[] = " Byte "; 00034 static const char UnityStrMemory[] = " Memory Mismatch."; 00035 static const char UnityStrDelta[] = " Values Not Within Delta "; 00036 static const char UnityStrPointless[] = " You Asked Me To Compare Nothing, Which Was Pointless."; 00037 static const char UnityStrNullPointerForExpected[] = " Expected pointer to be NULL"; 00038 static const char UnityStrNullPointerForActual[] = " Actual pointer was NULL"; 00039 static const char UnityStrNot[] = "Not "; 00040 static const char UnityStrInf[] = "Infinity"; 00041 static const char UnityStrNegInf[] = "Negative Infinity"; 00042 static const char UnityStrNaN[] = "NaN"; 00043 static const char UnityStrDet[] = "Determinate"; 00044 static const char UnityStrInvalidFloatTrait[] = "Invalid Float Trait"; 00045 const char UnityStrErrFloat[] = "Unity Floating Point Disabled"; 00046 const char UnityStrErrDouble[] = "Unity Double Precision Disabled"; 00047 const char UnityStrErr64[] = "Unity 64-bit Support Disabled"; 00048 static const char UnityStrBreaker[] = "-----------------------"; 00049 static const char UnityStrResultsTests[] = " Tests "; 00050 static const char UnityStrResultsFailures[] = " Failures "; 00051 static const char UnityStrResultsIgnored[] = " Ignored "; 00052 static const char UnityStrDetail1Name[] = UNITY_DETAIL1_NAME " "; 00053 static const char UnityStrDetail2Name[] = " " UNITY_DETAIL2_NAME " "; 00054 00055 #ifdef UNITY_FLOAT_NEEDS_ZERO 00056 /* Dividing by these constants produces +/- infinity. 00057 * The rationale is given in UnityAssertFloatIsInf's body. */ 00058 static const _UF f_zero = 0.0f; 00059 #endif 00060 00061 /* compiler-generic print formatting masks */ 00062 static const _U_UINT UnitySizeMask[] = 00063 { 00064 255u, /* 0xFF */ 00065 65535u, /* 0xFFFF */ 00066 65535u, 00067 4294967295u, /* 0xFFFFFFFF */ 00068 4294967295u, 00069 4294967295u, 00070 4294967295u 00071 #ifdef UNITY_SUPPORT_64 00072 ,0xFFFFFFFFFFFFFFFF 00073 #endif 00074 }; 00075 00076 /*----------------------------------------------- 00077 * Pretty Printers & Test Result Output Handlers 00078 *-----------------------------------------------*/ 00079 00080 void UnityPrint(const char* string) 00081 { 00082 const char* pch = string; 00083 00084 if (pch != NULL) 00085 { 00086 while (*pch) 00087 { 00088 /* printable characters plus CR & LF are printed */ 00089 if ((*pch <= 126) && (*pch >= 32)) 00090 { 00091 UNITY_OUTPUT_CHAR(*pch); 00092 } 00093 /* write escaped carriage returns */ 00094 else if (*pch == 13) 00095 { 00096 UNITY_OUTPUT_CHAR('\\'); 00097 UNITY_OUTPUT_CHAR('r'); 00098 } 00099 /* write escaped line feeds */ 00100 else if (*pch == 10) 00101 { 00102 UNITY_OUTPUT_CHAR('\\'); 00103 UNITY_OUTPUT_CHAR('n'); 00104 } 00105 /* unprintable characters are shown as codes */ 00106 else 00107 { 00108 UNITY_OUTPUT_CHAR('\\'); 00109 UnityPrintNumberHex((_U_UINT)*pch, 2); 00110 } 00111 pch++; 00112 } 00113 } 00114 } 00115 00116 void UnityPrintLen(const char* string, const _UU32 length); 00117 void UnityPrintLen(const char* string, const _UU32 length) 00118 { 00119 const char* pch = string; 00120 00121 if (pch != NULL) 00122 { 00123 while (*pch && (_UU32)(pch - string) < length) 00124 { 00125 /* printable characters plus CR & LF are printed */ 00126 if ((*pch <= 126) && (*pch >= 32)) 00127 { 00128 UNITY_OUTPUT_CHAR(*pch); 00129 } 00130 /* write escaped carriage returns */ 00131 else if (*pch == 13) 00132 { 00133 UNITY_OUTPUT_CHAR('\\'); 00134 UNITY_OUTPUT_CHAR('r'); 00135 } 00136 /* write escaped line feeds */ 00137 else if (*pch == 10) 00138 { 00139 UNITY_OUTPUT_CHAR('\\'); 00140 UNITY_OUTPUT_CHAR('n'); 00141 } 00142 /* unprintable characters are shown as codes */ 00143 else 00144 { 00145 UNITY_OUTPUT_CHAR('\\'); 00146 UnityPrintNumberHex((_U_UINT)*pch, 2); 00147 } 00148 pch++; 00149 } 00150 } 00151 } 00152 00153 /*-----------------------------------------------*/ 00154 void UnityPrintNumberByStyle(const _U_SINT number, const UNITY_DISPLAY_STYLE_T style) 00155 { 00156 if ((style & UNITY_DISPLAY_RANGE_INT) == UNITY_DISPLAY_RANGE_INT) 00157 { 00158 UnityPrintNumber(number); 00159 } 00160 else if ((style & UNITY_DISPLAY_RANGE_UINT) == UNITY_DISPLAY_RANGE_UINT) 00161 { 00162 UnityPrintNumberUnsigned( (_U_UINT)number & UnitySizeMask[((_U_UINT)style & (_U_UINT)0x0F) - 1] ); 00163 } 00164 else 00165 { 00166 UnityPrintNumberHex((_U_UINT)number, (char)((style & 0x000F) << 1)); 00167 } 00168 } 00169 00170 /*-----------------------------------------------*/ 00171 void UnityPrintNumber(const _U_SINT number_to_print) 00172 { 00173 _U_UINT number = (_U_UINT)number_to_print; 00174 00175 if (number_to_print < 0) 00176 { 00177 /* A negative number, including MIN negative */ 00178 UNITY_OUTPUT_CHAR('-'); 00179 number = (_U_UINT)(-number_to_print); 00180 } 00181 UnityPrintNumberUnsigned(number); 00182 } 00183 00184 /*----------------------------------------------- 00185 * basically do an itoa using as little ram as possible */ 00186 void UnityPrintNumberUnsigned(const _U_UINT number) 00187 { 00188 _U_UINT divisor = 1; 00189 00190 /* figure out initial divisor */ 00191 while (number / divisor > 9) 00192 { 00193 divisor *= 10; 00194 } 00195 00196 /* now mod and print, then divide divisor */ 00197 do 00198 { 00199 UNITY_OUTPUT_CHAR((char)('0' + (number / divisor % 10))); 00200 divisor /= 10; 00201 } 00202 while (divisor > 0); 00203 } 00204 00205 /*-----------------------------------------------*/ 00206 void UnityPrintNumberHex(const _U_UINT number, const char nibbles_to_print) 00207 { 00208 _U_UINT nibble; 00209 char nibbles = nibbles_to_print; 00210 UNITY_OUTPUT_CHAR('0'); 00211 UNITY_OUTPUT_CHAR('x'); 00212 00213 while (nibbles > 0) 00214 { 00215 nibble = (number >> (--nibbles << 2)) & 0x0000000F; 00216 if (nibble <= 9) 00217 { 00218 UNITY_OUTPUT_CHAR((char)('0' + nibble)); 00219 } 00220 else 00221 { 00222 UNITY_OUTPUT_CHAR((char)('A' - 10 + nibble)); 00223 } 00224 } 00225 } 00226 00227 /*-----------------------------------------------*/ 00228 void UnityPrintMask(const _U_UINT mask, const _U_UINT number) 00229 { 00230 _U_UINT current_bit = (_U_UINT)1 << (UNITY_INT_WIDTH - 1); 00231 _US32 i; 00232 00233 for (i = 0; i < UNITY_INT_WIDTH; i++) 00234 { 00235 if (current_bit & mask) 00236 { 00237 if (current_bit & number) 00238 { 00239 UNITY_OUTPUT_CHAR('1'); 00240 } 00241 else 00242 { 00243 UNITY_OUTPUT_CHAR('0'); 00244 } 00245 } 00246 else 00247 { 00248 UNITY_OUTPUT_CHAR('X'); 00249 } 00250 current_bit = current_bit >> 1; 00251 } 00252 } 00253 00254 /*-----------------------------------------------*/ 00255 #ifdef UNITY_FLOAT_VERBOSE 00256 #include <stdio.h> 00257 00258 #ifndef UNITY_VERBOSE_NUMBER_MAX_LENGTH 00259 # ifdef UNITY_DOUBLE_VERBOSE 00260 # define UNITY_VERBOSE_NUMBER_MAX_LENGTH 317 00261 # else 00262 # define UNITY_VERBOSE_NUMBER_MAX_LENGTH 47 00263 # endif 00264 #endif 00265 00266 void UnityPrintFloat(_UF number) 00267 { 00268 char TempBuffer[UNITY_VERBOSE_NUMBER_MAX_LENGTH + 1]; 00269 snprintf(TempBuffer, sizeof(TempBuffer), "%.6f", number); 00270 UnityPrint(TempBuffer); 00271 } 00272 #endif 00273 00274 /*-----------------------------------------------*/ 00275 00276 void UnityPrintFail(void); 00277 void UnityPrintFail(void) 00278 { 00279 UnityPrint(UnityStrFail); 00280 } 00281 00282 void UnityPrintOk(void); 00283 void UnityPrintOk(void) 00284 { 00285 UnityPrint(UnityStrOk); 00286 } 00287 00288 /*-----------------------------------------------*/ 00289 static void UnityTestResultsBegin(const char* file, const UNITY_LINE_TYPE line); 00290 static void UnityTestResultsBegin(const char* file, const UNITY_LINE_TYPE line) 00291 { 00292 #ifndef UNITY_FIXTURES 00293 UnityPrint(file); 00294 UNITY_OUTPUT_CHAR(':'); 00295 UnityPrintNumber((_U_SINT)line); 00296 UNITY_OUTPUT_CHAR(':'); 00297 UnityPrint(Unity.CurrentTestName); 00298 UNITY_OUTPUT_CHAR(':'); 00299 #else 00300 UNITY_UNUSED(file); 00301 UNITY_UNUSED(line); 00302 #endif 00303 } 00304 00305 /* SA_PATCH: Make failures more noticable. */ 00306 static void UnityPrintVisibleFailure(void); 00307 static void UnityPrintVisibleFailure(void) 00308 { 00309 UNITY_OUTPUT_CHAR('\n'); 00310 UnityPrint("===!!!===> "); 00311 /* SA_PATCH: Output results using easy to parse tags. */ 00312 UnityPrint(UNITY_RESULTS_TAGS_RESULT_START); 00313 UnityPrint(UnityStrFail); 00314 /* SA_PATCH: Output results using easy to parse tags. */ 00315 UnityPrint(UNITY_RESULTS_TAGS_RESULT_END); 00316 UnityPrint(" <===!!!==="); 00317 } 00318 /*-----------------------------------------------*/ 00319 static void UnityTestResultsFailBegin(const UNITY_LINE_TYPE line); 00320 static void UnityTestResultsFailBegin(const UNITY_LINE_TYPE line) 00321 { 00322 #ifndef UNITY_FIXTURES 00323 UnityTestResultsBegin(Unity.TestFile, line); 00324 #else 00325 UNITY_UNUSED(line); 00326 #endif 00327 /* SA_PATCH: Make failures more noticable. */ 00328 UnityPrintVisibleFailure(); 00329 //UnityPrint(UnityStrFail); 00330 UNITY_OUTPUT_CHAR(':'); 00331 } 00332 00333 /*-----------------------------------------------*/ 00334 void UnityConcludeTest(void) 00335 { 00336 if (Unity.CurrentTestIgnored) 00337 { 00338 Unity.TestIgnores++; 00339 } 00340 else if (!Unity.CurrentTestFailed) 00341 { 00342 UnityTestResultsBegin(Unity.TestFile, Unity.CurrentTestLineNumber); 00343 UnityPrint(UnityStrPass); 00344 } 00345 else 00346 { 00347 Unity.TestFailures++; 00348 } 00349 00350 Unity.CurrentTestFailed = 0; 00351 Unity.CurrentTestIgnored = 0; 00352 UNITY_PRINT_EOL(); 00353 UNITY_FLUSH_CALL(); 00354 } 00355 00356 /*-----------------------------------------------*/ 00357 static void UnityAddMsgIfSpecified(const char* msg); 00358 static void UnityAddMsgIfSpecified(const char* msg) 00359 { 00360 if (msg) 00361 { 00362 UnityPrint(UnityStrSpacer); 00363 #ifndef UNITY_EXCLUDE_DETAILS 00364 if (Unity.CurrentDetail1) 00365 { 00366 UnityPrint(UnityStrDetail1Name); 00367 UnityPrint(Unity.CurrentDetail1); 00368 if (Unity.CurrentDetail2) 00369 { 00370 UnityPrint(UnityStrDetail2Name); 00371 UnityPrint(Unity.CurrentDetail2); 00372 } 00373 UnityPrint(UnityStrSpacer); 00374 } 00375 #endif 00376 UnityPrint(msg); 00377 } 00378 } 00379 00380 /*-----------------------------------------------*/ 00381 static void UnityPrintExpectedAndActualStrings(const char* expected, const char* actual); 00382 static void UnityPrintExpectedAndActualStrings(const char* expected, const char* actual) 00383 { 00384 UnityPrint(UnityStrExpected); 00385 if (expected != NULL) 00386 { 00387 UNITY_OUTPUT_CHAR('\''); 00388 UnityPrint(expected); 00389 UNITY_OUTPUT_CHAR('\''); 00390 } 00391 else 00392 { 00393 UnityPrint(UnityStrNull); 00394 } 00395 UnityPrint(UnityStrWas); 00396 if (actual != NULL) 00397 { 00398 UNITY_OUTPUT_CHAR('\''); 00399 UnityPrint(actual); 00400 UNITY_OUTPUT_CHAR('\''); 00401 } 00402 else 00403 { 00404 UnityPrint(UnityStrNull); 00405 } 00406 } 00407 00408 /*-----------------------------------------------*/ 00409 static void UnityPrintExpectedAndActualStringsLen(const char* expected, const char* actual, const _UU32 length) 00410 { 00411 UnityPrint(UnityStrExpected); 00412 if (expected != NULL) 00413 { 00414 UNITY_OUTPUT_CHAR('\''); 00415 UnityPrintLen(expected, length); 00416 UNITY_OUTPUT_CHAR('\''); 00417 } 00418 else 00419 { 00420 UnityPrint(UnityStrNull); 00421 } 00422 UnityPrint(UnityStrWas); 00423 if (actual != NULL) 00424 { 00425 UNITY_OUTPUT_CHAR('\''); 00426 UnityPrintLen(actual, length); 00427 UNITY_OUTPUT_CHAR('\''); 00428 } 00429 else 00430 { 00431 UnityPrint(UnityStrNull); 00432 } 00433 } 00434 00435 00436 00437 /*----------------------------------------------- 00438 * Assertion & Control Helpers 00439 *-----------------------------------------------*/ 00440 00441 static int UnityCheckArraysForNull(UNITY_INTERNAL_PTR expected, UNITY_INTERNAL_PTR actual, const UNITY_LINE_TYPE lineNumber, const char* msg) 00442 { 00443 /* return true if they are both NULL */ 00444 if ((expected == NULL) && (actual == NULL)) 00445 return 1; 00446 00447 /* throw error if just expected is NULL */ 00448 if (expected == NULL) 00449 { 00450 UnityTestResultsFailBegin(lineNumber); 00451 UnityPrint(UnityStrNullPointerForExpected); 00452 UnityAddMsgIfSpecified(msg); 00453 UNITY_FAIL_AND_BAIL; 00454 } 00455 00456 /* throw error if just actual is NULL */ 00457 if (actual == NULL) 00458 { 00459 UnityTestResultsFailBegin(lineNumber); 00460 UnityPrint(UnityStrNullPointerForActual); 00461 UnityAddMsgIfSpecified(msg); 00462 UNITY_FAIL_AND_BAIL; 00463 } 00464 00465 /* return false if neither is NULL */ 00466 return 0; 00467 } 00468 00469 /*----------------------------------------------- 00470 * Assertion Functions 00471 *-----------------------------------------------*/ 00472 00473 void UnityAssertBits(const _U_SINT mask, 00474 const _U_SINT expected, 00475 const _U_SINT actual, 00476 const char* msg, 00477 const UNITY_LINE_TYPE lineNumber) 00478 { 00479 UNITY_SKIP_EXECUTION; 00480 00481 if ((mask & expected) != (mask & actual)) 00482 { 00483 UnityTestResultsFailBegin(lineNumber); 00484 UnityPrint(UnityStrExpected); 00485 UnityPrintMask((_U_UINT)mask, (_U_UINT)expected); 00486 UnityPrint(UnityStrWas); 00487 UnityPrintMask((_U_UINT)mask, (_U_UINT)actual); 00488 UnityAddMsgIfSpecified(msg); 00489 UNITY_FAIL_AND_BAIL; 00490 } 00491 } 00492 00493 /*-----------------------------------------------*/ 00494 void UnityAssertEqualNumber(const _U_SINT expected, 00495 const _U_SINT actual, 00496 const char* msg, 00497 const UNITY_LINE_TYPE lineNumber, 00498 const UNITY_DISPLAY_STYLE_T style) 00499 { 00500 UNITY_SKIP_EXECUTION; 00501 00502 if (expected != actual) 00503 { 00504 UnityTestResultsFailBegin(lineNumber); 00505 UnityPrint(UnityStrExpected); 00506 UnityPrintNumberByStyle(expected, style); 00507 UnityPrint(UnityStrWas); 00508 UnityPrintNumberByStyle(actual, style); 00509 UnityAddMsgIfSpecified(msg); 00510 UNITY_FAIL_AND_BAIL; 00511 } 00512 } 00513 00514 #define UnityPrintPointlessAndBail() \ 00515 { \ 00516 UnityTestResultsFailBegin(lineNumber); \ 00517 UnityPrint(UnityStrPointless); \ 00518 UnityAddMsgIfSpecified(msg); \ 00519 UNITY_FAIL_AND_BAIL; } 00520 00521 /*-----------------------------------------------*/ 00522 void UnityAssertEqualIntArray(UNITY_INTERNAL_PTR expected, 00523 UNITY_INTERNAL_PTR actual, 00524 const _UU32 num_elements, 00525 const char* msg, 00526 const UNITY_LINE_TYPE lineNumber, 00527 const UNITY_DISPLAY_STYLE_T style) 00528 { 00529 _UU32 elements = num_elements; 00530 UNITY_INTERNAL_PTR ptr_exp = (UNITY_INTERNAL_PTR)expected; 00531 UNITY_INTERNAL_PTR ptr_act = (UNITY_INTERNAL_PTR)actual; 00532 00533 UNITY_SKIP_EXECUTION; 00534 00535 if (elements == 0) 00536 { 00537 UnityPrintPointlessAndBail(); 00538 } 00539 00540 if (UnityCheckArraysForNull((UNITY_INTERNAL_PTR)expected, (UNITY_INTERNAL_PTR)actual, lineNumber, msg) == 1) 00541 return; 00542 00543 /* If style is UNITY_DISPLAY_STYLE_INT, we'll fall into the default case rather than the INT16 or INT32 (etc) case 00544 * as UNITY_DISPLAY_STYLE_INT includes a flag for UNITY_DISPLAY_RANGE_AUTO, which the width-specific 00545 * variants do not. Therefore remove this flag. */ 00546 switch(style & (UNITY_DISPLAY_STYLE_T)(~UNITY_DISPLAY_RANGE_AUTO)) 00547 { 00548 case UNITY_DISPLAY_STYLE_HEX8: 00549 case UNITY_DISPLAY_STYLE_INT8: 00550 case UNITY_DISPLAY_STYLE_UINT8: 00551 while (elements--) 00552 { 00553 if (*(UNITY_PTR_ATTRIBUTE const _US8*)ptr_exp != *(UNITY_PTR_ATTRIBUTE const _US8*)ptr_act) 00554 { 00555 UnityTestResultsFailBegin(lineNumber); 00556 UnityPrint(UnityStrElement); 00557 UnityPrintNumberUnsigned(num_elements - elements - 1); 00558 UnityPrint(UnityStrExpected); 00559 UnityPrintNumberByStyle(*(UNITY_PTR_ATTRIBUTE const _US8*)ptr_exp, style); 00560 UnityPrint(UnityStrWas); 00561 UnityPrintNumberByStyle(*(UNITY_PTR_ATTRIBUTE const _US8*)ptr_act, style); 00562 UnityAddMsgIfSpecified(msg); 00563 UNITY_FAIL_AND_BAIL; 00564 } 00565 ptr_exp = (UNITY_INTERNAL_PTR)((_UP)ptr_exp + 1); 00566 ptr_act = (UNITY_INTERNAL_PTR)((_UP)ptr_act + 1); 00567 } 00568 break; 00569 case UNITY_DISPLAY_STYLE_HEX16: 00570 case UNITY_DISPLAY_STYLE_INT16: 00571 case UNITY_DISPLAY_STYLE_UINT16: 00572 while (elements--) 00573 { 00574 if (*(UNITY_PTR_ATTRIBUTE const _US16*)ptr_exp != *(UNITY_PTR_ATTRIBUTE const _US16*)ptr_act) 00575 { 00576 UnityTestResultsFailBegin(lineNumber); 00577 UnityPrint(UnityStrElement); 00578 UnityPrintNumberUnsigned(num_elements - elements - 1); 00579 UnityPrint(UnityStrExpected); 00580 UnityPrintNumberByStyle(*(UNITY_PTR_ATTRIBUTE const _US16*)ptr_exp, style); 00581 UnityPrint(UnityStrWas); 00582 UnityPrintNumberByStyle(*(UNITY_PTR_ATTRIBUTE const _US16*)ptr_act, style); 00583 UnityAddMsgIfSpecified(msg); 00584 UNITY_FAIL_AND_BAIL; 00585 } 00586 ptr_exp = (UNITY_INTERNAL_PTR)((_UP)ptr_exp + 2); 00587 ptr_act = (UNITY_INTERNAL_PTR)((_UP)ptr_act + 2); 00588 } 00589 break; 00590 #ifdef UNITY_SUPPORT_64 00591 case UNITY_DISPLAY_STYLE_HEX64: 00592 case UNITY_DISPLAY_STYLE_INT64: 00593 case UNITY_DISPLAY_STYLE_UINT64: 00594 while (elements--) 00595 { 00596 if (*(UNITY_PTR_ATTRIBUTE const _US64*)ptr_exp != *(UNITY_PTR_ATTRIBUTE const _US64*)ptr_act) 00597 { 00598 UnityTestResultsFailBegin(lineNumber); 00599 UnityPrint(UnityStrElement); 00600 UnityPrintNumberUnsigned(num_elements - elements - 1); 00601 UnityPrint(UnityStrExpected); 00602 UnityPrintNumberByStyle(*(UNITY_PTR_ATTRIBUTE const _US64*)ptr_exp, style); 00603 UnityPrint(UnityStrWas); 00604 UnityPrintNumberByStyle(*(UNITY_PTR_ATTRIBUTE const _US64*)ptr_act, style); 00605 UnityAddMsgIfSpecified(msg); 00606 UNITY_FAIL_AND_BAIL; 00607 } 00608 ptr_exp = (UNITY_INTERNAL_PTR)((_UP)ptr_exp + 8); 00609 ptr_act = (UNITY_INTERNAL_PTR)((_UP)ptr_act + 8); 00610 } 00611 break; 00612 #endif 00613 default: 00614 while (elements--) 00615 { 00616 if (*(UNITY_PTR_ATTRIBUTE const _US32*)ptr_exp != *(UNITY_PTR_ATTRIBUTE const _US32*)ptr_act) 00617 { 00618 UnityTestResultsFailBegin(lineNumber); 00619 UnityPrint(UnityStrElement); 00620 UnityPrintNumberUnsigned(num_elements - elements - 1); 00621 UnityPrint(UnityStrExpected); 00622 UnityPrintNumberByStyle(*(UNITY_PTR_ATTRIBUTE const _US32*)ptr_exp, style); 00623 UnityPrint(UnityStrWas); 00624 UnityPrintNumberByStyle(*(UNITY_PTR_ATTRIBUTE const _US32*)ptr_act, style); 00625 UnityAddMsgIfSpecified(msg); 00626 UNITY_FAIL_AND_BAIL; 00627 } 00628 ptr_exp = (UNITY_INTERNAL_PTR)((_UP)ptr_exp + 4); 00629 ptr_act = (UNITY_INTERNAL_PTR)((_UP)ptr_act + 4); 00630 } 00631 break; 00632 } 00633 } 00634 00635 /*-----------------------------------------------*/ 00636 #ifndef UNITY_EXCLUDE_FLOAT 00637 void UnityAssertEqualFloatArray(UNITY_PTR_ATTRIBUTE const _UF* expected, 00638 UNITY_PTR_ATTRIBUTE const _UF* actual, 00639 const _UU32 num_elements, 00640 const char* msg, 00641 const UNITY_LINE_TYPE lineNumber) 00642 { 00643 _UU32 elements = num_elements; 00644 UNITY_PTR_ATTRIBUTE const _UF* ptr_expected = expected; 00645 UNITY_PTR_ATTRIBUTE const _UF* ptr_actual = actual; 00646 _UF diff, tol; 00647 00648 UNITY_SKIP_EXECUTION; 00649 00650 if (elements == 0) 00651 { 00652 UnityPrintPointlessAndBail(); 00653 } 00654 00655 if (UnityCheckArraysForNull((UNITY_INTERNAL_PTR)expected, (UNITY_INTERNAL_PTR)actual, lineNumber, msg) == 1) 00656 return; 00657 00658 while (elements--) 00659 { 00660 diff = *ptr_expected - *ptr_actual; 00661 if (diff < 0.0f) 00662 diff = 0.0f - diff; 00663 tol = UNITY_FLOAT_PRECISION * *ptr_expected; 00664 if (tol < 0.0f) 00665 tol = 0.0f - tol; 00666 00667 /* This first part of this condition will catch any NaN or Infinite values */ 00668 if (isnan(diff) || isinf(diff) || (diff > tol)) 00669 { 00670 UnityTestResultsFailBegin(lineNumber); 00671 UnityPrint(UnityStrElement); 00672 UnityPrintNumberUnsigned(num_elements - elements - 1); 00673 #ifdef UNITY_FLOAT_VERBOSE 00674 UnityPrint(UnityStrExpected); 00675 UnityPrintFloat(*ptr_expected); 00676 UnityPrint(UnityStrWas); 00677 UnityPrintFloat(*ptr_actual); 00678 #else 00679 UnityPrint(UnityStrDelta); 00680 #endif 00681 UnityAddMsgIfSpecified(msg); 00682 UNITY_FAIL_AND_BAIL; 00683 } 00684 ptr_expected++; 00685 ptr_actual++; 00686 } 00687 } 00688 00689 /*-----------------------------------------------*/ 00690 void UnityAssertFloatsWithin(const _UF delta, 00691 const _UF expected, 00692 const _UF actual, 00693 const char* msg, 00694 const UNITY_LINE_TYPE lineNumber) 00695 { 00696 _UF diff = actual - expected; 00697 _UF pos_delta = delta; 00698 00699 UNITY_SKIP_EXECUTION; 00700 00701 if (diff < 0.0f) 00702 { 00703 diff = 0.0f - diff; 00704 } 00705 if (pos_delta < 0.0f) 00706 { 00707 pos_delta = 0.0f - pos_delta; 00708 } 00709 00710 /* This first part of this condition will catch any NaN or Infinite values */ 00711 if (isnan(diff) || isinf(diff) || (pos_delta < diff)) 00712 { 00713 UnityTestResultsFailBegin(lineNumber); 00714 #ifdef UNITY_FLOAT_VERBOSE 00715 UnityPrint(UnityStrExpected); 00716 UnityPrintFloat(expected); 00717 UnityPrint(UnityStrWas); 00718 UnityPrintFloat(actual); 00719 #else 00720 UnityPrint(UnityStrDelta); 00721 #endif 00722 UnityAddMsgIfSpecified(msg); 00723 UNITY_FAIL_AND_BAIL; 00724 } 00725 } 00726 00727 /*-----------------------------------------------*/ 00728 void UnityAssertFloatSpecial(const _UF actual, 00729 const char* msg, 00730 const UNITY_LINE_TYPE lineNumber, 00731 const UNITY_FLOAT_TRAIT_T style) 00732 { 00733 const char* trait_names[] = { UnityStrInf, UnityStrNegInf, UnityStrNaN, UnityStrDet }; 00734 _U_SINT should_be_trait = ((_U_SINT)style & 1); 00735 _U_SINT is_trait = !should_be_trait; 00736 _U_SINT trait_index = (_U_SINT)(style >> 1); 00737 00738 UNITY_SKIP_EXECUTION; 00739 00740 switch(style) 00741 { 00742 /* To determine Inf / Neg Inf, we compare to an Inf / Neg Inf value we create on the fly 00743 * We are using a variable to hold the zero value because some compilers complain about dividing by zero otherwise */ 00744 case UNITY_FLOAT_IS_INF: 00745 case UNITY_FLOAT_IS_NOT_INF: 00746 is_trait = isinf(actual) & ispos(actual); 00747 break; 00748 case UNITY_FLOAT_IS_NEG_INF: 00749 case UNITY_FLOAT_IS_NOT_NEG_INF: 00750 is_trait = isinf(actual) & isneg(actual); 00751 break; 00752 00753 /* NaN is the only floating point value that does NOT equal itself. Therefore if Actual == Actual, then it is NOT NaN. */ 00754 case UNITY_FLOAT_IS_NAN: 00755 case UNITY_FLOAT_IS_NOT_NAN: 00756 is_trait = isnan(actual); 00757 break; 00758 00759 /* A determinate number is non infinite and not NaN. (therefore the opposite of the two above) */ 00760 case UNITY_FLOAT_IS_DET: 00761 case UNITY_FLOAT_IS_NOT_DET: 00762 if (isinf(actual) | isnan(actual)) 00763 is_trait = 0; 00764 else 00765 is_trait = 1; 00766 break; 00767 00768 default: 00769 trait_index = 0; 00770 trait_names[0] = UnityStrInvalidFloatTrait; 00771 break; 00772 } 00773 00774 if (is_trait != should_be_trait) 00775 { 00776 UnityTestResultsFailBegin(lineNumber); 00777 UnityPrint(UnityStrExpected); 00778 if (!should_be_trait) 00779 UnityPrint(UnityStrNot); 00780 UnityPrint(trait_names[trait_index]); 00781 UnityPrint(UnityStrWas); 00782 #ifdef UNITY_FLOAT_VERBOSE 00783 UnityPrintFloat(actual); 00784 #else 00785 if (should_be_trait) 00786 UnityPrint(UnityStrNot); 00787 UnityPrint(trait_names[trait_index]); 00788 #endif 00789 UnityAddMsgIfSpecified(msg); 00790 UNITY_FAIL_AND_BAIL; 00791 } 00792 } 00793 00794 #endif /* not UNITY_EXCLUDE_FLOAT */ 00795 00796 /*-----------------------------------------------*/ 00797 #ifndef UNITY_EXCLUDE_DOUBLE 00798 void UnityAssertEqualDoubleArray(UNITY_PTR_ATTRIBUTE const _UD* expected, 00799 UNITY_PTR_ATTRIBUTE const _UD* actual, 00800 const _UU32 num_elements, 00801 const char* msg, 00802 const UNITY_LINE_TYPE lineNumber) 00803 { 00804 _UU32 elements = num_elements; 00805 UNITY_PTR_ATTRIBUTE const _UD* ptr_expected = expected; 00806 UNITY_PTR_ATTRIBUTE const _UD* ptr_actual = actual; 00807 _UD diff, tol; 00808 00809 UNITY_SKIP_EXECUTION; 00810 00811 if (elements == 0) 00812 { 00813 UnityPrintPointlessAndBail(); 00814 } 00815 00816 if (UnityCheckArraysForNull((UNITY_INTERNAL_PTR)expected, (UNITY_INTERNAL_PTR)actual, lineNumber, msg) == 1) 00817 return; 00818 00819 while (elements--) 00820 { 00821 diff = *ptr_expected - *ptr_actual; 00822 if (diff < 0.0) 00823 diff = 0.0 - diff; 00824 tol = UNITY_DOUBLE_PRECISION * *ptr_expected; 00825 if (tol < 0.0) 00826 tol = 0.0 - tol; 00827 00828 /* This first part of this condition will catch any NaN or Infinite values */ 00829 if (isnan(diff) || isinf(diff) || (diff > tol)) 00830 { 00831 UnityTestResultsFailBegin(lineNumber); 00832 UnityPrint(UnityStrElement); 00833 UnityPrintNumberUnsigned(num_elements - elements - 1); 00834 #ifdef UNITY_DOUBLE_VERBOSE 00835 UnityPrint(UnityStrExpected); 00836 UnityPrintFloat((float)(*ptr_expected)); 00837 UnityPrint(UnityStrWas); 00838 UnityPrintFloat((float)(*ptr_actual)); 00839 #else 00840 UnityPrint(UnityStrDelta); 00841 #endif 00842 UnityAddMsgIfSpecified(msg); 00843 UNITY_FAIL_AND_BAIL; 00844 } 00845 ptr_expected++; 00846 ptr_actual++; 00847 } 00848 } 00849 00850 /*-----------------------------------------------*/ 00851 void UnityAssertDoublesWithin(const _UD delta, 00852 const _UD expected, 00853 const _UD actual, 00854 const char* msg, 00855 const UNITY_LINE_TYPE lineNumber) 00856 { 00857 _UD diff = actual - expected; 00858 _UD pos_delta = delta; 00859 00860 UNITY_SKIP_EXECUTION; 00861 00862 if (diff < 0.0) 00863 { 00864 diff = 0.0 - diff; 00865 } 00866 if (pos_delta < 0.0) 00867 { 00868 pos_delta = 0.0 - pos_delta; 00869 } 00870 00871 /* This first part of this condition will catch any NaN or Infinite values */ 00872 if (isnan(diff) || isinf(diff) || (pos_delta < diff)) 00873 { 00874 UnityTestResultsFailBegin(lineNumber); 00875 #ifdef UNITY_DOUBLE_VERBOSE 00876 UnityPrint(UnityStrExpected); 00877 UnityPrintFloat((float)expected); 00878 UnityPrint(UnityStrWas); 00879 UnityPrintFloat((float)actual); 00880 #else 00881 UnityPrint(UnityStrDelta); 00882 #endif 00883 UnityAddMsgIfSpecified(msg); 00884 UNITY_FAIL_AND_BAIL; 00885 } 00886 } 00887 00888 /*-----------------------------------------------*/ 00889 00890 void UnityAssertDoubleSpecial(const _UD actual, 00891 const char* msg, 00892 const UNITY_LINE_TYPE lineNumber, 00893 const UNITY_FLOAT_TRAIT_T style) 00894 { 00895 const char* trait_names[] = { UnityStrInf, UnityStrNegInf, UnityStrNaN, UnityStrDet }; 00896 _U_SINT should_be_trait = ((_U_SINT)style & 1); 00897 _U_SINT is_trait = !should_be_trait; 00898 _U_SINT trait_index = (_U_SINT)(style >> 1); 00899 00900 UNITY_SKIP_EXECUTION; 00901 00902 switch(style) 00903 { 00904 /* To determine Inf / Neg Inf, we compare to an Inf / Neg Inf value we create on the fly 00905 * We are using a variable to hold the zero value because some compilers complain about dividing by zero otherwise */ 00906 case UNITY_FLOAT_IS_INF: 00907 case UNITY_FLOAT_IS_NOT_INF: 00908 is_trait = isinf(actual) & ispos(actual); 00909 break; 00910 case UNITY_FLOAT_IS_NEG_INF: 00911 case UNITY_FLOAT_IS_NOT_NEG_INF: 00912 is_trait = isinf(actual) & isneg(actual); 00913 break; 00914 00915 /* NaN is the only floating point value that does NOT equal itself. Therefore if Actual == Actual, then it is NOT NaN. */ 00916 case UNITY_FLOAT_IS_NAN: 00917 case UNITY_FLOAT_IS_NOT_NAN: 00918 is_trait = isnan(actual); 00919 break; 00920 00921 /* A determinate number is non infinite and not NaN. (therefore the opposite of the two above) */ 00922 case UNITY_FLOAT_IS_DET: 00923 case UNITY_FLOAT_IS_NOT_DET: 00924 if (isinf(actual) | isnan(actual)) 00925 is_trait = 0; 00926 else 00927 is_trait = 1; 00928 break; 00929 00930 default: 00931 trait_index = 0; 00932 trait_names[0] = UnityStrInvalidFloatTrait; 00933 break; 00934 } 00935 00936 if (is_trait != should_be_trait) 00937 { 00938 UnityTestResultsFailBegin(lineNumber); 00939 UnityPrint(UnityStrExpected); 00940 if (!should_be_trait) 00941 UnityPrint(UnityStrNot); 00942 UnityPrint(trait_names[trait_index]); 00943 UnityPrint(UnityStrWas); 00944 #ifdef UNITY_DOUBLE_VERBOSE 00945 UnityPrintFloat(actual); 00946 #else 00947 if (should_be_trait) 00948 UnityPrint(UnityStrNot); 00949 UnityPrint(trait_names[trait_index]); 00950 #endif 00951 UnityAddMsgIfSpecified(msg); 00952 UNITY_FAIL_AND_BAIL; 00953 } 00954 } 00955 00956 00957 #endif /* not UNITY_EXCLUDE_DOUBLE */ 00958 00959 /*-----------------------------------------------*/ 00960 void UnityAssertNumbersWithin( const _U_UINT delta, 00961 const _U_SINT expected, 00962 const _U_SINT actual, 00963 const char* msg, 00964 const UNITY_LINE_TYPE lineNumber, 00965 const UNITY_DISPLAY_STYLE_T style) 00966 { 00967 UNITY_SKIP_EXECUTION; 00968 00969 if ((style & UNITY_DISPLAY_RANGE_INT) == UNITY_DISPLAY_RANGE_INT) 00970 { 00971 if (actual > expected) 00972 Unity.CurrentTestFailed = ((_U_UINT)(actual - expected) > delta); 00973 else 00974 Unity.CurrentTestFailed = ((_U_UINT)(expected - actual) > delta); 00975 } 00976 else 00977 { 00978 if ((_U_UINT)actual > (_U_UINT)expected) 00979 Unity.CurrentTestFailed = ((_U_UINT)(actual - expected) > delta); 00980 else 00981 Unity.CurrentTestFailed = ((_U_UINT)(expected - actual) > delta); 00982 } 00983 00984 if (Unity.CurrentTestFailed) 00985 { 00986 UnityTestResultsFailBegin(lineNumber); 00987 UnityPrint(UnityStrDelta); 00988 UnityPrintNumberByStyle((_U_SINT)delta, style); 00989 UnityPrint(UnityStrExpected); 00990 UnityPrintNumberByStyle(expected, style); 00991 UnityPrint(UnityStrWas); 00992 UnityPrintNumberByStyle(actual, style); 00993 UnityAddMsgIfSpecified(msg); 00994 UNITY_FAIL_AND_BAIL; 00995 } 00996 } 00997 00998 /*-----------------------------------------------*/ 00999 void UnityAssertEqualString(const char* expected, 01000 const char* actual, 01001 const char* msg, 01002 const UNITY_LINE_TYPE lineNumber) 01003 { 01004 _UU32 i; 01005 01006 UNITY_SKIP_EXECUTION; 01007 01008 /* if both pointers not null compare the strings */ 01009 if (expected && actual) 01010 { 01011 for (i = 0; expected[i] || actual[i]; i++) 01012 { 01013 if (expected[i] != actual[i]) 01014 { 01015 Unity.CurrentTestFailed = 1; 01016 break; 01017 } 01018 } 01019 } 01020 else 01021 { /* handle case of one pointers being null (if both null, test should pass) */ 01022 if (expected != actual) 01023 { 01024 Unity.CurrentTestFailed = 1; 01025 } 01026 } 01027 01028 if (Unity.CurrentTestFailed) 01029 { 01030 UnityTestResultsFailBegin(lineNumber); 01031 UnityPrintExpectedAndActualStrings(expected, actual); 01032 UnityAddMsgIfSpecified(msg); 01033 UNITY_FAIL_AND_BAIL; 01034 } 01035 } 01036 01037 /*-----------------------------------------------*/ 01038 void UnityAssertEqualStringLen(const char* expected, 01039 const char* actual, 01040 const _UU32 length, 01041 const char* msg, 01042 const UNITY_LINE_TYPE lineNumber) 01043 { 01044 _UU32 i; 01045 01046 UNITY_SKIP_EXECUTION; 01047 01048 /* if both pointers not null compare the strings */ 01049 if (expected && actual) 01050 { 01051 for (i = 0; (expected[i] || actual[i]) && i < length; i++) 01052 { 01053 if (expected[i] != actual[i]) 01054 { 01055 Unity.CurrentTestFailed = 1; 01056 break; 01057 } 01058 } 01059 } 01060 else 01061 { /* handle case of one pointers being null (if both null, test should pass) */ 01062 if (expected != actual) 01063 { 01064 Unity.CurrentTestFailed = 1; 01065 } 01066 } 01067 01068 if (Unity.CurrentTestFailed) 01069 { 01070 UnityTestResultsFailBegin(lineNumber); 01071 UnityPrintExpectedAndActualStringsLen(expected, actual, length); 01072 UnityAddMsgIfSpecified(msg); 01073 UNITY_FAIL_AND_BAIL; 01074 } 01075 } 01076 01077 01078 /*-----------------------------------------------*/ 01079 void UnityAssertEqualStringArray( const char** expected, 01080 const char** actual, 01081 const _UU32 num_elements, 01082 const char* msg, 01083 const UNITY_LINE_TYPE lineNumber) 01084 { 01085 _UU32 i, j = 0; 01086 01087 UNITY_SKIP_EXECUTION; 01088 01089 /* if no elements, it's an error */ 01090 if (num_elements == 0) 01091 { 01092 UnityPrintPointlessAndBail(); 01093 } 01094 01095 if (UnityCheckArraysForNull((UNITY_INTERNAL_PTR)expected, (UNITY_INTERNAL_PTR)actual, lineNumber, msg) == 1) 01096 return; 01097 01098 do 01099 { 01100 /* if both pointers not null compare the strings */ 01101 if (expected[j] && actual[j]) 01102 { 01103 for (i = 0; expected[j][i] || actual[j][i]; i++) 01104 { 01105 if (expected[j][i] != actual[j][i]) 01106 { 01107 Unity.CurrentTestFailed = 1; 01108 break; 01109 } 01110 } 01111 } 01112 else 01113 { /* handle case of one pointers being null (if both null, test should pass) */ 01114 if (expected[j] != actual[j]) 01115 { 01116 Unity.CurrentTestFailed = 1; 01117 } 01118 } 01119 01120 if (Unity.CurrentTestFailed) 01121 { 01122 UnityTestResultsFailBegin(lineNumber); 01123 if (num_elements > 1) 01124 { 01125 UnityPrint(UnityStrElement); 01126 UnityPrintNumberUnsigned(j); 01127 } 01128 UnityPrintExpectedAndActualStrings((const char*)(expected[j]), (const char*)(actual[j])); 01129 UnityAddMsgIfSpecified(msg); 01130 UNITY_FAIL_AND_BAIL; 01131 } 01132 } while (++j < num_elements); 01133 } 01134 01135 /*-----------------------------------------------*/ 01136 void UnityAssertEqualMemory( UNITY_INTERNAL_PTR expected, 01137 UNITY_INTERNAL_PTR actual, 01138 const _UU32 length, 01139 const _UU32 num_elements, 01140 const char* msg, 01141 const UNITY_LINE_TYPE lineNumber) 01142 { 01143 UNITY_PTR_ATTRIBUTE const unsigned char* ptr_exp = (UNITY_PTR_ATTRIBUTE const unsigned char*)expected; 01144 UNITY_PTR_ATTRIBUTE const unsigned char* ptr_act = (UNITY_PTR_ATTRIBUTE const unsigned char*)actual; 01145 _UU32 elements = num_elements; 01146 _UU32 bytes; 01147 01148 UNITY_SKIP_EXECUTION; 01149 01150 if ((elements == 0) || (length == 0)) 01151 { 01152 UnityPrintPointlessAndBail(); 01153 } 01154 01155 if (UnityCheckArraysForNull((UNITY_INTERNAL_PTR)expected, (UNITY_INTERNAL_PTR)actual, lineNumber, msg) == 1) 01156 return; 01157 01158 while (elements--) 01159 { 01160 /* /////////////////////////////////// */ 01161 bytes = length; 01162 while (bytes--) 01163 { 01164 if (*ptr_exp != *ptr_act) 01165 { 01166 UnityTestResultsFailBegin(lineNumber); 01167 UnityPrint(UnityStrMemory); 01168 if (num_elements > 1) 01169 { 01170 UnityPrint(UnityStrElement); 01171 UnityPrintNumberUnsigned(num_elements - elements - 1); 01172 } 01173 UnityPrint(UnityStrByte); 01174 UnityPrintNumberUnsigned(length - bytes - 1); 01175 UnityPrint(UnityStrExpected); 01176 UnityPrintNumberByStyle(*ptr_exp, UNITY_DISPLAY_STYLE_HEX8); 01177 UnityPrint(UnityStrWas); 01178 UnityPrintNumberByStyle(*ptr_act, UNITY_DISPLAY_STYLE_HEX8); 01179 UnityAddMsgIfSpecified(msg); 01180 UNITY_FAIL_AND_BAIL; 01181 } 01182 ptr_exp = (UNITY_INTERNAL_PTR)((_UP)ptr_exp + 1); 01183 ptr_act = (UNITY_INTERNAL_PTR)((_UP)ptr_act + 1); 01184 } 01185 /* /////////////////////////////////// */ 01186 01187 } 01188 } 01189 01190 /*----------------------------------------------- 01191 * Control Functions 01192 *-----------------------------------------------*/ 01193 01194 void UnityFail(const char* msg, const UNITY_LINE_TYPE line) 01195 { 01196 UNITY_SKIP_EXECUTION; 01197 01198 UnityTestResultsBegin(Unity.TestFile, line); 01199 /* SA_PATCH: Make failures more noticable. */ 01200 UnityPrintVisibleFailure(); 01201 //UnityPrintFail(); 01202 if (msg != NULL) 01203 { 01204 UNITY_OUTPUT_CHAR(':'); 01205 01206 #ifndef UNITY_EXCLUDE_DETAILS 01207 if (Unity.CurrentDetail1) 01208 { 01209 UnityPrint(UnityStrDetail1Name); 01210 UnityPrint(Unity.CurrentDetail1); 01211 if (Unity.CurrentDetail2) 01212 { 01213 UnityPrint(UnityStrDetail2Name); 01214 UnityPrint(Unity.CurrentDetail2); 01215 } 01216 UnityPrint(UnityStrSpacer); 01217 } 01218 #endif 01219 if (msg[0] != ' ') 01220 { 01221 UNITY_OUTPUT_CHAR(' '); 01222 } 01223 UnityPrint(msg); 01224 } 01225 01226 UNITY_FAIL_AND_BAIL; 01227 } 01228 01229 /*-----------------------------------------------*/ 01230 void UnityIgnore(const char* msg, const UNITY_LINE_TYPE line) 01231 { 01232 UNITY_SKIP_EXECUTION; 01233 01234 UnityTestResultsBegin(Unity.TestFile, line); 01235 UnityPrint(UnityStrIgnore); 01236 if (msg != NULL) 01237 { 01238 UNITY_OUTPUT_CHAR(':'); 01239 UNITY_OUTPUT_CHAR(' '); 01240 UnityPrint(msg); 01241 } 01242 UNITY_IGNORE_AND_BAIL; 01243 } 01244 01245 /*-----------------------------------------------*/ 01246 #if defined(UNITY_WEAK_ATTRIBUTE) 01247 UNITY_WEAK_ATTRIBUTE void setUp(void) { } 01248 UNITY_WEAK_ATTRIBUTE void tearDown(void) { } 01249 #elif defined(UNITY_WEAK_PRAGMA) 01250 # pragma weak setUp 01251 void setUp(void) { } 01252 # pragma weak tearDown 01253 void tearDown(void) { } 01254 #endif 01255 /*-----------------------------------------------*/ 01256 void UnityDefaultTestRun(UnityTestFunction Func, const char* FuncName, const int FuncLineNum) 01257 { 01258 Unity.CurrentTestName = FuncName; 01259 Unity.CurrentTestLineNumber = (UNITY_LINE_TYPE)FuncLineNum; 01260 Unity.NumberOfTests++; 01261 UNITY_CLR_DETAILS(); 01262 if (TEST_PROTECT()) 01263 { 01264 setUp(); 01265 Func(); 01266 } 01267 if (TEST_PROTECT() && !(Unity.CurrentTestIgnored)) 01268 { 01269 tearDown(); 01270 } 01271 UnityConcludeTest(); 01272 } 01273 01274 /*-----------------------------------------------*/ 01275 void UnityBegin(const char* filename) 01276 { 01277 Unity.TestFile = filename; 01278 Unity.CurrentTestName = NULL; 01279 Unity.CurrentTestLineNumber = 0; 01280 Unity.NumberOfTests = 0; 01281 Unity.TestFailures = 0; 01282 Unity.TestIgnores = 0; 01283 Unity.CurrentTestFailed = 0; 01284 Unity.CurrentTestIgnored = 0; 01285 01286 UNITY_CLR_DETAILS(); 01287 UNITY_OUTPUT_START(); 01288 } 01289 01290 /*-----------------------------------------------*/ 01291 int UnityEnd(void) 01292 { 01293 UNITY_PRINT_EOL(); 01294 UnityPrint(UnityStrBreaker); 01295 UNITY_PRINT_EOL(); 01296 UnityPrintNumber((_U_SINT)(Unity.NumberOfTests)); 01297 UnityPrint(UnityStrResultsTests); 01298 UnityPrintNumber((_U_SINT)(Unity.TestFailures)); 01299 UnityPrint(UnityStrResultsFailures); 01300 UnityPrintNumber((_U_SINT)(Unity.TestIgnores)); 01301 UnityPrint(UnityStrResultsIgnored); 01302 UNITY_PRINT_EOL(); 01303 if (Unity.TestFailures == 0U) 01304 { 01305 UnityPrintOk(); 01306 } 01307 else 01308 { 01309 UnityPrintFail(); 01310 #ifdef UNITY_DIFFERENTIATE_FINAL_FAIL 01311 UNITY_OUTPUT_CHAR('E'); UNITY_OUTPUT_CHAR('D'); 01312 #endif 01313 } 01314 UNITY_PRINT_EOL(); 01315 UNITY_FLUSH_CALL(); 01316 UNITY_OUTPUT_COMPLETE(); 01317 return (int)(Unity.TestFailures); 01318 } 01319 01320 /*-----------------------------------------------*/
Generated on Tue Jul 12 2022 19:12:17 by
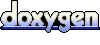